What You Can Learn About Programming from Solving Everyday Problems
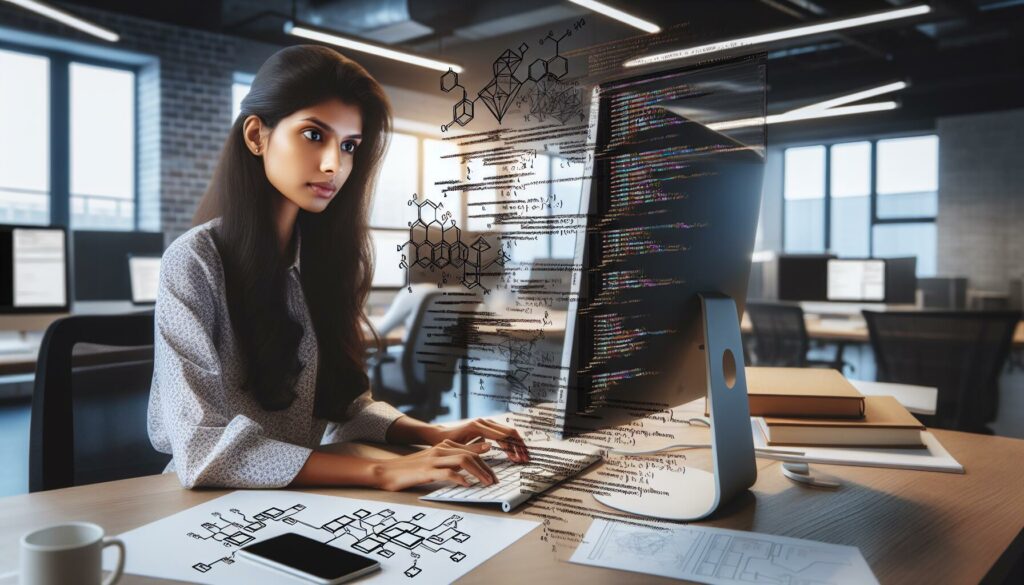
Programming is often perceived as a complex, technical skill reserved for those who spend their days hunched over computers in dimly lit rooms. However, the core principles of programming are deeply rooted in everyday problem-solving skills that we all use, often without realizing it. By understanding this connection, we can demystify programming and make it more accessible to everyone. In this article, we’ll explore how everyday problem-solving relates to programming concepts and how you can leverage this knowledge to become a better coder.
The Parallels Between Everyday Problems and Programming
Before we dive into specific examples, let’s consider the general parallels between solving everyday problems and writing code:
- Breaking down complex tasks: Just as you might break down the process of cooking a meal into smaller steps, programmers break down complex problems into manageable chunks of code.
- Following a logical sequence: Whether you’re assembling furniture or writing a function, you need to follow a logical order of operations to achieve the desired result.
- Handling variables: In cooking, you adjust ingredients based on the number of people you’re serving. In programming, you use variables to store and manipulate data.
- Making decisions: Everyday life is full of if-then scenarios, which directly translate to conditional statements in programming.
- Repetition: Many tasks in life involve repetition, just as loops are used in programming to perform actions multiple times.
Now, let’s explore some specific everyday scenarios and their programming counterparts.
1. Recipe Following: The Essence of Algorithms
Following a recipe is perhaps one of the most straightforward analogies to programming. A recipe is essentially an algorithm – a step-by-step set of instructions to achieve a specific outcome. Let’s break down how a simple recipe relates to programming concepts:
The Recipe:
1. Preheat oven to 350°F (175°C) 2. Mix 2 cups of flour, 1 cup of sugar, and 1 teaspoon of baking powder in a bowl 3. In another bowl, beat 2 eggs and add 1 cup of milk 4. Combine wet and dry ingredients 5. Pour batter into a greased baking pan 6. Bake for 30 minutes or until a toothpick comes out clean
The Programming Equivalent:
def bake_cake():
preheat_oven(350)
dry_ingredients = mix(["2 cups flour", "1 cup sugar", "1 tsp baking powder"])
wet_ingredients = beat_eggs(2) + "1 cup milk"
batter = combine(dry_ingredients, wet_ingredients)
pan = grease_pan()
pour(batter, pan)
bake(30)
if toothpick_test():
return "Cake is ready!"
else:
return "Bake for a few more minutes"
bake_cake()
This simple example illustrates several programming concepts:
- Functions: The entire recipe is encapsulated in a function called
bake_cake()
. - Variables: Ingredients are stored in variables like
dry_ingredients
andwet_ingredients
. - Conditional statements: The toothpick test is an if-else statement in programming.
