What is DevOps, and How Does It Relate to Software Development?
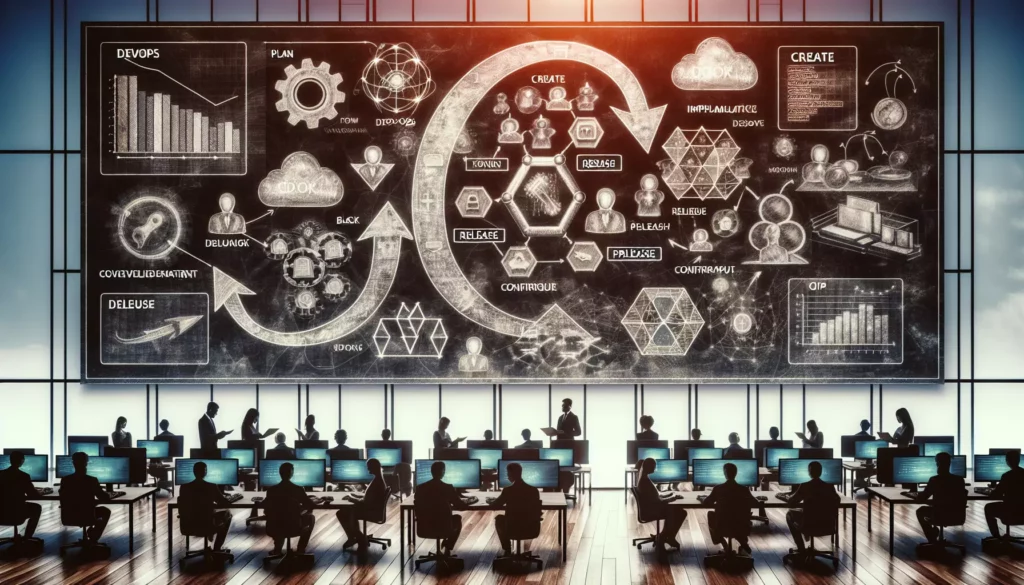
In the ever-evolving landscape of software development, DevOps has emerged as a crucial methodology that bridges the gap between development and operations teams. As coding education platforms like AlgoCademy focus on nurturing programming skills and preparing individuals for technical interviews, understanding DevOps becomes increasingly important for aspiring developers. In this comprehensive guide, we’ll explore what DevOps is, its relationship to software development, and why it’s a valuable skill set for programmers at all levels.
Understanding DevOps: The Basics
DevOps, a portmanteau of “Development” and “Operations,” is a set of practices that combines software development (Dev) with IT operations (Ops). It aims to shorten the systems development life cycle and provide continuous delivery with high software quality. DevOps is complementary to Agile software development; several DevOps aspects came from the Agile methodology.
Key Principles of DevOps
- Collaboration: Breaking down silos between development and operations teams.
- Automation: Streamlining repetitive tasks to increase efficiency and reduce errors.
- Continuous Integration and Continuous Delivery (CI/CD): Regularly merging code changes and deploying to production.
- Monitoring and Feedback: Constantly measuring performance and gathering user feedback.
- Iterative Improvement: Continuously refining processes and systems based on feedback and metrics.
The Relationship Between DevOps and Software Development
DevOps is not a replacement for traditional software development methodologies but rather an enhancement that focuses on the entire software delivery pipeline. Here’s how DevOps relates to and impacts various aspects of software development:
1. Streamlined Development Process
DevOps practices encourage developers to think beyond just writing code. They need to consider how their code will be deployed, maintained, and scaled in production environments. This holistic approach leads to more robust and maintainable software.
2. Faster Time-to-Market
By automating many aspects of the development and deployment process, DevOps enables teams to release new features and updates more frequently. This agility is crucial in today’s fast-paced tech landscape.
3. Improved Collaboration
DevOps breaks down the traditional barriers between developers, operations teams, and even quality assurance. This increased collaboration leads to better communication, shared responsibilities, and a more efficient workflow.
4. Enhanced Quality and Reliability
Through practices like continuous integration and automated testing, DevOps helps catch and resolve issues earlier in the development cycle. This results in higher quality software and more reliable deployments.
5. Scalability and Performance Focus
DevOps practices often involve cloud technologies and containerization, which allow for easier scaling of applications. This focus on scalability and performance is crucial for modern, high-traffic applications.
