What Is Express.js? A Comprehensive Guide for Web Developers
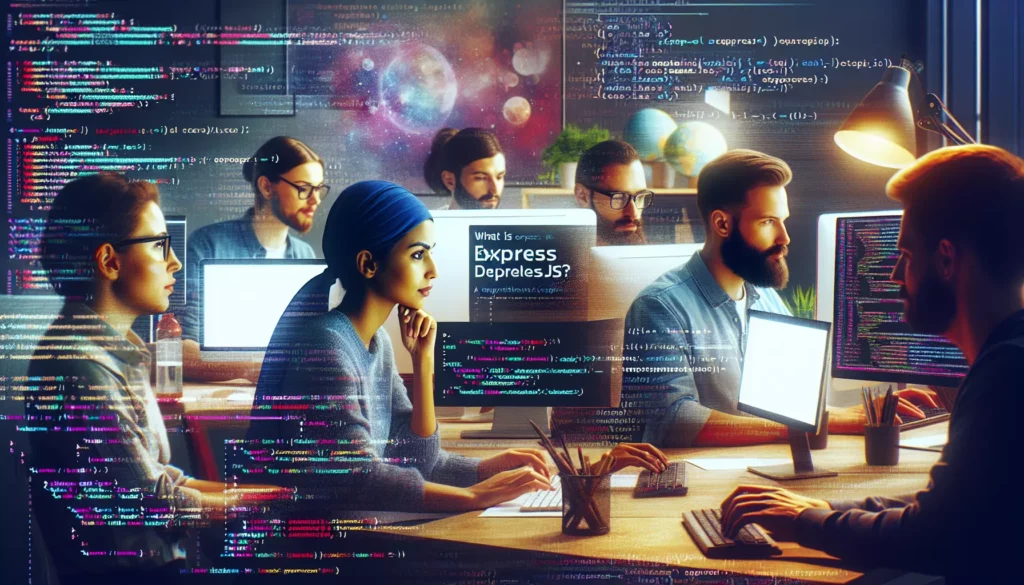
In the ever-evolving landscape of web development, having the right tools at your disposal can make all the difference in creating efficient, scalable, and robust web applications. One such tool that has gained immense popularity among developers is Express.js. If you’re looking to enhance your web development skills and streamline your backend processes, understanding Express.js is crucial. In this comprehensive guide, we’ll dive deep into what Express.js is, its features, benefits, and how it can elevate your web development projects.
Table of Contents
- What is Express.js?
- History and Evolution of Express.js
- Key Features of Express.js
- Why Use Express.js?
- Getting Started with Express.js
- Core Concepts in Express.js
- Understanding Middleware in Express.js
- Routing in Express.js
- Working with Template Engines
- Database Integration
- Best Practices and Tips
- Express.js vs. Alternatives
- Real-World Applications of Express.js
- The Future of Express.js
- Conclusion
What is Express.js?
Express.js, often simply referred to as Express, is a minimal and flexible Node.js web application framework that provides a robust set of features for building single-page, multi-page, and hybrid web applications. It is designed to make the process of building web applications and APIs simpler and more streamlined.
At its core, Express.js is a layer built on top of Node.js that helps manage servers and routes. It provides a thin layer of fundamental web application features, without obscuring Node.js features that you know and love. This “unopinionated” nature of Express means that it doesn’t impose a specific structure or paradigm on your application, giving you the freedom to organize your code as you see fit.
History and Evolution of Express.js
Express.js was created by TJ Holowaychuk and released in November 2010. It quickly gained popularity due to its simplicity and flexibility. Over the years, it has evolved to become one of the most widely used web application frameworks for Node.js.
Key milestones in Express.js history:
- 2010: Initial release
- 2014: Express 4.0 released, introducing significant changes and improvements
- 2015: The Express project joined the Node.js Foundation as an incubator project
- 2016: Express 5.0 alpha released, promising further enhancements
Throughout its evolution, Express.js has maintained its core philosophy of being minimalist and flexible, while continuously improving its performance and feature set.
Key Features of Express.js
Express.js comes packed with a variety of features that make it a popular choice among developers. Here are some of its key features:
- Routing: Express provides a robust routing mechanism to define routes for your application.
