When to Consider Using a Circular Buffer: A Comprehensive Guide
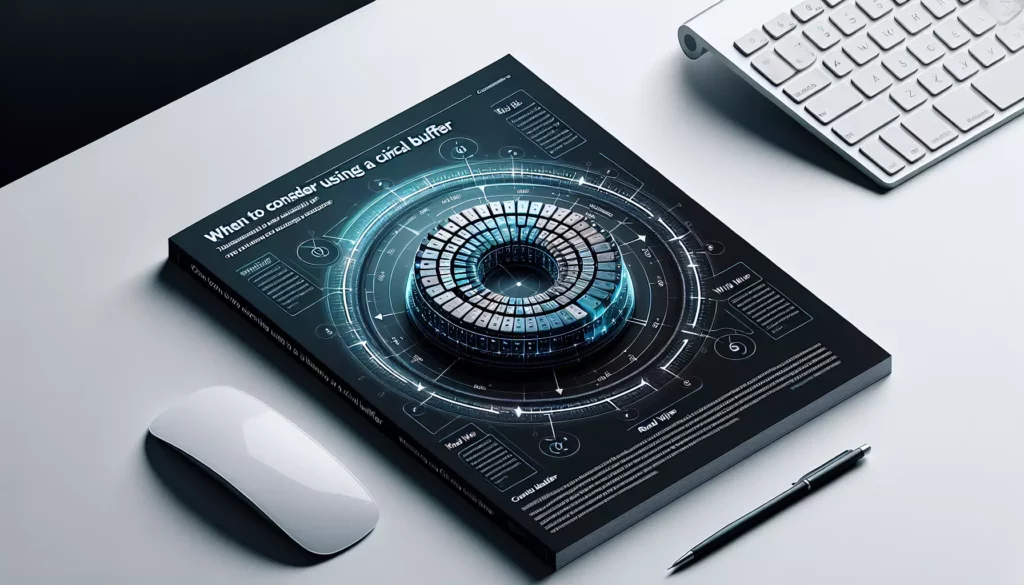
In the world of programming and data structures, efficiency and optimization are key. One data structure that often flies under the radar but can be incredibly useful in certain scenarios is the circular buffer, also known as a ring buffer or circular queue. In this comprehensive guide, we’ll explore what a circular buffer is, how it works, and most importantly, when you should consider using one in your projects.
What is a Circular Buffer?
Before we dive into the use cases, let’s start with a clear definition of what a circular buffer is:
A circular buffer is a fixed-size buffer that operates as if the beginning and end of the buffer are connected. When the buffer fills up, adding a new element overwrites the oldest element in the buffer. This structure is particularly useful for buffering data streams or implementing a queue with a fixed maximum size.
The key characteristics of a circular buffer include:
- Fixed size: The buffer has a predetermined maximum capacity.
- Circular nature: When the end is reached, it wraps around to the beginning.
- Overwriting: New elements replace the oldest when the buffer is full.
- Efficient insertion and deletion: Both operations are O(1) time complexity.
How Does a Circular Buffer Work?
To understand when to use a circular buffer, it’s crucial to grasp how it operates. Here’s a simple explanation of its mechanics:
- The buffer is initialized with a fixed size.
- Two pointers are maintained: one for the read position (head) and one for the write position (tail).
- As elements are added, the write pointer advances.
- As elements are removed, the read pointer advances.
- When either pointer reaches the end of the buffer, it wraps around to the beginning.
- If the buffer is full and a new element is added, it overwrites the oldest element.
Here’s a simple implementation of a circular buffer in Python to illustrate these concepts:
class CircularBuffer:
def __init__(self, capacity):
self.buffer = [None] * capacity
self.capacity = capacity
self.size = 0
self.head = 0
self.tail = 0
def is_empty(self):
return self.size == 0
def is_full(self):
return self.size == self.capacity
def enqueue(self, item):
if self.is_full():
# Overwrite the oldest element
self.head = (self.head + 1) % self.capacity
elif not self.is_empty():
self.tail = (self.tail + 1) % self.capacity
self.buffer[self.tail] = item
self.size = min(self.size + 1, self.capacity)
def dequeue(self):
if self.is_empty():
return None
item = self.buffer[self.head]
self.head = (self.head + 1) % self.capacity
self.size -= 1
return item
def peek(self):
if self.is_empty():
return None
return self.buffer[self.head]
This implementation showcases the core operations of a circular buffer: enqueue (add an item), dequeue (remove an item), and peek (view the next item without removing it).
