When to Use a Bottom-Up Approach in Programming and Problem Solving
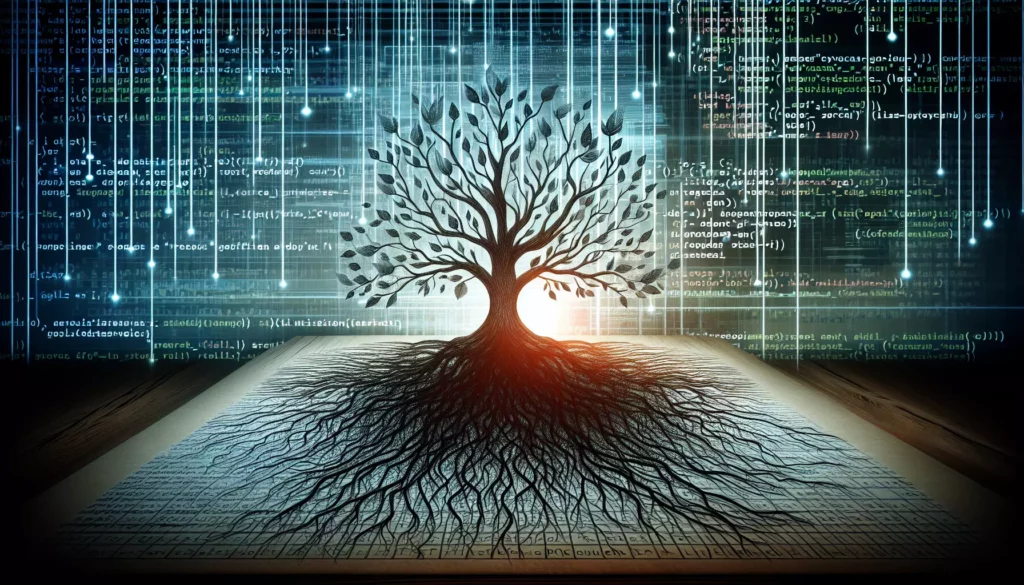
In the world of coding and algorithm design, there are various approaches to tackling complex problems. Two of the most common methodologies are the top-down and bottom-up approaches. While both have their merits, today we’ll focus on the bottom-up approach, exploring when it’s most effective and how it can be applied in programming and problem-solving scenarios.
Understanding the Bottom-Up Approach
Before diving into when to use a bottom-up approach, let’s first define what it means. The bottom-up approach is a method of problem-solving where you start with the smallest, most basic components of a problem and gradually build up to the complete solution. This is in contrast to the top-down approach, which begins with the overall problem and breaks it down into smaller sub-problems.
In programming terms, a bottom-up approach often involves:
- Starting with the most fundamental functions or classes
- Testing and perfecting these basic components
- Gradually combining these components to create more complex structures
- Building up to the final, complete solution
Scenarios Where Bottom-Up Shines
Now that we understand what the bottom-up approach entails, let’s explore scenarios where it’s particularly effective:
1. Dynamic Programming Problems
Dynamic programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It’s an area where the bottom-up approach often excels. Here’s why:
- Efficiency: Bottom-up dynamic programming often leads to more efficient solutions by avoiding redundant calculations.
- Tabulation: It naturally lends itself to tabulation (iterative) methods, which can be more intuitive and easier to implement than memoization (recursive) methods.
- Space Optimization: In many cases, a bottom-up approach allows for better space optimization.
Let’s look at a classic dynamic programming problem: calculating Fibonacci numbers.
def fibonacci_bottom_up(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
print(fibonacci_bottom_up(10)) # Output: 55
In this example, we start by calculating the smallest Fibonacci numbers and work our way up to the desired number. This approach is more efficient than a recursive top-down approach, especially for larger values of n.
2. System Design and Architecture
When designing complex systems or architectures, a bottom-up approach can be very effective. Here’s why:
- Modularity: It encourages the development of small, reusable components that can be thoroughly tested independently.
- Flexibility: As you build up from basic components, it’s easier to pivot or make changes without affecting the entire system.
- Parallel Development: Different team members can work on different components simultaneously.
For instance, when building a web application, you might start by developing individual components like user authentication, database interactions, and API endpoints before integrating them into a complete system.
3. Parsing and Compiler Design
In the realm of compiler design and parsing, bottom-up approaches are often preferred. Here’s why:
- Efficiency: Bottom-up parsers, like LR parsers, are generally more efficient than top-down parsers.
- Power: They can handle a wider range of grammars, including left-recursive grammars.
- Error Detection: Bottom-up parsers often provide better error detection and recovery mechanisms.
For example, when implementing a simple calculator, you might start by parsing individual numbers and operators before combining them into complex expressions.
4. Machine Learning Model Development
In machine learning, a bottom-up approach can be beneficial when developing complex models. Here’s how:
- Feature Engineering: Start by developing and testing individual features before combining them into more complex feature sets.
- Model Architecture: Begin with simple models and gradually increase complexity, adding layers or components as needed.
- Ensemble Methods: Build individual models and then combine them into ensemble models.
For instance, when developing a neural network for image classification, you might start with a simple convolutional layer, test its performance, and then gradually add more layers and complexity.
5. Testing and Debugging
The bottom-up approach is often invaluable in testing and debugging complex systems. Here’s why:
- Isolation: It allows you to isolate and fix issues at the component level before they propagate to higher levels.
- Confidence: By thoroughly testing each component, you can have more confidence in the overall system.
- Regression Prevention: It’s easier to prevent regression issues when you have a solid foundation of well-tested components.
