When to Consider Using a Stack for Depth-First Search (DFS)
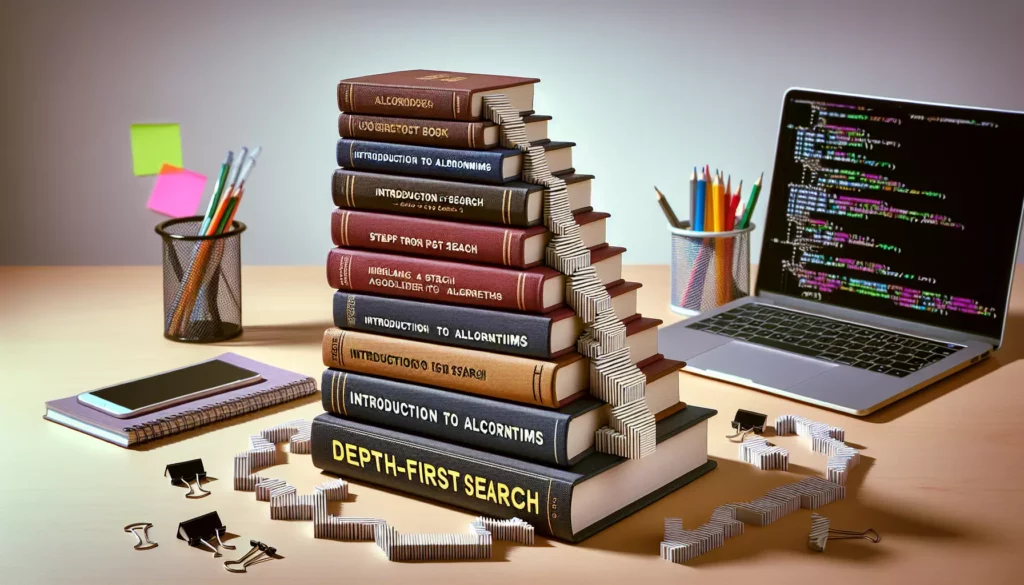
Depth-First Search (DFS) is a fundamental algorithm in computer science, widely used for traversing or searching tree or graph data structures. While DFS can be implemented using both recursive and iterative approaches, there are scenarios where using a stack-based iterative implementation is particularly advantageous. In this comprehensive guide, we’ll explore when and why you should consider using a stack for DFS, along with practical examples and implementation details.
Understanding Depth-First Search
Before diving into the specifics of stack-based DFS, let’s briefly review what Depth-First Search is and how it works:
- DFS is an algorithm for traversing or searching tree or graph data structures.
- It starts at a root node (in a tree) or an arbitrary node (in a graph) and explores as far as possible along each branch before backtracking.
- The algorithm goes deep into the data structure before going wide, hence the name “depth-first”.
DFS can be implemented in two main ways:
- Recursive DFS: Using function calls to implicitly manage the traversal stack.
- Iterative DFS: Using an explicit stack data structure to manage the traversal.
Advantages of Stack-Based DFS
While recursive DFS is often more intuitive and easier to implement, there are several scenarios where a stack-based approach is preferable:
1. Avoiding Stack Overflow
One of the primary reasons to use a stack-based DFS is to avoid stack overflow errors in deep recursive calls. When dealing with large or deep data structures, recursive DFS can lead to excessive function call stack growth, potentially causing a stack overflow. An iterative approach using an explicit stack allows for better control over memory usage.
2. Performance Optimization
In some programming languages and environments, managing your own stack can be more efficient than relying on the call stack. This is because you have more control over memory allocation and can optimize for your specific use case.
3. Easier State Management
When you need to maintain complex state information during the traversal, an iterative approach with an explicit stack can make it easier to manage and modify this state as you go.
4. Interruptible Traversal
If you need to pause and resume the traversal (e.g., in a game engine or a long-running process), an iterative approach allows you to more easily save and restore the state of the traversal.
5. Language Limitations
Some programming languages have limitations on recursion depth or do not optimize tail recursion. In these cases, an iterative approach is necessary for handling large datasets.
Implementing Stack-Based DFS
Let’s look at a basic implementation of stack-based DFS for a binary tree:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def iterative_dfs(root):
if not root:
return []
result = []
stack = [root]
while stack:
node = stack.pop()
result.append(node.val)
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
return result
In this implementation:
- We use a list (
stack
) to simulate a stack data structure. - We start by pushing the root node onto the stack.
- In each iteration, we pop a node from the stack, process it (in this case, add its value to the result), and then push its children onto the stack.
- By pushing the right child before the left child, we ensure that the left subtree is processed before the right subtree, maintaining the DFS order.
