What is Full Stack Development? Is It Right for You?
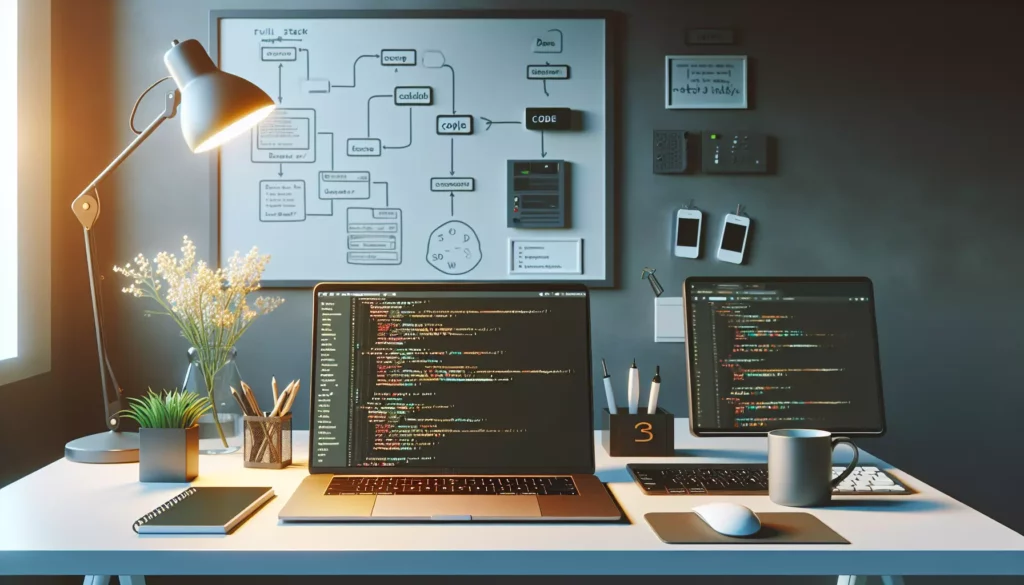
In the ever-evolving world of technology, full stack development has become a buzzword that’s hard to ignore. But what exactly does it mean, and more importantly, is it the right career path for you? This comprehensive guide will delve into the intricacies of full stack development, exploring its definition, required skills, advantages, challenges, and how to determine if it’s the right fit for your career aspirations.
Understanding Full Stack Development
Full stack development refers to the end-to-end application software development, including the front end and back end. A full stack developer is someone who can work on both the client-side and server-side of software development. This means they have the ability to create a complete web application from scratch.
The “Stack” in Full Stack
The term “stack” refers to the different layers involved in the development of a web or mobile application. These layers typically include:
- Front-end (Client-side): This is what users see and interact with directly. It involves HTML, CSS, and JavaScript.
- Back-end (Server-side): This is the behind-the-scenes functionality, including servers, databases, and application logic.
- Database: Where all the data is stored and managed.
A full stack developer is proficient in all these layers and can seamlessly work across them to create a complete application.
Skills Required for Full Stack Development
Becoming a full stack developer requires a diverse skill set. Here are some of the key skills you’ll need to master:
1. Front-end Technologies
- HTML/CSS: The building blocks of web pages
- JavaScript: The programming language of the web
- Front-end Frameworks: Such as React, Angular, or Vue.js
2. Back-end Technologies
- Server-side Languages: Such as Python, Ruby, Java, or Node.js
- Databases: Both SQL (e.g., MySQL, PostgreSQL) and NoSQL (e.g., MongoDB)
- APIs: Creating and consuming RESTful APIs
3. DevOps and Version Control
- Git: For version control and collaboration
- CI/CD: Understanding continuous integration and deployment
- Cloud Platforms: Familiarity with AWS, Google Cloud, or Azure
4. Web Architecture
Understanding how to structure the code, where to store data, which technologies to use, and how different parts of the application will interact with each other.
5. Basic Design Skills
While not required to be a professional designer, having a good eye for design and understanding basic design principles can be beneficial.
Advantages of Being a Full Stack Developer
Full stack development offers several advantages that make it an attractive career path:
1. Versatility
As a full stack developer, you have the ability to work on all aspects of a project. This versatility makes you a valuable asset to any team and increases your employability.
2. Better Problem-Solving Skills
Understanding both front-end and back-end allows you to see the big picture and solve problems more effectively. You can identify issues and implement solutions across the entire stack.
3. Cost-Effective for Employers
Companies, especially startups, often prefer full stack developers as they can handle multiple aspects of development, reducing the need for specialized roles.
4. Continuous Learning
The field of full stack development is always evolving, providing ample opportunities for continuous learning and growth.
5. Career Flexibility
With a broad skill set, you have the flexibility to specialize in a particular area later in your career if you choose to do so.
Challenges of Full Stack Development
While full stack development offers many benefits, it also comes with its own set of challenges:
1. Keeping Up with Technology
The tech world moves fast, and as a full stack developer, you need to stay updated with changes in both front-end and back-end technologies.
2. Breadth vs. Depth
While full stack developers have a broad knowledge base, they may not have the same depth of expertise in specific areas as specialists do.
3. Increased Responsibility
Being responsible for the entire stack can be overwhelming, especially when working on large, complex projects.
4. Potential for Burnout
The need to constantly learn and adapt can lead to burnout if not managed properly.
Is Full Stack Development Right for You?
