What Learning to Code Can Teach You About Resilience and Grit
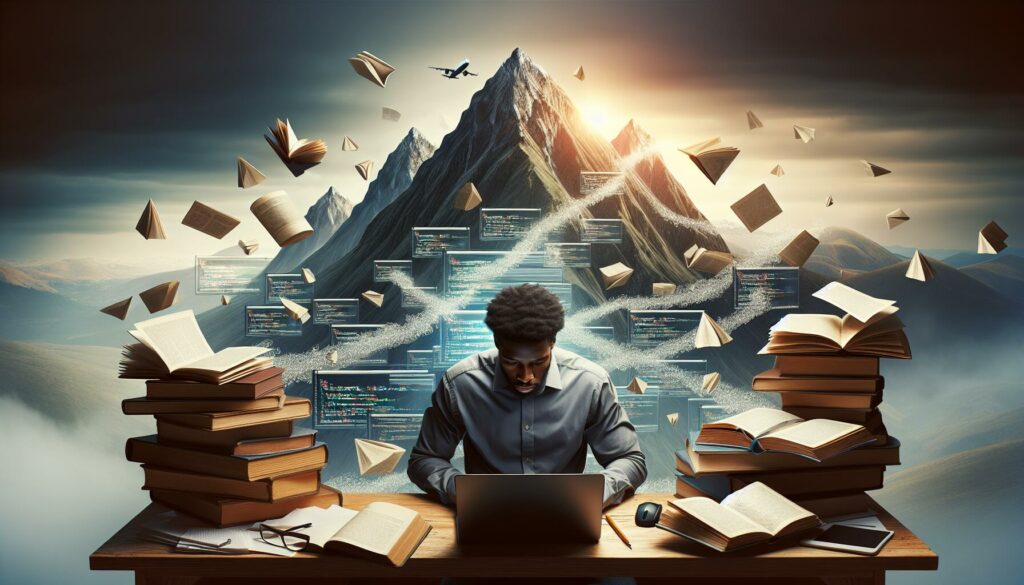
In today’s rapidly evolving digital landscape, learning to code has become more than just a valuable skill for career advancement. It’s a journey that can profoundly impact your personal growth, teaching you invaluable lessons about resilience and grit. As we explore this topic, we’ll delve into how the process of learning to code mirrors life’s challenges and how it can shape your mindset to overcome obstacles in all areas of your life.
The Coding Journey: More Than Just Syntax
When most people think about learning to code, they often focus on the technical aspects: memorizing syntax, understanding algorithms, and mastering programming languages. However, the journey of becoming a proficient coder involves much more than just technical knowledge. It’s a path that tests your patience, challenges your problem-solving abilities, and pushes you to persevere in the face of seemingly insurmountable obstacles.
Platforms like AlgoCademy recognize this multifaceted nature of coding education. They don’t just provide syntax tutorials; they offer a comprehensive learning experience that nurtures both technical skills and personal growth. Through interactive coding challenges, AI-powered assistance, and a focus on algorithmic thinking, these platforms create an environment where learners can develop resilience and grit alongside their coding abilities.
Embracing Failure: The First Step Towards Resilience
One of the most crucial lessons that coding teaches is the importance of embracing failure. In the world of programming, errors and bugs are not just common; they’re an integral part of the learning process. Every programmer, from novices to experts, encounters errors regularly. The key difference lies in how they respond to these setbacks.
When you’re learning to code, you’ll quickly realize that your programs won’t work perfectly on the first try. You’ll encounter syntax errors, logical flaws, and unexpected outputs. Initially, these failures can be frustrating and disheartening. However, as you progress in your coding journey, you’ll start to see these errors not as failures, but as opportunities for growth.
This shift in perspective is a cornerstone of resilience. By reframing errors as learning experiences rather than personal shortcomings, you develop a growth mindset. This mindset doesn’t just apply to coding; it can transform how you approach challenges in all aspects of your life.
The Debugger’s Mindset
Learning to debug code is an excellent exercise in developing resilience. When faced with a bug, programmers don’t give up; they investigate. They analyze the problem, form hypotheses, and systematically test solutions. This process of methodical problem-solving is a valuable life skill that extends far beyond the realm of coding.
