What Is the MERN Stack? A Guide for Full-Stack Developers
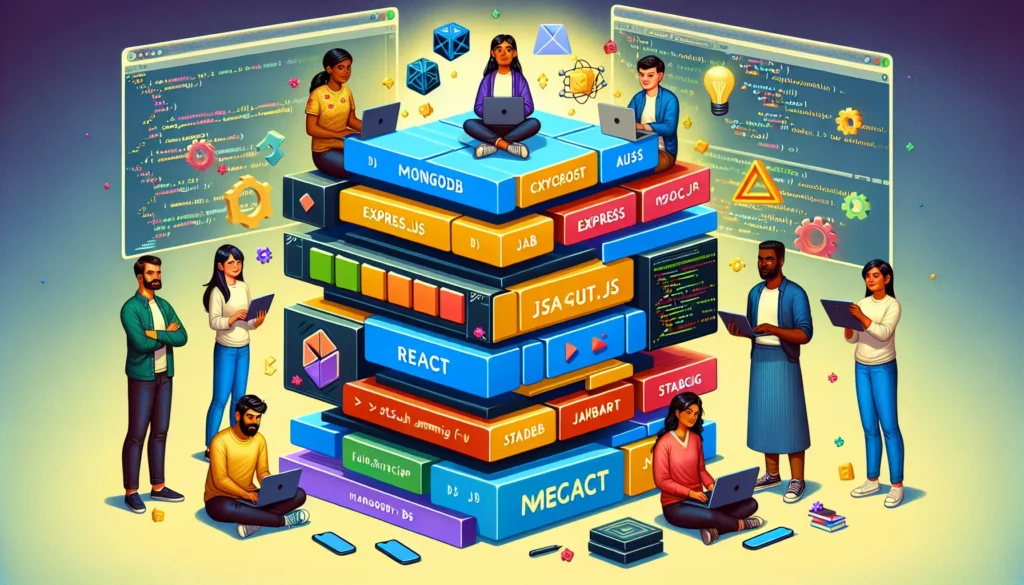
In the ever-evolving world of web development, staying up-to-date with the latest technologies and frameworks is crucial for developers looking to build modern, efficient, and scalable applications. One such popular technology stack that has gained significant traction in recent years is the MERN stack. Whether you’re a seasoned developer or just starting your journey in web development, understanding the MERN stack can open up new opportunities and enhance your skill set. In this comprehensive guide, we’ll dive deep into the MERN stack, exploring its components, advantages, and how it can be used to create powerful full-stack applications.
What is the MERN Stack?
The MERN stack is a collection of four powerful technologies that work together seamlessly to create full-stack web applications. MERN is an acronym that stands for:
- MongoDB: A NoSQL database
- Express.js: A web application framework for Node.js
- React: A JavaScript library for building user interfaces
- Node.js: A JavaScript runtime environment
These four technologies combine to create a robust and flexible framework for building modern web applications. Let’s explore each component in detail to understand its role in the MERN stack.
MongoDB: The Database Layer
MongoDB is a popular NoSQL database that stores data in flexible, JSON-like documents. Unlike traditional relational databases, MongoDB doesn’t require a predefined schema, allowing for greater flexibility and easier scalability. Here are some key features of MongoDB:
- Document-oriented storage: Data is stored in flexible BSON (Binary JSON) documents
- Scalability: Supports horizontal scaling through sharding
- Flexible schema: Allows for easy modifications to data structure
- Rich query language: Supports complex queries and indexing
- Aggregation framework: Powerful tool for data analysis and processing
MongoDB is particularly well-suited for applications that deal with large volumes of unstructured or semi-structured data. Its flexibility makes it an excellent choice for startups and projects where the data model may evolve over time.
Example of creating a MongoDB document:
db.users.insertOne({
name: "John Doe",
email: "john@example.com",
age: 30,
interests: ["coding", "reading", "traveling"]
})
Express.js: The Web Application Framework
Express.js is a minimal and flexible web application framework for Node.js. It provides a robust set of features for building web and mobile applications. Express.js simplifies the process of creating server-side logic and APIs. Some key features of Express.js include:
- Routing: Easily define routes for handling HTTP requests
- Middleware support: Extend functionality through middleware functions
- Template engine integration: Render dynamic content using various template engines
- Static file serving: Serve static files like images, CSS, and JavaScript
- Error handling: Built-in error handling middleware
Express.js acts as the backend framework in the MERN stack, handling server-side logic, routing, and API creation.
