What to Expect in a Senior Developer Interview: A Comprehensive Guide
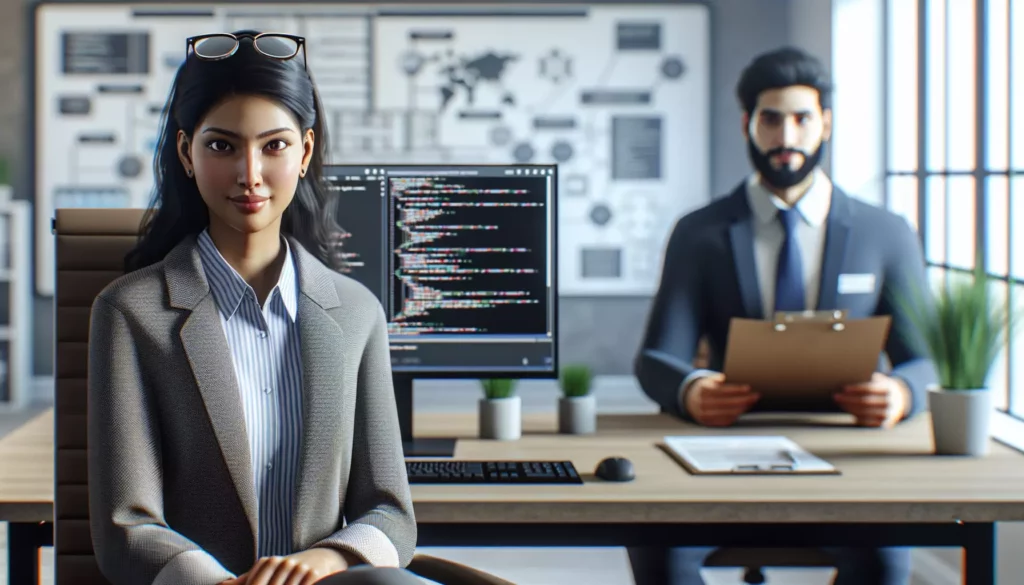
As you progress in your software development career, the prospect of interviewing for a senior developer position can be both exciting and daunting. Senior developer roles come with increased responsibilities, higher expectations, and a more rigorous interview process. This comprehensive guide will walk you through what to expect in a senior developer interview, helping you prepare effectively and boost your chances of success.
1. The Importance of Senior Developer Interviews
Senior developer interviews are crucial for both the candidate and the hiring company. For the candidate, it’s an opportunity to showcase years of experience, technical expertise, and leadership skills. For the company, it’s a chance to assess whether the candidate can contribute significantly to their projects, mentor junior developers, and drive technical decisions.
These interviews are typically more in-depth and multifaceted than those for junior or mid-level positions. They aim to evaluate not just coding skills, but also system design abilities, architectural knowledge, and soft skills like communication and leadership.
2. Common Stages of a Senior Developer Interview Process
While interview processes can vary between companies, most senior developer interviews follow a similar structure:
- Initial phone or video screening
- Technical phone interview
- Take-home coding assignment (optional)
- On-site interviews (or virtual equivalent)
- Final interview with senior management
Let’s dive into each of these stages and what you can expect.
2.1 Initial Phone or Video Screening
This first step is usually a brief conversation with a recruiter or HR representative. They’ll verify your experience, discuss your career goals, and ensure there’s a mutual fit in terms of role expectations and compensation. Be prepared to:
- Summarize your experience and key projects
- Explain why you’re interested in the role and company
- Discuss your salary expectations
- Ask high-level questions about the role and company culture
2.2 Technical Phone Interview
If you pass the initial screening, you’ll likely have a technical phone interview with a senior engineer or hiring manager. This interview focuses on your technical knowledge and problem-solving skills. Expect:
- Questions about your past projects and technical decisions
- Coding problems (usually solved in a shared online editor)
- System design questions
- Discussions about your preferred technologies and why you choose them
2.3 Take-Home Coding Assignment (Optional)
Some companies include a take-home coding assignment in their interview process. This allows you to showcase your skills in a less pressured environment. The assignment might involve:
- Building a small application or feature
- Refactoring existing code
- Solving a complex algorithmic problem
Pay attention to code quality, documentation, and testing in your submission.
2.4 On-Site Interviews
The on-site interview (or its virtual equivalent) is the most comprehensive part of the process. It typically lasts several hours and includes multiple rounds with different interviewers. You can expect:
2.4.1 Coding Interviews
These sessions test your ability to write clean, efficient code under pressure. You might be asked to:
- Solve algorithmic problems on a whiteboard or computer
- Implement a specific feature or data structure
- Debug and optimize existing code
2.4.2 System Design Interview
This crucial component evaluates your ability to design large-scale systems. You might be asked to:
- Design a system like Twitter, Netflix, or Uber
- Discuss trade-offs between different architectural choices
- Explain how you’d handle scalability, reliability, and performance issues
2.4.3 Architecture and Best Practices Discussion
Expect questions about software architecture, design patterns, and best practices. Be prepared to:
- Discuss different architectural styles (e.g., microservices, monolithic)
- Explain when and why you’d use certain design patterns
- Share your approach to code quality, testing, and continuous integration
2.4.4 Behavioral Interview
This assesses your soft skills and cultural fit. You might be asked about:
- Your leadership experience and mentoring approach
- How you handle conflicts or disagreements in a team
- Your biggest professional achievements and challenges
- Your approach to learning and staying updated with new technologies
2.4.5 Team Fit Interview
You may have a session with potential team members to assess how well you’d fit into the team. This could involve:
- Discussing your working style and preferences
- Sharing your thoughts on teamwork and collaboration
- Asking questions about the team’s processes and culture
2.5 Final Interview with Senior Management
If you’ve made it this far, you might have a final interview with senior management or executives. This is often less technical and more focused on your overall fit with the company’s vision and culture.
