What Is Spring Boot? A Comprehensive Guide for Java Developers
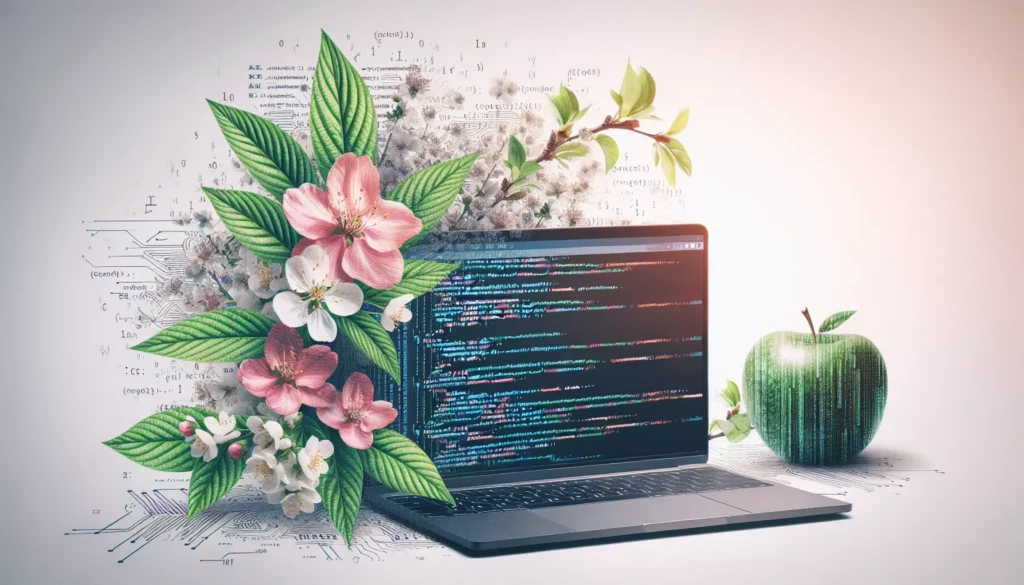
In the ever-evolving landscape of Java development, Spring Boot has emerged as a game-changer, simplifying the process of building robust and scalable applications. Whether you’re a seasoned Java developer or just starting your journey in the world of coding, understanding Spring Boot is crucial for staying competitive in today’s tech industry. In this comprehensive guide, we’ll dive deep into what Spring Boot is, its key features, and why it has become an essential tool for Java developers worldwide.
Table of Contents
- Introduction to Spring Boot
- A Brief History of Spring Boot
- Key Features of Spring Boot
- Advantages of Using Spring Boot
- Getting Started with Spring Boot
- Core Concepts in Spring Boot
- Best Practices for Spring Boot Development
- Spring Boot vs. Traditional Spring Framework
- Real-World Applications of Spring Boot
- The Future of Spring Boot
- Conclusion
Introduction to Spring Boot
Spring Boot is an open-source Java-based framework used to create stand-alone, production-grade Spring-based applications with minimal effort. It’s built on top of the popular Spring Framework and provides a simpler and faster way to set up, configure, and run both simple and web-based applications.
At its core, Spring Boot aims to simplify the development process by eliminating the need for complex XML configurations and providing a range of out-of-the-box features. It follows the “Convention over Configuration” principle, which means it comes with sensible defaults that can be easily overridden when needed.
A Brief History of Spring Boot
To truly appreciate Spring Boot, it’s essential to understand its origins and evolution:
- 2002: The Spring Framework is introduced, revolutionizing Java development with its dependency injection and inversion of control principles.
- 2004-2013: The Spring ecosystem grows, but with increased complexity in configuration and setup.
- 2014: Spring Boot 1.0 is released, addressing the complexity issues and providing a simpler way to create Spring-based applications.
- 2017: Spring Boot 2.0 is launched, bringing significant improvements and new features.
- 2021: Spring Boot 2.5 and 2.6 versions are released, further enhancing developer productivity and application performance.
- 2022: Spring Boot 3.0 is introduced, with native support for GraalVM native images and other major improvements.
