What to Do When You Don’t Know the Answer: Strategies for Navigating Unknown Problems
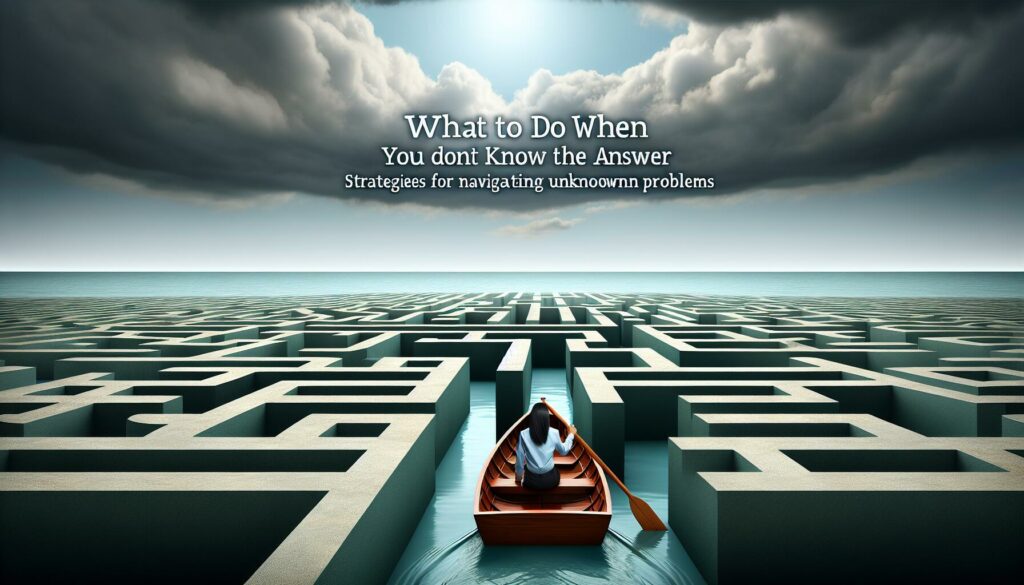
As aspiring software engineers and developers, we often find ourselves in situations where we’re faced with coding problems that seem unfamiliar or downright perplexing. This is especially true during technical interviews, where the pressure is high and the stakes even higher. But fear not! In this comprehensive guide, we’ll explore effective strategies for tackling unknown coding problems, demonstrating your problem-solving skills, and impressing your interviewers even when you’re not sure of the exact solution.
The Importance of a Methodical Approach
Before we dive into specific strategies, it’s crucial to understand why a methodical approach is so important, even when you’re unsure of the answer. Interviewers are often more interested in your problem-solving process than the final solution itself. Here’s why:
- It demonstrates your analytical thinking skills
- It shows how you break down complex problems
- It reveals your ability to communicate technical concepts
- It highlights your persistence and creativity in the face of challenges
Remember, in real-world software development, you’ll often encounter problems you haven’t seen before. Your ability to approach these challenges systematically is a valuable skill that employers are looking for.
Strategy 1: Break Down the Problem
When faced with an unfamiliar coding problem, the first step is to break it down into smaller, more manageable parts. This approach, often called “divide and conquer,” can help you gain clarity and make progress even when the overall solution isn’t immediately apparent.
Steps for Breaking Down a Problem:
- Identify the inputs and expected outputs
- List the constraints and edge cases
- Divide the problem into smaller sub-problems
- Solve each sub-problem independently
- Combine the solutions to solve the original problem
Example: Finding the Longest Palindromic Substring
Let’s say you’re asked to find the longest palindromic substring in a given string. If you’re not familiar with this problem, here’s how you might break it down:
- Input: A string of characters
- Output: The longest substring that’s a palindrome
- Sub-problems:
- How to check if a string is a palindrome
- How to generate all possible substrings
- How to keep track of the longest palindrome found
By breaking down the problem this way, you can start tackling each sub-problem individually, even if you’re not sure how to solve the entire problem at once.
Strategy 2: Use Analogies and Familiar Concepts
When you encounter a problem that seems entirely new, try to draw analogies to concepts or problems you’re already familiar with. This can help you leverage your existing knowledge and potentially find a path to the solution.
