When to Use a Backtracking Approach in Problem-Solving
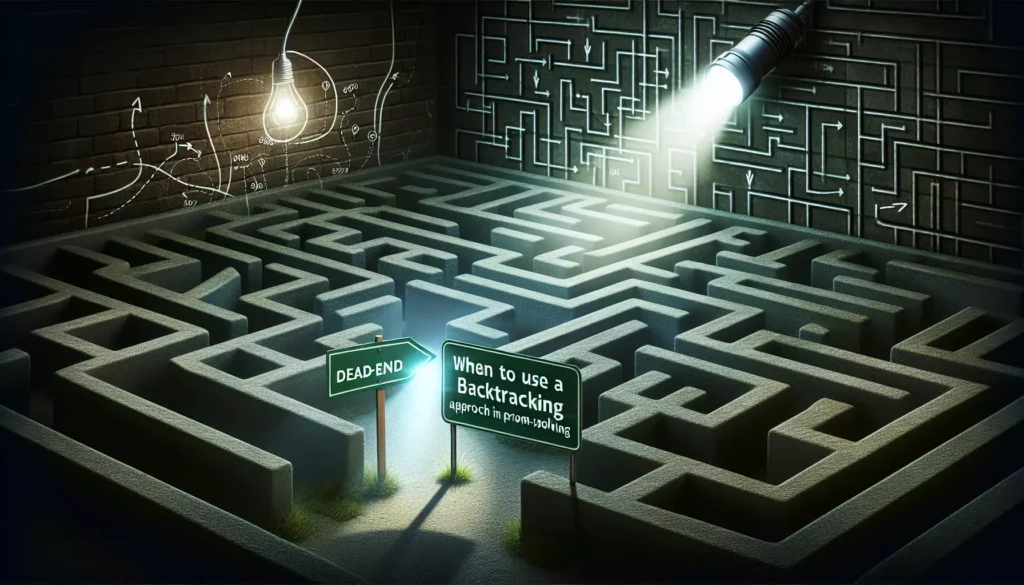
In the world of algorithmic problem-solving, backtracking stands out as a powerful technique that can crack some of the most challenging computational puzzles. But when exactly should you reach for this tool in your coding arsenal? This comprehensive guide will explore the ins and outs of backtracking, helping you recognize when it’s the right approach and how to implement it effectively.
Understanding Backtracking
Before we dive into when to use backtracking, let’s ensure we have a solid grasp of what it is. Backtracking is an algorithmic technique for solving problems recursively by trying to build a solution incrementally, one piece at a time, removing those solutions that fail to satisfy the constraints of the problem at any point of time (by time, here, is referred to the time elapsed till reaching any level of the search tree).
Essentially, backtracking is a refined brute force approach. It systematically searches for a solution to a problem among all available options. What sets it apart from simple brute force is its ability to abandon a path as soon as it determines that the path cannot lead to a viable solution. This “fail fast and fall back” approach can significantly reduce the number of possibilities to explore, making it more efficient than trying every single combination.
Key Characteristics of Problems Suitable for Backtracking
Certain problem characteristics make backtracking an ideal choice. Let’s explore these in detail:
1. Choice Points
Problems that involve making a series of choices are prime candidates for backtracking. At each step of the solution, you have multiple options to choose from. For example, in the classic N-Queens problem, you have a choice of where to place each queen on the chessboard.
2. Constraints
The problem should have clear constraints that limit the choices at each step. These constraints help prune the search tree, making the backtracking approach more efficient. In the Sudoku-solving problem, the constraints are the rules of Sudoku itself – no repeated numbers in rows, columns, or 3×3 grids.
3. Goal State
There should be a well-defined goal state or set of goal states. This allows the algorithm to recognize when it has found a valid solution. In a maze-solving problem, the goal state is reaching the exit of the maze.
4. Optimization Not Required
Backtracking is typically used to find all possible solutions or to find if a solution exists. It’s not inherently designed for optimization problems where you need to find the best solution among many. However, it can be adapted for such purposes with additional logic.