Key Features of Spring Boot
Spring Boot comes packed with features that make it a preferred choice for Java developers. Let’s explore some of its key features:
1. Opinionated Defaults
Spring Boot provides sensible defaults for application configuration, reducing the need for manual setup. This “opinionated” approach allows developers to get started quickly without worrying about intricate configurations.
2. Embedded Server
With Spring Boot, you can run your application as a standalone JAR file, thanks to its embedded server (like Tomcat, Jetty, or Undertow). This eliminates the need for external server deployment and simplifies the development and deployment process.
3. Auto-configuration
Spring Boot automatically configures your application based on the dependencies you’ve added to your project. This intelligent feature saves time and reduces the potential for configuration errors.
4. Starter Dependencies
Spring Boot offers a range of “starter” dependencies that bundle commonly used libraries for specific functionalities. For example, spring-boot-starter-web
includes everything you need for building web applications.
5. Actuator
The Spring Boot Actuator provides built-in endpoints for monitoring and managing your application. It offers insights into application health, metrics, and more, which is crucial for production environments.
6. Easy Testing
Spring Boot supports testing out of the box, providing utilities and annotations to make unit and integration testing straightforward and efficient.
Advantages of Using Spring Boot
The features of Spring Boot translate into several significant advantages for developers and organizations:
1. Rapid Development
With its auto-configuration and starter dependencies, Spring Boot significantly reduces the time required to set up and develop applications. This rapid development cycle allows teams to focus on business logic rather than boilerplate code.
2. Increased Productivity
By eliminating the need for complex configurations and providing a wealth of pre-built integrations, Spring Boot enhances developer productivity. This means faster time-to-market for applications and features.
3. Simplified Dependency Management
Spring Boot’s starter dependencies handle compatibility issues, ensuring that all the libraries in your project work well together. This reduces the time spent on resolving dependency conflicts.
4. Microservices Ready
Spring Boot is designed with microservices architecture in mind. Its lightweight nature and embedded server make it ideal for creating and deploying microservices at scale.
5. Production-Ready Features
With features like the Actuator, Spring Boot provides out-of-the-box support for monitoring, health checks, and metrics, making applications production-ready from the start.
6. Large and Active Community
Being part of the Spring ecosystem, Spring Boot benefits from a large, active community. This means ample resources, regular updates, and quick problem-solving support.
Getting Started with Spring Boot
Now that we understand what Spring Boot is and its benefits, let’s look at how to get started with a simple Spring Boot application:
Step 1: Set Up Your Development Environment
Ensure you have Java Development Kit (JDK) installed on your system. Spring Boot 3.0+ requires Java 17 or later.
Step 2: Create a New Spring Boot Project
You can create a new Spring Boot project using Spring Initializr (https://start.spring.io/) or through your preferred IDE (like IntelliJ IDEA or Eclipse).
Step 3: Add Dependencies
Select the dependencies you need. For a simple web application, you might choose “Spring Web” as your starter dependency.
Step 4: Write Your Application
Here’s a simple “Hello World” Spring Boot application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class HelloWorldApplication {
public static void main(String[] args) {
SpringApplication.run(HelloWorldApplication.class, args);
}
@GetMapping("/")
public String hello() {
return "Hello, Spring Boot!";
}
}
Step 5: Run Your Application
You can run your application directly from your IDE or use the command line:
./mvnw spring-boot:run
Your application will start, and you can access it at http://localhost:8080
.
Core Concepts in Spring Boot
To effectively use Spring Boot, it’s important to understand some core concepts:
1. Dependency Injection (DI)
Spring Boot heavily relies on the principle of Dependency Injection, where objects are given their dependencies instead of creating them internally. This promotes loose coupling and easier testing.
2. Inversion of Control (IoC)
IoC is a design principle where the control flow of a program is inverted: instead of the programmer controlling the flow of a program, the framework controls the flow.
