What Is Node.js? A Comprehensive Guide for Beginners
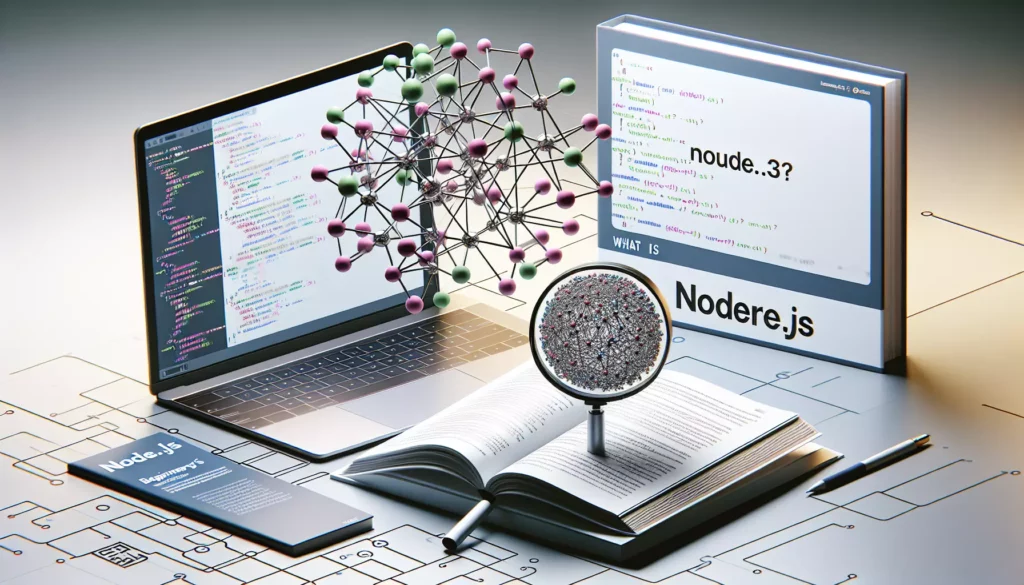
In the ever-evolving world of web development, Node.js has emerged as a powerful and popular technology that has revolutionized how we build server-side applications. Whether you’re a beginner just starting your coding journey or an experienced developer looking to expand your skillset, understanding Node.js is crucial in today’s tech landscape. In this comprehensive guide, we’ll explore what Node.js is, its key features, and why it has become an essential tool for modern web development.
Table of Contents
- What is Node.js?
- A Brief History of Node.js
- How Node.js Works
- Key Features of Node.js
- Common Use Cases for Node.js
- Advantages of Using Node.js
- Potential Disadvantages of Node.js
- Getting Started with Node.js
- The Node.js Ecosystem
- The Future of Node.js
- Conclusion
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment that allows developers to run JavaScript code outside of a web browser. Traditionally, JavaScript was primarily used for client-side scripting in web browsers. However, Node.js extends the capabilities of JavaScript by enabling server-side execution, making it possible to build full-stack applications using a single programming language.
At its core, Node.js is built on Chrome’s V8 JavaScript engine, which is designed for high-performance execution of JavaScript code. This foundation allows Node.js to provide a fast and efficient runtime for server-side applications, making it an excellent choice for building scalable and real-time web applications.
A Brief History of Node.js
Node.js was created by Ryan Dahl in 2009. Dahl’s primary motivation was to develop a system that could handle concurrent connections efficiently. He was inspired by applications like Gmail, which could handle multiple concurrent connections without creating separate threads for each connection.
The first version of Node.js was released in 2009, and it quickly gained popularity among developers due to its innovative approach to server-side JavaScript execution. Over the years, Node.js has evolved significantly, with major version releases introducing new features, performance improvements, and enhanced security measures.
