When to Consider Using a Count Array: A Comprehensive Guide
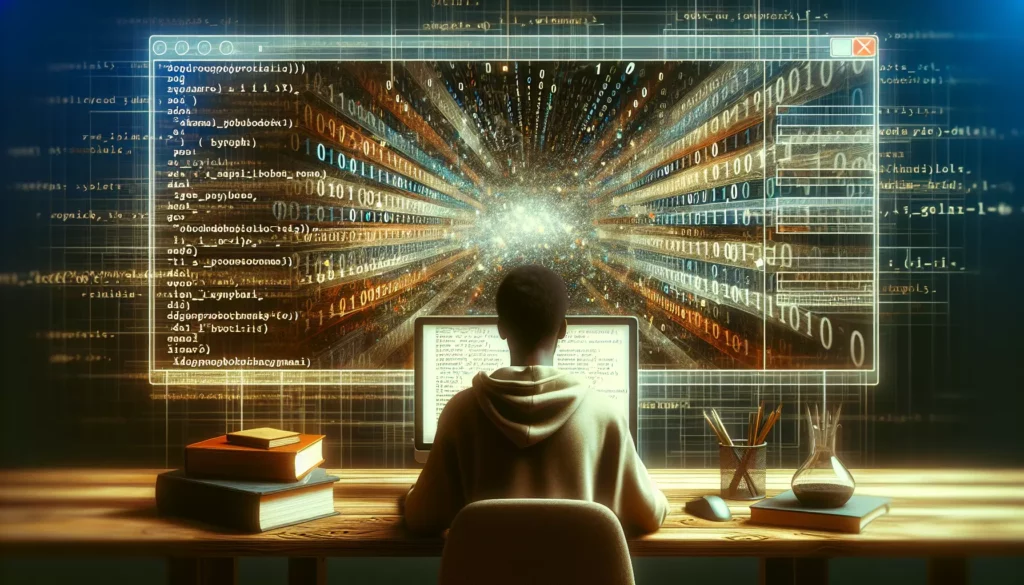
In the world of programming and algorithm design, efficiency is key. As developers, we’re constantly searching for ways to optimize our code and solve problems more effectively. One powerful technique that often flies under the radar is the use of count arrays. In this comprehensive guide, we’ll explore when and why you should consider using count arrays, along with practical examples and use cases.
What is a Count Array?
Before diving into the specifics, let’s start with a basic definition. A count array, also known as a frequency array, is a data structure used to keep track of the occurrences of elements in a collection. It’s typically implemented as an integer array where each index represents a unique element, and the value at that index represents the count or frequency of that element.
When Should You Use a Count Array?
Count arrays are particularly useful in scenarios where you need to:
- Count the frequency of elements in a collection
- Efficiently look up the occurrence count of elements
- Sort elements based on their frequency
- Solve problems involving character or number distributions
Let’s explore each of these scenarios in detail.
1. Counting Element Frequencies
One of the most common use cases for count arrays is to efficiently count the frequency of elements in a collection, such as an array or a string. This is particularly useful when dealing with a limited range of values.
Example: Counting the frequency of characters in a string
public static int[] countCharacters(String s) {
int[] count = new int[26]; // Assuming lowercase English letters only
for (char c : s.toCharArray()) {
count[c - 'a']++;
}
return count;
}
// Usage
String s = "hello";
int[] charCounts = countCharacters(s);
System.out.println("Frequency of 'l': " + charCounts['l' - 'a']); // Output: 2
In this example, we use a count array of size 26 to represent the lowercase English alphabet. Each character’s frequency is stored at its corresponding index (e.g., ‘a’ at index 0, ‘b’ at index 1, etc.).
2. Efficient Lookup of Occurrence Counts
Count arrays provide O(1) time complexity for looking up the occurrence count of an element, making them extremely efficient for frequency-based queries.
