What It Means to Think Like a Programmer
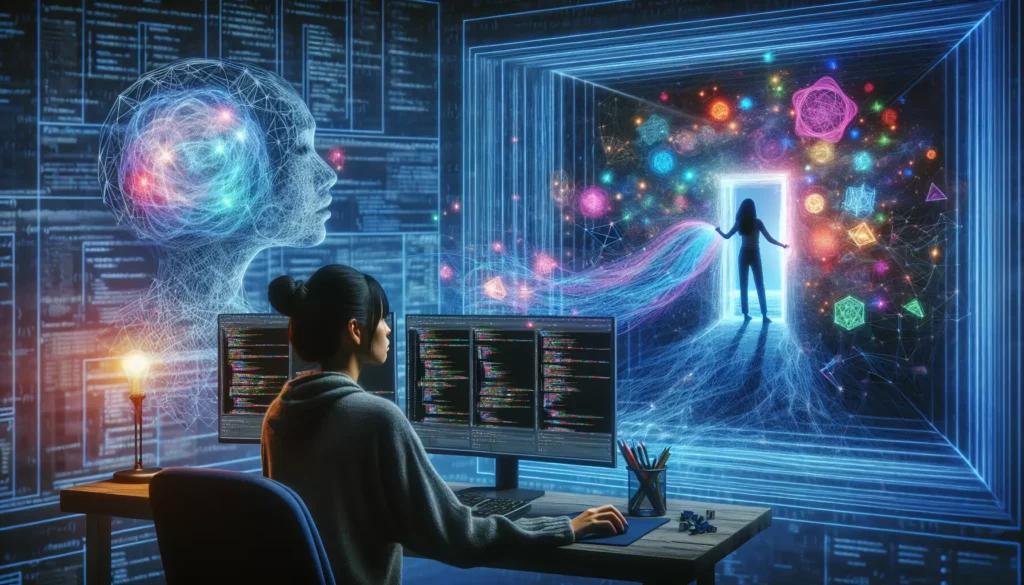
In the ever-evolving world of technology, the ability to think like a programmer has become an increasingly valuable skill. Whether you’re aspiring to join the ranks of software developers at major tech companies or simply looking to enhance your problem-solving abilities, understanding the programmer’s mindset is crucial. This article will delve into the essence of thinking like a programmer, exploring the key concepts, strategies, and habits that define this unique approach to problem-solving.
The Essence of Programmer Thinking
At its core, thinking like a programmer involves a systematic and logical approach to problem-solving. It’s not just about writing code; it’s about developing a mindset that allows you to break down complex problems into manageable components, identify patterns, and create efficient solutions. This way of thinking extends beyond the realm of coding and can be applied to various aspects of life and work.
Key Characteristics of Programmer Thinking
- Analytical mindset
- Attention to detail
- Persistence in problem-solving
- Ability to think abstractly
- Creativity in finding solutions
- Continuous learning and adaptability
Breaking Down Problems
One of the fundamental skills in programmer thinking is the ability to break down complex problems into smaller, more manageable parts. This process, often referred to as decomposition, allows programmers to tackle intricate challenges step by step.
Steps in Problem Decomposition:
- Identify the main problem
- Break it down into smaller sub-problems
- Analyze each sub-problem individually
- Determine the relationships between sub-problems
- Develop solutions for each sub-problem
- Integrate the solutions to solve the main problem
By mastering this approach, you can tackle even the most daunting programming challenges with confidence and clarity.
Algorithmic Thinking
Algorithmic thinking is a cornerstone of programmer thinking. It involves developing step-by-step procedures to solve problems efficiently. This approach is not limited to computer science; it can be applied to various real-world scenarios.
Key Components of Algorithmic Thinking:
- Problem definition: Clearly stating what needs to be solved
- Algorithm design: Creating a series of steps to solve the problem
- Efficiency consideration: Optimizing the solution for speed and resource usage
- Implementation: Translating the algorithm into code
- Testing and refinement: Verifying the solution and improving it
Platforms like AlgoCademy focus on developing these skills through interactive coding tutorials and challenges, helping learners progress from basic concepts to advanced algorithmic problem-solving.
Pattern Recognition
Recognizing patterns is a crucial skill in programming. It allows developers to identify similarities in problems and apply proven solutions or adapt existing code to new situations. This ability not only saves time but also leads to more elegant and efficient solutions.