Some key milestones in the history of Node.js include:
- 2009: Initial release of Node.js
- 2011: Introduction of npm (Node Package Manager)
- 2015: Formation of the Node.js Foundation
- 2018: Merger of Node.js Foundation and JS Foundation to form OpenJS Foundation
- 2019: Release of Node.js 12.0 with improved performance and security features
- 2021: Release of Node.js 16.0 with enhanced stability and developer experience
How Node.js Works
To understand how Node.js works, it’s essential to grasp its core architectural principles:
1. Single-threaded Event Loop
Node.js operates on a single-threaded event loop model. This means that all client requests are processed on a single thread, making it highly efficient in handling concurrent connections. The event loop continuously checks for pending events and executes them asynchronously.
2. Non-blocking I/O
Node.js uses non-blocking I/O operations, which means that when a request is made, Node.js doesn’t wait for the operation to complete before moving on to the next task. Instead, it registers a callback function that will be executed when the operation is finished. This approach allows Node.js to handle multiple requests simultaneously without getting blocked by long-running operations.
3. Asynchronous Programming
Asynchronous programming is at the heart of Node.js. It allows developers to write code that can handle multiple operations concurrently without the need for complex multi-threading. This is achieved through the use of callbacks, promises, and async/await syntax.
Here’s a simple example of asynchronous programming in Node.js:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
console.log('This message appears before the file contents');
In this example, the readFile
function is non-blocking, allowing the program to continue executing while the file is being read. The callback function is called once the file reading operation is complete.
Key Features of Node.js
Node.js offers several key features that make it a popular choice for developers:
1. Fast Execution
Built on the V8 JavaScript engine, Node.js provides fast code execution, making it suitable for high-performance applications.
2. NPM (Node Package Manager)
NPM is the largest ecosystem of open-source libraries in the world. It allows developers to easily install, share, and manage package dependencies.
3. Cross-platform Compatibility
Node.js can run on various platforms, including Windows, macOS, and Linux, making it versatile for different development environments.
4. Scalability
The event-driven, non-blocking I/O model of Node.js makes it highly scalable, capable of handling a large number of simultaneous connections with high throughput.
5. Rich Ecosystem
Node.js has a vast ecosystem of libraries and frameworks that can be leveraged to build various types of applications quickly and efficiently.
Common Use Cases for Node.js
Node.js is versatile and can be used for a wide range of applications. Some common use cases include:
1. Web Applications
Node.js is excellent for building web applications, especially those requiring real-time updates or streaming capabilities. Popular frameworks like Express.js make it easy to create robust web applications.
2. API Development
With its ability to handle multiple concurrent connections efficiently, Node.js is well-suited for building RESTful APIs and microservices.
3. Real-time Applications
Node.js excels in building real-time applications like chat systems, gaming servers, or collaborative tools due to its event-driven architecture.
4. Streaming Applications
The non-blocking I/O model of Node.js makes it ideal for building streaming applications, such as video or audio streaming services.
5. Command-line Tools
Node.js can be used to create powerful command-line tools and utilities, leveraging its extensive package ecosystem.
Advantages of Using Node.js
Node.js offers several advantages that have contributed to its widespread adoption:
1. JavaScript Everywhere
With Node.js, developers can use JavaScript for both client-side and server-side programming, reducing the need to switch between different languages and improving code reusability.
2. High Performance
The non-blocking, event-driven architecture of Node.js allows it to handle a large number of concurrent connections efficiently, resulting in high-performance applications.
3. Large and Active Community
Node.js has a vast and active community of developers, which means abundant resources, libraries, and support are available.
4. Rapid Development
The extensive package ecosystem and the ability to reuse code between frontend and backend contribute to faster development cycles.
5. Scalability
Node.js applications are inherently scalable due to their event-driven, non-blocking nature, making it easier to handle increasing loads.
Potential Disadvantages of Node.js
While Node.js has many advantages, it’s important to consider some potential drawbacks:
1. CPU-Intensive Tasks
Node.js may not be the best choice for CPU-intensive tasks, as its single-threaded nature can lead to performance issues in such scenarios.
