What to Do When You Feel Stuck: Mental Strategies for Breaking Through Coding Blocks
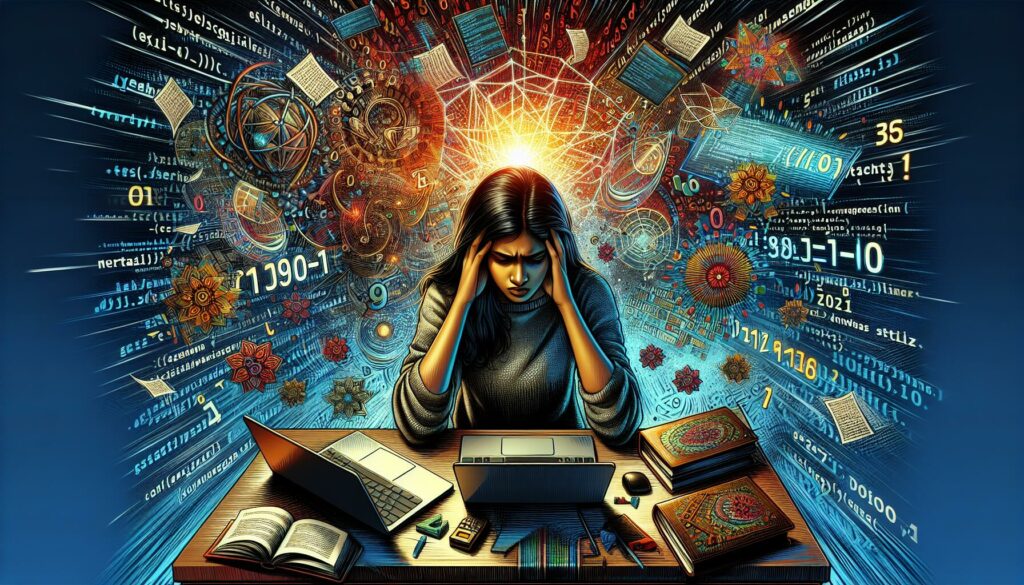
As a programmer, you’ve likely encountered moments where you feel completely stuck, staring at your screen, unable to move forward with your code. These coding blocks can be frustrating and demotivating, but they’re a normal part of the development process. In this comprehensive guide, we’ll explore various mental strategies to help you break through these barriers and get back to productive coding.
Understanding Coding Blocks
Before diving into solutions, it’s essential to understand what coding blocks are and why they occur. A coding block is a mental state where a programmer feels unable to progress in their work, often due to a complex problem, lack of inspiration, or mental fatigue. These blocks can happen to developers of all skill levels, from beginners to seasoned professionals.
Common Causes of Coding Blocks
- Overwhelm from complex problems
- Lack of clear project requirements
- Mental fatigue or burnout
- Perfectionism and fear of making mistakes
- Lack of knowledge or experience in a particular area
- Distractions and poor focus
Mental Strategies for Breaking Through Coding Blocks
1. Take a Step Back and Reassess
When you’re deeply immersed in a problem, it’s easy to lose sight of the bigger picture. Taking a step back allows you to reassess the situation with fresh eyes.
- Review the problem statement: Ensure you fully understand what you’re trying to accomplish.
- Break down the problem: Divide the larger issue into smaller, manageable tasks.
- Identify what you know and what you don’t know: This can help pinpoint areas where you might need additional research or assistance.
2. Change Your Environment
Sometimes, a change of scenery can spark creativity and help you see problems from a new perspective.
- Work from a different location, like a coffee shop or a park
- Rearrange your workspace
- Try standing or walking while thinking about the problem
3. Practice Mindfulness and Meditation
Mindfulness and meditation can help clear your mind and reduce stress, allowing you to approach problems with renewed focus.
- Try a short guided meditation focused on concentration
- Practice deep breathing exercises
- Use mindfulness apps like Headspace or Calm
4. Implement the Pomodoro Technique
The Pomodoro Technique is a time management method that can help maintain focus and prevent burnout.
- Set a timer for 25 minutes and focus solely on your task
- Take a 5-minute break when the timer goes off
- After four “pomodoros,” take a longer 15-30 minute break
5. Rubber Duck Debugging
This technique involves explaining your code or problem to an inanimate object (traditionally a rubber duck). The act of articulating the problem out loud can often lead to insights and solutions.
- Choose any object or even an imaginary person
- Explain your code line by line
- Verbalize your thought process and the problem you’re facing
6. Seek Inspiration from Others
Looking at how others have solved similar problems can provide new perspectives and ideas.
- Browse coding forums like Stack Overflow or Reddit’s programming communities
- Review open-source projects on GitHub
- Read coding blogs or watch programming tutorials
7. Use Pseudocode or Flowcharts
Sometimes, stepping away from actual code and using more abstract representations can help clarify your thinking.
- Write out your algorithm in plain language (pseudocode)
- Create a flowchart to visualize the logic flow
- Use tools like draw.io or Lucidchart for digital diagramming
8. Practice Active Problem-Solving Techniques
Engage in deliberate problem-solving exercises to sharpen your skills and approach coding challenges more effectively.
- The Five Whys: Ask “why” five times to get to the root of the problem
- IDEAL Problem Solving: Identify, Define, Explore, Act, and Look back
- Lateral Thinking: Generate creative solutions by approaching problems from unexpected angles
9. Implement Time-boxing
Set a specific time limit for working on a problem. This can help prevent overthinking and encourage decisive action.
