What is the Fastest Way to Learn JavaScript? A Comprehensive Guide
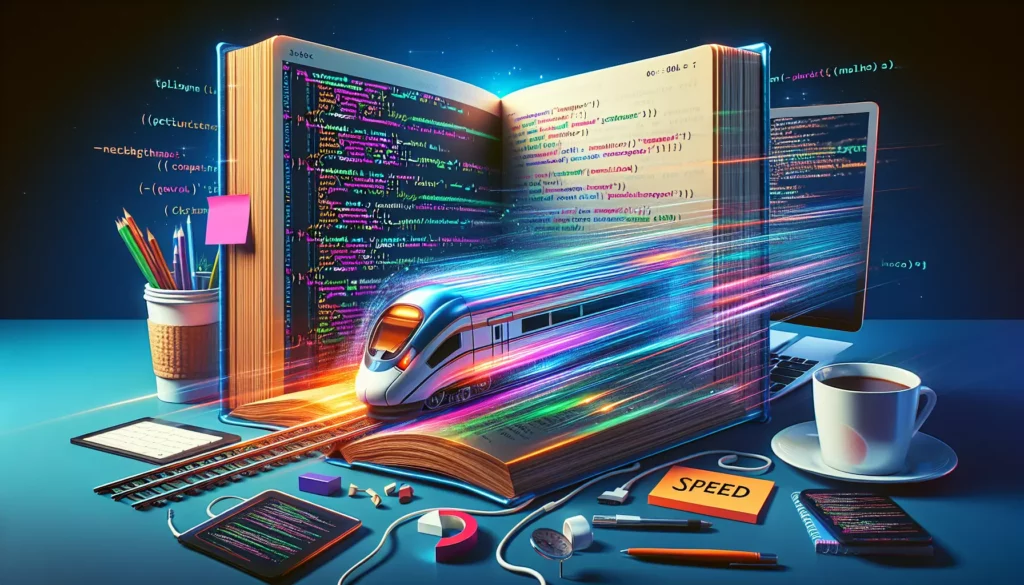
JavaScript has become an essential language for web development, powering interactive and dynamic websites across the internet. Whether you’re a beginner looking to start your coding journey or an experienced developer aiming to add JavaScript to your skill set, you might be wondering: what is the fastest way to learn JavaScript? In this comprehensive guide, we’ll explore various strategies, resources, and techniques to help you master JavaScript efficiently and effectively.
Table of Contents
- Understanding the Basics
- Hands-on Practice: The Key to Rapid Learning
- Leveraging Online Resources
- Tackling Coding Challenges
- Building Real-world Projects
- Engaging with the Developer Community
- Diving into Advanced Concepts
- Staying Updated with JavaScript Trends
- Preparing for Technical Interviews
- Conclusion
Understanding the Basics
Before diving into complex concepts, it’s crucial to build a solid foundation in JavaScript basics. Here are some fundamental topics you should focus on:
- Variables and data types
- Operators and expressions
- Control structures (if statements, loops)
- Functions and scope
- Arrays and objects
- DOM manipulation
Start by dedicating time to understand these core concepts thoroughly. Many beginners make the mistake of rushing through the basics, only to struggle with more advanced topics later. Take your time to experiment with each concept and ensure you have a firm grasp before moving forward.
Hands-on Practice: The Key to Rapid Learning
The fastest way to learn JavaScript is through consistent, hands-on practice. Reading about concepts is important, but actually writing code is where the real learning happens. Here are some ways to incorporate regular practice into your learning routine:
- Code every day: Set aside dedicated time each day, even if it’s just 30 minutes, to write JavaScript code.
- Use interactive coding environments: Platforms like CodePen, JSFiddle, or Repl.it allow you to experiment with code snippets quickly without setting up a local development environment.
- Recreate existing features: Try to replicate simple features you encounter on websites, such as dropdown menus, image sliders, or form validation.
- Solve coding exercises: Websites like LeetCode, HackerRank, or Codewars offer a variety of JavaScript challenges to test and improve your skills.
