When to Consider Using a Running Maximum in Programming
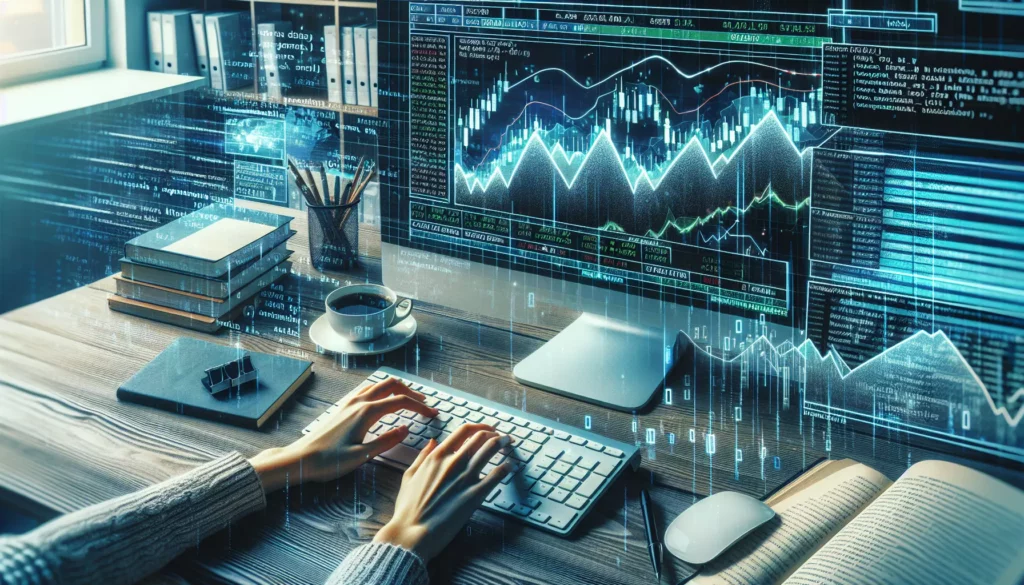
In the world of programming and algorithmic problem-solving, efficiency is key. One technique that often proves invaluable in certain scenarios is the concept of a “running maximum.” This approach can significantly optimize algorithms dealing with sequences or streams of data. In this comprehensive guide, we’ll explore when and how to use a running maximum, its benefits, and real-world applications.
What is a Running Maximum?
Before diving into the specifics, let’s define what we mean by a “running maximum”:
A running maximum (also known as a “sliding maximum” or “moving maximum”) is a technique used to keep track of the maximum value in a sequence or stream of data as you process it, without the need to store or re-examine all previous elements.
This technique is particularly useful when you need to find the maximum value within a specific window or range of elements, especially when dealing with large datasets or real-time data streams.
When Should You Consider Using a Running Maximum?
There are several scenarios where implementing a running maximum can be beneficial:
1. Processing Large Datasets
When working with extensive datasets that don’t fit entirely in memory, a running maximum allows you to find the maximum value without loading all the data at once. This is particularly useful in big data applications or when processing files that exceed available RAM.
2. Real-time Data Streams
In scenarios where you’re dealing with continuous data streams (e.g., sensor readings, stock prices, network traffic), a running maximum helps you maintain the current maximum value without storing the entire history of data.
3. Sliding Window Problems
Many algorithmic problems involve finding the maximum (or minimum) value within a sliding window of fixed size as it moves through an array or list. A running maximum is perfect for these types of problems.
4. Time Series Analysis
In time series data analysis, you might need to find the peak value over a certain time period. A running maximum can efficiently track this as you process the time series data.
5. Optimization Problems
Some optimization algorithms require keeping track of the best solution found so far. A running maximum (or minimum, depending on the problem) can serve this purpose effectively.
Implementing a Running Maximum
Let’s look at a basic implementation of a running maximum in Python:
def running_maximum(data):
if not data:
return None
max_so_far = float('-inf')
for item in data:
if item > max_so_far:
max_so_far = item
yield max_so_far
# Example usage
data = [1, 3, 2, 5, 4, 7, 6]
for max_value in running_maximum(data):
print(max_value)
This implementation uses a generator function to yield the maximum value seen so far as it iterates through the data. The output would be:
1
3
3
5
5
7
7
This approach is memory-efficient as it doesn’t need to store all the data, and it processes each element only once, resulting in O(n) time complexity.
Advanced Applications of Running Maximum
While the basic concept is straightforward, running maximums can be applied in more complex scenarios. Let’s explore some advanced applications:
1. Sliding Window Maximum
One classic problem that leverages the running maximum concept is finding the maximum in all subarrays of size k in an array. This problem is often referred to as the “Sliding Window Maximum” problem.
Here’s an efficient solution using a deque (double-ended queue) in Python:
from collections import deque
def sliding_window_maximum(nums, k):
result = []
window = deque()
for i, num in enumerate(nums):
# Remove indices that are out of the current window
if window and window[0] == i - k:
window.popleft()
# Remove all smaller elements from the back
while window and nums[window[-1]] < num:
window.pop()
window.append(i)
# Start appending to result once we have processed k elements
if i >= k - 1:
result.append(nums[window[0]])
return result
# Example usage
nums = [1, 3, -1, -3, 5, 3, 6, 7]
k = 3
print(sliding_window_maximum(nums, k))
This algorithm maintains a deque of indices where the corresponding values are in decreasing order. The front of the deque always contains the index of the maximum value in the current window.