- Return values: The function returns a string indicating whether the cake is ready or needs more time.
2. Navigation: Understanding Algorithms and Optimization
Consider the everyday task of finding the best route to work. This process involves several key programming concepts:
The Everyday Scenario:
- You have multiple route options
- You consider factors like traffic, distance, and time
- You might check real-time updates for road closures or accidents
- You choose the optimal route based on your priorities
The Programming Equivalent:
def find_best_route(start, end, priority):
routes = get_possible_routes(start, end)
for route in routes:
route.distance = calculate_distance(route)
route.estimated_time = estimate_time(route)
route.traffic_level = check_traffic(route)
route.score = calculate_score(route, priority)
best_route = max(routes, key=lambda x: x.score)
return best_route
start_point = "Home"
end_point = "Office"
priority = "Fastest"
optimal_route = find_best_route(start_point, end_point, priority)
print(f"The best route is: {optimal_route}")
This example demonstrates several advanced programming concepts:
- Data structures: Routes are likely represented as objects or dictionaries with multiple attributes.
- Loops: We iterate through each route to calculate its properties.
- Functions: Various functions are used to calculate distance, estimate time, etc.
- Optimization: We’re essentially solving an optimization problem by scoring each route based on the user’s priority.
- Lambda functions: Used here to define a key for finding the route with the maximum score.
3. Budgeting: Variables, Calculations, and Conditional Logic
Personal budgeting is an excellent example of how we use programming-like thinking in our daily lives. Let’s break down a simple budgeting process:
The Everyday Scenario:
- Calculate total income
- List all expenses
- Subtract expenses from income
- If there’s money left, allocate to savings or discretionary spending
- If expenses exceed income, identify areas to cut back
The Programming Equivalent:
def create_budget(income, expenses):
total_expenses = sum(expenses.values())
balance = income - total_expenses
if balance >= 0:
savings = balance * 0.5
discretionary = balance * 0.5
return f"Budget Balanced! Savings: ${savings:.2f}, Discretionary: ${discretionary:.2f}"
else:
deficit = abs(balance)
return f"Budget Deficit of ${deficit:.2f}. Need to reduce expenses."
income = 5000
expenses = {
"rent": 1500,
"utilities": 200,
"groceries": 400,
"transportation": 300,
"entertainment": 200
}
result = create_budget(income, expenses)
print(result)
This budgeting example showcases several programming concepts:
- Dictionaries: Expenses are stored in a dictionary, allowing for easy categorization and access.
- Arithmetic operations: We perform addition and subtraction to calculate the balance.
- Conditional statements: We use an if-else statement to handle different scenarios based on the balance.
- String formatting: We use f-strings to format the output with calculated values.
- Functions: The entire budgeting process is encapsulated in a function that takes income and expenses as parameters.
4. Time Management: Scheduling and Priority Queues
Effective time management is crucial in both everyday life and programming. Let’s explore how managing your daily tasks relates to programming concepts:
The Everyday Scenario:
- List all tasks for the day
- Assign priorities to each task
- Estimate time required for each task
- Schedule tasks based on priority and time constraints
- Adjust schedule as new tasks arise or priorities change
The Programming Equivalent:
import heapq
from dataclasses import dataclass, field
from typing import List
@dataclass(order=True)
class Task:
priority: int
description: str = field(compare=False)
estimated_time: int = field(compare=False)
class Schedule:
def __init__(self):
self.tasks = []
def add_task(self, priority, description, estimated_time):
heapq.heappush(self.tasks, Task(priority, description, estimated_time))
def get_next_task(self):
return heapq.heappop(self.tasks) if self.tasks else None
def total_time(self):
return sum(task.estimated_time for task in self.tasks)
# Usage
schedule = Schedule()
schedule.add_task(1, "Finish project report", 120)
schedule.add_task(2, "Respond to emails", 30)
schedule.add_task(1, "Prepare for meeting", 60)
print(f"Total scheduled time: {schedule.total_time()} minutes")
while True:
next_task = schedule.get_next_task()
if not next_task:
break
print(f"Next task: {next_task.description} (Est. time: {next_task.estimated_time} min)")
This time management example introduces several advanced programming concepts:
- Data classes: We use Python’s
dataclass
to create aTask
class with built-in comparison methods. - Priority queues: We use Python’s
heapq
module to implement a priority queue for tasks. - Object-Oriented Programming (OOP): The
Schedule
class encapsulates the logic for managing tasks. - List comprehensions: Used in the
total_time()
method to sum up estimated times. - While loops: Used to continuously retrieve and display tasks until the schedule is empty.
