When to Consider Using Bit Manipulation in Programming
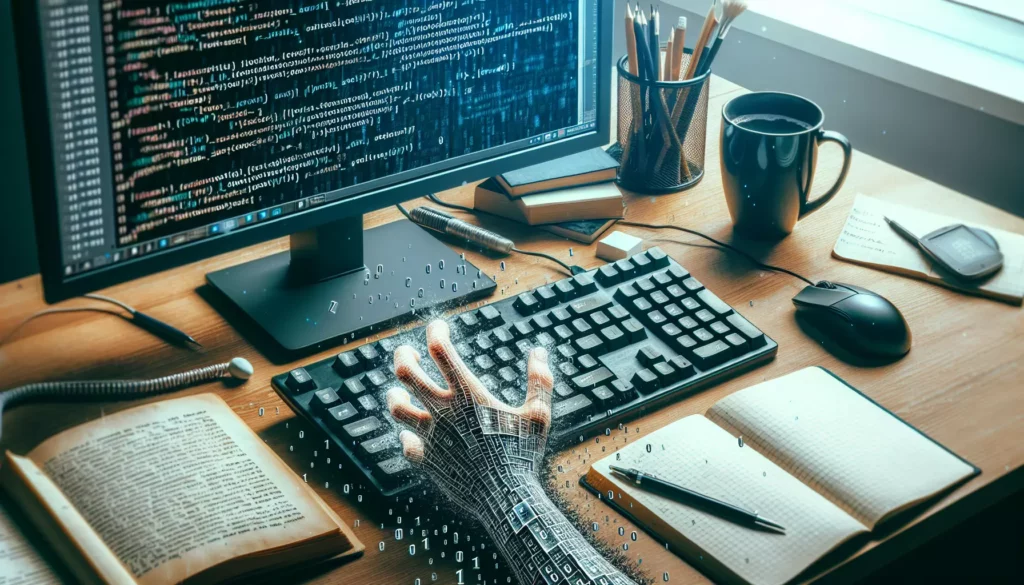
In the world of programming, efficiency and optimization are key factors that can set apart good code from great code. One technique that often flies under the radar but can provide significant performance improvements in certain scenarios is bit manipulation. This powerful tool in a programmer’s arsenal can lead to faster execution times and reduced memory usage when applied correctly. In this comprehensive guide, we’ll explore when and why you should consider using bit manipulation in your code, along with practical examples and best practices.
What is Bit Manipulation?
Before diving into the when and why, let’s briefly recap what bit manipulation entails. Bit manipulation involves the direct manipulation of individual bits within a computer’s memory. It leverages bitwise operators to perform operations on binary representations of data, allowing for low-level control and optimization.
The most common bitwise operators include:
- AND (&)
- OR (|)
- XOR (^)
- NOT (~)
- Left Shift (<<)
- Right Shift (>>)
These operators work directly on the binary representation of numbers, enabling efficient manipulation of data at the bit level.
When to Consider Bit Manipulation
While bit manipulation isn’t necessary for every programming task, there are several scenarios where it can provide significant advantages. Let’s explore some of the key situations where you should consider employing bit manipulation techniques:
1. Optimizing Space Usage
One of the primary reasons to use bit manipulation is to optimize space usage, especially when dealing with large datasets or memory-constrained environments. By using individual bits to represent boolean flags or small integer values, you can pack multiple pieces of information into a single integer, significantly reducing memory consumption.
For example, consider a scenario where you need to store information about various attributes of an object, each represented by a boolean value. Instead of using separate boolean variables, you can use a single integer and manipulate its bits to store this information:
// Using separate boolean variables
boolean isActive = true;
boolean isAdmin = false;
boolean hasPremiumAccess = true;
boolean isVerified = false;
// Using bit manipulation
int attributes = 0;
attributes |= (1 << 0); // Set isActive
attributes |= (1 << 2); // Set hasPremiumAccess
// Checking attributes
boolean isActive = (attributes & (1 << 0)) != 0;
boolean isAdmin = (attributes & (1 << 1)) != 0;
boolean hasPremiumAccess = (attributes & (1 << 2)) != 0;
boolean isVerified = (attributes & (1 << 3)) != 0;
In this example, we’ve used a single integer to store four boolean values, effectively reducing memory usage by a factor of 4 (assuming a 32-bit integer and boolean variables).
2. Improving Performance in Low-Level Operations
Bit manipulation can lead to significant performance improvements in low-level operations, particularly when dealing with hardware interfaces, embedded systems, or performance-critical code. Bitwise operations are typically faster than their arithmetic counterparts and can be executed in a single CPU cycle on many processors.
For instance, consider the task of determining if a number is even or odd. While you could use the modulo operator, a bitwise AND operation is more efficient:
// Using modulo operator
boolean isEven(int num) {
return num % 2 == 0;
}
// Using bit manipulation
boolean isEven(int num) {
return (num & 1) == 0;
}
The bitwise AND operation with 1 checks if the least significant bit is set, which determines whether the number is odd (1) or even (0). This approach is faster and more efficient, especially when performed repeatedly in a loop.