Consider this simple Python code snippet that demonstrates a common debugging scenario:
def calculate_average(numbers):
total = 0
for num in numbers:
total += num
return total / len(numbers)
# Test the function
test_numbers = [1, 2, 3, 4, 5]
result = calculate_average(test_numbers)
print(f"The average is: {result}")
# What if we pass an empty list?
empty_list = []
result = calculate_average(empty_list)
print(f"The average of an empty list is: {result}")
This code works fine for non-empty lists, but what happens when we pass an empty list? It raises a ZeroDivisionError. A resilient programmer doesn’t see this as a failure, but as an opportunity to improve the code’s robustness:
def calculate_average(numbers):
if not numbers:
return 0 # Return 0 for empty lists
total = sum(numbers)
return total / len(numbers)
# Now test with both non-empty and empty lists
test_numbers = [1, 2, 3, 4, 5]
result = calculate_average(test_numbers)
print(f"The average is: {result}")
empty_list = []
result = calculate_average(empty_list)
print(f"The average of an empty list is: {result}")
This process of identifying problems, thinking through solutions, and implementing fixes is a microcosm of how resilience works in the broader context of life’s challenges.
The Power of Persistence: Grit in Action
Grit, as defined by psychologist Angela Duckworth, is the combination of passion and perseverance for long-term goals. In the context of coding, grit is what keeps you going when you’re stuck on a particularly challenging problem or when you’re trying to master a complex concept.
Learning to code is rarely a linear process. You’ll encounter concepts that seem incomprehensible at first, only to have “aha!” moments days or weeks later. This non-linear progress can be frustrating, but it’s also where the true value of grit becomes apparent.
The Long Game of Skill Acquisition
Consider the journey of learning data structures and algorithms, a crucial aspect of coding education emphasized by platforms like AlgoCademy. Understanding complex data structures like balanced binary search trees or mastering advanced algorithms like dynamic programming doesn’t happen overnight. It requires consistent effort, repeated practice, and a willingness to push through periods of confusion and self-doubt.
Here’s a simple example of a binary search tree implementation in Python:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
elif value > node.value:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
Understanding and implementing such data structures requires grit. You need to persist through the initial confusion, practice implementing and using these structures, and gradually build your understanding over time.
The Compounding Effect of Consistent Effort
One of the most valuable lessons that coding teaches about grit is the power of consistent, incremental progress. In coding, as in life, major breakthroughs often come not from sporadic bursts of effort, but from steady, persistent work over time.
This principle is exemplified in the way coding skills develop. You might spend days struggling with a concept, making what feels like minimal progress. But each small step forward, each minor victory in understanding, compounds over time. Suddenly, you find yourself tackling problems that would have seemed impossible weeks or months ago.
This compounding effect of grit is beautifully illustrated in the realm of algorithmic problem-solving. Consider the journey of preparing for technical interviews, a focus area for many coding education platforms. Solving complex algorithmic problems requires not just knowledge, but the ability to apply that knowledge creatively under pressure.
Here’s an example of a classic algorithmic problem – finding the longest palindromic substring:
def longest_palindrome_substring(s):
if not s:
return ""
start = 0
max_length = 1
for i in range(1, len(s)):
# Even length palindrome
left, right = i - 1, i
while left >= 0 and right < len(s) and s[left] == s[right]:
if right - left + 1 > max_length:
start = left
max_length = right - left + 1
left -= 1
right += 1
# Odd length palindrome
left, right = i - 1, i + 1
while left >= 0 and right < len(s) and s[left] == s[right]:
if right - left + 1 > max_length:
start = left
max_length = right - left + 1
left -= 1
right += 1
return s[start:start + max_length]
# Test the function
test_string = "babad"
result = longest_palindrome_substring(test_string)
print(f"The longest palindrome substring in '{test_string}' is: {result}")
Mastering such algorithms doesn’t happen overnight. It requires repeated practice, analyzing multiple approaches, and the grit to keep pushing forward even when solutions don’t come easily. This process of gradual mastery through persistent effort is a powerful lesson in the value of grit, applicable far beyond the realm of coding.
Problem-Solving: The Core of Coding and Life
At its heart, coding is about problem-solving. Every program you write is a solution to a problem, whether it’s as simple as calculating an average or as complex as building a machine learning model. This focus on problem-solving is perhaps the most transferable skill that coding imparts.
Learning to code teaches you to approach problems systematically:
- Understand the problem: Before you can solve a problem, you need to fully understand what it is. In coding, this often involves carefully reading the problem statement, identifying inputs and expected outputs, and clarifying any ambiguities.
- Break it down: Complex problems become manageable when broken down into smaller, more manageable sub-problems. This skill of decomposition is crucial in both coding and life.
- Plan your approach: Before diving into coding, experienced programmers often plan their approach. This might involve sketching out the algorithm, considering different data structures, or outlining the main functions needed.
- Implement and test: With a plan in place, you can start implementing your solution. In coding, this means writing the actual code. But the process doesn’t end there – testing and debugging are crucial steps.