Common Problem Types Suited for Backtracking
Now that we understand the characteristics, let’s look at some common types of problems where backtracking shines:
1. Combinatorial Problems
These involve finding all possible combinations or arrangements of a set of elements. Examples include:
- Generating all possible subsets of a set
- Finding all permutations of a string
- Combination Sum problems
2. Constraint Satisfaction Problems
These problems require finding a solution that satisfies a set of constraints. Examples include:
- Sudoku
- N-Queens problem
- Map Coloring
3. Decision Problems
These involve determining whether a solution exists that meets certain criteria. Examples include:
- Maze solving
- Word Search in a grid
- Hamiltonian Path problem
Implementing Backtracking: A Step-by-Step Guide
Now that we know when to use backtracking, let’s look at how to implement it. The general structure of a backtracking algorithm typically follows these steps:
- Choose: Select a candidate for the solution.
- Constraint Check: Check if the candidate violates any constraints.
- Goal Check: If not, check if it’s a complete solution.
- Recurse or Backtrack: If it’s not a complete solution, make a recursive call to proceed further. If the recursive call fails, undo the current choice (backtrack) and try the next candidate.
Let’s implement this structure in Python with a classic example: generating all possible subsets of a given set.
def generate_subsets(nums):
def backtrack(start, current):
# Add the current subset to the result
result.append(current[:])
# Explore further possibilities
for i in range(start, len(nums)):
# Choose
current.append(nums[i])
# Recurse
backtrack(i + 1, current)
# Unchoose (backtrack)
current.pop()
result = []
backtrack(0, [])
return result
# Example usage
nums = [1, 2, 3]
subsets = generate_subsets(nums)
print(subsets)
# Output: [[], [1], [1, 2], [1, 2, 3], [1, 3], [2], [2, 3], [3]]
In this example, we’re generating all possible subsets of the given set. The backtracking occurs when we’ve added an element to our current subset, explored all possibilities with that element included, and then remove it to explore possibilities without it.
Optimizing Backtracking Algorithms
While backtracking is more efficient than brute force, it can still be slow for large problem sizes. Here are some strategies to optimize backtracking algorithms:
1. Pruning
Pruning involves eliminating choices early if you can determine they won’t lead to a solution. This can significantly reduce the search space. For example, in the N-Queens problem, once you place a queen, you can immediately eliminate all positions in the same row, column, and diagonals for subsequent queens.
2. Ordering
The order in which you explore choices can impact efficiency. Try to make choices that are more likely to lead to a solution first. In Sudoku, for instance, filling cells with fewer possible values first can lead to faster solutions.
3. Constraint Propagation
This involves using the constraints of the problem to deduce additional constraints. This can help reduce the search space further. In Sudoku, if you know a cell must be either 2 or 3, you can eliminate 2 and 3 as possibilities for other cells in the same row, column, and 3×3 grid.
4. Memoization
If your backtracking algorithm repeatedly solves the same subproblems, consider using memoization to store and reuse these solutions. This can transform an exponential-time algorithm into a polynomial-time one for certain problems.
Real-World Applications of Backtracking
Backtracking isn’t just a theoretical concept; it has numerous practical applications in computer science and beyond. Here are some areas where backtracking is commonly used:
1. Artificial Intelligence and Machine Learning
Backtracking is used in various AI algorithms, particularly in game-playing and puzzle-solving applications. For instance:
- Chess engines use backtracking to explore possible moves and their consequences.
- Constraint satisfaction problems in AI, such as scheduling and resource allocation, often employ backtracking.
2. Computer Networking
Backtracking is used in routing algorithms to find paths in networks. When a route is blocked or inefficient, the algorithm can backtrack to find alternative paths.
3. Compiler Design
Backtracking is used in the parsing phase of compilation, particularly for handling ambiguous grammars in programming languages.
4. Computational Biology
In bioinformatics, backtracking is used for tasks such as sequence alignment and protein folding prediction.
5. Operations Research
Many optimization problems in operations research, such as the traveling salesman problem, use backtracking as part of their solution strategies.
Common Pitfalls and How to Avoid Them
While backtracking is a powerful technique, it’s not without its challenges. Here are some common pitfalls and how to avoid them:
1. Infinite Recursion
If not implemented correctly, backtracking can lead to infinite recursion. Always ensure you have a base case and that you’re making progress towards it with each recursive call.
2. Memory Overflow
Deep recursion can lead to stack overflow errors. If you’re dealing with very large problem sizes, consider an iterative implementation or tail recursion optimization if your language supports it.
3. Inefficient Pruning
Not pruning the search space effectively can lead to unnecessary exploration of invalid paths. Spend time understanding the problem constraints and implement efficient pruning strategies.
