When to Consider Using a Sentinel Node in Data Structures
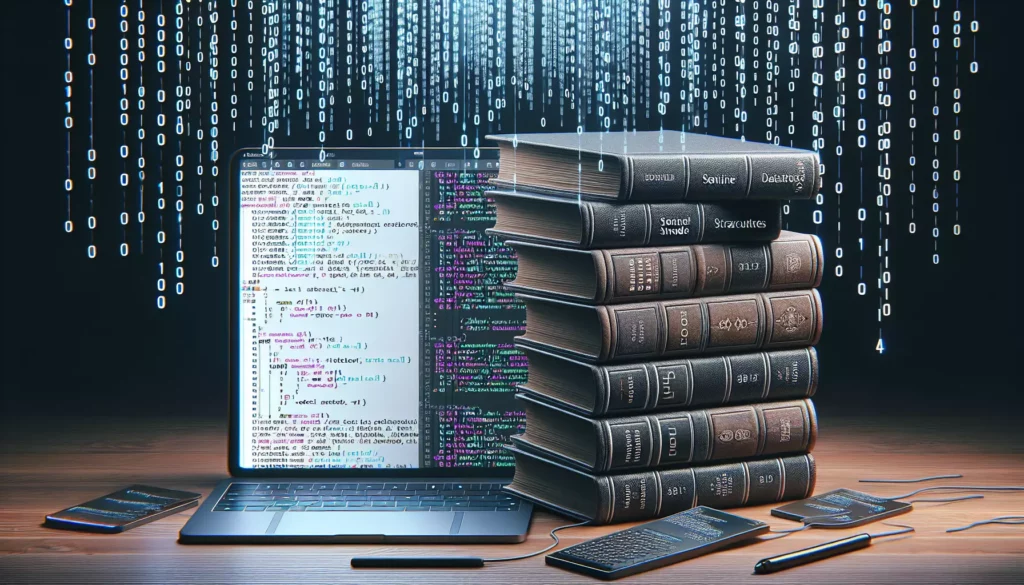
In the world of data structures and algorithms, efficiency and simplicity are key. As programmers, we’re constantly seeking ways to optimize our code and make our data structures more robust. One technique that often flies under the radar but can be incredibly useful in certain scenarios is the use of sentinel nodes. In this comprehensive guide, we’ll explore what sentinel nodes are, when to use them, and how they can improve your data structure implementations.
What is a Sentinel Node?
Before we dive into the specifics of when to use sentinel nodes, let’s first establish what they are. A sentinel node, also known as a dummy node, is a special node in a data structure that doesn’t hold any actual data but serves as a marker. It’s typically used at the beginning or end of a linked data structure, such as a linked list or a tree.
The primary purpose of a sentinel node is to simplify boundary conditions and edge cases in algorithms that operate on the data structure. By having a sentinel node, you can avoid null checks and special cases for the first or last elements, making your code cleaner and potentially more efficient.
When Should You Consider Using a Sentinel Node?
Now that we understand what sentinel nodes are, let’s explore the situations where using them can be beneficial:
1. Simplifying Linked List Operations
One of the most common applications of sentinel nodes is in linked lists. Here are some scenarios where they prove particularly useful:
Insertion at the Beginning
Without a sentinel node, inserting an element at the beginning of a linked list requires a special case:
if (head == null) {
head = newNode;
} else {
newNode.next = head;
head = newNode;
}
With a sentinel node, the insertion becomes uniform regardless of whether the list is empty:
newNode.next = sentinel.next;
sentinel.next = newNode;
Deletion from an Empty List
Deleting from an empty list without a sentinel node requires an additional check:
if (head == null) {
// List is already empty
return;
}
With a sentinel node, you don’t need this check because the sentinel node is always present.
Simplifying Algorithms
Many linked list algorithms become simpler with a sentinel node. For example, reversing a linked list or finding the middle element can be implemented more cleanly.
2. Improving Tree Traversals
In tree data structures, sentinel nodes can be used to simplify certain operations:
Boundary Conditions in Binary Search Trees
When implementing a binary search tree, using sentinel nodes for leaf nodes can simplify insertion and deletion operations by eliminating the need to handle null pointers.
Simplifying Red-Black Tree Operations
In self-balancing trees like Red-Black trees, sentinel nodes can greatly simplify the implementation of rotation and recoloring operations during insertion and deletion.
3. Enhancing Graph Algorithms
Sentinel nodes can also be useful in graph algorithms:
Simplifying Adjacency List Representation
In an adjacency list representation of a graph, using a sentinel node at the beginning of each vertex’s list can simplify insertion and traversal operations.
Marking Special Vertices
In some graph algorithms, sentinel nodes can be used to mark special vertices, such as the source or sink in a flow network.
4. Optimizing Cache Performance
In some cases, using sentinel nodes can lead to better cache performance:
Avoiding Null Checks
By eliminating the need for null checks, sentinel nodes can reduce branch mispredictions, potentially leading to better performance on modern processors.