Steps for Using Analogies:
- Identify key elements of the problem
- Think of similar problems or concepts you’ve encountered before
- Draw parallels between the familiar and unfamiliar elements
- Adapt solutions from the familiar problem to the new one
Example: Graph Traversal as a Maze
Suppose you’re asked about a graph traversal algorithm you’re not familiar with. You could draw an analogy to navigating a maze:
- Nodes in the graph are like rooms in the maze
- Edges are like doorways connecting the rooms
- Visiting all nodes is like exploring every room in the maze
- Avoiding cycles is like not revisiting rooms you’ve already been to
By thinking about the problem in terms of a maze, you might be able to come up with a solution based on how you’d explore a physical maze, even if you don’t know the specific graph algorithm.
Strategy 3: Start with a Brute Force Approach
When you’re stuck, it’s often helpful to start with a brute force solution. While it may not be the most efficient, it demonstrates that you understand the problem and can think through a basic approach.
Steps for Developing a Brute Force Solution:
- Consider all possible inputs
- Outline a step-by-step process to check each possibility
- Implement the solution, even if it’s inefficient
- Analyze the time and space complexity
- Discuss potential optimizations
Example: Two Sum Problem
Let’s say you’re asked to find two numbers in an array that add up to a target sum. A brute force approach might look like this:
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
While this solution has a time complexity of O(n^2), it’s a valid starting point. You can then discuss potential optimizations, such as using a hash table to improve efficiency.
Strategy 4: Visualize the Problem
Sometimes, a visual representation can help you understand and solve a problem more easily. Don’t hesitate to use diagrams, flowcharts, or other visual aids during your interview.
Steps for Visualizing a Problem:
- Identify key components of the problem
- Choose an appropriate visual representation (e.g., tree, graph, array)
- Draw the initial state of the problem
- Illustrate how the solution would progress step-by-step
- Use the visual to explain your thought process to the interviewer
Example: Binary Search Tree Operations
If you’re asked about operations on a Binary Search Tree (BST) and you’re not entirely sure, you could start by drawing a simple BST:
8
/ \
3 10
/ \ \
1 6 14
/ \ /
4 7 13
Using this visual, you can walk through operations like insertion, deletion, or searching, even if you don’t remember the exact algorithms. This approach shows your understanding of the BST structure and properties.
Strategy 5: Think Aloud and Collaborate
One of the most important strategies when facing an unknown problem is to think aloud and engage with your interviewer. This not only demonstrates your problem-solving process but also opens up opportunities for hints and guidance.
Tips for Thinking Aloud:
- Clearly state your assumptions about the problem
- Verbalize your thought process as you consider different approaches
- Ask clarifying questions about the problem constraints or requirements
- Discuss trade-offs between different potential solutions
- Be open about areas where you’re unsure or need more information
Example: Collaborating on a Dynamic Programming Problem
Suppose you’re faced with a dynamic programming problem you’re not familiar with, like finding the longest increasing subsequence. Here’s how you might approach it:
“I’m not immediately familiar with this problem, but it sounds like it might be solved using dynamic programming. Can you confirm if that’s a valid approach? If so, I’d like to think through how we might break this down into subproblems.”
By engaging the interviewer this way, you’re showing your willingness to learn and collaborate, which are valuable traits in a software engineer.
Strategy 6: Draw from Similar Past Experiences
Even if you haven’t encountered the exact problem before, you may have solved something similar in the past. Drawing parallels to your previous experiences can help you approach the new problem.
Steps for Leveraging Past Experiences:
- Identify key characteristics of the current problem
- Recall similar problems you’ve solved before
- Analyze how the solutions to those problems might apply
- Adapt and combine relevant aspects of past solutions
- Explain your reasoning to the interviewer
Example: Adapting Array Techniques to Strings
Let’s say you’re asked about manipulating strings, but you’re more comfortable with array operations. You could approach it like this:
“While I haven’t worked extensively with string manipulation, I’ve done similar operations with arrays. In many programming languages, strings can be treated similarly to arrays of characters. So, if we were to reverse a string, we could potentially use a two-pointer technique similar to reversing an array. Would you like me to elaborate on that approach?”
