When to Consider Using a State Machine in Software Development
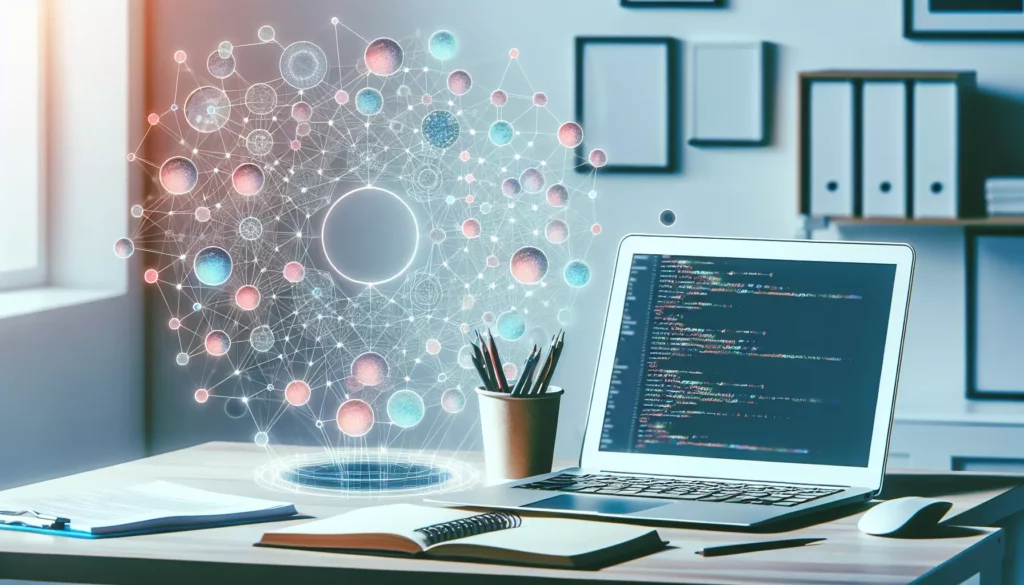
In the world of software development, managing complex systems and behaviors is a constant challenge. As applications grow in complexity, developers need robust tools and design patterns to maintain control and clarity in their code. One such powerful tool is the state machine. But when should you consider using a state machine in your projects? This comprehensive guide will explore the concept of state machines, their benefits, and the scenarios where they shine brightest.
What is a State Machine?
Before diving into when to use state machines, let’s establish a clear understanding of what they are. A state machine, also known as a finite state machine (FSM), is a computational model used to design systems that can be in one of a finite number of states at any given time. The machine can transition from one state to another based on specific inputs or conditions.
Key components of a state machine include:
- States: The possible conditions or modes the system can be in.
- Transitions: The rules for moving between states.
- Events: Triggers that cause state transitions.
- Actions: Behaviors or operations executed during transitions or while in a particular state.
State machines can be implemented in various programming languages and are often visualized using state diagrams, making them a powerful tool for both design and implementation.
Benefits of Using State Machines
State machines offer several advantages that make them attractive for certain types of software development:
- Clarity and Predictability: State machines provide a clear, visual representation of system behavior, making it easier to understand and predict how the system will react to different inputs.
- Reduced Complexity: By breaking down complex behaviors into discrete states and transitions, state machines can simplify the overall design of a system.
- Improved Maintainability: The structured nature of state machines makes it easier to modify and extend the system’s behavior over time.
- Better Error Handling: State machines can help identify and handle edge cases and unexpected inputs more effectively.
- Easier Testing: The well-defined states and transitions in a state machine facilitate more thorough and systematic testing.
When to Consider Using a State Machine
Now that we understand what state machines are and their benefits, let’s explore the scenarios where they are particularly useful:
1. Managing Complex Workflows
State machines excel at managing complex workflows or processes with multiple stages. For example, consider an e-commerce order fulfillment system:
States:
- New Order
- Payment Pending
- Payment Received
- Processing
- Shipped
- Delivered
- Cancelled
Transitions:
New Order -> Payment Pending
Payment Pending -> Payment Received
Payment Received -> Processing
Processing -> Shipped
Shipped -> Delivered
Any State -> Cancelled (except Delivered)
In this scenario, a state machine can clearly define the possible states of an order and the allowed transitions between them. This approach ensures that orders follow a logical progression and prevents invalid state changes (e.g., moving directly from “New Order” to “Shipped”).
2. User Interface Management
State machines are excellent for managing complex user interfaces, especially those with multiple modes or views. Consider a drawing application:
States:
- Idle
- Drawing
- Erasing
- Text Entry
- Shape Selection
Transitions:
Idle -> Drawing (when draw tool selected)
Idle -> Erasing (when eraser tool selected)
Idle -> Text Entry (when text tool selected)
Idle -> Shape Selection (when selection tool activated)
Any State -> Idle (when ESC key pressed)
Using a state machine in this context helps manage the application’s behavior based on the current tool selection and user actions, ensuring a consistent and intuitive user experience.
3. Game Development
State machines are widely used in game development to manage game states, character behaviors, and AI. For instance, consider a simple enemy AI in a game:
States:
- Patrolling
- Chasing
- Attacking
- Fleeing
Transitions:
Patrolling -> Chasing (when player detected)
Chasing -> Attacking (when player in range)
Attacking -> Fleeing (when health low)
Fleeing -> Patrolling (when health restored or player lost)
This state machine defines clear behaviors for the enemy AI and how it should react to different game conditions, making the AI more predictable and easier to implement and debug.
