When to Use a Breadth-First Approach in Programming and Problem-Solving
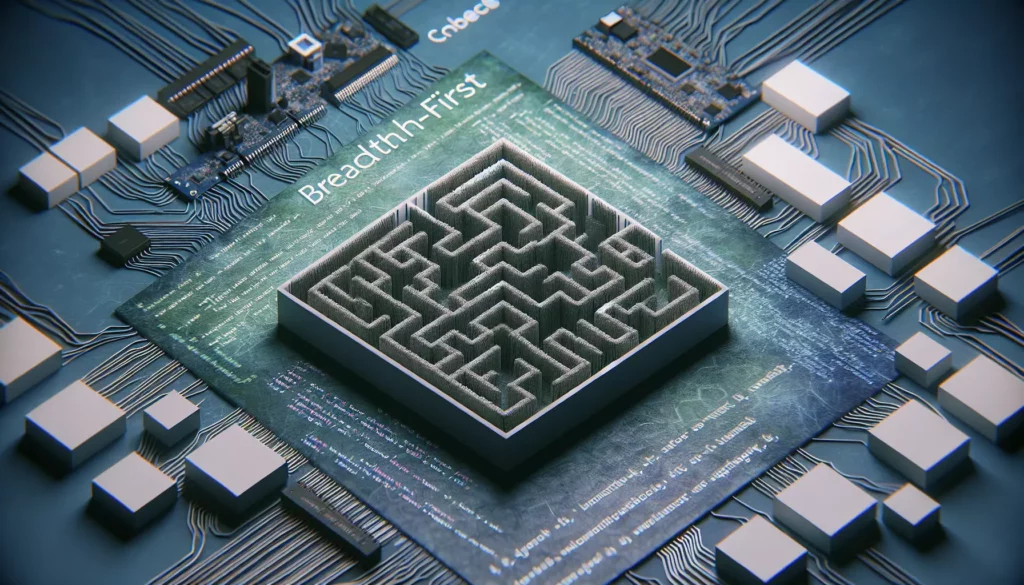
In the world of programming and algorithm design, choosing the right approach to solve a problem can make a significant difference in the efficiency and effectiveness of your solution. One such approach that often proves invaluable is the breadth-first approach. This method, while not always the go-to solution, can be incredibly powerful when applied in the right contexts. In this comprehensive guide, we’ll explore when and why you should consider using a breadth-first approach, its advantages, and practical examples to help you master this essential problem-solving technique.
Understanding the Breadth-First Approach
Before diving into when to use a breadth-first approach, let’s briefly recap what it entails. The breadth-first approach, often implemented as Breadth-First Search (BFS) in graph and tree traversal algorithms, is a strategy that explores all the neighbor nodes at the present depth before moving on to nodes at the next depth level.
In simpler terms, it’s like exploring a tree level by level, ensuring that you visit all nodes at one level before proceeding to the next. This contrasts with the depth-first approach, which explores as far as possible along each branch before backtracking.
Key Scenarios for Using a Breadth-First Approach
Now that we’ve refreshed our understanding of the breadth-first approach, let’s explore the scenarios where it shines:
1. Finding the Shortest Path in Unweighted Graphs
One of the most common and powerful applications of the breadth-first approach is in finding the shortest path between two nodes in an unweighted graph. Since BFS explores nodes level by level, it guarantees that the first time a node is discovered during the traversal, it is reached by the shortest path from the source.
