When to Consider Using a Running Product in Your Coding Journey
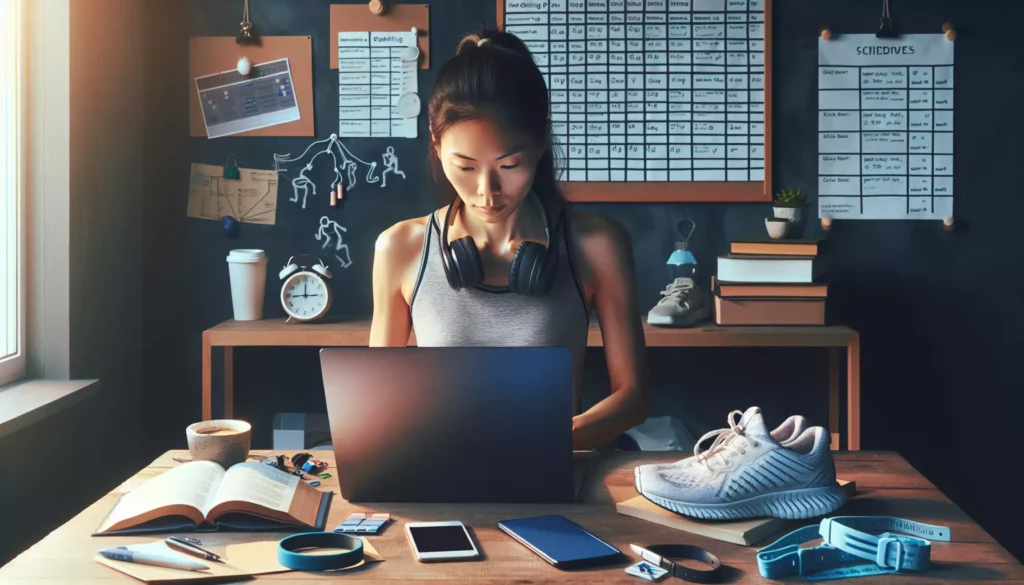
In the fast-paced world of software development and coding education, staying ahead of the curve is crucial. As you progress on your journey from a beginner coder to a proficient programmer ready to tackle technical interviews at major tech companies, you might come across the concept of a “running product.” But what exactly is a running product, and when should you consider using one in your coding education? Let’s dive deep into this topic and explore how it fits into the broader landscape of coding skills development, particularly in the context of platforms like AlgoCademy.
Understanding the Concept of a Running Product
Before we delve into when to use a running product, it’s essential to understand what it is. In the context of coding and software development, a running product refers to a live, functioning application or system that is actively being used and continuously improved. This is in contrast to static projects or theoretical exercises that may not reflect real-world scenarios.
A running product can be:
- A web application serving actual users
- A mobile app available on app stores
- A backend service processing real data
- An open-source project with active contributors
The key characteristic of a running product is that it’s “alive” – it’s not just code sitting in a repository, but software that’s out in the wild, facing real users, real data, and real challenges.
The Benefits of Working with a Running Product
Working with a running product can offer numerous advantages for coders at various stages of their learning journey:
- Real-world Experience: Engaging with a running product exposes you to genuine scenarios that you might encounter in a professional setting.
- Practical Problem-Solving: You’ll face actual issues that require immediate attention and creative solutions.
- Understanding Scale: Running products often deal with scalability concerns, giving you insights into handling larger user bases and data volumes.
- Collaboration Skills: Many running products involve team efforts, helping you develop crucial collaboration and communication skills.
- Continuous Learning: As the product evolves, you’ll need to adapt and learn new technologies and methodologies.
When to Consider Using a Running Product
Now that we understand what a running product is and its benefits, let’s explore the scenarios where incorporating one into your coding education can be particularly beneficial:
1. When You’re Ready to Move Beyond Tutorials
If you’ve been following coding tutorials and completing small projects but feel ready for a more substantial challenge, it might be time to consider working with a running product. This transition can help you apply your knowledge in a more complex and realistic environment.
For example, instead of just creating a simple to-do list app as a learning exercise, you could contribute to an open-source project management tool that’s actually being used by real teams. This would expose you to more intricate codebases and real user feedback.