3. Key Areas to Prepare For
To excel in a senior developer interview, focus on preparing in these key areas:
3.1 Technical Skills
Brush up on your coding skills, focusing on:
- Data structures and algorithms
- Time and space complexity analysis
- Problem-solving strategies
- Language-specific features and best practices
Here’s a simple example of a coding question you might encounter:
// Given an array of integers, return indices of the two numbers
// such that they add up to a specific target.
function twoSum(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
return [];
}
// Example usage:
console.log(twoSum([2, 7, 11, 15], 9)); // Output: [0, 1]
3.2 System Design
Study system design principles and practice designing large-scale systems. Key areas include:
- Scalability and performance optimization
- Database design and selection (SQL vs. NoSQL)
- Caching strategies
- Load balancing and microservices architecture
- API design
3.3 Architecture and Design Patterns
Familiarize yourself with various architectural styles and design patterns. Be prepared to discuss:
- Monolithic vs. microservices architecture
- Event-driven architecture
- Common design patterns (e.g., Singleton, Factory, Observer)
- SOLID principles
3.4 Leadership and Soft Skills
Prepare examples that demonstrate your:
- Leadership and mentoring experience
- Conflict resolution skills
- Ability to communicate technical concepts to non-technical stakeholders
- Experience in making and justifying technical decisions
3.5 Project Experience
Be ready to discuss your past projects in detail, including:
- Technical challenges you faced and how you overcame them
- Architectural decisions you made and their outcomes
- Your role in the project and how you collaborated with others
- Metrics that demonstrate the project’s success
4. Tips for Success in Senior Developer Interviews
4.1 Communicate Clearly
Clear communication is crucial in senior roles. During your interview:
- Explain your thought process as you solve problems
- Use analogies to explain complex concepts
- Ask clarifying questions when needed
- Be concise but thorough in your explanations
4.2 Show Leadership and Mentorship
Demonstrate your ability to lead and mentor by:
- Sharing experiences where you’ve guided junior developers
- Discussing how you’ve influenced technical decisions in past roles
- Explaining your approach to code reviews and knowledge sharing
4.3 Highlight Your Problem-Solving Approach
When solving coding or design problems:
- Start by clarifying requirements and constraints
- Consider multiple approaches before diving into implementation
- Discuss trade-offs between different solutions
- Think about edge cases and potential optimizations
4.4 Demonstrate Continuous Learning
Show your commitment to staying current in the field by:
- Discussing recent technologies or techniques you’ve learned
- Sharing your go-to resources for keeping up with industry trends
- Explaining how you’ve applied new knowledge in your work
4.5 Ask Thoughtful Questions
Prepare insightful questions about the role, team, and company. This shows your genuine interest and helps you assess if the opportunity is right for you. Consider asking about:
- The team’s biggest technical challenges
- How the company approaches professional development
- The roadmap for the products or services you’d be working on
- The company’s approach to work-life balance and remote work (if applicable)
5. Common Mistakes to Avoid
Be aware of these common pitfalls in senior developer interviews:
5.1 Overconfidence
While confidence is important, avoid coming across as arrogant. Be open to feedback and alternative viewpoints.
5.2 Neglecting Soft Skills
Don’t focus solely on technical skills. Emphasize your ability to collaborate, communicate, and lead.
5.3 Failing to Admit Knowledge Gaps
It’s okay not to know everything. If you’re unsure about something, admit it and explain how you’d go about finding the answer.
5.4 Ignoring the Big Picture
Don’t get lost in technical details. Show that you can think at a higher level about architecture, scalability, and business impact.
5.5 Not Asking Questions
Failing to ask thoughtful questions can signal a lack of interest or engagement.
6. Post-Interview Follow-Up
After the interview:
- Send a thank-you email to your interviewers within 24 hours
- Reflect on the interview and note any areas where you could improve
- Follow up with the recruiter if you haven’t heard back within the expected timeframe
7. Conclusion
Interviewing for a senior developer position is a comprehensive process that evaluates your technical skills, system design abilities, leadership potential, and cultural fit. By thoroughly preparing in each of these areas and following the tips outlined in this guide, you’ll be well-equipped to showcase your expertise and land that senior developer role.
Remember, the key to success lies not just in your technical prowess, but also in your ability to communicate effectively, think strategically, and demonstrate your potential as a leader in the field of software development. With the right preparation and mindset, you can approach your senior developer interview with confidence and increase your chances of success.
Good luck with your interview preparation, and may your next step as a senior developer be a rewarding one!