What is the Typical Format of a Coding Interview?
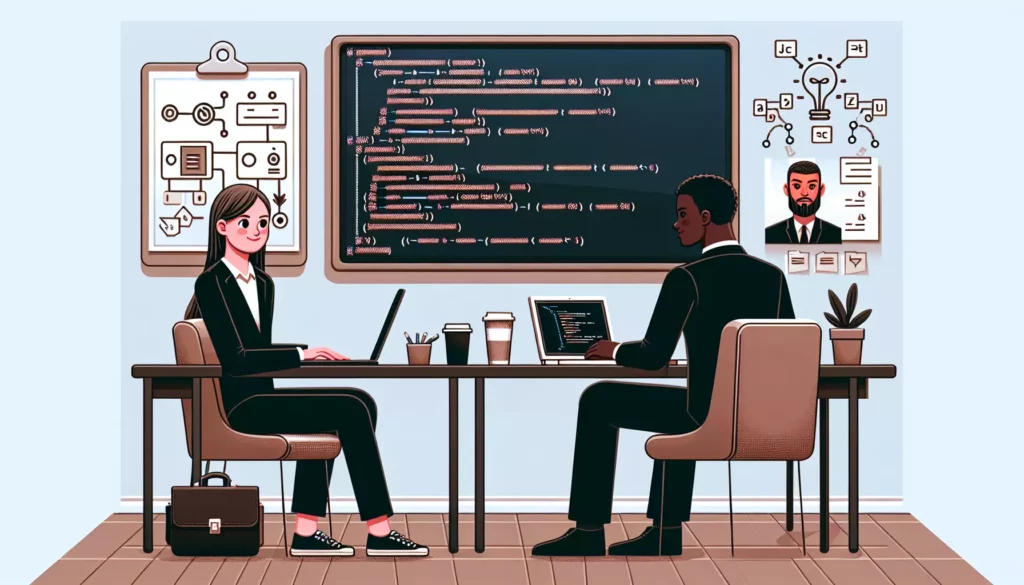
Coding interviews are a crucial part of the hiring process for software engineering positions, especially at major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google) or MANGA (Microsoft, Amazon, Netflix, Google, Apple). These interviews are designed to assess a candidate’s technical skills, problem-solving abilities, and coding proficiency. Understanding the typical format of a coding interview can help you prepare effectively and increase your chances of success. In this comprehensive guide, we’ll explore the various components of a coding interview and provide insights on how to excel in each stage.
1. Introduction and Icebreaker
Most coding interviews begin with a brief introduction and an icebreaker conversation. This phase usually lasts about 5-10 minutes and serves several purposes:
- Helping the candidate feel more comfortable
- Allowing the interviewer to assess communication skills
- Setting the tone for the rest of the interview
During this time, the interviewer might ask about your background, experience, and interest in the company. It’s an opportunity for you to make a good first impression and establish rapport with the interviewer.
2. Technical Questions
After the introduction, the interviewer will typically move on to technical questions. These questions are designed to assess your knowledge of computer science fundamentals, programming concepts, and specific technologies relevant to the position. Some common topics include:
- Data structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- Object-oriented programming principles
- Database concepts and SQL
- System design and architecture
- Programming language-specific questions (e.g., Java, Python, JavaScript)
The technical questions phase usually lasts about 10-15 minutes and serves as a warm-up for the main coding challenge.
3. Coding Challenge
The core of most coding interviews is the coding challenge. This is where you’ll be asked to solve one or more programming problems in real-time. The format of the coding challenge can vary depending on the company and the specific role, but it generally falls into one of these categories:
a. Whiteboard Coding
In traditional in-person interviews, you might be asked to write code on a whiteboard. This format tests not only your coding skills but also your ability to explain your thought process and communicate effectively.
b. Online Coding Platforms
Many companies, especially for initial screenings or remote interviews, use online coding platforms like HackerRank, LeetCode, or CoderPad. These platforms allow you to write, run, and test your code in a browser-based environment.
c. Pair Programming
Some interviews involve pair programming, where you collaborate with the interviewer to solve a problem. This format assesses your teamwork and communication skills in addition to your coding abilities.
d. Take-home Assignments
While less common for on-site interviews, some companies may give you a take-home coding assignment to complete within a specified timeframe. This allows for a more realistic assessment of your coding skills in a less pressured environment.
Regardless of the specific format, the coding challenge typically follows this structure:
- Problem Statement: The interviewer presents a problem or task for you to solve.
- Clarification: You ask questions to clarify any ambiguities in the problem statement.
- Problem-solving: You discuss your approach and potential solutions with the interviewer.
- Coding: You implement your solution in the chosen programming language.