2. Stock Price Problems
Running maximums (and minimums) are often used in stock price problems. For example, to find the maximum profit from buying and selling stocks, you can use a running minimum to keep track of the lowest price seen so far:
def max_profit(prices):
if not prices:
return 0
min_price = float('inf')
max_profit = 0
for price in prices:
if price < min_price:
min_price = price
else:
max_profit = max(max_profit, price - min_price)
return max_profit
# Example usage
prices = [7, 1, 5, 3, 6, 4]
print(max_profit(prices)) # Output: 5
This algorithm uses a running minimum (min_price) to keep track of the lowest price seen so far, allowing it to calculate the maximum profit in a single pass through the prices.
3. Longest Increasing Subsequence
While not a direct application of running maximum, the problem of finding the Longest Increasing Subsequence (LIS) uses a similar concept. Here’s an O(n log n) solution that uses binary search:
import bisect
def longest_increasing_subsequence(nums):
if not nums:
return 0
tails = [0] * len(nums)
size = 0
for num in nums:
i = bisect.bisect_left(tails, num, 0, size)
tails[i] = num
if i == size:
size += 1
return size
# Example usage
nums = [10, 9, 2, 5, 3, 7, 101, 18]
print(longest_increasing_subsequence(nums)) # Output: 4
This algorithm maintains an array tails where tails[i] is the smallest tail of all increasing subsequences of length i+1. It uses binary search to efficiently update this array as it processes each number.
Performance Considerations
When implementing a running maximum, it’s crucial to consider the performance implications:
Time Complexity
In most cases, a running maximum algorithm will have a time complexity of O(n), where n is the number of elements in the dataset. This is because each element is typically processed once.
However, in more complex scenarios like the Sliding Window Maximum problem, additional data structures (like a deque) may be used to achieve O(n) time complexity, even though each element might be processed multiple times.
Space Complexity
One of the main advantages of using a running maximum is its space efficiency. In its simplest form, it requires only O(1) extra space to keep track of the maximum value.
More complex applications might require additional space. For example, the Sliding Window Maximum algorithm uses a deque that could potentially store up to k elements, resulting in O(k) space complexity.
Comparison with Other Methods
Let’s compare the running maximum approach with other methods for finding the maximum value in different scenarios:
Scenario | Running Maximum | Naive Approach |
---|---|---|
Finding max in entire dataset | O(n) time, O(1) space | O(n) time, O(n) space (if storing all data) |
Sliding Window Maximum | O(n) time, O(k) space | O(nk) time, O(1) space (brute force) |
Real-time stream processing | O(1) time per element, O(1) space | O(n) time, O(n) space (storing all data) |
As we can see, the running maximum approach often provides a good balance between time and space efficiency, especially in scenarios involving large datasets or real-time processing.
Real-World Applications
The concept of a running maximum finds applications in various real-world scenarios:
1. Financial Analysis
In financial markets, tracking the highest stock price over a given period or finding the maximum drawdown (the largest peak-to-trough decline) are common applications of running maximum/minimum algorithms.
2. Environmental Monitoring
Weather stations and environmental monitoring systems often need to track maximum temperatures, rainfall, or pollution levels over various time periods.
3. Network Traffic Analysis
Network administrators might use running maximum algorithms to monitor peak traffic levels or to detect unusual spikes in network activity.
4. Manufacturing and Quality Control
In manufacturing processes, running maximums can be used to track peak pressures, temperatures, or other critical parameters to ensure they stay within acceptable limits.
5. Sports Analytics
Sports statisticians might use running maximums to track records or peak performances over time.
Common Pitfalls and How to Avoid Them
While implementing a running maximum can be straightforward, there are some common pitfalls to be aware of:
1. Initialization
Ensure that you initialize your running maximum correctly. For finding a maximum, initialize with negative infinity (or the smallest possible value in your data type). For a minimum, use positive infinity.
max_so_far = float('-inf') # For maximum
min_so_far = float('inf') # For minimum
2. Handling Empty Datasets
Always consider what should happen if the input dataset is empty. Should you return None, raise an exception, or handle it in some other way?
def running_maximum(data):
if not data:
return None # or raise an exception
# rest of the implementation
3. Floating-Point Precision
When working with floating-point numbers, be aware of precision issues. In some cases, you might need to use a tolerance value when comparing numbers.
import math
def is_greater(a, b, tolerance=1e-9):
return (a - b) > tolerance
# Use in your running maximum function
if is_greater(item, max_so_far):
max_so_far = item
4. Overflow and Underflow
In languages with fixed-size integer types, be cautious of overflow when dealing with very large numbers or underflow with very small numbers.
5. Modifying the Input
Be careful not to modify the input data unless that’s explicitly part of your algorithm. It’s often better to work with a copy or use read-only access to the original data.
Conclusion
The running maximum is a powerful technique that can significantly optimize algorithms dealing with sequences or streams of data. Its ability to efficiently track the maximum value without storing all previous elements makes it invaluable in scenarios involving large datasets, real-time data streams, and sliding window problems.
By understanding when and how to apply a running maximum, you can write more efficient and scalable code. Whether you’re working on financial analysis, environmental monitoring, or solving complex algorithmic problems, the running maximum technique can be a valuable tool in your programming arsenal.
Remember to consider the specific requirements of your problem, the nature of your data, and potential edge cases when implementing a running maximum. With careful implementation, this technique can lead to elegant and efficient solutions to a wide range of programming challenges.
As you continue to develop your programming skills, keep the running maximum in mind as a go-to technique for problems involving sequential data processing and optimization. It’s a testament to how seemingly simple concepts can have profound impacts on algorithm efficiency and problem-solving in computer science.