This property makes BFS ideal for problems like:
- Finding the shortest route between two points in a maze
- Determining the minimum number of moves in puzzle games
- Calculating the shortest distance between two people in a social network
Here’s a simple example of using BFS to find the shortest path in a graph:
from collections import deque
def bfs_shortest_path(graph, start, goal):
queue = deque([[start]])
visited = set([start])
while queue:
path = queue.popleft()
node = path[-1]
if node == goal:
return path
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
new_path = list(path)
new_path.append(neighbor)
queue.append(new_path)
return None
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
shortest_path = bfs_shortest_path(graph, 'A', 'F')
print(f"Shortest path: {' -> '.join(shortest_path)}")
2. Level Order Traversal of Trees
When you need to process or print the nodes of a tree level by level, the breadth-first approach is your best friend. This is particularly useful in scenarios like:
- Visualizing the structure of a tree
- Finding the minimum depth of a binary tree
- Solving problems that require processing nodes based on their level in the tree
Here’s an example of level order traversal using BFS:
from collections import deque
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def levelOrder(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result
# Example usage
root = TreeNode(3)
root.left = TreeNode(9)
root.right = TreeNode(20)
root.right.left = TreeNode(15)
root.right.right = TreeNode(7)
print(levelOrder(root)) # Output: [[3], [9, 20], [15, 7]]
3. State Space Search in Puzzle Solving
Many puzzle-solving algorithms benefit from a breadth-first approach, especially when the goal is to find the solution with the minimum number of moves. This is because BFS guarantees finding the optimal solution in terms of the number of steps.
Examples of puzzles where BFS is effective include:
- Sliding puzzle games
- Word ladder problems
- Rubik’s cube solving
Here’s a simplified example of using BFS to solve a word ladder problem:
from collections import deque
def word_ladder(start, end, word_list):
word_set = set(word_list)
queue = deque([(start, [start])])
while queue:
word, path = queue.popleft()
if word == end:
return path
for i in range(len(word)):
for c in 'abcdefghijklmnopqrstuvwxyz':
next_word = word[:i] + c + word[i+1:]
if next_word in word_set:
word_set.remove(next_word)
queue.append((next_word, path + [next_word]))
return None
# Example usage
start = "hit"
end = "cog"
word_list = ["hot", "dot", "dog", "lot", "log", "cog"]
result = word_ladder(start, end, word_list)
print(f"Shortest transformation sequence: {' -> '.join(result) if result else 'No solution'}")
4. Finding All Nodes Within a Certain Distance
When you need to find all nodes within a specific distance from a starting point, BFS is an excellent choice. This is because it naturally expands outward from the starting point, level by level.
This approach is useful in scenarios such as:
- Finding all friends within a certain degree of separation in a social network
- Determining all locations reachable within a specific time or distance on a map
- Exploring game states within a certain number of moves
Here’s an example of finding all nodes within a certain distance in a graph:
from collections import deque
def nodes_within_distance(graph, start, max_distance):
queue = deque([(start, 0)])
visited = set([start])
result = []
while queue:
node, distance = queue.popleft()
if distance <= max_distance:
result.append(node)
if distance < max_distance:
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
queue.append((neighbor, distance + 1))
return result
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
nodes = nodes_within_distance(graph, 'A', 2)
print(f"Nodes within distance 2 from 'A': {', '.join(nodes)}")
5. Web Crawling and Link Analysis
In web crawling and link analysis, a breadth-first approach can be very effective. It allows you to explore web pages level by level, which is useful for:
- Discovering and indexing web pages
- Analyzing the structure of websites
- Finding the shortest path between two web pages
Here’s a simplified example of a web crawler using BFS:
import requests
from bs4 import BeautifulSoup
from collections import deque
from urllib.parse import urljoin
def web_crawler(start_url, max_pages):
queue = deque([start_url])
visited = set([start_url])
pages_crawled = 0
while queue and pages_crawled < max_pages:
url = queue.popleft()
pages_crawled += 1
print(f"Crawling: {url}")
try:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
href = link.get('href')
if href:
full_url = urljoin(url, href)
if full_url not in visited:
visited.add(full_url)
queue.append(full_url)
except Exception as e:
print(f"Error crawling {url}: {e}")
print(f"Crawled {pages_crawled} pages")
# Example usage
web_crawler('https://example.com', 10)
Advantages of the Breadth-First Approach
Now that we’ve explored various scenarios where the breadth-first approach excels, let’s summarize its key advantages:
- Optimal Solution Guarantee: In unweighted graphs or when each step has equal cost, BFS guarantees finding the optimal (shortest) solution.