Remember, consistency is key. Regular practice, even in small doses, is more effective than sporadic, lengthy coding sessions.
Leveraging Online Resources
The internet is filled with valuable resources for learning JavaScript. Here are some top recommendations:
- MDN Web Docs: Mozilla’s official documentation is an excellent reference for JavaScript syntax and features.
- freeCodeCamp: Offers a comprehensive JavaScript curriculum with interactive lessons and projects.
- Codecademy: Provides interactive JavaScript courses suitable for beginners.
- JavaScript.info: A modern tutorial covering JavaScript fundamentals and advanced topics.
- YouTube tutorials: Channels like Traversy Media, The Net Ninja, and Fun Fun Function offer in-depth JavaScript tutorials.
While these resources are valuable, it’s important not to get caught in “tutorial hell.” Balance your learning by applying what you learn to your own projects.
Tackling Coding Challenges
Coding challenges are an excellent way to sharpen your problem-solving skills and deepen your understanding of JavaScript. Platforms like LeetCode, HackerRank, and AlgoCademy offer a wide range of problems, from beginner to advanced levels.
Here’s how to make the most of coding challenges:
- Start with easy problems and gradually increase difficulty.
- Try to solve problems on your own before looking at solutions.
- After solving a problem, study other solutions to learn different approaches.
- Focus on understanding the underlying concepts rather than memorizing solutions.
- Regularly revisit and re-solve problems to reinforce your learning.
Remember, the goal is not just to solve the problem, but to improve your overall coding skills and algorithmic thinking.
Building Real-world Projects
One of the most effective ways to rapidly improve your JavaScript skills is by building real-world projects. Projects allow you to apply your knowledge in practical scenarios, encounter and solve real problems, and create something tangible that you can showcase.
Here are some project ideas to get you started:
- To-do list application: A classic project that covers DOM manipulation, event handling, and local storage.
- Weather app: Build an app that fetches weather data from an API and displays it, introducing you to asynchronous JavaScript and API integration.
- Quiz game: Create an interactive quiz that tests users’ knowledge on a topic, practicing array manipulation and DOM updates.
- Personal portfolio website: Showcase your projects and skills while learning about responsive design and advanced CSS.
- E-commerce site: A more advanced project involving product listings, shopping cart functionality, and payment integration.
As you work on these projects, you’ll encounter challenges that will push you to learn new concepts and techniques. This hands-on experience is invaluable for rapid skill development.
Engaging with the Developer Community
Learning JavaScript doesn’t have to be a solitary journey. Engaging with the developer community can significantly accelerate your learning process. Here’s how you can get involved:
- Join coding forums: Websites like Stack Overflow, Reddit’s r/learnjavascript, or the freeCodeCamp forum are great places to ask questions and help others.
- Participate in local meetups: Many cities have JavaScript or web development meetups where you can network with other developers and learn from talks and workshops.
- Contribute to open-source projects: Once you’ve gained some experience, contributing to open-source JavaScript projects can be an excellent way to improve your skills and give back to the community.
- Attend coding bootcamps or workshops: While often more intensive and potentially costly, these can provide structured learning and networking opportunities.
Remember, every experienced developer was once a beginner. Don’t be afraid to ask questions or seek help when you’re stuck.
Diving into Advanced Concepts
Once you’ve mastered the basics and built a few projects, it’s time to explore more advanced JavaScript concepts. These topics will help you write more efficient, maintainable, and powerful code:
- Closures and scope: Understanding how JavaScript handles variable scope and the power of closures.
- Prototypal inheritance: Learn how JavaScript’s object system works under the hood.
- Asynchronous JavaScript: Master callbacks, promises, and async/await for handling asynchronous operations.
- Functional programming: Explore concepts like pure functions, immutability, and higher-order functions.
- ES6+ features: Get familiar with modern JavaScript syntax and features like arrow functions, destructuring, and modules.
- Design patterns: Learn common patterns like Module, Observer, and Singleton to solve recurring design problems.
