When and How to Ask Clarifying Questions About Edge Cases
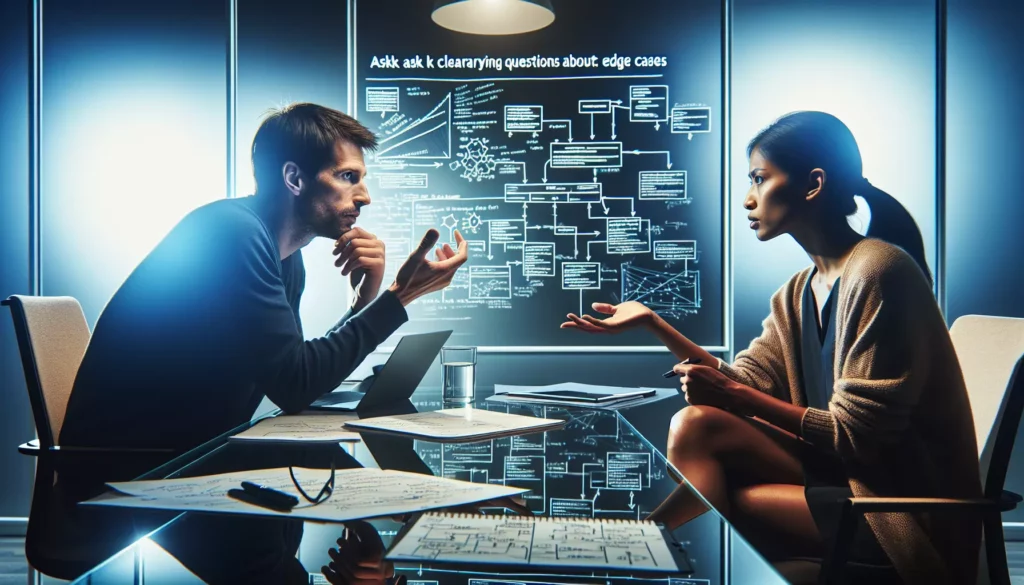
As software developers, we often encounter situations where we need to handle edge cases – those unusual or extreme scenarios that can occur in our code. Understanding and addressing these edge cases is crucial for creating robust and reliable software. However, it’s not always clear when and how to ask clarifying questions about these edge cases during the development process. In this comprehensive guide, we’ll explore the importance of identifying edge cases, when to ask questions about them, and how to frame those questions effectively.
The Importance of Edge Cases in Software Development
Before diving into the specifics of asking questions about edge cases, let’s first understand why they are so crucial in software development:
- Reliability: Handling edge cases properly ensures that your software works correctly under various conditions, including unexpected or rare scenarios.
- User Experience: By addressing edge cases, you can provide a smoother and more consistent user experience, even in unusual situations.
- Bug Prevention: Identifying and handling edge cases early in the development process can prevent bugs and issues from arising later.
- Performance Optimization: Understanding edge cases can help you optimize your code for better performance across different scenarios.
- Security: Many security vulnerabilities arise from unhandled edge cases, making their identification crucial for maintaining secure software.
When to Ask Clarifying Questions About Edge Cases
Knowing when to ask questions about edge cases is as important as knowing how to ask them. Here are some key moments in the development process when you should consider raising questions about potential edge cases:
1. During Requirements Gathering
The requirements gathering phase is an ideal time to start thinking about edge cases. As you discuss the project’s goals and functionality with stakeholders, consider asking:
- What are the limits or boundaries of the system?
- Are there any rare or unusual scenarios we should account for?
- How should the system behave when it encounters unexpected input?
2. In Design Discussions
When you’re designing the architecture or specific components of your software, it’s crucial to consider edge cases. During design discussions, ask:
- How will our design handle extreme data loads or user behaviors?
- What fail-safes or error handling mechanisms should we incorporate?
- Are there any potential conflicts between different parts of the system in edge scenarios?
3. During Code Reviews
Code reviews provide an excellent opportunity to identify and discuss potential edge cases. When reviewing code, consider asking:
- How does this function handle null or empty inputs?