3. Implementing Efficient Data Structures
Bit manipulation techniques can be invaluable when implementing certain data structures, particularly those that benefit from compact representation or fast operations. Some examples include:
- Bitmap indexes
- Bloom filters
- Bitboards in chess engines
- Efficient hash table implementations
For instance, let’s look at a simple implementation of a bitmap to store the presence or absence of integers in a range:
public class Bitmap {
private int[] bits;
public Bitmap(int size) {
bits = new int[(size + 31) / 32];
}
public void set(int n) {
bits[n / 32] |= (1 << (n % 32));
}
public void clear(int n) {
bits[n / 32] &= ~(1 << (n % 32));
}
public boolean get(int n) {
return (bits[n / 32] & (1 << (n % 32))) != 0;
}
}
This bitmap implementation uses bit manipulation to efficiently store and retrieve information about the presence of integers, using significantly less memory than an array of booleans would require.
4. Optimizing Arithmetic Operations
Certain arithmetic operations can be optimized using bit manipulation techniques. While modern compilers often perform these optimizations automatically, understanding these techniques can be beneficial for writing efficient code and for scenarios where compiler optimizations might not be available or sufficient.
Some common arithmetic optimizations using bit manipulation include:
- Multiplying or dividing by powers of 2
- Finding the absolute value of an integer
- Determining the sign of a number
Here’s an example of using bit manipulation to efficiently multiply a number by 4:
// Standard multiplication
int result = num * 4;
// Using bit manipulation
int result = num << 2;
The left shift operation effectively multiplies the number by 2^n, where n is the number of positions shifted. This operation is typically faster than multiplication, especially on processors with limited multiplication capabilities.
5. Implementing Cryptographic Algorithms
Bit manipulation plays a crucial role in many cryptographic algorithms, where operations on individual bits are essential for encryption, decryption, and hashing processes. Understanding and implementing these bit-level operations is crucial for developing secure and efficient cryptographic systems.
For example, the XOR operation is widely used in cryptography due to its unique properties. Here’s a simple example of using XOR for basic encryption and decryption:
public class SimpleXORCipher {
public static String encrypt(String message, int key) {
StringBuilder result = new StringBuilder();
for (char c : message.toCharArray()) {
result.append((char) (c ^ key));
}
return result.toString();
}
public static String decrypt(String encrypted, int key) {
return encrypt(encrypted, key); // XOR is its own inverse
}
public static void main(String[] args) {
String message = "Hello, World!";
int key = 42;
String encrypted = encrypt(message, key);
System.out.println("Encrypted: " + encrypted);
String decrypted = decrypt(encrypted, key);
System.out.println("Decrypted: " + decrypted);
}
}
While this is a very basic example and not suitable for real-world encryption, it demonstrates the principle of using XOR operations in cryptographic processes.
6. Solving Algorithmic Problems
Many algorithmic problems, especially those encountered in competitive programming or technical interviews, can be efficiently solved using bit manipulation techniques. These problems often involve tasks such as:
- Finding the single non-repeating element in an array where every other element appears twice
- Generating all possible subsets of a set
- Implementing efficient power functions
- Solving puzzles involving binary representations
Let’s look at an example of finding a single non-repeating element in an array where every other element appears twice:
public class SingleNumber {
public static int findSingleNumber(int[] nums) {
int result = 0;
for (int num : nums) {
result ^= num;
}
return result;
}
public static void main(String[] args) {
int[] nums = {4, 1, 2, 1, 2};
System.out.println("The single number is: " + findSingleNumber(nums));
}
}
This solution uses the XOR operation’s property that a ^ a = 0 and a ^ 0 = a. By XORing all elements in the array, the duplicate elements cancel out, leaving only the single non-repeating element.
Best Practices and Considerations
While bit manipulation can offer significant benefits in certain scenarios, it’s important to use it judiciously and with careful consideration. Here are some best practices and considerations to keep in mind:
1. Readability vs. Efficiency
Bit manipulation code can often be less readable than more straightforward implementations. Always consider the trade-off between efficiency gains and code readability. If the performance improvement is minimal, it might be better to opt for more readable code.
2. Documentation
When using bit manipulation techniques, especially in complex scenarios, make sure to thoroughly document your code. Explain the purpose of each bitwise operation and how it contributes to the overall logic of your program.
3. Testing
Bit manipulation code can be prone to subtle errors. Implement comprehensive unit tests to ensure your bit-level operations are functioning as expected across various input scenarios.
4. Portability
Be aware of potential portability issues when using bit manipulation, especially when dealing with signed integers or when the size of integer types may vary across different systems.
5. Compiler Optimizations
Modern compilers are often capable of performing many bit-level optimizations automatically. In some cases, explicitly using bit manipulation might not provide significant benefits over simpler, more readable code that the compiler can optimize.
6. Maintainability
Consider the long-term maintainability of your code. While bit manipulation might offer performance benefits, it can make future modifications and debugging more challenging, especially for developers who are less familiar with these techniques.
Conclusion
Bit manipulation is a powerful technique that can significantly improve the efficiency and performance of your code in certain scenarios. It’s particularly useful for optimizing space usage, improving performance in low-level operations, implementing efficient data structures, optimizing arithmetic operations, developing cryptographic algorithms, and solving specific types of algorithmic problems.
However, it’s crucial to approach bit manipulation with careful consideration. Always weigh the benefits of improved efficiency against potential drawbacks in terms of code readability, maintainability, and portability. When used appropriately, bit manipulation can be a valuable tool in your programming toolkit, allowing you to write more efficient and optimized code.
As you continue to develop your programming skills, consider exploring bit manipulation techniques further and practice applying them in relevant scenarios. With time and experience, you’ll develop a keen sense of when and how to leverage these powerful low-level operations to enhance your code’s performance and efficiency.
Remember, the key to mastering bit manipulation, like any programming technique, lies in understanding its principles, practicing its application, and judiciously employing it in your projects. Happy coding!