Example of a basic Express.js server:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
React: The Frontend Library
React is a popular JavaScript library for building user interfaces. Developed and maintained by Facebook, React has become the go-to choice for many developers when it comes to creating interactive and dynamic front-end applications. Some of the key features of React include:
- Component-based architecture: Build UIs using reusable components
- Virtual DOM: Efficiently update and render components
- JSX: Write HTML-like syntax within JavaScript
- Unidirectional data flow: Manage application state more predictably
- Rich ecosystem: Access to a vast library of third-party components and tools
React forms the frontend part of the MERN stack, allowing developers to create responsive and interactive user interfaces.
Example of a simple React component:
import React from 'react';
function Greeting({ name }) {
return (
<div>
<h1>Hello, {name}!</h1>
<p>Welcome to our MERN stack application.</p>
</div>
);
}
export default Greeting;
Node.js: The Runtime Environment
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows developers to run JavaScript on the server-side, enabling the creation of scalable and high-performance web applications. Key features of Node.js include:
- Asynchronous and event-driven: Efficiently handle concurrent operations
- NPM (Node Package Manager): Access to a vast ecosystem of open-source packages
- Cross-platform compatibility: Run on various operating systems
- Built-in modules: Includes modules for file system operations, networking, and more
- Scalability: Well-suited for building real-time and data-intensive applications
In the MERN stack, Node.js serves as the runtime environment for both the Express.js server and any server-side JavaScript code.
Example of a simple Node.js script:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
Advantages of the MERN Stack
Now that we’ve explored each component of the MERN stack, let’s discuss why it has become such a popular choice for full-stack development:
- JavaScript Everywhere: One of the biggest advantages of the MERN stack is that it uses JavaScript throughout the entire stack. This allows developers to use a single language for both frontend and backend development, reducing the learning curve and increasing productivity.
- Flexible and Scalable: MongoDB’s flexible schema and Node.js’s scalability make the MERN stack suitable for projects of all sizes, from small prototypes to large-scale applications.
- Large and Active Community: All components of the MERN stack have large and active communities, which means plenty of resources, tutorials, and third-party packages are available.
- Efficient Development: The combination of React’s component-based architecture and Express.js’s minimalist approach allows for rapid development and easy maintenance of applications.
- Real-time Applications: The MERN stack is well-suited for building real-time applications, thanks to Node.js’s event-driven architecture and React’s efficient update mechanism.
- Cost-effective: All components of the MERN stack are open-source and free to use, making it a cost-effective solution for businesses and individual developers alike.
- Easy Testing: With tools like Jest for React and Mocha for Node.js, testing MERN stack applications is straightforward and efficient.
Building a MERN Stack Application: A Basic Example
To better understand how the MERN stack works together, let’s walk through a basic example of creating a simple MERN stack application. We’ll create a basic “Todo” application that allows users to add and view tasks.