- What happens if this loop encounters an unexpectedly large dataset?
- Are there any assumptions in this code that might not always hold true?
4. In Testing Phases
Testing is where many edge cases are discovered. During testing phases, ask:
- Have we tested the system with boundary values and extreme inputs?
- What happens when the system encounters unexpected or malformed data?
- Are there any race conditions or timing-related edge cases we should consider?
5. When Refactoring or Optimizing Code
When you’re improving existing code, it’s a good time to reconsider edge cases. Ask:
- Does this optimization maintain the handling of all previously considered edge cases?
- Are there any new edge cases introduced by these changes?
- How do these refactoring efforts impact the system’s behavior in extreme scenarios?
How to Ask Clarifying Questions About Edge Cases
Now that we know when to ask questions about edge cases, let’s explore how to frame these questions effectively:
1. Be Specific and Provide Context
When asking about edge cases, it’s important to be as specific as possible and provide context. Instead of asking a vague question like “What about edge cases?”, try something more specific:
“I noticed that our user registration function doesn’t handle cases where the email field is left empty. How should we handle this scenario?”
2. Use Examples
Providing concrete examples can help illustrate the edge case you’re concerned about. For instance:
“If a user tries to divide by zero in our calculator app, what should the output be? Should we display an error message or return a special value like Infinity?”
3. Consider the Impact
When asking about an edge case, it’s helpful to consider and communicate its potential impact. This can help prioritize the discussion:
“If our system doesn’t handle concurrent writes to the same database record, we might end up with data inconsistencies. How critical is this scenario, and how should we address it?”
4. Propose Potential Solutions
When possible, come prepared with potential solutions or approaches to handling the edge case. This shows initiative and can lead to more productive discussions:
“I’ve identified that our sorting algorithm doesn’t handle arrays with duplicate values correctly. We could modify it to use a stable sort or implement a custom comparator. Which approach do you think would be more appropriate?”
5. Ask About Testing Strategies
When discussing edge cases, it’s also valuable to inquire about how to test for them:
“We’ve identified several edge cases for our file upload feature. What testing strategies should we employ to ensure we’re covering these scenarios adequately?”
Examples of Clarifying Questions for Common Edge Cases
Let’s look at some examples of how to ask clarifying questions for common edge cases in different programming scenarios:
1. Input Validation
function processUserInput(input: string): void {
// Process the input
}
Clarifying question: “How should we handle cases where the user input is an empty string or contains only whitespace? Should we throw an error, return early, or process it as is?”
2. Array Operations
function findMaximum(numbers: number[]): number {
// Find and return the maximum number
}
Clarifying question: “What should our function return if the input array is empty? Should we throw an error, return a special value like null, or perhaps return negative infinity?”
3. Asynchronous Operations
async function fetchUserData(userId: string): Promise<UserData> {
// Fetch and return user data
}
Clarifying question: “How long should we wait for the user data to be fetched before timing out? And what should be our strategy if the fetch operation fails – should we retry, and if so, how many times?”
4. File Operations
function saveFile(data: string, path: string): void {
// Save data to file at specified path
}
Clarifying question: “What should happen if a file already exists at the specified path? Should we overwrite it, append to it, or throw an error and ask the user for confirmation?”
5. Numeric Calculations
function calculatePercentage(value: number, total: number): number {
// Calculate and return percentage
}
Clarifying question: “How should we handle cases where the total is zero? Should we return 0%, throw an error, or return a special value to indicate an undefined result?”
Strategies for Identifying Edge Cases
To ask effective questions about edge cases, you first need to be able to identify them. Here are some strategies to help you spot potential edge cases:
1. Boundary Value Analysis
Consider the extreme ends of your input ranges. For example, if a function accepts numbers between 1 and 100, test with 0, 1, 100, and 101.
2. Equivalence Partitioning
Divide your input domain into classes of equivalent inputs and test with representatives from each class, including the boundaries between classes.