5. Shopping: Lists, Sorting, and Searching
The process of creating a shopping list and navigating a store efficiently involves several programming concepts. Let’s explore this everyday scenario:
The Everyday Scenario:
- Create a list of items to buy
- Organize the list by store sections (produce, dairy, etc.)
- Check for any special deals or coupons
- Navigate the store efficiently
- Cross off items as you find them
The Programming Equivalent:
from collections import defaultdict
def create_shopping_list():
return [
("apples", "produce"),
("milk", "dairy"),
("bread", "bakery"),
("eggs", "dairy"),
("tomatoes", "produce"),
("cheese", "dairy"),
]
def organize_by_section(shopping_list):
organized = defaultdict(list)
for item, section in shopping_list:
organized[section].append(item)
return organized
def find_deals(shopping_list, deals):
return [item for item, _ in shopping_list if item in deals]
def shop(organized_list):
cart = []
for section, items in organized_list.items():
print(f"Now in {section}:")
for item in items:
cart.append(item)
print(f" Added {item} to cart")
return cart
# Usage
shopping_list = create_shopping_list()
organized_list = organize_by_section(shopping_list)
deals = {"apples", "bread", "cheese"}
print("Items on sale:", find_deals(shopping_list, deals))
print("\nShopping:")
final_cart = shop(organized_list)
print(f"\nFinal cart: {final_cart}")
This shopping example demonstrates several important programming concepts:
- Tuples: Each item in the shopping list is represented as a tuple of (item, section).
- Defaultdict: Used to easily group items by section without explicitly handling the case of a new section.
- List comprehensions: Used in the
find_deals
function to efficiently filter items. - Sets: The
deals
variable is a set, allowing for fast membership testing. - Iteration: We iterate over the organized list to simulate moving through the store.
Applying Everyday Problem-Solving Skills to Programming
Now that we’ve explored these everyday scenarios and their programming counterparts, let’s discuss how you can leverage these insights to improve your programming skills:
1. Break Down Problems
Just as you break down complex tasks in daily life, practice breaking down programming problems into smaller, manageable steps. This approach, known as “decomposition” in computer science, is crucial for tackling complex coding challenges.
2. Think Algorithmically
Start viewing everyday tasks as algorithms. Try to identify the steps, decision points, and repetitions in your daily routines. This mindset will help you approach programming problems more systematically.
3. Recognize Patterns
Look for patterns in how you solve different problems. Do you use similar approaches for certain types of tasks? In programming, recognizing and applying common patterns (like design patterns) can greatly improve your code’s efficiency and readability.
4. Embrace Optimization
Just as you might look for the fastest route to work or the most efficient way to complete chores, always consider optimization in your code. Look for ways to make your algorithms faster or use less memory.
5. Learn from Mistakes
When something goes wrong in daily life, you typically analyze what happened and try to prevent it in the future. Apply this same approach to debugging. Don’t just fix the immediate issue; understand why it occurred and how to prevent similar problems.
6. Collaborate and Communicate
Many everyday problems are solved through collaboration and clear communication. In programming, these skills are equally important. Practice explaining your code and problem-solving approach to others, and be open to feedback and alternative solutions.
Conclusion
Programming is not an isolated skill set reserved for computer scientists. It’s an extension of the problem-solving abilities we use every day. By recognizing the parallels between everyday tasks and programming concepts, you can demystify coding and approach it with more confidence and intuition.
Remember, every time you plan a trip, follow a recipe, or manage your time, you’re essentially “programming” in real life. By consciously applying these problem-solving skills to your coding practice, you’ll find that programming becomes more intuitive and less daunting.
As you continue your journey in learning to code, whether through platforms like AlgoCademy or other resources, keep these connections in mind. Look for opportunities to relate new programming concepts to familiar real-world scenarios. This approach will not only make learning more engaging but also help you develop a deeper understanding of programming principles.
Ultimately, becoming a proficient programmer is about developing a problem-solving mindset. By honing this skill in your everyday life and consciously applying it to your coding practice, you’ll be well on your way to mastering the art of programming.