Improving Memory Layout
In certain data structures, using sentinel nodes can lead to a more cache-friendly memory layout, improving overall performance.
Implementing Sentinel Nodes: Best Practices
When you decide to use sentinel nodes in your data structures, keep these best practices in mind:
1. Clear Distinction
Make sure your sentinel nodes are clearly distinguishable from regular nodes. This could be through a special flag or by using a dedicated sentinel class.
2. Consistency
Use sentinel nodes consistently throughout your data structure. If you’re using them for the head of a linked list, consider using them for the tail as well.
3. Documentation
Clearly document the use of sentinel nodes in your code. This is especially important if you’re working on a team or if your code might be maintained by others in the future.
4. Performance Considerations
While sentinel nodes can simplify code, they do introduce some overhead in terms of memory usage. Consider the trade-offs in your specific use case.
Example: Implementing a Circular Linked List with a Sentinel Node
Let’s look at a practical example of how to implement a circular linked list using a sentinel node in Java:
public class CircularLinkedList<T> {
private static class Node<T> {
T data;
Node<T> next;
Node(T data) {
this.data = data;
this.next = null;
}
}
private Node<T> sentinel;
public CircularLinkedList() {
sentinel = new Node<>(null);
sentinel.next = sentinel; // Points to itself when empty
}
public void insert(T data) {
Node<T> newNode = new Node<>(data);
newNode.next = sentinel.next;
sentinel.next = newNode;
}
public void delete(T data) {
Node<T> current = sentinel;
while (current.next != sentinel) {
if (current.next.data.equals(data)) {
current.next = current.next.next;
return;
}
current = current.next;
}
}
public void display() {
Node<T> current = sentinel.next;
while (current != sentinel) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("sentinel");
}
}
In this implementation, the sentinel node simplifies the insertion and deletion operations. There’s no need to check for an empty list or handle the case of deleting the last element separately.
Potential Drawbacks of Using Sentinel Nodes
While sentinel nodes can be very useful, they’re not without their drawbacks. Here are some potential issues to consider:
1. Increased Memory Usage
Each sentinel node takes up additional memory. In data structures with many instances (e.g., in a graph with many vertices), this can add up.
2. Complexity for Newcomers
For developers unfamiliar with the concept, sentinel nodes might initially seem confusing or unnecessary, potentially making the code harder to understand at first glance.
3. Potential for Misuse
If not implemented correctly, sentinel nodes can lead to bugs. For example, if a sentinel node is accidentally treated as a regular node, it could corrupt the data structure.
4. Overhead in Simple Cases
For very simple data structures or in cases where the extra complexity isn’t needed, using sentinel nodes might be overkill and could actually make the code more complex than necessary.
Alternatives to Sentinel Nodes
In some cases, there might be alternatives to using sentinel nodes that could be more appropriate:
1. Null Object Pattern
Instead of using a sentinel node, you could use the Null Object pattern to represent the absence of a node. This can achieve similar simplification in some cases without the need for an extra node.
2. Special Case Pattern
In some scenarios, using the Special Case pattern (as described by Martin Fowler) could be a good alternative to sentinel nodes.
3. Careful Null Handling
In languages with good null-handling features (like Kotlin’s null safety or Rust’s Option type), carefully handling null cases might be preferable to using sentinel nodes.
When Not to Use Sentinel Nodes
While sentinel nodes can be very useful, they’re not always the best choice. Here are some situations where you might want to avoid using them:
1. Simple Data Structures
For very simple data structures where the additional complexity isn’t warranted, it’s often better to stick with a straightforward implementation without sentinel nodes.
2. Memory-Constrained Environments
In environments where memory is at a premium, the extra memory used by sentinel nodes might not be justifiable.
3. When Clarity is Paramount
In educational settings or when writing code that needs to be as clear and straightforward as possible, avoiding sentinel nodes might make the code easier to understand for beginners.
4. When Performance is Critical
In some high-performance scenarios, the extra memory access required by sentinel nodes might introduce unacceptable overhead.
Conclusion
Sentinel nodes are a powerful tool in the data structure implementer’s toolkit. They can significantly simplify code, eliminate edge cases, and in some scenarios, even improve performance. However, like any tool, they should be used judiciously.
When deciding whether to use sentinel nodes, consider the following:
- The complexity of your data structure and its operations
- The frequency of edge cases in your algorithms
- The memory constraints of your environment
- The familiarity of your team with the concept
- The potential for performance improvements
By carefully weighing these factors, you can make an informed decision about whether sentinel nodes are the right choice for your specific use case.
Remember, the goal is always to write code that is efficient, maintainable, and easy to understand. Sometimes sentinel nodes will help you achieve that goal, and sometimes they won’t. The key is to understand the trade-offs and make the best decision for your particular situation.
As you continue to develop your skills in data structures and algorithms, keep sentinel nodes in mind as a potential tool to simplify your implementations and make your code more robust. With practice and experience, you’ll develop an intuition for when they’re the right choice and when other approaches might be more appropriate.