- Refine and optimize: Once you have a working solution, there’s often room for improvement. This might involve optimizing for efficiency, improving readability, or adding features.
This structured approach to problem-solving is a valuable life skill. Whether you’re facing a career challenge, a personal dilemma, or a complex project at work, the problem-solving mindset developed through coding can help you navigate the situation more effectively.
Algorithmic Thinking in Daily Life
The concept of algorithmic thinking, which is central to coding education, has applications far beyond writing computer programs. It’s about developing a systematic, step-by-step approach to solving problems and making decisions.
Consider how you might apply algorithmic thinking to a common life situation, like planning a vacation:
def plan_vacation(budget, duration, preferences):
destinations = research_destinations(preferences)
viable_options = filter_by_budget_and_duration(destinations, budget, duration)
if not viable_options:
return "No suitable destinations found. Consider adjusting your criteria."
best_option = choose_best_option(viable_options)
itinerary = create_itinerary(best_option, duration)
bookings = make_bookings(itinerary)
return bookings, itinerary
def research_destinations(preferences):
# Logic to search and return destinations based on preferences
pass
def filter_by_budget_and_duration(destinations, budget, duration):
# Logic to filter destinations based on budget and trip duration
pass
def choose_best_option(options):
# Logic to select the best option, possibly involving user input
pass
def create_itinerary(destination, duration):
# Logic to create a day-by-day itinerary
pass
def make_bookings(itinerary):
# Logic to book flights, accommodations, activities, etc.
pass
# Usage
budget = 2000
duration = 7 # days
preferences = ["beach", "culture", "food"]
bookings, itinerary = plan_vacation(budget, duration, preferences)
While this is a simplified representation, it demonstrates how the structured thinking developed through coding can be applied to organize and tackle real-life tasks. This ability to break down complex problems, consider different factors, and proceed systematically is a powerful tool for building resilience and demonstrating grit in various life situations.
The Growth Mindset: A Coder’s Greatest Asset
Perhaps the most valuable lesson that learning to code imparts is the cultivation of a growth mindset. Coined by psychologist Carol Dweck, a growth mindset is the belief that abilities can be developed through dedication and hard work. This is in contrast to a fixed mindset, which sees abilities as static and unchangeable.
In the world of coding, a growth mindset is not just beneficial – it’s essential. Technology evolves rapidly, new programming languages emerge, and best practices change. To succeed as a programmer, you need to embrace continuous learning and see challenges as opportunities for growth.
Embracing the Unknown
Coding often involves working with unfamiliar technologies or tackling problems you’ve never encountered before. This constant exposure to the unknown helps develop a comfort with uncertainty – a crucial component of resilience.
Consider the experience of learning a new programming language or framework. At first, the syntax might seem alien, the concepts confusing. But as you persist, things start to click. This experience of pushing through initial discomfort to achieve understanding is a powerful builder of resilience.
Here’s an example of how the same concept (a simple function to greet a user) might be implemented in three different languages:
# Python
def greet(name):
return f"Hello, {name}!"
# JavaScript
function greet(name) {
return `Hello, ${name}!`;
}
// Java
public class Greeter {
public static String greet(String name) {
return "Hello, " + name + "!";
}
}
Learning to navigate these differences, to see the underlying patterns, and to adapt your thinking to different paradigms is an exercise in cognitive flexibility – a key component of resilience.
The Joy of Problem-Solving
One of the most rewarding aspects of coding is the thrill of solving a challenging problem. This experience – the frustration of being stuck, the persistence required to keep trying different approaches, and the eventual elation of finding a solution – is a microcosm of how grit operates in the face of life’s challenges.
Platforms like AlgoCademy tap into this aspect of coding education by providing a structured approach to problem-solving. They offer a progression of challenges that grow in complexity, allowing learners to experience this cycle of struggle and success repeatedly. This repeated exposure builds not just coding skills, but also the resilience to tackle increasingly difficult problems.
Building a Support System: The Power of Community
While much of coding involves individual effort, the importance of community cannot be overstated. The coding community, both online and offline, is known for its collaborative nature and willingness to help others. Engaging with this community teaches valuable lessons about seeking help, offering support, and the power of collective knowledge.
Participating in coding forums, contributing to open-source projects, or simply pair programming with a friend all help build a support system. This network becomes a source of resilience, providing encouragement during tough times and celebrating successes together.
The Art of Asking for Help
Learning to ask for help effectively is a crucial skill in coding and in life. It involves clearly articulating your problem, showing what you’ve tried so far, and being open to guidance. This process of reaching out for support when needed is a key aspect of resilience.