4. Protocol Implementation
State machines are invaluable when implementing network protocols or communication systems. For example, consider a simplified TCP connection:
States:
- CLOSED
- LISTEN
- SYN_SENT
- SYN_RECEIVED
- ESTABLISHED
- FIN_WAIT_1
- FIN_WAIT_2
- CLOSING
- TIME_WAIT
- CLOSE_WAIT
- LAST_ACK
Transitions:
CLOSED -> LISTEN (passive open)
CLOSED -> SYN_SENT (active open)
LISTEN -> SYN_RECEIVED (receive SYN)
SYN_SENT -> ESTABLISHED (receive SYN+ACK, send ACK)
SYN_RECEIVED -> ESTABLISHED (receive ACK)
ESTABLISHED -> FIN_WAIT_1 (close)
// ... (other transitions omitted for brevity)
Using a state machine ensures that the protocol implementation follows the correct sequence of steps and handles various edge cases appropriately.
5. Hardware Control Systems
State machines are commonly used in embedded systems and hardware control applications. For instance, consider a simple vending machine controller:
States:
- Idle
- Coin Inserted
- Product Selected
- Dispensing
- Change Return
Transitions:
Idle -> Coin Inserted (coin detected)
Coin Inserted -> Product Selected (product button pressed)
Product Selected -> Dispensing (if sufficient funds)
Dispensing -> Change Return (if change needed)
Change Return -> Idle
Product Selected -> Coin Inserted (if insufficient funds)
This state machine ensures that the vending machine follows a logical sequence of operations and handles various scenarios correctly.
6. Parser and Compiler Design
State machines are fundamental in designing parsers and compilers. They help manage the different stages of lexical analysis and syntax parsing. For example, a simple lexical analyzer for a programming language might use a state machine like this:
States:
- Start
- In Identifier
- In Number
- In String
- In Comment
Transitions:
Start -> In Identifier (letter encountered)
Start -> In Number (digit encountered)
Start -> In String (quote encountered)
Start -> In Comment (comment symbol encountered)
In Identifier -> Start (whitespace or operator encountered)
In Number -> Start (non-digit encountered)
In String -> Start (closing quote encountered)
In Comment -> Start (newline encountered)
This state machine helps the lexical analyzer correctly identify and tokenize different elements of the programming language.
7. Error Handling and Recovery
State machines can be particularly useful for managing error states and recovery processes in systems where reliability is crucial. Consider a fault-tolerant distributed system:
States:
- Normal Operation
- Degraded Performance
- Partial Outage
- Full Outage
- Recovery Mode
Transitions:
Normal Operation -> Degraded Performance (minor issues detected)
Degraded Performance -> Partial Outage (critical component failure)
Partial Outage -> Full Outage (multiple component failures)
Any State -> Recovery Mode (recovery process initiated)
Recovery Mode -> Normal Operation (system restored)
This state machine helps manage the system’s behavior under different fault conditions and ensures a structured approach to error handling and recovery.
Implementing State Machines in Code
While the concept of state machines is language-agnostic, the implementation can vary depending on the programming language and specific requirements. Here’s a simple example of how you might implement a basic state machine in Python:
class StateMachine:
def __init__(self):
self.state = 'idle'
def coin_inserted(self):
if self.state == 'idle':
self.state = 'coin_inserted'
print("Coin inserted. Please select a product.")
else:
print("Error: Can only insert coin when idle.")
def select_product(self):
if self.state == 'coin_inserted':
self.state = 'dispensing'
print("Product selected. Dispensing...")
else:
print("Error: Please insert a coin first.")
def dispense_complete(self):
if self.state == 'dispensing':
self.state = 'idle'
print("Product dispensed. Thank you!")
else:
print("Error: Nothing to dispense.")
# Usage
vending_machine = StateMachine()
vending_machine.coin_inserted()
vending_machine.select_product()
vending_machine.dispense_complete()
This simple implementation demonstrates how a state machine can manage the behavior of a vending machine, ensuring that actions occur in the correct sequence and preventing invalid operations.
Considerations and Potential Drawbacks
While state machines offer many benefits, it’s important to consider some potential drawbacks:
- Overhead for Simple Systems: For very simple systems or behaviors, implementing a full state machine might introduce unnecessary complexity.
- Scalability Challenges: As the number of states and transitions grows, state machines can become difficult to manage without proper tools or frameworks.
- Limited Flexibility: Strict state machines may not always be suitable for systems that require more dynamic or adaptive behavior.
- Learning Curve: Developers unfamiliar with state machine concepts may need time to adapt to this approach.
Conclusion
State machines are a powerful tool in a developer’s arsenal, offering a structured and clear approach to managing complex behaviors and systems. They excel in scenarios involving workflow management, user interfaces, game development, protocol implementation, hardware control, parser design, and error handling.
When considering whether to use a state machine in your project, ask yourself:
- Does the system have clearly defined states and transitions?
- Would a visual representation of the system’s behavior be beneficial?
- Is predictability and error handling crucial for the application?
- Could the system benefit from a more structured approach to managing complexity?
If you answer yes to these questions, a state machine might be the right choice for your project. Remember, like any design pattern or tool, state machines are not a one-size-fits-all solution. Always consider the specific needs and constraints of your project when deciding on the best approach.
By understanding when and how to use state machines effectively, you can create more robust, maintainable, and predictable software systems. Whether you’re building a complex workflow, designing a user interface, or implementing a communication protocol, state machines can provide the structure and clarity needed to tackle challenging development tasks with confidence.