What is the Fastest Way to Learn JavaScript? A Comprehensive Guide
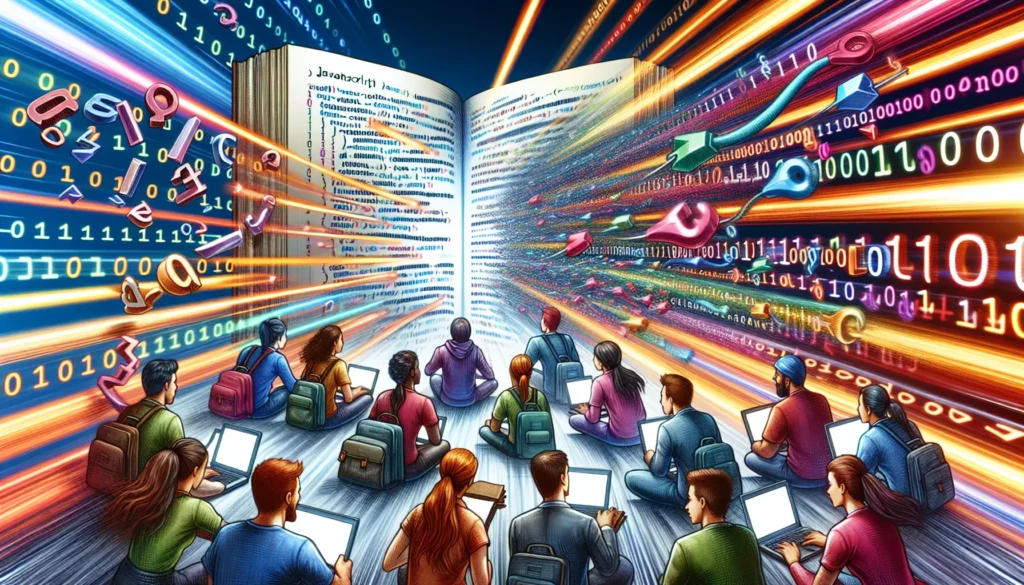
JavaScript has become an essential skill for any aspiring web developer or programmer. As one of the most popular programming languages, it powers the interactive elements of countless websites and web applications. If you’re looking to dive into the world of coding or enhance your existing skills, learning JavaScript quickly and effectively can be a game-changer. In this comprehensive guide, we’ll explore the fastest ways to learn JavaScript, providing you with strategies, resources, and tips to accelerate your learning journey.
Table of Contents
- Why Learn JavaScript?
- Set Clear Goals and Expectations
- Start with the Basics
- Embrace Interactive Learning Platforms
- Practice, Practice, Practice
- Build Real Projects
- Join a Coding Community
- Read Documentation and Books
- Watch Video Tutorials and Courses
- Try Pair Programming
- Tackle Coding Challenges
- Teach Others What You Learn
- Stay Updated with the Latest Trends
- Leverage Developer Tools and Extensions
- Optimize Your Learning Environment
- Conclusion
Why Learn JavaScript?
Before we dive into the fastest ways to learn JavaScript, it’s important to understand why it’s such a valuable skill to acquire:
- Versatility: JavaScript can be used for front-end and back-end development, making it a full-stack language.
- High Demand: JavaScript developers are in high demand across various industries.
- Community Support: A large and active community means plenty of resources and support for learners.
- Constant Evolution: The language is continuously improving, with new features and frameworks regularly introduced.
- Career Opportunities: Proficiency in JavaScript can open doors to numerous career paths in tech.
Set Clear Goals and Expectations
The fastest way to learn JavaScript starts with setting clear goals and realistic expectations. Here’s how to approach this:
- Define Your Objectives: Determine what you want to achieve with JavaScript. Do you want to build websites, create mobile apps, or focus on server-side programming?
- Set a Timeline: Establish a realistic timeframe for achieving your goals. Be specific about how much time you can dedicate daily or weekly to learning.
- Break Down Your Goals: Divide your main objective into smaller, manageable milestones. This makes the learning process less overwhelming and more structured.
- Be Realistic: Remember that learning a programming language takes time and effort. Don’t expect to become an expert overnight.