Example: Checking if a string is an anagram
public static boolean isAnagram(String s, String t) {
if (s.length() != t.length()) return false;
int[] count = new int[26];
for (int i = 0; i < s.length(); i++) {
count[s.charAt(i) - 'a']++;
count[t.charAt(i) - 'a']--;
}
for (int i : count) {
if (i != 0) return false;
}
return true;
}
// Usage
System.out.println(isAnagram("anagram", "nagaram")); // Output: true
System.out.println(isAnagram("rat", "car")); // Output: false
This algorithm uses a count array to efficiently check if two strings are anagrams. It increments the count for characters in the first string and decrements for characters in the second string. If the strings are anagrams, all counts should be zero at the end.
3. Sorting Based on Frequency
Count arrays can be used to implement efficient sorting algorithms based on the frequency of elements, such as counting sort.
Example: Sorting an array of integers using counting sort
public static void countingSort(int[] arr) {
int max = Arrays.stream(arr).max().getAsInt();
int min = Arrays.stream(arr).min().getAsInt();
int range = max - min + 1;
int[] count = new int[range];
int[] output = new int[arr.length];
for (int i : arr) {
count[i - min]++;
}
for (int i = 1; i < count.length; i++) {
count[i] += count[i - 1];
}
for (int i = arr.length - 1; i >= 0; i--) {
output[count[arr[i] - min] - 1] = arr[i];
count[arr[i] - min]--;
}
System.arraycopy(output, 0, arr, 0, arr.length);
}
// Usage
int[] arr = {4, 2, 2, 8, 3, 3, 1};
countingSort(arr);
System.out.println(Arrays.toString(arr)); // Output: [1, 2, 2, 3, 3, 4, 8]
Counting sort uses a count array to determine the position of each element in the sorted output. This algorithm is particularly efficient when the range of input values is not significantly larger than the number of elements to be sorted.
4. Solving Distribution-based Problems
Count arrays are invaluable when solving problems that involve the distribution of characters or numbers, such as finding the most frequent element or checking for balanced distributions.
Example: Finding the most frequent element in an array
public static int mostFrequent(int[] arr) {
Map<Integer, Integer> count = new HashMap<>();
int maxCount = 0;
int mostFrequent = arr[0];
for (int num : arr) {
count.put(num, count.getOrDefault(num, 0) + 1);
if (count.get(num) > maxCount) {
maxCount = count.get(num);
mostFrequent = num;
}
}
return mostFrequent;
}
// Usage
int[] arr = {1, 3, 2, 1, 4, 1};
System.out.println("Most frequent element: " + mostFrequent(arr)); // Output: 1
In this example, we use a HashMap as a count array to find the most frequent element in the array. While not a traditional array, this approach demonstrates the concept of counting occurrences to solve a distribution-based problem.
Advantages of Using Count Arrays
Now that we’ve seen some practical examples, let’s discuss the advantages of using count arrays:
- Efficiency: Count arrays provide O(1) time complexity for lookups and updates, making them extremely efficient for frequency-based operations.
- Simplicity: The concept is straightforward and easy to implement, making count arrays a go-to solution for many frequency-related problems.
- Space-Time Tradeoff: While they may use additional memory, count arrays often lead to significant time savings in algorithms.
- Versatility: They can be used to solve a wide range of problems, from basic counting to more complex algorithmic challenges.
Limitations and Considerations
While count arrays are powerful, they do have some limitations to keep in mind:
- Memory Usage: For large ranges of values, count arrays can consume significant memory. Consider using more memory-efficient data structures like hash maps for sparse data.
- Range Constraints: Traditional count arrays work best with a known, limited range of values. For unbounded or very large ranges, alternative approaches may be necessary.
- Negative Numbers: Basic count arrays don’t handle negative numbers well. You may need to offset the indices or use a different approach for datasets with negative values.
Advanced Applications of Count Arrays
Beyond the basic use cases, count arrays can be applied to solve more complex problems. Let’s explore some advanced applications:
1. Sliding Window Problems
Count arrays are often used in sliding window problems, especially when dealing with strings or arrays with a limited range of elements.