Benefits of Pattern Recognition in Programming:
- Faster problem-solving
- Code reusability
- Improved system design
- Enhanced debugging skills
- Better understanding of complex systems
As you gain experience, you’ll start to recognize common patterns in programming problems, allowing you to apply tried-and-tested solutions more readily.
Logical Reasoning
Logical reasoning is the foundation of programming. It involves using facts, patterns, and logic to draw conclusions and make decisions. In programming, this skill is essential for everything from writing conditional statements to designing complex algorithms.
Types of Logical Reasoning in Programming:
- Deductive reasoning: Drawing specific conclusions from general principles
- Inductive reasoning: Inferring general principles from specific observations
- Abductive reasoning: Forming the best explanation for an incomplete set of observations
Developing strong logical reasoning skills will help you write more robust and error-free code, as well as debug issues more effectively.
Abstraction and Generalization
Abstraction is the process of simplifying complex systems by focusing on essential features while ignoring unnecessary details. In programming, abstraction allows developers to create more manageable and reusable code.
Levels of Abstraction in Programming:
- Problem abstraction: Identifying the core problem to be solved
- Data abstraction: Representing data in simplified forms
- Procedural abstraction: Creating reusable functions and procedures
- Object-oriented abstraction: Encapsulating data and behavior into objects
Generalization, on the other hand, involves creating solutions that can be applied to a wide range of similar problems. This skill is crucial for developing flexible and scalable software systems.
Debugging and Troubleshooting
The ability to effectively debug code and troubleshoot issues is a hallmark of experienced programmers. This skill involves not only finding and fixing errors but also understanding why they occur and how to prevent them in the future.
Effective Debugging Strategies:
- Reproduce the error: Consistently recreate the problem
- Isolate the issue: Narrow down the source of the problem
- Use debugging tools: Leverage IDEs and debuggers
- Read error messages carefully: Understand what the errors are telling you
- Check recent changes: Review recent code modifications
- Use print statements or logging: Track program flow and variable values
- Take breaks: Step away to gain a fresh perspective
Developing strong debugging skills will save you countless hours of frustration and make you a more efficient programmer.
Continuous Learning and Adaptability
The field of programming is constantly evolving, with new languages, frameworks, and technologies emerging regularly. Thinking like a programmer means embracing a mindset of continuous learning and adaptability.
Ways to Stay Current in Programming:
- Follow industry blogs and news sites
- Participate in online coding communities
- Attend workshops and conferences
- Experiment with new technologies
- Contribute to open-source projects
- Take online courses and tutorials
Platforms like AlgoCademy provide resources and tools to help programmers stay up-to-date with the latest trends and prepare for technical interviews at major tech companies.
Problem-Solving Techniques
Effective problem-solving is at the heart of programming. Developing a systematic approach to tackling challenges will greatly enhance your ability to think like a programmer.
Common Problem-Solving Techniques in Programming:
- Understand the problem: Clearly define what needs to be solved
- Plan the solution: Outline the steps needed to solve the problem
- Divide and conquer: Break the problem into smaller, manageable parts
- Start with what you know: Begin with familiar concepts and build from there
- Use pseudocode: Write out the logic in plain language before coding
- Test and iterate: Continuously test your solution and refine it
- Seek feedback: Collaborate with others and learn from their perspectives
By applying these techniques consistently, you’ll develop a more structured and effective approach to problem-solving in programming.
Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for thinking like a programmer. These fundamental concepts form the building blocks of efficient and scalable software solutions.
Key Data Structures:
- Arrays
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
Essential Algorithms:
- Sorting algorithms (e.g., Quick Sort, Merge Sort)
- Searching algorithms (e.g., Binary Search)
- Graph algorithms (e.g., Breadth-First Search, Depth-First Search)
- Dynamic Programming
- Greedy Algorithms
Mastering these concepts will enable you to choose the most appropriate tools for solving specific problems and optimize your code for performance.
Code Organization and Design Patterns
Thinking like a programmer also involves considering the overall structure and organization of your code. This includes understanding design patterns, which are reusable solutions to common programming problems.
Principles of Good Code Organization:
- Modularity: Breaking code into logical, reusable components
- Readability: Writing clear, self-documenting code
- Maintainability: Structuring code for easy updates and modifications
- Scalability: Designing systems that can grow and adapt
Common Design Patterns:
- Singleton Pattern
- Factory Pattern
- Observer Pattern
- Strategy Pattern
- Model-View-Controller (MVC) Pattern
By incorporating these principles and patterns into your thinking, you’ll create more robust and flexible software solutions.