Start with the Basics
To build a solid foundation in JavaScript, focus on mastering these fundamental concepts:
- Variables and Data Types
- Operators and Expressions
- Control Structures (if statements, loops)
- Functions
- Arrays and Objects
- DOM Manipulation
- Event Handling
Here’s a simple example of a JavaScript function that demonstrates some of these basics:
function greet(name) {
if (name) {
console.log("Hello, " + name + "!");
} else {
console.log("Hello, stranger!");
}
}
greet("Alice"); // Outputs: Hello, Alice!
greet(); // Outputs: Hello, stranger!
Embrace Interactive Learning Platforms
Interactive learning platforms can significantly speed up your JavaScript learning process. These platforms offer hands-on coding experiences, immediate feedback, and structured curricula. Some popular options include:
- Codecademy: Offers interactive JavaScript courses with coding exercises and projects.
- freeCodeCamp: Provides a comprehensive JavaScript curriculum with certification.
- AlgoCademy: Focuses on algorithmic thinking and problem-solving skills, which are crucial for mastering JavaScript.
- LeetCode: Offers coding challenges and interview preparation, including JavaScript-specific problems.
- Codewars: Provides gamified coding challenges to improve your JavaScript skills.
Practice, Practice, Practice
The key to rapidly improving your JavaScript skills is consistent practice. Here are some ways to incorporate regular coding practice into your routine:
- Daily Coding Sessions: Set aside time each day, even if it’s just 30 minutes, to write JavaScript code.
- Code Katas: Solve small, focused programming exercises to build muscle memory and improve problem-solving skills.
- Recreate Existing Websites: Try to recreate the functionality of popular websites or web applications using JavaScript.
- Experiment with Code: Don’t be afraid to experiment and break things. Learning from errors is an essential part of the process.
Build Real Projects
Building real-world projects is one of the most effective ways to learn JavaScript quickly. It allows you to apply your knowledge, face practical challenges, and create something tangible. Here are some project ideas to get you started:
- A simple to-do list application
- A calculator with basic arithmetic operations
- A weather app that fetches data from an API
- A personal portfolio website
- A basic game like Tic-Tac-Toe or Snake
Here’s a simple example of how you might start a to-do list application:
let todos = [];
function addTodo(task) {
todos.push({ task: task, completed: false });
}
function displayTodos() {
for (let i = 0; i < todos.length; i++) {
console.log((i + 1) + ". " + todos[i].task + (todos[i].completed ? " (Completed)" : ""));
}
}
addTodo("Learn JavaScript");
addTodo("Build a project");
displayTodos();
// Outputs:
// 1. Learn JavaScript
// 2. Build a project
Join a Coding Community
Becoming part of a coding community can accelerate your learning process by providing support, motivation, and opportunities for collaboration. Consider these options:
- GitHub: Contribute to open-source projects and learn from other developers’ code.
- Stack Overflow: Ask questions and help others with their JavaScript problems.
- Reddit: Join subreddits like r/learnjavascript or r/webdev for discussions and resources.
- Discord: Join JavaScript-focused Discord servers to chat with other learners and experienced developers.
- Meetups: Attend local JavaScript meetups or coding events to network and learn from others in person.
Read Documentation and Books
While interactive learning is crucial, reading documentation and books can provide in-depth knowledge and help you understand JavaScript concepts more thoroughly. Here are some recommended resources:
- MDN Web Docs: Mozilla’s comprehensive documentation for JavaScript and web technologies.
- “Eloquent JavaScript” by Marijn Haverbeke: A free online book that covers JavaScript in depth.
- “You Don’t Know JS” series by Kyle Simpson: A series of books that dive deep into JavaScript’s core mechanisms.
- JavaScript.info: A modern JavaScript tutorial with simple but detailed explanations.
Watch Video Tutorials and Courses
Visual learners may find video tutorials and online courses particularly helpful in accelerating their JavaScript learning. Some popular platforms and channels include:
- YouTube: Channels like Traversy Media, The Net Ninja, and FreeCodeCamp offer free JavaScript tutorials.
- Udemy: Offers a wide range of JavaScript courses, from beginner to advanced levels.
- Pluralsight: Provides in-depth JavaScript courses for various skill levels.
- egghead.io: Offers bite-sized video tutorials on JavaScript and related technologies.