- Middleware: It supports middleware to process requests before they reach the route handlers.
- Template Engines: Express can be integrated with various template engines like EJS, Pug, and Handlebars.
- Static File Serving: It offers built-in middleware for serving static files.
- Error Handling: Express includes a built-in error handler that takes care of any errors that might occur in the app.
- HTTP Utility Methods: It provides utility methods for HTTP verb-based routing (get, post, put, etc.).
- RESTful API Support: Express makes it easy to create RESTful APIs.
- Performance: It’s designed to be fast and efficient, with a minimal overhead.
Why Use Express.js?
There are several compelling reasons to choose Express.js for your web development projects:
- Simplicity: Express.js has a gentle learning curve, making it accessible for beginners while still being powerful enough for experienced developers.
- Flexibility: Its unopinionated nature allows developers to structure their applications as they see fit.
- Large Community: Express has a vast and active community, which means plenty of resources, plugins, and support are available.
- Middleware Ecosystem: There’s a rich ecosystem of middleware packages that can be easily integrated to add functionality to your application.
- Performance: Express is known for its high performance and low overhead.
- Integration: It integrates well with various databases, template engines, and other Node.js packages.
- Scalability: Express applications can be easily scaled to handle increased load.
Getting Started with Express.js
To start using Express.js, you’ll need to have Node.js installed on your system. Once you have Node.js, you can create a new Express.js project by following these steps:
- Create a new directory for your project:
mkdir my-express-app cd my-express-app
- Initialize a new Node.js project:
npm init -y
- Install Express:
npm install express
- Create a new file named
app.js
and add the following code:const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello World!'); }); app.listen(port, () => { console.log(`Example app listening at http://localhost:${port}`); });
- Run your application:
node app.js
Now, if you open a browser and navigate to http://localhost:3000
, you should see “Hello World!” displayed.
Core Concepts in Express.js
To effectively use Express.js, it’s important to understand its core concepts:
- Application Object: The
express()
function creates an Express application. This object, conventionally namedapp
, has methods for routing HTTP requests, configuring middleware, rendering HTML views, registering a template engine, and modifying application settings. - Request Object: The
req
object represents the HTTP request and has properties for the request query string, parameters, body, HTTP headers, and so on. - Response Object: The
res
object represents the HTTP response that an Express app sends when it gets an HTTP request. - Routing: Refers to how an application’s endpoints (URIs) respond to client requests. You define routing using methods of the Express app object that correspond to HTTP methods.
- Middleware: Functions that have access to the request object, the response object, and the next middleware function in the application’s request-response cycle.
Understanding Middleware in Express.js
Middleware functions are functions that have access to the request object (req
), the response object (res
), and the next middleware function in the application’s request-response cycle, commonly denoted by a variable named next
.
Middleware functions can:
- Execute any code.
- Make changes to the request and response objects.
- End the request-response cycle.
- Call the next middleware in the stack.
Here’s an example of a simple middleware function:
app.use((req, res, next) => {
console.log('Time:', Date.now());
next();
});
This middleware function will be executed for every request to the app. It logs the current timestamp and then calls next()
to pass control to the next middleware function.
Routing in Express.js
Routing refers to determining how an application responds to a client request to a particular endpoint, which is a URI (or path) and a specific HTTP request method (GET, POST, etc.).
Basic route structure:
app.METHOD(PATH, HANDLER)
Where:
app
is an instance of express.METHOD
is an HTTP request method, in lowercase.PATH
is a path on the server.HANDLER
is the function executed when the route is matched.
Example of different routes:
// GET method route
app.get('/', (req, res) => {
res.send('GET request to the homepage');
});
// POST method route
app.post('/', (req, res) => {
res.send('POST request to the homepage');
});
// Route parameters
app.get('/users/:userId', (req, res) => {
res.send(`User ID: ${req.params.userId}`);
});
Working with Template Engines
Template engines allow you to use static template files in your application. At runtime, the template engine replaces variables in a template file with actual values, and transforms the template into an HTML file sent to the client.