Strategy 7: Start with Edge Cases and Examples
When you’re unsure about a problem, starting with edge cases and specific examples can help you understand the problem better and potentially lead you to a solution.
Steps for Using Edge Cases and Examples:
- Identify potential edge cases (empty input, very large input, etc.)
- Create small, manageable examples
- Solve these examples manually
- Look for patterns or insights from these solutions
- Use these insights to develop a general approach
Example: Finding the Median of Two Sorted Arrays
If faced with this complex problem, you might start with simple examples:
arr1 = [1, 3]
arr2 = [2]
arr1 = [1, 2]
arr2 = [3, 4]
By working through these examples, you might notice patterns about how the median relates to the middle elements of the merged arrays, leading you towards a more general solution.
Strategy 8: Propose Multiple Approaches
Even if you’re not sure of the optimal solution, proposing multiple approaches shows your ability to think critically about the problem from different angles.
Steps for Proposing Multiple Approaches:
- Brainstorm different ways to tackle the problem
- Outline the basic steps for each approach
- Discuss the pros and cons of each method
- Analyze the time and space complexity if possible
- Ask the interviewer for feedback on which approach to pursue
Example: Finding Duplicate Numbers in an Array
For this problem, you might propose several approaches:
- Sorting the array first (O(n log n) time, O(1) space)
- Using a hash set (O(n) time, O(n) space)
- Using the array itself to mark visited numbers (O(n) time, O(1) space, but modifies the input)
- Using a bit vector for space efficiency (O(n) time, O(n/32) space)
By presenting multiple options, you demonstrate your ability to consider trade-offs and adapt to different constraints.
Strategy 9: Implement a Partial Solution
If you can’t solve the entire problem, implementing a partial solution or solving a simplified version of the problem can still showcase your coding skills and problem-solving approach.
Steps for Implementing a Partial Solution:
- Identify a subset of the problem you can solve
- Clearly communicate which part you’re addressing
- Implement the solution for that part
- Explain how you would extend this to the full problem
- Discuss any challenges or limitations of your approach
Example: Implementing a Basic Calculator
If asked to implement a calculator that handles complex expressions with parentheses and you’re not sure how to handle that, you might start with a simpler version:
def basic_calculator(expression):
tokens = expression.split()
result = int(tokens[0])
for i in range(1, len(tokens), 2):
operator = tokens[i]
operand = int(tokens[i+1])
if operator == '+':
result += operand
elif operator == '-':
result -= operand
return result
# Example usage
print(basic_calculator("5 + 3 - 2")) # Output: 6
You can then explain how you would extend this to handle multiplication, division, and eventually parentheses using a stack-based approach.
Strategy 10: Learn from the Experience
Regardless of whether you solve the problem completely, every interview is a learning opportunity. Here’s how to make the most of it:
Steps for Learning from the Experience:
- Ask for feedback on your approach
- Request an explanation of the optimal solution if you didn’t reach it
- Take notes after the interview about what you learned
- Research the problem and related concepts afterwards
- Practice similar problems to reinforce your learning
Example: Following Up on a Graph Problem
If you struggled with a graph traversal problem, you might say:
“Thank you for walking me through this problem. I realize now that I need to strengthen my understanding of graph algorithms. Could you recommend any resources or specific areas I should focus on to improve in this area?”
This shows your eagerness to learn and improve, which is a valuable trait in any software engineer.
Conclusion
Facing unknown problems in coding interviews can be daunting, but with these strategies, you can approach them with confidence and demonstrate your problem-solving skills effectively. Remember, the goal is not just to find the right answer, but to show your thought process, your ability to break down complex problems, and your capacity to learn and adapt on the fly.
By mastering these techniques, you’ll be better prepared to handle whatever challenges come your way, not just in interviews, but in your future career as a software engineer. Keep practicing, stay curious, and never stop learning!
At AlgoCademy, we’re committed to helping you develop these crucial problem-solving skills. Our interactive coding tutorials, AI-powered assistance, and comprehensive resources are designed to take you from a coding beginner to a confident interviewee ready to tackle even the most challenging problems at top tech companies.
Remember, every great software engineer started where you are now. With persistence, practice, and the right strategies, you’ll be well on your way to acing those technical interviews and launching your dream career in software development. Happy coding!