DevOps Tools and Technologies
To implement DevOps practices effectively, teams rely on a variety of tools and technologies. Here are some key categories and examples:
Version Control
- Git
- GitHub
- GitLab
- Bitbucket
Continuous Integration/Continuous Deployment (CI/CD)
- Jenkins
- GitLab CI
- Travis CI
- CircleCI
Configuration Management
- Ansible
- Puppet
- Chef
Containerization and Orchestration
- Docker
- Kubernetes
Monitoring and Logging
- Prometheus
- Grafana
- ELK Stack (Elasticsearch, Logstash, Kibana)
Implementing DevOps in Software Development
Adopting DevOps practices in a software development workflow involves several key steps:
1. Establishing a DevOps Culture
The first step is to foster a culture of collaboration and shared responsibility. This often requires breaking down existing silos and encouraging open communication between different teams.
2. Automating the Build and Deploy Process
Implement CI/CD pipelines to automate the process of building, testing, and deploying code. This might involve setting up a Jenkins server or using a cloud-based CI/CD service.
3. Implementing Infrastructure as Code (IaC)
Use tools like Terraform or CloudFormation to define and manage infrastructure through code. This allows for version-controlled, reproducible infrastructure setups.
4. Containerizing Applications
Adopt containerization technologies like Docker to ensure consistency across different environments and simplify deployment processes.
5. Setting Up Monitoring and Logging
Implement comprehensive monitoring and logging solutions to gain visibility into application performance and quickly identify and resolve issues.
DevOps Best Practices for Developers
As a developer, incorporating DevOps principles into your workflow can greatly enhance your effectiveness and the quality of your code. Here are some best practices to consider:
1. Embrace Version Control
Use Git or another version control system for all your projects. This allows for better collaboration, code review processes, and the ability to roll back changes if needed.
2. Write Testable Code
Design your code with testing in mind. Write unit tests, integration tests, and end-to-end tests to ensure your code behaves as expected.
3. Automate Everything Possible
Look for opportunities to automate repetitive tasks, from code linting to deployment processes. This saves time and reduces the chance of human error.
4. Practice Continuous Integration
Regularly merge your code changes into a central repository and run automated tests. This helps catch integration issues early.
5. Monitor Your Applications
Implement logging and monitoring in your applications. This will help you understand how your code performs in production and quickly identify issues.
DevOps and Coding Education
As platforms like AlgoCademy focus on developing coding skills and preparing individuals for technical interviews, understanding DevOps principles becomes increasingly valuable. Here’s why:
1. Holistic Understanding of Software Development
DevOps knowledge provides a more complete picture of the software development lifecycle, beyond just writing code.
2. Improved Collaboration Skills
DevOps emphasizes teamwork and communication, which are crucial skills in any development role.
3. Increased Employability
Many companies now look for DevOps skills in addition to core programming abilities when hiring developers.
4. Better Problem-Solving Abilities
DevOps practices often involve troubleshooting complex systems, which can enhance overall problem-solving skills.
Implementing DevOps Concepts in Coding Practice
Even as a beginner or intermediate programmer, you can start incorporating DevOps concepts into your coding practice. Here are some ways to do this:
1. Use Version Control for Personal Projects
Start using Git for all your projects, even personal ones. This will help you become comfortable with version control workflows.
2. Set Up a CI/CD Pipeline for a Personal Project
Use a free CI/CD service like GitHub Actions to automatically run tests and deploy your code. Here’s a simple example of a GitHub Actions workflow:
name: CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.8
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m unittest discover tests
3. Experiment with Containerization
Try containerizing one of your applications using Docker. Here’s a simple Dockerfile for a Python application:
FROM python:3.8-slim-buster
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip3 install -r requirements.txt
COPY . .
CMD [ "python3", "app.py" ]
4. Implement Logging in Your Applications
Start adding meaningful log statements to your code. In Python, you can use the built-in logging module:
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def some_function():
logger.info("Starting some_function")
# Function logic here
logger.info("Finished some_function")
Conclusion
DevOps is more than just a set of practices; it’s a philosophy that aims to improve the entire software development lifecycle. By breaking down silos, automating processes, and fostering a culture of collaboration, DevOps enables teams to deliver high-quality software more rapidly and reliably.
For aspiring developers focusing on coding education and interview preparation, understanding DevOps principles can provide a significant advantage. It offers a broader perspective on software development, enhances problem-solving skills, and increases employability in an increasingly competitive job market.
As you continue your journey in software development, whether through platforms like AlgoCademy or personal projects, consider incorporating DevOps practices into your workflow. Start small by using version control, implementing automated tests, or experimenting with containerization. Over time, these practices will become second nature, making you a more well-rounded and effective developer.
Remember, DevOps is not just for operations teams or senior developers. It’s a mindset and a set of skills that can benefit developers at all levels. By embracing DevOps principles early in your career, you’ll be well-positioned to tackle the challenges of modern software development and contribute effectively to any development team.