Scenarios Where Stack-Based DFS Shines
Now that we understand the basics of stack-based DFS, let’s explore specific scenarios where this approach is particularly useful:
1. Traversing Extremely Deep Trees or Graphs
When dealing with trees or graphs that have a very deep structure, recursive DFS can quickly lead to stack overflow errors. Consider a scenario where you’re traversing a file system with deeply nested directories:
import os
def iterative_file_system_dfs(root_path):
stack = [root_path]
while stack:
current_path = stack.pop()
print(current_path) # Process the current path
try:
# List contents of the directory
contents = os.listdir(current_path)
for item in contents:
item_path = os.path.join(current_path, item)
if os.path.isdir(item_path):
stack.append(item_path)
except PermissionError:
print(f"Permission denied: {current_path}")
except Exception as e:
print(f"Error processing {current_path}: {e}")
# Usage
iterative_file_system_dfs("/path/to/root/directory")
This implementation can handle extremely deep directory structures without risking stack overflow, and it allows for easy error handling and continuation of traversal even if some directories are inaccessible.
2. Graph Cycle Detection
When detecting cycles in a graph, a stack-based approach can be more intuitive and easier to implement than a recursive one. Here’s an example of cycle detection in a directed graph:
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def is_cyclic(self):
visited = set()
rec_stack = set()
for node in self.graph:
if self._is_cyclic_util(node, visited, rec_stack):
return True
return False
def _is_cyclic_util(self, v, visited, rec_stack):
stack = [(v, True)] # (node, is_new)
while stack:
node, is_new = stack.pop()
if is_new:
if node in visited:
if node in rec_stack:
return True
continue
visited.add(node)
rec_stack.add(node)
stack.append((node, False)) # Mark for removal from rec_stack
for neighbor in self.graph[node]:
stack.append((neighbor, True))
else:
rec_stack.remove(node)
return False
# Usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(1, 2)
g.add_edge(2, 3)
g.add_edge(3, 1) # Creates a cycle
print("Graph contains cycle:", g.is_cyclic())
This implementation uses a stack to simulate the recursion stack, allowing for better control over the traversal process and easier cycle detection.
3. Iterative Deepening Depth-First Search (IDDFS)
IDDFS is a state space/graph search strategy that combines depth-first search’s space-efficiency and breadth-first search’s completeness. It’s particularly useful when searching very large (or even infinite) state spaces. Here’s an implementation using a stack-based approach:
def iddfs(root, goal, max_depth):
for depth in range(max_depth + 1):
if dls(root, goal, depth):
return True
return False
def dls(node, goal, depth):
stack = [(node, 0)]
while stack:
current, current_depth = stack.pop()
if current == goal:
return True
if current_depth < depth:
for child in get_children(current):
stack.append((child, current_depth + 1))
return False
def get_children(node):
# This function should return the children of the given node
# Implementation depends on your specific problem
pass
# Usage
root_node = ... # Define your root node
goal_node = ... # Define your goal node
max_search_depth = 100
if iddfs(root_node, goal_node, max_search_depth):
print("Goal found!")
else:
print("Goal not found within the maximum depth.")
This implementation allows for efficient searching of large state spaces without the risk of getting stuck in an infinite path, as might happen with standard DFS.
4. Web Crawling
Web crawling is another area where stack-based DFS can be very useful. It allows for better control over the crawling process and easier implementation of features like depth limiting. Here’s a simple example:
import requests
from bs4 import BeautifulSoup
from urllib.parse import urljoin, urlparse
def web_crawler(start_url, max_depth=3):
stack = [(start_url, 0)]
visited = set()
while stack:
url, depth = stack.pop()
if depth > max_depth:
continue
if url in visited:
continue
visited.add(url)
print(f"Crawling: {url} (Depth: {depth})")
try:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
href = link.get('href')
if href:
full_url = urljoin(url, href)
if urlparse(full_url).netloc == urlparse(start_url).netloc:
stack.append((full_url, depth + 1))
except Exception as e:
print(f"Error crawling {url}: {e}")
# Usage
web_crawler("https://example.com")
This crawler uses a stack to manage the URLs to be visited, allowing for easy depth control and the ability to interrupt and resume the crawling process if needed.
Best Practices for Stack-Based DFS
When implementing stack-based DFS, keep these best practices in mind:
- Use an appropriate stack data structure: While a list can work as a stack in Python, consider using
collections.deque
for more efficient pop and append operations in larger datasets. - Handle cycles: In graph traversals, always keep track of visited nodes to avoid infinite loops.
- Consider space complexity: While stack-based DFS can help avoid call stack overflow, be mindful of the space used by your explicit stack, especially for very large datasets.
- Optimize for your specific use case: Tailor your implementation to the specific requirements of your problem, such as early termination conditions or custom node processing logic.
- Use iterators for memory efficiency: When dealing with large datasets, consider using iterators to generate children nodes on-the-fly instead of storing all of them in memory.
Conclusion
Stack-based Depth-First Search is a powerful tool in a programmer’s arsenal, offering advantages in terms of memory management, performance, and flexibility. While recursive DFS remains a valid and often more intuitive approach for many scenarios, understanding when and how to use stack-based DFS can significantly enhance your ability to tackle complex traversal and search problems efficiently.
By mastering this technique, you’ll be better equipped to handle deep data structures, implement interruptible traversals, optimize performance in certain languages, and manage complex state during traversals. As with any programming technique, the key is to understand the trade-offs and choose the right tool for the specific problem at hand.
Remember, the examples provided here are starting points. As you encounter more complex scenarios in your coding journey, you’ll find opportunities to adapt and expand upon these concepts to create even more sophisticated and efficient solutions.