What is Test-Driven Development (TDD)? A Comprehensive Guide for Aspiring Developers
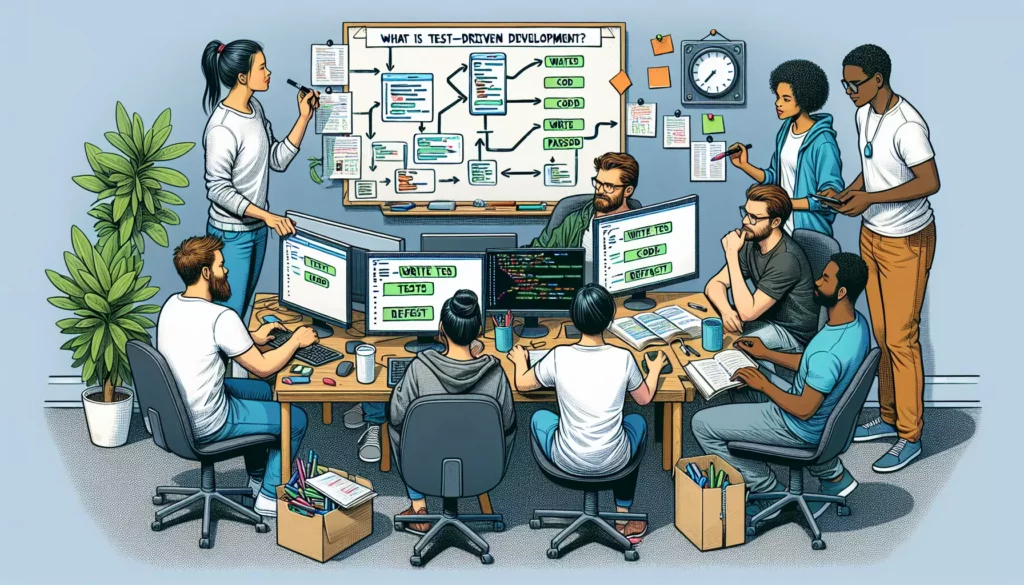
In the world of software development, methodologies and practices come and go, but some stand the test of time due to their effectiveness and the value they bring to the development process. One such practice that has gained significant traction and maintained its relevance is Test-Driven Development (TDD). Whether you’re a beginner coder or preparing for technical interviews at major tech companies, understanding TDD can significantly enhance your programming skills and make you a more effective developer.
Understanding Test-Driven Development
Test-Driven Development is a software development approach where tests are written before the actual code. This might sound counterintuitive at first, but it’s a powerful technique that can lead to better designed, more reliable, and easier to maintain software.
The basic TDD cycle, often referred to as “Red-Green-Refactor,” consists of three steps:
- Red: Write a failing test for the functionality you want to implement.
- Green: Write the minimum amount of code necessary to make the test pass.
- Refactor: Improve the code without changing its functionality.
This cycle is repeated for each new piece of functionality, resulting in a comprehensive suite of tests and well-structured code.
The Benefits of Test-Driven Development
TDD offers numerous advantages that make it a valuable practice for developers at all levels:
1. Improved Code Quality
By writing tests first, you’re forced to think about the design and functionality of your code before you start implementing it. This often leads to cleaner, more modular code that’s easier to understand and maintain.
2. Better Documentation
Tests serve as living documentation for your code. They describe what the code should do in various scenarios, making it easier for other developers (or yourself in the future) to understand the intended behavior of the system.
3. Faster Debugging
When a test fails, you immediately know where the problem is. This can significantly reduce debugging time, especially in large codebases.
4. Confidence in Refactoring
With a comprehensive test suite, you can refactor your code with confidence. If you accidentally break something, your tests will catch it immediately.
5. Reduced Overall Development Time
While writing tests upfront might seem like it slows down development initially, it often leads to faster overall development times. This is because bugs are caught earlier in the development process when they’re cheaper and easier to fix.
Implementing TDD: A Step-by-Step Guide
Let’s walk through a simple example of implementing TDD. We’ll create a function that checks if a number is prime. We’ll use Python for this example, but the principles apply to any programming language.
Step 1: Write a Failing Test
First, we’ll write a test for our yet-to-be-implemented is_prime
function:
import unittest
class TestPrimeChecker(unittest.TestCase):
def test_is_prime(self):
self.assertTrue(is_prime(2))
self.assertTrue(is_prime(3))
self.assertFalse(is_prime(4))
self.assertTrue(is_prime(5))
self.assertFalse(is_prime(6))
self.assertTrue(is_prime(7))
if __name__ == '__main__':
unittest.main()
If we run this test now, it will fail because we haven’t implemented the is_prime
function yet.