For example, when debugging a large application, you might start by testing individual functions or classes before moving on to integration tests and full system tests.
Implementing Bottom-Up Approach: Best Practices
Now that we’ve explored when to use a bottom-up approach, let’s discuss some best practices for implementing it effectively:
1. Start with Clear Specifications
Even though you’re building from the bottom up, it’s crucial to have a clear understanding of the overall goal. This helps ensure that the components you’re building will ultimately fit together to solve the larger problem.
2. Emphasize Modularity
Design your components to be as modular as possible. This not only makes testing easier but also increases reusability and maintainability.
3. Test Thoroughly
One of the key advantages of the bottom-up approach is the ability to thoroughly test each component. Take full advantage of this by implementing comprehensive unit tests for each module.
4. Document as You Go
As you build each component, document its functionality, interfaces, and any important implementation details. This will make it easier to integrate components later and maintain the system in the long run.
5. Plan for Integration
While focusing on individual components, keep in mind how they will eventually fit together. Design your interfaces with integration in mind.
6. Be Flexible
As you build up your solution, you may discover better ways to structure your components or solve sub-problems. Be open to refactoring and adjusting your approach as you go.
Bottom-Up vs. Top-Down: A Comparison
While we’ve focused on the bottom-up approach, it’s worth comparing it to the top-down approach to understand their relative strengths and weaknesses:
Aspect | Bottom-Up | Top-Down |
---|---|---|
Starting Point | Smallest components | Overall problem |
Testing | Early and thorough component testing | Testing of complete or partial systems |
Flexibility | More flexible to changes | Less flexible, may require significant rework |
Visibility of Overall Structure | May be less clear initially | Clearer from the beginning |
Parallelization | Easier to parallelize development | May be more challenging to parallelize |
Risk Management | Risks at component level identified early | High-level risks identified early |
Real-World Examples of Bottom-Up Approach
To further illustrate the effectiveness of the bottom-up approach, let’s look at some real-world examples:
1. Linux Kernel Development
The development of the Linux kernel is a prime example of a bottom-up approach in action. The kernel started as a small, basic system and gradually grew into the complex, feature-rich operating system kernel we know today. Developers continually add new features and optimize existing ones, building upon the solid foundation laid by earlier work.
2. Agile Software Development
Agile methodologies often employ a bottom-up approach. Teams start by developing small, functional pieces of software and iteratively build upon them, gradually creating a complete product. This approach allows for frequent releases, continuous feedback, and the flexibility to adapt to changing requirements.
3. Machine Learning Libraries
Popular machine learning libraries like TensorFlow and PyTorch have been developed using a bottom-up approach. They started with basic tensor operations and gradually built up to include complex neural network architectures, optimization algorithms, and high-level APIs.
4. Web Development Frameworks
Many web development frameworks, such as React for frontend development, have evolved using a bottom-up approach. React started with the simple concept of components and gradually added features like state management, hooks, and server-side rendering.
Challenges and Limitations of the Bottom-Up Approach
While the bottom-up approach has many advantages, it’s important to be aware of its potential challenges and limitations:
1. Lack of Big Picture
When focusing on individual components, it can be easy to lose sight of the overall goal. This may lead to developing components that don’t quite fit together or miss crucial aspects of the larger problem.
2. Over-Engineering
There’s a risk of spending too much time perfecting low-level components that may not be critical to the overall solution. This can lead to inefficient use of development time and resources.
3. Integration Challenges
While individual components may work perfectly, integrating them into a cohesive system can be challenging. Issues may arise that weren’t apparent when working on isolated components.
4. Scalability Concerns
Solutions built from the bottom up may sometimes struggle with scalability. What works well for small-scale problems might not efficiently scale to larger, more complex scenarios.
5. Requirements Changes
If high-level requirements change significantly during development, a bottom-up approach may require substantial rework of already-developed components.
Combining Bottom-Up and Top-Down Approaches
In practice, many successful projects use a combination of bottom-up and top-down approaches. This hybrid strategy can leverage the strengths of both methodologies while mitigating their weaknesses.
Here’s how you might combine these approaches:
- Start with a high-level design (top-down) to establish the overall structure and goals of the project.
- Identify key components or modules that can be developed independently.
- Use a bottom-up approach to develop and thoroughly test these individual components.
- Continuously integrate these components into the larger system, refining the high-level design as needed.
- Iterate this process, moving between high-level design and low-level implementation as the project progresses.
This combined approach allows for both a clear overall vision and the flexibility to adapt and optimize at the component level.
Conclusion: Choosing the Right Approach
The bottom-up approach is a powerful tool in a programmer’s arsenal, particularly well-suited for scenarios involving dynamic programming, system design, parsing, machine learning model development, and testing and debugging. It promotes modularity, flexibility, and thorough testing of individual components.
However, like any methodology, it’s not a one-size-fits-all solution. The key is to understand the nature of your problem, the constraints of your project, and the strengths and weaknesses of different approaches. Often, the most effective strategy is to combine bottom-up and top-down methodologies, leveraging the strengths of each to create robust, efficient, and well-structured solutions.
As you continue to develop your programming skills, practice identifying situations where a bottom-up approach might be beneficial. Experiment with implementing solutions using this methodology, and pay attention to how it affects your development process and the quality of your final product. Remember, the goal is not to rigidly adhere to any single approach, but to have a variety of tools at your disposal and the wisdom to know when to use each one.
By mastering the bottom-up approach and understanding its applications, you’ll be better equipped to tackle complex programming challenges, design efficient algorithms, and create scalable, maintainable software systems. Happy coding!