2. When Preparing for Technical Interviews
Many technical interviews, especially for positions at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), involve discussing large-scale systems and complex scenarios. Having experience with a running product can give you valuable insights and talking points for these interviews.
For instance, if you’ve worked on a running product that handles user authentication, you’ll be better equipped to discuss security considerations, scalability issues, and best practices during an interview.
3. When You Want to Improve Your Debugging Skills
Running products often encounter unexpected issues and bugs that need to be resolved quickly. This presents an excellent opportunity to hone your debugging skills in a high-stakes environment.
Consider this scenario: You’re working on a running e-commerce platform, and suddenly there’s a spike in cart abandonment rates. Debugging this issue would require you to analyze logs, reproduce the problem, and implement a fix – all valuable skills that are best learned in a real-world context.
4. When You’re Looking to Specialize in a Particular Area
If you’re interested in specializing in a specific area of software development, such as backend systems, front-end frameworks, or mobile development, working with a running product in that domain can provide focused, in-depth experience.
For example, if you’re aiming to become a mobile app developer, contributing to a running mobile application can give you hands-on experience with app store deployments, user analytics, and mobile-specific optimizations.
5. When You Want to Understand the Full Software Development Lifecycle
Running products go through various stages of development, deployment, maintenance, and iteration. By engaging with one, you can gain a holistic understanding of the entire software development lifecycle.
This experience can be invaluable when you’re trying to grasp concepts like Continuous Integration/Continuous Deployment (CI/CD), version control best practices, and Agile methodologies in a practical context.
6. When You’re Building Your Portfolio
For many aspiring developers, building a strong portfolio is crucial for landing job opportunities. Contributing to a running product, especially an open-source one, can significantly enhance your portfolio.
Imagine being able to point to specific features or improvements you’ve made to a widely-used application. This concrete evidence of your skills can be far more impressive to potential employers than academic projects alone.
7. When You Want to Improve Your Code Quality and Best Practices
Running products often have established coding standards, review processes, and quality assurance measures. Engaging with such a product can help you internalize best practices and improve the overall quality of your code.
For instance, you might learn about writing effective unit tests, adhering to specific code style guidelines, or implementing design patterns in a real-world context.
How to Find and Engage with Running Products
Now that we’ve established when to consider using a running product, you might be wondering how to find and engage with one. Here are some strategies:
1. Open Source Contributions
Many open-source projects are running products used by thousands or even millions of users. Platforms like GitHub and GitLab host numerous open-source projects that welcome contributions from developers at various skill levels.
To get started:
- Search for projects in your area of interest
- Look for issues labeled “good first issue” or “beginner-friendly”
- Read the project’s contribution guidelines
- Start with small, manageable contributions and gradually take on more complex tasks
2. Internships and Co-op Programs
Many companies offer internships or co-op programs where you can work on their running products. These opportunities often provide structured mentorship and a chance to contribute to large-scale, production-level systems.
3. Side Projects with Real Users
Consider turning one of your personal projects into a running product by releasing it to real users. This could be as simple as publishing a mobile app on an app store or deploying a web application and promoting it to potential users.
4. Contribute to Academic or Non-Profit Projects
Universities and non-profit organizations often have ongoing software projects that serve real users. These can be excellent opportunities to work on running products while also contributing to meaningful causes.
5. Participate in Hackathons
Some hackathons focus on improving or building upon existing running products. These events can provide a concentrated, immersive experience in working with live systems.
Integrating Running Product Experience with AlgoCademy
While platforms like AlgoCademy provide excellent resources for learning coding concepts, algorithmic thinking, and preparing for technical interviews, integrating this knowledge with experience from running products can create a powerful combination.
Here’s how you can effectively combine your AlgoCademy learning with running product experience:
1. Apply AlgoCademy Concepts to Real Problems
As you learn about data structures, algorithms, and problem-solving techniques on AlgoCademy, look for opportunities to apply these concepts in the running product you’re working on. This could involve optimizing a slow database query, improving the efficiency of a sorting algorithm, or implementing a more effective caching strategy.
2. Use Running Product Scenarios in Interview Prep
When practicing for technical interviews on AlgoCademy, use real scenarios from your running product experience as context for problem-solving. This can help you provide more detailed and authentic responses during actual interviews.