Step 2: Write the Minimum Code to Pass the Test
Now, let’s implement the is_prime
function with the minimum code necessary to pass our tests:
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
This implementation should pass all our tests.
Step 3: Refactor
In this case, our implementation is already quite efficient, so there’s not much to refactor. However, we could add some more tests to cover edge cases:
class TestPrimeChecker(unittest.TestCase):
def test_is_prime(self):
self.assertTrue(is_prime(2))
self.assertTrue(is_prime(3))
self.assertFalse(is_prime(4))
self.assertTrue(is_prime(5))
self.assertFalse(is_prime(6))
self.assertTrue(is_prime(7))
self.assertFalse(is_prime(1)) # 1 is not considered prime
self.assertFalse(is_prime(0))
self.assertFalse(is_prime(-1))
self.assertTrue(is_prime(97)) # A larger prime number
if __name__ == '__main__':
unittest.main()
Now we have a more comprehensive test suite that covers more cases, including edge cases.
TDD in Practice: Real-World Scenarios
While the prime number checker is a good introductory example, let’s look at how TDD can be applied in more complex, real-world scenarios that you might encounter in technical interviews or actual development projects.
Scenario 1: Implementing a Stack Data Structure
Let’s implement a Stack data structure using TDD. We’ll start with the tests:
import unittest
class TestStack(unittest.TestCase):
def test_push_and_pop(self):
stack = Stack()
stack.push(1)
stack.push(2)
self.assertEqual(stack.pop(), 2)
self.assertEqual(stack.pop(), 1)
def test_peek(self):
stack = Stack()
stack.push(1)
stack.push(2)
self.assertEqual(stack.peek(), 2)
self.assertEqual(stack.pop(), 2)
self.assertEqual(stack.peek(), 1)
def test_is_empty(self):
stack = Stack()
self.assertTrue(stack.is_empty())
stack.push(1)
self.assertFalse(stack.is_empty())
stack.pop()
self.assertTrue(stack.is_empty())
def test_pop_empty_stack(self):
stack = Stack()
with self.assertRaises(IndexError):
stack.pop()
if __name__ == '__main__':
unittest.main()
Now, let’s implement the Stack class to pass these tests:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if self.is_empty():
raise IndexError("Stack is empty")
return self.items.pop()
def peek(self):
if self.is_empty():
raise IndexError("Stack is empty")
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
This implementation should pass all our tests. The TDD approach ensured that we considered various scenarios, including edge cases like popping from an empty stack.
Scenario 2: Implementing a Simple API Endpoint
Let’s consider a more complex scenario: implementing a simple API endpoint using Flask. We’ll create an endpoint that returns user information. Here’s how we might approach this using TDD:
First, let’s write our tests:
import unittest
import json
from app import app
class TestUserAPI(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
def test_get_user(self):
response = self.app.get('/user/1')
data = json.loads(response.data)
self.assertEqual(response.status_code, 200)
self.assertEqual(data['id'], 1)
self.assertEqual(data['name'], 'John Doe')
self.assertEqual(data['email'], 'john@example.com')
def test_user_not_found(self):
response = self.app.get('/user/999')
self.assertEqual(response.status_code, 404)
def test_invalid_user_id(self):
response = self.app.get('/user/invalid')
self.assertEqual(response.status_code, 400)
if __name__ == '__main__':
unittest.main()
Now, let’s implement the API to pass these tests:
from flask import Flask, jsonify
app = Flask(__name__)
users = {
1: {"id": 1, "name": "John Doe", "email": "john@example.com"}
}
@app.route('/user/<int:user_id>')
def get_user(user_id):
user = users.get(user_id)
if user:
return jsonify(user), 200
else:
return jsonify({"error": "User not found"}), 404
@app.errorhandler(404)
def not_found(error):
return jsonify({"error": "Not found"}), 404
@app.errorhandler(400)
def bad_request(error):
return jsonify({"error": "Bad request"}), 400
if __name__ == '__main__':
app.run(debug=True)
This implementation should pass all our tests. By using TDD, we ensured that our API handles different scenarios correctly, including successful requests, not found errors, and invalid input.
TDD and Algorithmic Problem Solving
Test-Driven Development isn’t just for building applications; it can also be a powerful tool when solving algorithmic problems, which are common in coding interviews, especially for major tech companies.
Let’s look at how TDD can be applied to a classic algorithmic problem: reversing a linked list.