When to Consider Using a Circular Buffer
Now that we understand what a circular buffer is and how it works, let’s explore the scenarios where it shines. Here are the key situations where you should consider using a circular buffer:
1. Buffering Data Streams
One of the most common use cases for circular buffers is in buffering data streams. This is particularly useful in scenarios where you have a producer generating data faster than a consumer can process it, or when you need to smooth out irregular data flows.
Example scenarios:
- Audio/Video streaming: Buffering audio or video data to ensure smooth playback.
- Network packet buffering: Temporarily storing network packets before processing.
- Sensor data collection: Buffering sensor readings in IoT devices.
In these cases, a circular buffer allows you to store a fixed amount of recent data, automatically discarding older data when the buffer is full. This prevents memory overflow while ensuring that the most recent data is always available.
2. Implementing a Fixed-Size Queue
When you need a queue with a maximum size limit, a circular buffer is an excellent choice. Unlike a standard queue that can grow indefinitely, a circular buffer enforces a size limit while maintaining efficient enqueue and dequeue operations.
Example scenarios:
- Task queues with limited capacity
- Caching recent items (e.g., most recent searches, recently viewed items)
- Managing a fixed number of connections in a server
The circular nature ensures that when the queue is full, adding a new item automatically removes the oldest one, maintaining the size limit without additional logic.
3. Real-Time Systems
In real-time systems where predictable performance is crucial, circular buffers are often employed due to their constant-time operations and fixed memory usage.
Example scenarios:
- Embedded systems with limited resources
- Real-time audio processing
- High-frequency trading systems
The predictable behavior of circular buffers makes them ideal for systems where timing is critical and memory allocation must be carefully controlled.
4. Implementing Undo/Redo Functionality
Circular buffers can be an efficient way to implement undo/redo functionality in applications, especially when you want to limit the number of actions that can be undone.
Example scenarios:
- Text editors
- Graphics software
- Game state management
By using a circular buffer, you can store a fixed number of recent actions or states, allowing users to undo and redo within that limit.
5. Logging and Monitoring
Circular buffers are excellent for implementing logging systems, especially when you want to maintain a fixed-size log of recent events.
Example scenarios:
- System logs with a rolling window
- Performance monitoring with recent metrics
- Error tracking with the most recent errors
This approach ensures that you always have the most recent log entries available without consuming unlimited memory.
Advantages of Using Circular Buffers
Understanding the advantages of circular buffers can help you make an informed decision about when to use them. Here are some key benefits:
1. Efficient Memory Usage
Circular buffers use a fixed amount of memory, which is allocated upfront. This predictable memory usage is particularly beneficial in systems with limited resources or where memory management is critical.
2. Constant-Time Operations
Both enqueue and dequeue operations in a circular buffer are O(1) time complexity. This constant-time performance is crucial for real-time applications and high-performance systems.
3. No Need for Resizing
Unlike dynamic arrays or standard queues that may need to resize as they grow, circular buffers have a fixed size. This eliminates the overhead and unpredictability associated with resizing operations.
4. Automatic Overwriting of Old Data
In scenarios where you only need to keep the most recent data, circular buffers automatically overwrite old data when full. This simplifies the logic for managing data with a sliding window.
5. Lock-Free Implementations
For multi-threaded applications, it’s possible to implement lock-free circular buffers, which can provide high-performance concurrent access in producer-consumer scenarios.
Limitations and Considerations
While circular buffers are powerful in certain scenarios, they’re not a one-size-fits-all solution. Here are some limitations and considerations to keep in mind:
1. Fixed Size
The fixed size of a circular buffer can be both an advantage and a limitation. If you’re unsure about the maximum size you’ll need, or if the size requirements can change dramatically, a circular buffer might not be the best choice.
2. Data Loss
In scenarios where the buffer fills up, new data overwrites old data. If preserving all data is crucial, and you can’t guarantee that data will be processed before the buffer fills, a circular buffer might lead to unacceptable data loss.
3. Complexity in Some Languages
While the concept of a circular buffer is straightforward, implementing one efficiently can be tricky in some programming languages, especially those without direct memory management.
4. Not Suitable for Random Access
If you need frequent random access to elements in the middle of the buffer, a circular buffer might not be the most efficient structure. It’s optimized for FIFO (First-In-First-Out) access patterns.