2. Callback Hell
Asynchronous programming in Node.js can sometimes lead to complex nested callbacks, known as “callback hell,” which can make code harder to read and maintain. However, modern JavaScript features like Promises and async/await help mitigate this issue.
3. Immature Modules
While the npm ecosystem is vast, not all packages are of high quality or well-maintained. Developers need to be cautious when selecting third-party modules.
4. Rapid Evolution
The fast-paced development of Node.js and its ecosystem can sometimes lead to compatibility issues or the need for frequent updates.
Getting Started with Node.js
If you’re new to Node.js, here’s a quick guide to help you get started:
1. Installation
Visit the official Node.js website (https://nodejs.org) and download the appropriate version for your operating system. Follow the installation instructions provided.
2. Verify Installation
Open a terminal or command prompt and type the following commands to verify your installation:
node --version
npm --version
These commands should display the installed versions of Node.js and npm, respectively.
3. Create Your First Node.js Application
Create a new file named app.js
and add the following code:
console.log('Hello, Node.js!');
Run the application by executing the following command in your terminal:
node app.js
You should see the message “Hello, Node.js!” printed in the console.
4. Explore Node.js Modules
Node.js comes with a set of built-in modules that provide essential functionality. Here’s an example using the http
module to create a simple web server:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!');
});
server.listen(3000, () => {
console.log('Server running on http://localhost:3000/');
});
Save this code in a file named server.js
and run it using node server.js
. You can then visit http://localhost:3000
in your web browser to see the “Hello, World!” message.
The Node.js Ecosystem
One of the strengths of Node.js is its vast ecosystem of packages and tools. Here are some popular components of the Node.js ecosystem:
1. Express.js
Express.js is a minimal and flexible web application framework that provides a robust set of features for building web and mobile applications. It’s one of the most popular frameworks in the Node.js ecosystem.
2. Socket.io
Socket.io is a library that enables real-time, bidirectional communication between web clients and servers. It’s commonly used for building real-time applications like chat systems and multiplayer games.
3. Mongoose
Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js. It provides a straightforward, schema-based solution to model your application data.
4. Passport
Passport is an authentication middleware for Node.js that simplifies the process of implementing various authentication strategies in web applications.
5. Jest
Jest is a popular testing framework for JavaScript applications, including Node.js projects. It provides a complete and easy-to-set-up testing solution.
The Future of Node.js
As Node.js continues to evolve, several trends and developments are shaping its future:
1. Improved Performance
Ongoing improvements to the V8 engine and Node.js core are likely to result in even better performance in future versions.
2. Enhanced Security
With the growing importance of cybersecurity, future versions of Node.js are expected to include more robust security features and best practices.
3. Serverless Computing
Node.js is well-suited for serverless architectures, and its role in this area is likely to grow as serverless computing becomes more prevalent.
4. IoT and Edge Computing
Node.js’s lightweight nature makes it a good fit for IoT devices and edge computing scenarios, areas that are expected to see significant growth in the coming years.
5. WebAssembly Integration
As WebAssembly gains traction, Node.js is likely to see improved integration with this technology, opening up new possibilities for high-performance applications.
Conclusion
Node.js has revolutionized server-side development by bringing JavaScript to the backend. Its event-driven, non-blocking I/O model, combined with a rich ecosystem of packages, has made it a popular choice for building scalable and high-performance web applications.
As a beginner, understanding Node.js opens up a world of possibilities in web development. Whether you’re interested in building APIs, real-time applications, or full-stack JavaScript applications, Node.js provides a solid foundation for your journey into modern web development.
As you continue your coding education with AlgoCademy, exploring Node.js and its ecosystem will undoubtedly enhance your skills and prepare you for the challenges of professional software development. Remember that mastering Node.js, like any technology, requires practice and hands-on experience. Start with small projects, experiment with different modules, and gradually build more complex applications to deepen your understanding of this powerful platform.