- Level-wise Exploration: BFS explores nodes level by level, which is crucial for problems requiring level-order processing or finding nodes at a specific depth.
- Completeness: If a solution exists, BFS will find it, provided the branching factor is finite.
- Systematic Exploration: BFS provides a systematic way to explore all possibilities, which is useful in state space searches and puzzle solving.
- Memory Efficiency for Shallow Solutions: If the solution is not too deep in the search tree, BFS can be more memory-efficient than depth-first search.
Considerations and Limitations
While the breadth-first approach is powerful, it’s important to be aware of its limitations:
- Memory Usage: BFS can consume a lot of memory, especially for problems with a large branching factor or deep solutions.
- Not Suitable for Infinite Graphs: BFS may not terminate if applied to infinite graphs or trees.
- Inefficient for Deep Solutions: If the solution is very deep in the search tree, BFS might be slower compared to depth-first search.
- Overhead in Simple Linear Structures: For simple linear data structures, BFS might introduce unnecessary overhead compared to straightforward iteration.
Implementing Breadth-First Search Efficiently
To implement BFS efficiently, consider the following tips:
- Use Appropriate Data Structures: Utilize a queue (often implemented with collections.deque in Python) for efficient node exploration.
- Avoid Revisiting Nodes: Keep track of visited nodes to prevent unnecessary re-exploration.
- Consider Space Optimization: In memory-constrained environments, consider techniques like iterative deepening search, which combines the benefits of BFS and DFS.
- Leverage Problem-Specific Heuristics: When applicable, use domain-specific knowledge to guide the search more efficiently.
Here’s an example of an efficient BFS implementation for graph traversal:
from collections import deque
def efficient_bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS traversal:")
efficient_bfs(graph, 'A')
Real-World Applications of Breadth-First Approach
The breadth-first approach finds applications in various real-world scenarios:
- GPS and Navigation Systems: Finding the shortest route between two points.
- Social Network Analysis: Determining degrees of separation or finding mutual friends.
- Network Broadcast: Efficiently broadcasting messages in a network.
- AI and Game Development: Solving puzzles and implementing game AI for strategy games.
- Web Crawlers: Indexing web pages for search engines.
- Peer-to-Peer Networks: Finding the closest peers in a P2P network.
- Circuit Design: Analyzing connectivity in electrical circuits.
Comparing Breadth-First and Depth-First Approaches
To fully appreciate when to use a breadth-first approach, it’s helpful to compare it with its counterpart, the depth-first approach:
Aspect | Breadth-First | Depth-First |
---|---|---|
Memory Usage | Higher (stores all nodes at current level) | Lower (stores only nodes on current path) |
Completeness | Complete (finds solution if it exists) | Complete for finite graphs |
Optimality | Optimal for unweighted graphs | Not guaranteed to be optimal |
Time Complexity | O(V + E) for graphs | O(V + E) for graphs |
Space Complexity | O(V) worst case | O(h) where h is the height of the tree |
Best For | Shortest path, level-wise processing | Memory-constrained environments, deep graphs |
Conclusion
The breadth-first approach is a powerful tool in a programmer’s arsenal, particularly well-suited for scenarios involving shortest paths, level-wise processing, and systematic exploration of state spaces. Its ability to guarantee optimal solutions in unweighted graphs and provide a systematic exploration method makes it invaluable in many problem-solving contexts.
However, like any algorithmic approach, it’s crucial to understand its strengths and limitations. The higher memory usage of BFS can be a constraint in some scenarios, and for problems with deep solutions or in memory-constrained environments, alternatives like depth-first search might be more appropriate.
As you continue to develop your programming and problem-solving skills, practice recognizing situations where a breadth-first approach would be beneficial. Implement BFS in various contexts, experiment with optimizations, and compare it with other approaches to gain a deeper understanding of its capabilities and limitations.
Remember, the key to becoming a proficient programmer and problem solver is not just knowing various algorithms and approaches, but understanding when and how to apply them effectively. The breadth-first approach is a versatile tool that, when used appropriately, can lead to elegant and efficient solutions to a wide range of computational problems.