Determining whether full stack development is the right path for you depends on several factors:
1. Your Learning Style
If you enjoy learning new technologies and don’t mind juggling multiple concepts, full stack development could be a good fit. However, if you prefer to dive deep into a specific area, you might be better suited to a specialized role.
2. Career Goals
Consider your long-term career aspirations. Do you want to work on all aspects of web development, or do you have a particular area you’re passionate about?
3. Problem-Solving Skills
Full stack development requires strong problem-solving skills and the ability to see the big picture. If you enjoy tackling complex problems from multiple angles, this could be a great fit.
4. Adaptability
The tech industry is constantly evolving. If you’re comfortable with change and enjoy learning new things, you’ll likely thrive as a full stack developer.
5. Work Environment Preferences
Full stack developers are often in high demand in startups and small companies where versatility is key. If you enjoy wearing multiple hats and working in fast-paced environments, this could be ideal for you.
How to Become a Full Stack Developer
If you’ve decided that full stack development is the right path for you, here are some steps to get started:
1. Learn the Basics
Start with the fundamentals of web development. Learn HTML, CSS, and JavaScript for the front-end, and choose a back-end language like Python or JavaScript (Node.js).
2. Choose a Stack
There are several popular stacks to choose from, such as:
- MEAN Stack: MongoDB, Express.js, Angular, Node.js
- MERN Stack: MongoDB, Express.js, React, Node.js
- Python-Django Stack: Python, Django, MySQL
Choose one that aligns with your interests and career goals.
3. Practice, Practice, Practice
Build projects to apply your knowledge. Start with simple applications and gradually increase complexity as you learn.
4. Learn Version Control
Git is an essential tool for developers. Learn how to use Git and GitHub for version control and collaboration.
5. Understand Databases
Learn both relational (SQL) and non-relational (NoSQL) databases. Understand when to use each type.
6. Explore DevOps
Familiarize yourself with basic DevOps concepts and tools. Learn about CI/CD pipelines and cloud platforms.
7. Keep Learning
The learning never stops in full stack development. Stay updated with the latest trends and technologies through online courses, tutorials, and tech blogs.
Full Stack Development in Practice: A Simple Example
To give you a taste of what full stack development looks like in practice, let’s walk through a simple example of creating a basic web application that allows users to add and view items in a to-do list.
Front-end (HTML, CSS, JavaScript)
First, we’ll create a simple HTML structure with CSS for styling and JavaScript for interactivity:
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>To-Do List</title>
<style>
body { font-family: Arial, sans-serif; }
#todo-list { margin-top: 20px; }
</style>
</head>
<body>
<h1>My To-Do List</h1>
<input type="text" id="todo-input" placeholder="Enter a new task">
<button onclick="addTodo()">Add</button>
<ul id="todo-list"></ul>
<script>
function addTodo() {
const input = document.getElementById('todo-input');
const list = document.getElementById('todo-list');
if (input.value !== '') {
const li = document.createElement('li');
li.textContent = input.value;
list.appendChild(li);
input.value = '';
// Here we would typically send this data to the server
}
}
</script>
</body>
</html>
Back-end (Node.js with Express)
Now, let’s create a simple server using Node.js and Express to handle our to-do list data:
// server.js
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
const port = 3000;
app.use(bodyParser.json());
let todos = [];
app.get('/todos', (req, res) => {
res.json(todos);
});
app.post('/todos', (req, res) => {
const newTodo = req.body.todo;
todos.push(newTodo);
res.json({ message: 'Todo added successfully' });
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Database (MongoDB)
For persistence, we can add a MongoDB database to our application:
// server.js (updated with MongoDB)
const express = require('express');
const bodyParser = require('body-parser');
const MongoClient = require('mongodb').MongoClient;
const app = express();
const port = 3000;
app.use(bodyParser.json());
let db;
MongoClient.connect('mongodb://localhost:27017', (err, client) => {
if (err) return console.error(err);
console.log('Connected to Database');
db = client.db('todoapp');
});
app.get('/todos', (req, res) => {
db.collection('todos').find().toArray((err, result) => {
if (err) return console.error(err);
res.json(result);
});
});
app.post('/todos', (req, res) => {
db.collection('todos').insertOne(req.body, (err, result) => {
if (err) return console.error(err);
res.json({ message: 'Todo added successfully' });
});
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
This example demonstrates how a full stack developer works across different layers of an application. They create the user interface (front-end), set up the server to handle requests and business logic (back-end), and manage data persistence (database).
Conclusion: Is Full Stack Development Right for You?
Full stack development offers a challenging and rewarding career path for those who enjoy working with various technologies and seeing projects through from start to finish. It requires a broad skill set, continuous learning, and the ability to adapt to new technologies quickly.
If you’re someone who:
- Enjoys learning new technologies
- Likes to see the big picture in projects
- Is comfortable with both creative (front-end) and logical (back-end) tasks
- Thrives in dynamic work environments
- Enjoys problem-solving across different domains
Then full stack development could be an excellent fit for you.
However, if you prefer to specialize deeply in one area or find the prospect of constantly learning new technologies overwhelming, you might be better suited to a more specialized role in software development.
Ultimately, the decision to pursue full stack development should align with your personal interests, learning style, and career goals. Whether you choose to become a full stack developer or specialize in a particular area, the key is to stay curious, keep learning, and enjoy the process of creating technology that impacts people’s lives.
Remember, in the world of software development, your journey is unique. Full stack development is just one of many exciting paths you can take. The most important thing is to find the role that allows you to do your best work and brings you satisfaction in your career.