Implementing a Circular Buffer: Best Practices
If you’ve decided that a circular buffer is the right choice for your project, here are some best practices to keep in mind during implementation:
1. Use Power-of-Two Sizes
When possible, use buffer sizes that are powers of two. This allows you to use bitwise operations for wrapping around, which can be more efficient than modulo operations.
// Instead of:
index = (index + 1) % bufferSize;
// You can use:
index = (index + 1) & (bufferSize - 1); // Assuming bufferSize is a power of 2
2. Consider Thread Safety
If your circular buffer will be accessed by multiple threads, ensure thread safety. This might involve using atomic operations, locks, or implementing a lock-free algorithm.
3. Implement Clear Error Handling
Decide how to handle edge cases, such as trying to dequeue from an empty buffer or enqueue to a full buffer when overwriting is not allowed. Clear error handling will make your code more robust and easier to use.
4. Use Appropriate Data Types
Choose appropriate data types for your buffer indices. For very large buffers, ensure that your index variables won’t overflow.
5. Consider Providing Additional Operations
Depending on your use case, consider implementing additional operations such as:
- peek(): View the next item without removing it
- clear(): Reset the buffer to empty
- isFull(): Check if the buffer is full
- size(): Get the current number of elements in the buffer
Real-World Examples of Circular Buffer Usage
To further illustrate when and how circular buffers are used in practice, let’s look at some real-world examples:
1. Audio Processing in Digital Signal Processors (DSPs)
In digital audio processing, circular buffers are often used to implement delay lines and reverb effects. The circular nature of the buffer allows for efficient implementation of these time-based effects.
class DelayLine {
private:
CircularBuffer<float> buffer;
int delayLength;
public:
DelayLine(int maxDelay) : buffer(maxDelay), delayLength(maxDelay) {}
float process(float input) {
float delayed = buffer.peek();
buffer.enqueue(input);
return delayed;
}
void setDelay(int newDelay) {
delayLength = std::min(newDelay, buffer.capacity());
}
};
2. Network Packet Buffering
In network programming, circular buffers are used to temporarily store incoming packets before they can be processed. This helps manage situations where packets arrive faster than they can be handled.
class PacketBuffer {
private:
CircularBuffer<Packet> buffer;
public:
PacketBuffer(int capacity) : buffer(capacity) {}
void receivePacket(const Packet& packet) {
if (buffer.is_full()) {
// Log or handle buffer overflow
std::cout << "Buffer overflow, dropping oldest packet" << std::endl;
}
buffer.enqueue(packet);
}
void processPackets() {
while (!buffer.is_empty()) {
Packet packet = buffer.dequeue();
// Process the packet
processPacket(packet);
}
}
};
3. Undo/Redo in Text Editors
Text editors often use circular buffers to implement undo/redo functionality with a limited history.
class TextEditor {
private:
std::string text;
CircularBuffer<std::string> undoBuffer;
CircularBuffer<std::string> redoBuffer;
public:
TextEditor(int historySize) : undoBuffer(historySize), redoBuffer(historySize) {}
void applyEdit(const std::string& newText) {
undoBuffer.enqueue(text);
text = newText;
redoBuffer.clear(); // Clear redo history on new edit
}
void undo() {
if (!undoBuffer.is_empty()) {
redoBuffer.enqueue(text);
text = undoBuffer.dequeue();
}
}
void redo() {
if (!redoBuffer.is_empty()) {
undoBuffer.enqueue(text);
text = redoBuffer.dequeue();
}
}
};
Conclusion
Circular buffers are a powerful and efficient data structure that can significantly improve the performance and resource management of your applications in specific scenarios. They are particularly useful in situations involving data streaming, fixed-size queues, real-time systems, and scenarios where you need to maintain a rolling window of recent data.
Key takeaways for when to consider using a circular buffer:
- When you need to buffer streaming data with a fixed maximum size
- For implementing queues with a size limit
- In real-time systems where predictable performance is crucial
- For undo/redo functionality with a limited history
- In logging and monitoring systems with a rolling window of recent events
Remember to consider the limitations of circular buffers, such as their fixed size and potential for data loss when full. Always evaluate your specific requirements and constraints when choosing a data structure for your project.
By understanding when and how to use circular buffers effectively, you can optimize your code for better performance and resource management in these specific scenarios. As with any programming concept, practice and real-world application will help solidify your understanding and ability to leverage circular buffers in your projects.