Analytical and Critical Thinking
Analytical and critical thinking skills are essential components of the programmer’s mindset. These skills allow you to evaluate problems from multiple angles, consider alternative solutions, and make informed decisions.
Developing Analytical and Critical Thinking:
- Question assumptions: Don’t take things at face value
- Evaluate evidence: Base decisions on solid data and reasoning
- Consider multiple perspectives: Look at problems from different angles
- Analyze trade-offs: Weigh the pros and cons of different approaches
- Practice root cause analysis: Identify the underlying issues in problems
Strengthening these skills will enhance your ability to develop innovative solutions and make well-informed decisions in your programming projects.
Creativity and Innovation
While programming is often associated with logic and structure, creativity plays a crucial role in thinking like a programmer. The ability to come up with innovative solutions and think outside the box is what separates great programmers from good ones.
Fostering Creativity in Programming:
- Explore different approaches: Don’t always settle for the first solution
- Combine ideas: Merge concepts from different domains
- Challenge conventions: Question established methods and look for improvements
- Embrace constraints: Use limitations as catalysts for creative solutions
- Collaborate and brainstorm: Share ideas with others to spark innovation
By cultivating your creative thinking skills, you’ll be better equipped to tackle unique challenges and develop groundbreaking solutions in your programming career.
Time and Resource Management
Effective programmers are adept at managing their time and resources efficiently. This skill is crucial for completing projects on schedule and within budget constraints.
Time and Resource Management Strategies:
- Prioritize tasks: Focus on high-impact activities first
- Estimate accurately: Develop skills in predicting task duration
- Use time-boxing: Allocate fixed time periods for specific tasks
- Minimize distractions: Create a focused work environment
- Leverage tools: Utilize project management and productivity software
- Practice regular code reviews: Catch issues early to save time later
Mastering these strategies will help you become a more efficient and productive programmer, capable of delivering high-quality work consistently.
Collaboration and Communication
In today’s interconnected world, the ability to collaborate effectively and communicate clearly is an essential aspect of thinking like a programmer. Most software projects involve teamwork, and being able to express your ideas and understand others is crucial for success.
Key Collaboration and Communication Skills:
- Clear documentation: Write comprehensive and understandable documentation
- Code commenting: Provide clear and concise comments in your code
- Active listening: Pay attention to and understand your colleagues’ ideas
- Constructive feedback: Offer and receive feedback in a positive manner
- Version control: Use tools like Git to manage collaborative coding efforts
- Presentation skills: Clearly explain technical concepts to both technical and non-technical audiences
Developing these skills will not only make you a better team player but also enhance your ability to learn from others and share your knowledge effectively.
Ethical Considerations
Thinking like a programmer also involves considering the ethical implications of your work. As technology becomes increasingly integrated into our lives, programmers have a responsibility to consider the potential impacts of their creations.
Ethical Considerations in Programming:
- Privacy and data protection
- Algorithmic bias and fairness
- Cybersecurity and system vulnerabilities
- Environmental impact of software systems
- Accessibility and inclusive design
- Transparency in AI and machine learning models
By incorporating ethical considerations into your thinking process, you’ll contribute to the development of more responsible and beneficial technology.
Conclusion
Thinking like a programmer is a multifaceted skill that goes far beyond mere coding ability. It encompasses a wide range of cognitive skills, problem-solving techniques, and professional attributes that enable individuals to tackle complex challenges effectively. By developing these skills and adopting this mindset, you’ll be well-equipped to excel in the field of programming and contribute meaningfully to the world of technology.
Remember that becoming proficient in programmer thinking is a journey that requires continuous practice and learning. Platforms like AlgoCademy offer valuable resources and tools to help you develop these skills, from interactive coding tutorials to preparation for technical interviews at major tech companies. Embrace the challenges, stay curious, and never stop learning – these are the hallmarks of truly thinking like a programmer.
As you continue to develop your programming skills, keep in mind that the ability to think like a programmer extends beyond the realm of coding. It’s a valuable asset in many aspects of life, enabling you to approach problems systematically, think critically, and develop innovative solutions. Whether you’re aiming for a career in software development or simply looking to enhance your problem-solving abilities, cultivating the programmer’s mindset will serve you well in your personal and professional endeavors.