Step 1: Setting up the Backend
First, let’s set up our Express.js server and connect it to MongoDB:
// server.js
const express = require('express');
const mongoose = require('mongoose');
const cors = require('cors');
const app = express();
const PORT = process.env.PORT || 5000;
// Middleware
app.use(cors());
app.use(express.json());
// Connect to MongoDB
mongoose.connect('mongodb://localhost/mern_todo', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('MongoDB connected'))
.catch(err => console.log(err));
// Define Todo model
const Todo = mongoose.model('Todo', {
text: String,
completed: Boolean
});
// Routes
app.get('/api/todos', async (req, res) => {
const todos = await Todo.find();
res.json(todos);
});
app.post('/api/todos', async (req, res) => {
const newTodo = new Todo({
text: req.body.text,
completed: false
});
await newTodo.save();
res.json(newTodo);
});
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Step 2: Creating the React Frontend
Now, let’s create a simple React application to interact with our backend:
// App.js
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function App() {
const [todos, setTodos] = useState([]);
const [newTodo, setNewTodo] = useState('');
useEffect(() => {
fetchTodos();
}, []);
const fetchTodos = async () => {
const response = await axios.get('http://localhost:5000/api/todos');
setTodos(response.data);
};
const addTodo = async (e) => {
e.preventDefault();
await axios.post('http://localhost:5000/api/todos', { text: newTodo });
setNewTodo('');
fetchTodos();
};
return (
<div>
<h1>MERN Todo App</h1>
<form onSubmit={addTodo}>
<input
type="text"
value={newTodo}
onChange={(e) => setNewTodo(e.target.value)}
placeholder="Enter a new todo"
/>
<button type="submit">Add Todo</button>
</form>
<ul>
{todos.map((todo) => (
<li key={todo._id}>{todo.text}</li>
))}
</ul>
</div>
);
}
export default App;
This example demonstrates how the different components of the MERN stack work together to create a full-stack application. The MongoDB database stores the todos, Express.js provides the API endpoints, React renders the user interface, and Node.js runs the server-side code.
Best Practices for MERN Stack Development
To make the most of the MERN stack and ensure you’re building robust, scalable applications, consider the following best practices:
- Use Async/Await: Leverage async/await syntax for handling asynchronous operations in Node.js and React, making your code more readable and easier to maintain.
- Implement proper error handling: Use try-catch blocks and create custom error handlers to manage errors effectively in both frontend and backend code.
- Utilize environment variables: Store sensitive information like database credentials and API keys in environment variables to enhance security.
- Implement data validation: Use libraries like Joi or Yup to validate data on both the client and server sides to ensure data integrity.
- Use React Hooks: Leverage React Hooks like useState, useEffect, and useContext to manage state and side effects in your React components.
- Implement proper authentication and authorization: Use JWT (JSON Web Tokens) or other secure authentication methods to protect your application and its data.
- Optimize database queries: Use MongoDB’s indexing features and aggregation pipeline to optimize database operations for better performance.
- Implement caching: Use caching mechanisms like Redis to improve the performance of frequently accessed data.
- Write unit and integration tests: Implement comprehensive testing for both frontend and backend code to ensure reliability and catch bugs early.
- Use code linting and formatting tools: Employ tools like ESLint and Prettier to maintain consistent code style and catch potential issues early in the development process.
Challenges and Considerations
While the MERN stack offers numerous advantages, it’s important to be aware of potential challenges and considerations:
- Learning Curve: Although using JavaScript throughout the stack can be an advantage, mastering all components of the MERN stack can still be challenging, especially for beginners.
- Rapid Ecosystem Changes: The JavaScript ecosystem, particularly in the React world, evolves rapidly. Keeping up with the latest best practices and updates can be demanding.
- Scalability Considerations: While Node.js is known for its scalability, improper implementation can lead to performance issues in high-traffic applications. It’s crucial to implement proper scaling strategies.
- Security: As with any web application, security is a critical concern. Developers must be vigilant about implementing proper security measures, especially when dealing with user data and authentication.
- Choosing the Right Tools: The MERN ecosystem offers a wide variety of libraries and tools. Selecting the right ones for your project can be overwhelming and requires careful consideration.
Conclusion
The MERN stack has emerged as a powerful and flexible solution for full-stack web development. By combining MongoDB, Express.js, React, and Node.js, developers can create scalable, efficient, and modern web applications using JavaScript throughout the entire stack. The MERN stack’s popularity is due to its flexibility, scalability, and the strong community support behind each of its components.
As you embark on your journey with the MERN stack, remember that mastering these technologies takes time and practice. Start with small projects, gradually increasing complexity as you become more comfortable with each component. Take advantage of the vast resources available online, including documentation, tutorials, and community forums.
Whether you’re building a simple todo app or a complex enterprise-level application, the MERN stack provides the tools and flexibility to bring your ideas to life. By following best practices and staying up-to-date with the latest developments in each technology, you’ll be well-equipped to tackle the challenges of modern web development.
As you continue to explore and work with the MERN stack, you’ll discover its true power in creating robust, scalable, and efficient web applications. Happy coding!