- Testing and Debugging: You test your code with sample inputs and fix any bugs.
- Optimization: You discuss and implement potential optimizations to improve your solution.
The coding challenge typically takes 30-45 minutes, depending on the complexity of the problem and the interview format.
4. Code Review and Discussion
After you’ve completed the coding challenge, the interviewer will often review your code with you. This phase serves several purposes:
- Assessing your ability to explain your code and reasoning
- Evaluating your knowledge of best practices and coding standards
- Discussing potential improvements or alternative approaches
- Testing your ability to receive and incorporate feedback
During this phase, be prepared to justify your design choices, explain your problem-solving approach, and discuss the time and space complexity of your solution. The code review typically lasts about 10-15 minutes.
5. System Design (for more senior roles)
For senior or more experienced positions, many companies include a system design component in their coding interviews. This section focuses on assessing your ability to design and architect large-scale systems. Common topics include:
- Scalability and performance optimization
- Distributed systems
- Database design and data modeling
- API design
- Caching strategies
- Load balancing and fault tolerance
In a system design interview, you might be asked to design a high-level architecture for a complex system, such as a social media platform, a ride-sharing application, or a content delivery network. This phase typically lasts 30-45 minutes and involves a lot of discussion and diagramming.
6. Behavioral Questions
While the focus of a coding interview is primarily technical, many interviewers also include behavioral questions to assess your soft skills and cultural fit. These questions might cover topics such as:
- Your past experiences and projects
- How you handle conflicts or challenges in a team
- Your approach to learning new technologies
- Your career goals and motivations
Behavioral questions are often interspersed throughout the interview or may come at the end. They typically take about 10-15 minutes in total.
7. Questions for the Interviewer
At the end of the interview, you’ll usually be given the opportunity to ask questions about the company, team, or role. This is your chance to demonstrate your interest and enthusiasm for the position. Some good questions to ask include:
- What does a typical day look like for someone in this role?
- What are the biggest challenges facing the team right now?
- How does the company support professional development and learning?
- What’s the team’s development process and tech stack?
This phase typically lasts about 5-10 minutes and concludes the interview.
Tips for Success in Coding Interviews
Now that we’ve covered the typical format of a coding interview, here are some tips to help you succeed:
1. Practice, Practice, Practice
The key to performing well in coding interviews is consistent practice. Use platforms like LeetCode, HackerRank, or AlgoCademy to solve coding problems regularly. Focus on common data structures and algorithms, and try to solve problems under timed conditions to simulate the pressure of an actual interview.
2. Think Out Loud
During the coding challenge, verbalize your thought process. Interviewers are interested in how you approach problems, not just the final solution. Explaining your reasoning can also help you catch mistakes early and demonstrate your problem-solving skills.
3. Ask Clarifying Questions
Don’t hesitate to ask questions about the problem statement. Clarifying requirements and constraints upfront can save you time and prevent misunderstandings later.
4. Start with a Brute Force Solution
If you’re stuck, start by explaining a brute force solution. This shows that you can at least solve the problem, and you can then work on optimizing your approach.
5. Learn to Analyze Time and Space Complexity
Understanding Big O notation and being able to analyze the efficiency of your algorithms is crucial. Practice explaining the time and space complexity of your solutions.
6. Study System Design Concepts
For more senior positions, familiarize yourself with system design principles and practice designing large-scale systems. Resources like “Designing Data-Intensive Applications” by Martin Kleppmann can be very helpful.
7. Prepare for Behavioral Questions
Have a few stories ready about your past projects, challenges you’ve overcome, and your contributions to team efforts. Use the STAR method (Situation, Task, Action, Result) to structure your responses.
8. Stay Calm and Positive
Coding interviews can be stressful, but try to stay calm and maintain a positive attitude. If you get stuck, take a deep breath and don’t be afraid to ask for hints or clarification.
Conclusion
Understanding the typical format of a coding interview is the first step in preparing for success. By familiarizing yourself with each phase of the interview process and practicing regularly, you can build the skills and confidence needed to excel in technical interviews.
Remember that the goal of a coding interview is not just to assess your technical skills, but also to evaluate your problem-solving approach, communication abilities, and cultural fit. By focusing on all these aspects, you can present yourself as a well-rounded candidate who brings value beyond just writing code.
Platforms like AlgoCademy can be invaluable in your preparation journey, offering interactive coding tutorials, AI-powered assistance, and step-by-step guidance to help you master the skills needed for coding interviews. Whether you’re a beginner looking to build a strong foundation or an experienced developer preparing for interviews at top tech companies, consistent practice and a structured approach to learning can significantly improve your chances of success.
Good luck with your coding interviews, and remember that each interview, regardless of the outcome, is an opportunity to learn and grow as a developer!