Here’s a code snippet demonstrating the use of some ES6+ features:
// Using destructuring and arrow functions
const getFullName = ({ firstName, lastName }) => `${firstName} ${lastName}`;
// Using the spread operator and template literals
const numbers = [1, 2, 3];
console.log(`The numbers are: ${[...numbers, 4, 5]}`);
// Using async/await for asynchronous operations
const fetchData = async (url) => {
try {
const response = await fetch(url);
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
}
};
As you delve into these advanced concepts, make sure to apply them in your projects to solidify your understanding.
Staying Updated with JavaScript Trends
JavaScript is an evolving language, with new features and best practices emerging regularly. Staying updated with the latest trends is crucial for any JavaScript developer. Here are some ways to keep your knowledge current:
- Follow JavaScript blogs and news sites: Websites like JavaScript Weekly, CSS-Tricks, and Smashing Magazine often cover the latest JavaScript developments.
- Attend conferences or watch conference talks online: Many JavaScript conferences post their talks on YouTube, allowing you to learn from industry experts.
- Explore new libraries and frameworks: While mastering vanilla JavaScript is crucial, familiarizing yourself with popular libraries and frameworks like React, Vue, or Angular can broaden your skillset.
- Read ECMAScript proposals: Keep an eye on upcoming JavaScript features by following the ECMAScript proposal process.
Remember, while it’s important to be aware of new trends, focus on mastering the fundamentals first. New features and frameworks come and go, but solid core JavaScript skills will always be valuable.
Preparing for Technical Interviews
If your goal is to land a job as a JavaScript developer, you’ll need to prepare for technical interviews. Here are some tips to help you succeed:
- Review common JavaScript interview questions: Familiarize yourself with frequently asked questions about closures, hoisting, this keyword, and more.
- Practice coding on a whiteboard: Many interviews involve coding without the help of an IDE, so practice writing code by hand or on a whiteboard.
- Study data structures and algorithms: While not always directly related to JavaScript, these topics are common in technical interviews.
- Mock interviews: Practice with friends or use platforms like Pramp that offer peer-to-peer mock interviews.
- Review your projects: Be prepared to discuss the projects you’ve built, explaining your design decisions and the challenges you faced.
Here’s an example of a common JavaScript interview question and a potential solution:
// Question: Implement a function to flatten a nested array
function flattenArray(arr) {
return arr.reduce((flat, toFlatten) => {
return flat.concat(Array.isArray(toFlatten) ? flattenArray(toFlatten) : toFlatten);
}, []);
}
// Example usage:
const nestedArray = [1, [2, 3], [4, [5, 6]]];
console.log(flattenArray(nestedArray)); // Output: [1, 2, 3, 4, 5, 6]
This solution uses recursion and the reduce method to flatten the nested array. Understanding and being able to explain such solutions is crucial for technical interviews.
Conclusion
Learning JavaScript quickly requires a combination of structured learning, consistent practice, project building, and community engagement. While there’s no magic shortcut to mastering JavaScript, following the strategies outlined in this guide can significantly accelerate your learning process.
Remember these key points:
- Build a solid foundation in the basics before moving to advanced topics.
- Practice coding regularly, even if it’s just for short periods each day.
- Use a variety of resources, including documentation, tutorials, and coding challenges.
- Build real-world projects to apply your knowledge and face practical challenges.
- Engage with the developer community to learn from others and stay motivated.
- Stay updated with the latest JavaScript trends and best practices.
- Prepare thoroughly for technical interviews if you’re aiming for a developer role.
Learning JavaScript is a journey, and everyone’s path will be slightly different. Be patient with yourself, celebrate your progress, and remember that even experienced developers are constantly learning and improving their skills.
As you embark on your JavaScript learning journey, consider leveraging platforms like AlgoCademy, which offers interactive coding tutorials, AI-powered assistance, and resources specifically designed to help learners progress from beginner-level coding to preparing for technical interviews at major tech companies.
With dedication, practice, and the right resources, you’ll be well on your way to becoming a proficient JavaScript developer. Happy coding!