3. Error Guessing
Based on your experience, try to guess where errors are likely to occur. Common areas include null inputs, empty collections, and maximum/minimum values.
4. State Transition Analysis
If your system has different states, consider what happens during state transitions, especially unexpected or rapid transitions.
5. Load and Stress Testing
Think about how your system behaves under extreme loads or stress. This can reveal edge cases related to performance and resource management.
6. Timing and Concurrency Analysis
Consider scenarios involving precise timing or concurrent operations, which can often lead to race conditions or deadlocks.
The Role of Edge Cases in Technical Interviews
Understanding edge cases is not just crucial for real-world development; it’s also a key aspect of technical interviews, especially for major tech companies. Here’s how edge cases typically come into play during interviews:
1. Problem-Solving Questions
Interviewers often present coding problems and expect candidates to consider and handle edge cases. For example, if you’re asked to implement a function that reverses a string, the interviewer will likely expect you to consider cases like empty strings or strings with a single character.
2. Follow-Up Questions
Even if you provide a working solution, interviewers might ask about specific edge cases to test your thoroughness and attention to detail. Be prepared to explain how your solution handles various scenarios.
3. System Design Interviews
In system design interviews, discussing potential edge cases demonstrates your ability to think critically about large-scale systems. For instance, you might need to consider how a social media platform handles viral posts or how an e-commerce site manages flash sales.
4. Behavioral Questions
Some behavioral questions might ask about times when you encountered and resolved issues related to edge cases in your previous work. Having specific examples ready can be beneficial.
Best Practices for Handling Edge Cases
As you become more adept at identifying and asking about edge cases, it’s important to develop good practices for handling them in your code. Here are some best practices to consider:
1. Validate Inputs
Always validate inputs to your functions or methods. This can prevent many edge cases from causing issues later in your code.
function processUserData(userData: UserData): void {
if (!userData || typeof userData !== 'object') {
throw new Error('Invalid user data');
}
// Process valid user data
}
2. Use Default Values
When appropriate, use default values to handle cases where expected inputs are missing or invalid.
function greetUser(name: string = 'Guest'): string {
return `Hello, ${name}!`;
}
3. Implement Proper Error Handling
Use try-catch blocks and throw meaningful errors when encountering edge cases that can’t be handled gracefully.
function divideNumbers(a: number, b: number): number {
if (b === 0) {
throw new Error('Division by zero is not allowed');
}
return a / b;
}
4. Write Comprehensive Tests
Include unit tests that specifically target edge cases. This ensures that your handling of these cases doesn’t regress over time.
describe('divideNumbers', () => {
it('should throw an error when dividing by zero', () => {
expect(() => divideNumbers(10, 0)).toThrow('Division by zero is not allowed');
});
});
5. Document Edge Case Behavior
Clearly document how your code handles edge cases, especially if the behavior might not be immediately obvious to other developers.
/**
* Calculates the average of an array of numbers.
* @param {number[]} numbers - The array of numbers to average.
* @returns {number} The average of the numbers, or 0 if the array is empty.
*/
function calculateAverage(numbers: number[]): number {
if (numbers.length === 0) {
return 0; // Return 0 for empty arrays
}
const sum = numbers.reduce((acc, num) => acc + num, 0);
return sum / numbers.length;
}
Conclusion
Asking clarifying questions about edge cases is a crucial skill for any software developer. It demonstrates thoroughness, attention to detail, and a commitment to creating robust and reliable software. By knowing when and how to ask these questions, you can significantly improve the quality of your code and your team’s overall output.
Remember, the goal isn’t to anticipate and handle every possible edge case – that can lead to overly complex and hard-to-maintain code. Instead, focus on identifying and addressing the most important and likely edge cases that could significantly impact your software’s functionality, performance, or user experience.
As you continue to develop your skills in this area, you’ll find that considering edge cases becomes a natural part of your development process. This mindset will not only make you a better developer but also prepare you for technical interviews and real-world challenges in your software development career.
Keep practicing, stay curious, and don’t be afraid to ask those important questions about edge cases. Your code – and your users – will thank you for it!