- Decide on a reasonable time frame (e.g., 1 hour)
- Work intensively during this period
- If you haven’t solved the problem when time’s up, take a break or seek help
10. Use the Feynman Technique
Named after physicist Richard Feynman, this technique involves explaining complex concepts in simple terms to enhance understanding.
- Choose the concept or problem you’re stuck on
- Explain it as if you’re teaching it to someone with no background in programming
- Identify gaps in your explanation, which often reveal areas you don’t fully understand
- Review and simplify your explanation
Practical Coding Strategies
While mental strategies are crucial, combining them with practical coding techniques can significantly enhance your problem-solving abilities.
1. Start with a Minimal Working Example
Begin by creating the simplest possible version of your solution that works. This approach, often called a “minimum viable product” in software development, allows you to build a foundation and iterate from there.
// Example: Starting with a basic function
function add(a, b) {
return a + b;
}
// Test the basic functionality
console.log(add(2, 3)); // Output: 5
// Now you can expand on this, adding more features or error handling
function add(a, b) {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new Error('Both arguments must be numbers');
}
return a + b;
}
2. Use Console.log Liberally
When debugging, don’t hesitate to use console.log() statements to track the flow of your program and the values of variables at different points.
function complexCalculation(x, y) {
console.log('Inputs:', x, y);
let result = x * y;
console.log('After multiplication:', result);
result += Math.pow(x, 2);
console.log('After adding x squared:', result);
return result;
}
console.log(complexCalculation(3, 4));
3. Leverage Version Control
Use Git or another version control system to create checkpoints in your code. This allows you to experiment freely, knowing you can always revert to a working state.
// After making significant progress or before trying something risky
git add .
git commit -m "Implement basic functionality - all tests passing"
// If things go wrong, you can always revert
git reset --hard HEAD^
4. Write Tests First (Test-Driven Development)
Writing tests before implementing functionality can help clarify what you’re trying to achieve and provide a clear goal to work towards.
// Example using Jest testing framework
test('add function correctly adds two numbers', () => {
expect(add(2, 3)).toBe(5);
expect(add(-1, 1)).toBe(0);
expect(add(0, 0)).toBe(0);
});
// Now implement the function to pass these tests
function add(a, b) {
return a + b;
}
5. Use Code Linters and Formatters
Tools like ESLint for JavaScript or Black for Python can automatically detect potential errors and inconsistencies in your code, helping you focus on solving the core problem rather than getting caught up in syntax issues.
6. Pair Programming or Code Reviews
Collaborating with another developer, either through pair programming or code reviews, can provide new perspectives and catch issues you might have overlooked.
Leveraging AI and Tools for Problem-Solving
In today’s tech landscape, various AI-powered tools and platforms can assist in breaking through coding blocks. While these should not replace fundamental problem-solving skills, they can be valuable aids in your development process.
1. AI-Powered Code Completion
Tools like GitHub Copilot or TabNine use machine learning to suggest code completions based on context. While not always perfect, they can provide helpful starting points or alternative approaches.
2. Interactive Learning Platforms
Platforms like AlgoCademy offer interactive coding tutorials and AI-powered assistance, which can be particularly helpful when you’re stuck on algorithmic problems or preparing for technical interviews.
3. Code Analysis Tools
Static code analysis tools like SonarQube can identify potential bugs, code smells, and security vulnerabilities, helping you improve your code quality and catch issues early.
4. Online IDEs and Collaboration Platforms
Platforms like CodePen or JSFiddle allow you to quickly set up a coding environment and share it with others, making it easier to get help or collaborate on problem-solving.
Maintaining Long-Term Mental Resilience
While the strategies mentioned above can help in the moment, it’s also important to develop long-term habits that build mental resilience and prevent coding blocks from occurring frequently.
1. Continuous Learning
Regularly engage in learning activities to expand your knowledge and stay updated with new technologies and best practices.
- Follow tech blogs and newsletters
- Attend webinars or conferences
- Participate in coding challenges or hackathons
2. Build a Support Network
Cultivate relationships with other developers who can offer support, advice, and different perspectives when you’re stuck.
- Join local or online coding meetups
- Participate in online forums or communities
- Consider finding a mentor or becoming one yourself
3. Practice Self-Care
Maintain good physical and mental health to ensure you’re in the best condition to tackle coding challenges.
- Establish a regular sleep schedule
- Exercise regularly
- Practice stress-management techniques like meditation or yoga
4. Reflect on Your Successes
Keep a record of problems you’ve solved and challenges you’ve overcome. Reviewing these during difficult times can boost your confidence and provide valuable insights.
5. Embrace a Growth Mindset
View challenges and setbacks as opportunities for learning and growth rather than as failures. This perspective can help you approach problems with curiosity and resilience.
Conclusion
Coding blocks are an inevitable part of a programmer’s journey, but they don’t have to be insurmountable obstacles. By employing a combination of mental strategies, practical coding techniques, and leveraging available tools and resources, you can effectively break through these barriers and continue your growth as a developer.
Remember, the key is to stay patient, persistent, and open to different approaches. Every coding block you overcome not only solves the immediate problem but also contributes to your overall skill development and problem-solving abilities.
As you apply these strategies, you’ll likely find that what once seemed like frustrating roadblocks become valuable learning experiences, propelling you forward in your coding journey. Stay curious, keep practicing, and don’t hesitate to seek help when needed. With time and experience, you’ll develop your own unique set of strategies for tackling even the most challenging coding problems.