3. Leverage AI-Powered Assistance for Running Product Challenges
If you encounter a particularly challenging problem in your running product work, use AlgoCademy’s AI-powered assistance to break down the problem and explore potential solutions. This can help you approach complex real-world issues with a structured, algorithmic mindset.
4. Contribute Running Product Insights to the Community
Share your experiences and insights from working on running products with the AlgoCademy community. This could be through forum discussions, blog posts, or even suggesting new problem scenarios based on real-world challenges you’ve faced.
Code Example: Implementing a Feature in a Running Product
To illustrate how working on a running product might look in practice, let’s consider a scenario where you’re adding a new feature to a running e-commerce platform. The task is to implement a product recommendation system based on user browsing history.
Here’s a simplified example of how you might approach this:
// Assuming we have a User class and a Product class
class RecommendationEngine {
private Map<User, List<Product>> userBrowsingHistory;
private Map<Product, List<Product>> productSimilarities;
public RecommendationEngine() {
this.userBrowsingHistory = new HashMap<>();
this.productSimilarities = new HashMap<>();
}
public void recordUserBrowsing(User user, Product product) {
userBrowsingHistory.computeIfAbsent(user, k -> new ArrayList<>()).add(product);
}
public void updateProductSimilarities() {
// Implementation to update product similarities based on co-browsing patterns
// This would typically involve some form of collaborative filtering algorithm
}
public List<Product> getRecommendations(User user, int numRecommendations) {
List<Product> browsedProducts = userBrowsingHistory.getOrDefault(user, new ArrayList<>());
Set<Product> recommendations = new HashSet<>();
for (Product browsedProduct : browsedProducts) {
List<Product> similarProducts = productSimilarities.getOrDefault(browsedProduct, new ArrayList<>());
recommendations.addAll(similarProducts);
if (recommendations.size() >= numRecommendations) {
break;
}
}
return new ArrayList<>(recommendations).subList(0, Math.min(recommendations.size(), numRecommendations));
}
}
// Usage in the main application
public class EcommerceApp {
private RecommendationEngine recommendationEngine;
public EcommerceApp() {
this.recommendationEngine = new RecommendationEngine();
}
public void userViewedProduct(User user, Product product) {
// Other logic for recording the view
recommendationEngine.recordUserBrowsing(user, product);
}
public List<Product> getPersonalizedRecommendations(User user) {
return recommendationEngine.getRecommendations(user, 5);
}
// Other methods...
}
This example demonstrates several key aspects of working with a running product:
- Real-time Data Handling: The
recordUserBrowsing
method shows how you might handle real-time user interactions. - Scalability Considerations: The use of
Map
data structures suggests considerations for efficient data retrieval as the user base grows. - Algorithm Implementation: The
getRecommendations
method implements a basic recommendation algorithm, which you’d likely refine and optimize over time based on real user data and performance metrics. - Integration with Existing Systems: The
EcommerceApp
class shows how this new feature would be integrated into the broader application.
In a real running product, you’d also need to consider aspects like:
- Database interactions for persisting and retrieving data
- Concurrency handling for multi-user scenarios
- Performance optimizations for large datasets
- Error handling and logging for production environments
- Testing strategies, including unit tests and integration tests
Conclusion: Balancing Learning and Real-World Application
As you progress in your coding journey, the decision to engage with a running product should be based on your current skill level, learning goals, and career aspirations. While platforms like AlgoCademy provide invaluable resources for building a strong foundation in coding and algorithmic thinking, incorporating experience with running products can offer a complementary, practical dimension to your learning.
Remember, the timing doesn’t have to be perfect. You can start small, perhaps by contributing to an open-source project or turning a personal project into a running product. As you gain confidence and skills, you can gradually take on more complex challenges in larger-scale running products.
The key is to maintain a balance between structured learning (like what AlgoCademy offers) and practical application. This combination will not only make you a more well-rounded developer but also prepare you for the realities of professional software development, including the challenges you might face in technical interviews at major tech companies.
By thoughtfully incorporating running product experience into your coding education, you’ll be better equipped to bridge the gap between theoretical knowledge and practical application, setting yourself up for success in your programming career.