3. Annotations
Spring Boot makes extensive use of annotations to simplify configuration. Some key annotations include:
@SpringBootApplication
: Combines@Configuration
,@EnableAutoConfiguration
, and@ComponentScan
@RestController
: Marks a class as a REST controller@Autowired
: Used for automatic dependency injection@Service
: Indicates that a class is a service layer component@Repository
: Indicates that a class is a data access object
4. Auto-configuration
Spring Boot’s auto-configuration feature automatically configures your application based on the jar dependencies you have added. This reduces the need for explicit configuration.
5. Profiles
Profiles allow you to define different configurations for different environments (e.g., development, testing, production) within the same application.
Best Practices for Spring Boot Development
To make the most of Spring Boot, consider these best practices:
1. Use Spring Boot Starters
Leverage Spring Boot starters to easily add common functionalities to your application without worrying about version compatibility.
2. Externalize Configuration
Use application properties or YAML files to externalize configuration. This makes it easier to change settings without modifying code.
3. Implement Proper Exception Handling
Use Spring Boot’s global exception handling capabilities to manage errors consistently across your application.
4. Utilize Spring Boot Actuator
Implement Actuator endpoints for monitoring and managing your application in production.
5. Write Unit and Integration Tests
Take advantage of Spring Boot’s testing support to write comprehensive tests for your application.
6. Follow RESTful Principles
When building APIs, adhere to RESTful principles for better design and easier consumption of your services.
Spring Boot vs. Traditional Spring Framework
While Spring Boot is built on top of the Spring Framework, there are several key differences:
Feature | Spring Boot | Traditional Spring |
---|---|---|
Configuration | Minimal, auto-configuration | Extensive XML or Java-based configuration |
Setup Time | Quick and easy | More time-consuming |
Deployment | Embedded server, standalone JAR | Typically requires external server |
Boilerplate Code | Minimal | More boilerplate code required |
Learning Curve | Shorter | Steeper |
Real-World Applications of Spring Boot
Spring Boot’s versatility makes it suitable for a wide range of applications:
1. Microservices
Spring Boot’s lightweight nature and embedded server make it ideal for building microservices architectures.
2. RESTful APIs
With its excellent support for building RESTful services, Spring Boot is widely used for creating APIs.
3. Web Applications
From simple web apps to complex enterprise applications, Spring Boot provides the tools and integrations needed for web development.
4. Batch Processing
Spring Boot’s integration with Spring Batch makes it suitable for building robust batch processing applications.
5. Cloud-Native Applications
Spring Boot’s compatibility with cloud platforms and its support for containerization make it an excellent choice for cloud-native development.
The Future of Spring Boot
As we look to the future, Spring Boot continues to evolve and adapt to changing development needs:
1. Enhanced Cloud-Native Support
With the rise of cloud computing, Spring Boot is likely to offer even better integration with cloud platforms and serverless architectures.
2. Improved Performance
Future versions of Spring Boot are expected to focus on improving startup times and reducing memory footprint, especially important for microservices and serverless applications.
3. Better Reactive Programming Support
As reactive programming gains popularity, Spring Boot is likely to enhance its support for building reactive applications.
4. Increased AI and Machine Learning Integration
We may see more built-in support for AI and machine learning libraries, making it easier to incorporate these technologies into Spring Boot applications.
5. Continued Focus on Developer Productivity
Spring Boot will likely continue to introduce features that simplify development and increase productivity, staying true to its core philosophy.
Conclusion
Spring Boot has revolutionized Java development by simplifying the process of building robust, scalable applications. Its auto-configuration, embedded server, and production-ready features make it an invaluable tool for developers of all skill levels. Whether you’re building microservices, web applications, or APIs, Spring Boot provides the foundation you need to create high-quality software efficiently.
As you continue your journey in Java development, mastering Spring Boot will undoubtedly open up new opportunities and enhance your ability to create sophisticated applications. Remember, the key to becoming proficient with Spring Boot is practice and continuous learning. Explore its features, experiment with different configurations, and don’t hesitate to dive into the extensive Spring Boot documentation and community resources.
By understanding and leveraging the power of Spring Boot, you’re not just learning a framework; you’re equipping yourself with a skill set that’s highly valued in the Java ecosystem and the broader tech industry. So, embrace Spring Boot, and take your Java development skills to the next level!