Example: Find all anagrams in a string
public static List<Integer> findAnagrams(String s, String p) {
List<Integer> result = new ArrayList<>();
if (s.length() < p.length()) return result;
int[] count = new int[26];
for (char c : p.toCharArray()) {
count[c - 'a']++;
}
int left = 0, right = 0;
int counter = p.length();
while (right < s.length()) {
if (count[s.charAt(right) - 'a'] > 0) {
counter--;
}
count[s.charAt(right) - 'a']--;
right++;
if (counter == 0) {
result.add(left);
}
if (right - left == p.length()) {
if (count[s.charAt(left) - 'a'] >= 0) {
counter++;
}
count[s.charAt(left) - 'a']++;
left++;
}
}
return result;
}
// Usage
String s = "cbaebabacd";
String p = "abc";
System.out.println("Anagram start indices: " + findAnagrams(s, p)); // Output: [0, 6]
This algorithm uses a count array to efficiently find all anagrams of a pattern string within a larger string. It demonstrates how count arrays can be combined with the sliding window technique to solve more complex string manipulation problems.
2. Substring Problems
Count arrays are particularly useful in solving substring-related problems, especially when dealing with character frequencies.
Example: Longest Substring with At Most K Distinct Characters
public static int lengthOfLongestSubstringKDistinct(String s, int k) {
if (s == null || s.length() == 0 || k == 0) return 0;
int[] count = new int[256];
int num = 0, i = 0, res = 0;
for (int j = 0; j < s.length(); j++) {
if (count[s.charAt(j)]++ == 0) num++;
while (num > k) {
if (--count[s.charAt(i++)] == 0) num--;
}
res = Math.max(res, j - i + 1);
}
return res;
}
// Usage
String s = "eceba";
int k = 2;
System.out.println("Length of longest substring: " + lengthOfLongestSubstringKDistinct(s, k)); // Output: 3
This algorithm uses a count array to keep track of character frequencies in the current window. It efficiently solves the problem of finding the longest substring with at most K distinct characters.
3. Palindrome Problems
Count arrays can be instrumental in solving palindrome-related problems, especially when dealing with character frequencies.
Example: Longest Palindrome
public static int longestPalindrome(String s) {
int[] count = new int[128];
for (char c: s.toCharArray())
count[c]++;
int ans = 0;
for (int v: count) {
ans += v / 2 * 2;
if (ans % 2 == 0 && v % 2 == 1)
ans++;
}
return ans;
}
// Usage
String s = "abccccdd";
System.out.println("Length of longest palindrome: " + longestPalindrome(s)); // Output: 7
This algorithm uses a count array to determine the length of the longest palindrome that can be built with the characters in the given string. It demonstrates how count arrays can be used to solve problems involving character distribution and palindrome construction.
Count Arrays in Interview Scenarios
Count arrays are a popular topic in coding interviews, especially for problems involving strings, character frequencies, and sorting. Here are some tips for using count arrays effectively in interview scenarios:
- Recognize the Pattern: Look for problems involving frequency counting, character distribution, or sorting within a limited range of values.
- Consider the Range: Assess whether the range of possible values is small enough to make a count array feasible. If not, consider using a hash map instead.
- Optimize for Space: If memory usage is a concern, discuss potential optimizations or alternative approaches with your interviewer.
- Explain Your Reasoning: Clearly articulate why you’re choosing to use a count array and how it benefits your solution in terms of time and space complexity.
- Handle Edge Cases: Remember to consider edge cases, such as empty inputs or inputs with unexpected values.
Conclusion
Count arrays are a powerful tool in a programmer’s arsenal, offering efficient solutions to a wide range of problems involving frequency counting, sorting, and character distribution. By understanding when and how to use count arrays, you can significantly improve the efficiency of your algorithms and tackle complex problems with elegance and simplicity.
As you continue to develop your programming skills, keep count arrays in mind as a valuable technique for optimizing your code and solving algorithmic challenges. Practice implementing count arrays in various scenarios to become more comfortable with their usage and to recognize opportunities where they can be applied effectively.
Remember, the key to mastering any programming technique is practice and application. Try incorporating count arrays into your solutions for relevant coding problems, and you’ll soon find yourself reaching for this powerful tool with confidence in both interview situations and real-world programming tasks.