Step 1: Write the Tests
First, we’ll write tests for our linked list reversal function:
import unittest
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def create_linked_list(arr):
dummy = ListNode(0)
current = dummy
for val in arr:
current.next = ListNode(val)
current = current.next
return dummy.next
def linked_list_to_array(head):
arr = []
current = head
while current:
arr.append(current.val)
current = current.next
return arr
class TestReverseLinkedList(unittest.TestCase):
def test_reverse_empty_list(self):
self.assertIsNone(reverse_linked_list(None))
def test_reverse_single_node(self):
head = ListNode(1)
reversed_head = reverse_linked_list(head)
self.assertEqual(linked_list_to_array(reversed_head), [1])
def test_reverse_multiple_nodes(self):
head = create_linked_list([1, 2, 3, 4, 5])
reversed_head = reverse_linked_list(head)
self.assertEqual(linked_list_to_array(reversed_head), [5, 4, 3, 2, 1])
if __name__ == '__main__':
unittest.main()
Step 2: Implement the Function
Now, let’s implement the reverse_linked_list
function to pass these tests:
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
Step 3: Refactor (if necessary)
In this case, our implementation is already quite efficient and readable, so there’s no immediate need for refactoring. However, we could add more tests to cover additional edge cases or scenarios if needed.
By using TDD for this algorithmic problem, we ensured that our function correctly handles various scenarios, including empty lists, single-node lists, and multi-node lists. This approach can be particularly helpful when preparing for coding interviews, as it encourages thorough testing and consideration of edge cases.
Common Challenges and Misconceptions about TDD
While Test-Driven Development offers numerous benefits, it’s not without its challenges and misconceptions. Let’s address some of the common ones:
1. “TDD is Time-Consuming”
Misconception: Writing tests before code slows down development.
Reality: While TDD might seem slower initially, it often leads to faster overall development times. By catching bugs early and providing a safety net for refactoring, TDD can significantly reduce debugging time and make it easier to add new features.
2. “TDD is Only for Unit Testing”
Misconception: TDD is only applicable to unit tests.
Reality: While TDD is often associated with unit testing, it can be applied at various levels of testing, including integration tests and even end-to-end tests.
3. “TDD Leads to Over-Testing”
Misconception: TDD results in writing unnecessary tests.
Reality: Proper TDD focuses on writing meaningful tests that drive the design of your code. It’s about quality, not quantity. Well-written tests should provide value and catch potential issues.
4. “TDD is Difficult to Apply to Legacy Code”
Challenge: Applying TDD to existing codebases can be challenging.
Solution: While it’s true that retrofitting tests to legacy code can be difficult, it’s not impossible. Start by writing tests for new features or bug fixes, and gradually increase test coverage as you refactor existing code.
5. “TDD Doesn’t Work for All Types of Development”
Misconception: TDD is only suitable for certain types of projects.
Reality: While TDD might be easier to apply in some contexts than others, its principles can be adapted to various types of development, from web applications to embedded systems.
TDD Best Practices
To get the most out of Test-Driven Development, consider these best practices:
1. Keep Tests Small and Focused
Each test should focus on a single behavior or scenario. This makes tests easier to write, understand, and maintain.
2. Follow the AAA Pattern
Structure your tests using the Arrange-Act-Assert pattern:
- Arrange: Set up the test conditions
- Act: Perform the action being tested
- Assert: Check the results
3. Use Descriptive Test Names
Your test names should clearly describe what is being tested and under what conditions. This makes it easier to understand what a failing test is trying to tell you.
4. Don’t Test Implementation Details
Focus on testing the behavior of your code, not its internal implementation. This allows you to refactor your code without having to change your tests.
5. Refactor Regularly
Don’t skip the refactor step in the TDD cycle. Regular refactoring keeps your code clean and maintainable.
6. Maintain Your Test Suite
Treat your tests as first-class citizens. Keep them clean, up-to-date, and performant.
Conclusion
Test-Driven Development is a powerful practice that can significantly improve the quality of your code and your effectiveness as a developer. By writing tests first, you’re forced to think about the design and functionality of your code before you implement it, leading to cleaner, more modular, and more reliable software.
While TDD might feel unnatural at first, with practice, it becomes an invaluable tool in your development toolkit. Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews at major tech companies, incorporating TDD into your workflow can help you write better code, catch bugs earlier, and approach problem-solving more systematically.
Remember, the goal of TDD isn’t just to have tests, but to use tests as a tool for designing better software. As you continue your journey in software development, consider how you can incorporate TDD principles into your coding practice. It might just be the key to leveling up your programming skills and becoming a more effective, confident developer.