Try Pair Programming
Pair programming is a technique where two developers work together on the same code. This can be an excellent way to learn JavaScript faster:
- Find a Coding Partner: Look for someone with a similar skill level or a mentor who can guide you.
- Use Collaborative Tools: Utilize platforms like CodePen, JSFiddle, or VS Code Live Share for remote pair programming.
- Take Turns: Alternate between “driving” (writing code) and “navigating” (reviewing and suggesting improvements).
- Discuss Your Approach: Explain your thought process and listen to your partner’s ideas.
Tackle Coding Challenges
Solving coding challenges can sharpen your problem-solving skills and expose you to various JavaScript concepts. Here are some platforms that offer JavaScript-specific challenges:
- HackerRank: Offers a wide range of JavaScript challenges and competitions.
- Codewars: Provides gamified coding challenges called “katas” to improve your skills.
- Project Euler: Offers mathematically-oriented programming problems that can be solved using JavaScript.
- AlgoCademy: Focuses on algorithmic challenges that prepare you for technical interviews.
Here’s an example of a simple coding challenge you might encounter:
// Challenge: Write a function that reverses a string
function reverseString(str) {
return str.split("").reverse().join("");
}
console.log(reverseString("hello")); // Outputs: "olleh"
Teach Others What You Learn
Teaching is one of the most effective ways to solidify your own understanding of JavaScript. Here’s how you can incorporate teaching into your learning process:
- Start a Blog: Write articles explaining JavaScript concepts you’ve learned.
- Create YouTube Tutorials: Make video tutorials on specific JavaScript topics or projects.
- Answer Questions: Help others on forums like Stack Overflow or Reddit.
- Mentor a Beginner: Offer to mentor someone who’s just starting with JavaScript.
Stay Updated with the Latest Trends
JavaScript is an evolving language, and staying up-to-date with the latest features and best practices can give you an edge. Here’s how to keep yourself informed:
- Follow JavaScript Blogs: Read blogs like JavaScript Weekly, DailyJS, and 2ality.
- Attend Conferences: Participate in JavaScript conferences, either in-person or virtually.
- Listen to Podcasts: Subscribe to podcasts like JavaScript Jabber or Syntax.
- Follow Influencers: Follow JavaScript experts and influencers on social media platforms.
Leverage Developer Tools and Extensions
Using the right tools can significantly speed up your JavaScript learning and development process. Consider incorporating these tools into your workflow:
- Browser Developer Tools: Learn to use the JavaScript console and debugger in your browser.
- Code Editors: Use powerful editors like Visual Studio Code, Sublime Text, or WebStorm.
- Linters: Use tools like ESLint to catch errors and enforce coding standards.
- Version Control: Learn Git to manage your code and collaborate with others.
- Package Managers: Familiarize yourself with npm or Yarn for managing JavaScript libraries.
Optimize Your Learning Environment
Creating an optimal learning environment can significantly impact how quickly you learn JavaScript. Consider these tips:
- Minimize Distractions: Find a quiet space where you can focus on coding without interruptions.
- Use Productivity Techniques: Try methods like the Pomodoro Technique to manage your study time effectively.
- Take Regular Breaks: Give your brain time to process new information by taking short breaks between coding sessions.
- Stay Healthy: Maintain a balanced diet, exercise regularly, and get enough sleep to keep your mind sharp.
- Set Up a Comfortable Workspace: Ensure your desk, chair, and computer setup are ergonomic to prevent fatigue during long coding sessions.
Conclusion
Learning JavaScript quickly requires a combination of structured learning, consistent practice, and real-world application. By following the strategies outlined in this guide, you can accelerate your JavaScript learning journey and build a solid foundation for your programming career.
Remember, the key to mastering JavaScript (or any programming language) is persistence and dedication. Don’t get discouraged if you face challenges along the way – they’re an essential part of the learning process. Stay curious, keep experimenting, and most importantly, enjoy the process of creating with code.
As you progress in your JavaScript journey, consider exploring more advanced topics like:
- Asynchronous JavaScript and Promises
- ES6+ features and syntax
- JavaScript frameworks like React, Vue, or Angular
- Node.js for server-side JavaScript
- Testing frameworks and methodologies
By continuously challenging yourself and expanding your knowledge, you’ll not only learn JavaScript quickly but also develop the skills to become a proficient and versatile developer. Happy coding!