Here’s an example of how you might structure a question when seeking help on a coding forum:
Title: TypeError when trying to concatenate string and integer in Python
I'm trying to create a function that takes a person's name and age and returns a greeting. However, I'm getting a TypeError. Here's my code:
def greet_with_age(name, age):
return "Hello, " + name + "! You are " + age + " years old."
print(greet_with_age("Alice", 30))
The error message I'm getting is:
TypeError: can only concatenate str (not "int") to str
I've tried searching for solutions involving string concatenation, but I'm still confused. Any help would be appreciated!
This structured approach to asking for help – clearly stating the problem, showing your code, providing the error message, and indicating what you’ve already tried – is not only more likely to get you the help you need but also demonstrates a proactive approach to problem-solving.
Celebrating Small Wins: The Fuel for Perseverance
In the journey of learning to code, it’s crucial to celebrate small wins. Every bug fixed, every concept understood, and every program successfully run is a victory. These small successes are the fuel that keeps you going through the challenging times.
Recognizing and celebrating progress, no matter how small, is a powerful tool for building resilience and maintaining grit. It helps maintain motivation and provides tangible evidence of growth, reinforcing the belief that continued effort will lead to further improvement.
Tracking Progress
Many coders find it helpful to keep a learning journal or use project management tools to track their progress. This could be as simple as a list of completed tutorials or as complex as a GitHub repository showcasing your projects. The key is to have a tangible record of your growth.
Here’s an example of how you might structure a simple learning log:
Learning Log
Week 1:
- Completed Python basics tutorial
- Wrote first "Hello, World!" program
- Learned about variables and data types
Week 2:
- Studied control structures (if/else, loops)
- Completed 5 coding challenges on conditionals
- Started learning about functions
Week 3:
- Deepened understanding of functions
- Introduced to list comprehensions
- Built a simple calculator program
Goals for Next Week:
- Start learning about object-oriented programming
- Complete project: Build a text-based adventure game
This kind of structured logging not only helps you see your progress but also aids in setting achievable goals – another crucial aspect of maintaining motivation and building grit.
Applying Coding Resilience to Life Challenges
The resilience and grit developed through coding can be applied to various life challenges. Whether you’re facing a career setback, working towards a personal goal, or navigating a difficult relationship, the mindset cultivated through coding can be invaluable.
Breaking Down Big Goals
Just as you break down a complex coding problem into smaller, manageable tasks, you can apply the same approach to life goals. For instance, if your goal is to switch careers, you might break it down like this:
Career Change Plan:
1. Research:
- Identify target industry
- Research required skills and qualifications
- Find potential job roles
2. Skill Development:
- List skills to acquire
- Find resources (courses, books, mentors)
- Create a study schedule
3. Networking:
- Join professional associations
- Attend industry events
- Connect with professionals on LinkedIn
4. Experience Building:
- Look for internship opportunities
- Work on personal projects
- Volunteer in relevant roles
5. Job Search:
- Update resume and online profiles
- Prepare for interviews
- Apply for positions
6. Continuous Learning:
- Stay updated with industry trends
- Seek feedback and adapt plan as needed
This structured approach, inspired by the way coders tackle complex projects, can make even the most daunting life changes feel more manageable.
Embracing Iteration in Personal Growth
In coding, it’s common to start with a basic version of a program and then iteratively improve it. This concept of continuous improvement can be powerfully applied to personal development. Instead of aiming for perfection from the start, focus on making small, consistent improvements over time.
Conclusion: Code Your Way to Resilience
Learning to code is about much more than mastering a technical skill. It’s a journey that builds character, develops resilience, and cultivates grit. The problem-solving mindset, the ability to learn from failures, the persistence required to debug complex issues, and the joy of creating something from nothing – all these experiences contribute to personal growth that extends far beyond the realm of programming.
As you embark on or continue your coding journey, remember that every challenge you face is an opportunity to build your resilience. Every bug you fix, every concept you master, and every project you complete is not just a step towards becoming a better programmer, but also towards becoming a more resilient, gritty individual.
Platforms like AlgoCademy recognize this holistic nature of coding education. By providing structured learning paths, interactive challenges, and a supportive community, they create an environment where technical skills and personal growth go hand in hand. Whether you’re a beginner taking your first steps into the world of coding or an experienced developer preparing for technical interviews, remember that you’re not just learning to code – you’re coding your way to a more resilient, gritty you.
So, the next time you face a challenging bug or a complex algorithm, embrace it. See it not just as a coding problem, but as an opportunity to strengthen your resilience and demonstrate your grit. In doing so, you’ll not only become a better coder but also better equipped to handle whatever challenges life throws your way.