4. Incorrect State Management
Failing to properly manage the state (e.g., not undoing changes when backtracking) can lead to incorrect solutions. Always ensure you’re restoring the state correctly when backtracking.
Backtracking vs. Other Algorithmic Approaches
While backtracking is powerful, it’s not always the best choice. Let’s compare it with some other algorithmic approaches:
Backtracking vs. Dynamic Programming
Dynamic Programming (DP) is often more efficient for optimization problems where subproblems overlap. While backtracking explores all possibilities, DP stores solutions to subproblems to avoid redundant computations.
Use backtracking when:
- You need to find all possible solutions
- The problem doesn’t have overlapping subproblems
- Memory usage is a concern (DP often requires more memory)
Use DP when:
- You need to find an optimal solution
- The problem has overlapping subproblems
- The problem exhibits optimal substructure
Backtracking vs. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, hoping to find a global optimum. They’re often faster but don’t always find the best solution.
Use backtracking when:
- You need to guarantee finding the best solution
- The problem doesn’t have the “greedy choice property”
Use greedy algorithms when:
- The problem has the “greedy choice property”
- A locally optimal choice leads to a globally optimal solution
- You need a fast, “good enough” solution
Advanced Backtracking Techniques
As you become more comfortable with basic backtracking, you can explore some advanced techniques to tackle more complex problems:
1. Bit Manipulation in Backtracking
For certain problems, especially those involving subsets or combinations, using bit manipulation can significantly speed up your backtracking algorithm. Instead of using arrays to keep track of choices, you can use bits in an integer.
Here’s an example of generating all subsets using bit manipulation:
def generate_subsets_bit(nums):
n = len(nums)
result = []
for i in range(1 << n):
subset = []
for j in range(n):
if i & (1 << j):
subset.append(nums[j])
result.append(subset)
return result
# Example usage
nums = [1, 2, 3]
subsets = generate_subsets_bit(nums)
print(subsets)
# Output: [[], [1], [2], [1, 2], [3], [1, 3], [2, 3], [1, 2, 3]]
2. Backtracking with Heuristics
For some problems, you can use heuristics to guide your backtracking search. This can help you find solutions faster by exploring more promising paths first. This technique is often used in AI search algorithms like A* search.
3. Parallel Backtracking
For large problem spaces, you can parallelize your backtracking algorithm to explore multiple paths simultaneously. This can significantly speed up the search process on multi-core processors.
4. Iterative Deepening
This technique combines the space-efficiency of depth-first search with the completeness of breadth-first search. It’s particularly useful when the solution depth is unknown.
Practice Problems to Hone Your Backtracking Skills
To truly master backtracking, practice is key. Here are some classic problems to help you hone your skills:
- N-Queens Problem: Place N queens on an NxN chessboard so that no two queens threaten each other.
- Sudoku Solver: Implement a program to solve Sudoku puzzles.
- Permutations: Generate all permutations of a given string.
- Combination Sum: Find all unique combinations in a set of candidates that sum to a target number.
- Word Break: Given a string and a dictionary of words, determine if the string can be segmented into a space-separated sequence of dictionary words.
- Palindrome Partitioning: Partition a string into substrings, each of which is a palindrome.
- Graph Coloring: Color the vertices of a graph such that no two adjacent vertices share the same color, using at most k colors.
- Hamiltonian Cycle: Determine if a graph contains a Hamiltonian cycle (a cycle that visits each vertex exactly once).
These problems cover a wide range of applications of backtracking and will help you develop a strong intuition for when and how to apply this powerful technique.
Conclusion
Backtracking is a versatile and powerful algorithmic technique that’s essential for solving a wide range of computational problems. By understanding when to use backtracking, how to implement it effectively, and how to optimize your algorithms, you’ll be well-equipped to tackle complex problems in computer science and beyond.
Remember, the key to mastering backtracking is practice. Start with simple problems and gradually work your way up to more complex ones. As you gain experience, you’ll develop an intuition for when backtracking is the right approach and how to implement it most effectively.
Whether you’re preparing for technical interviews, working on a challenging coding project, or simply looking to expand your problem-solving toolkit, backtracking is a technique that will serve you well. So keep practicing, keep exploring, and don’t be afraid to backtrack when you hit a dead end – in coding and in life!