Express supports many template engines. Some popular ones include:
- EJS
- Pug
- Handlebars
To use a template engine with Express:
- Install the template engine:
npm install ejs
- Set the template engine in your Express app:
app.set('view engine', 'ejs');
- Create a views directory and add your .ejs files there.
- Render a view in your route:
app.get('/', (req, res) => { res.render('index', { title: 'Welcome' }); });
Database Integration
Express can be integrated with various databases, both SQL and NoSQL. Some popular choices include:
- MongoDB (using Mongoose ODM)
- PostgreSQL (using node-postgres)
- MySQL (using mysql2)
- SQLite (using sqlite3)
Here’s a basic example of integrating MongoDB with Express using Mongoose:
const mongoose = require('mongoose');
const express = require('express');
const app = express();
mongoose.connect('mongodb://localhost/myapp', { useNewUrlParser: true, useUnifiedTopology: true });
const User = mongoose.model('User', { name: String, email: String });
app.post('/users', async (req, res) => {
const user = new User(req.body);
await user.save();
res.send(user);
});
Best Practices and Tips
When working with Express.js, keep these best practices in mind:
- Use Async/Await: For handling asynchronous operations, use async/await instead of callbacks for cleaner and more readable code.
- Implement proper error handling: Use try-catch blocks and create a centralized error handling middleware.
- Use environment variables: Store configuration in the environment separate from code.
- Implement security best practices: Use helmet middleware to set various HTTP headers for app security.
- Use compression: Implement response compression for faster page loading.
- Implement logging: Use a logging library like Winston or Morgan for better debugging and monitoring.
- Organize your code: Structure your application using the MVC (Model-View-Controller) pattern or a similar architecture.
Express.js vs. Alternatives
While Express.js is popular, it’s not the only Node.js framework available. Here’s how it compares to some alternatives:
- Koa: Created by the same team behind Express, Koa aims to be a smaller, more expressive, and more robust foundation for web applications and APIs. It’s more modular than Express.
- Hapi: A powerful framework for building applications and services. It’s known for its plugin system and is often used for large-scale projects.
- Nest.js: A progressive Node.js framework for building efficient and scalable server-side applications. It uses TypeScript and is heavily inspired by Angular.
- Sails.js: A web framework that makes it easy to build custom, enterprise-grade Node.js apps. It’s more opinionated than Express and includes an ORM out of the box.
Express.js stands out for its simplicity, flexibility, and large community support. It’s an excellent choice for developers who want more control over their application structure and prefer a minimalist framework.
Real-World Applications of Express.js
Express.js is used by many large companies and popular applications. Some notable examples include:
- MySpace: The social networking site uses Express.js in its tech stack.
- Uber: Parts of Uber’s backend services are built with Express.
- IBM: Uses Express in some of its cloud services.
- Fox Sports: Their website is powered by Express.
- Paypal: Uses Express in parts of its payment system.
These examples demonstrate the scalability and reliability of Express.js in handling high-traffic, complex applications.
The Future of Express.js
Express.js continues to evolve and adapt to the changing landscape of web development. Some trends and future directions for Express include:
- TypeScript Support: Increasing adoption of TypeScript in the Node.js ecosystem is likely to influence Express.js development.
- Performance Improvements: Ongoing efforts to optimize performance and reduce overhead.
- Enhanced Security Features: As web security becomes increasingly important, Express is likely to incorporate more built-in security features.
- Better Async Support: Improved handling of asynchronous operations, possibly with built-in support for async/await.
- Microservices Architecture: Express may evolve to better support microservices architectures, which are becoming increasingly popular.
Conclusion
Express.js has established itself as a cornerstone in the world of Node.js web development. Its simplicity, flexibility, and robust feature set make it an excellent choice for both beginners and experienced developers. Whether you’re building a small personal project or a large-scale enterprise application, Express.js provides the tools and ecosystem to bring your ideas to life.
As you continue your journey in web development, mastering Express.js will undoubtedly be a valuable skill. It not only opens up numerous job opportunities but also provides a solid foundation for understanding backend development concepts that are applicable across various frameworks and languages.
Remember, the best way to learn Express.js is by doing. Start with small projects, experiment with different features, and gradually build more complex applications. With practice and persistence, you’ll soon be creating robust, efficient web applications with Express.js.
Happy coding!