What is Continuous Integration/Continuous Deployment (CI/CD)? A Comprehensive Guide
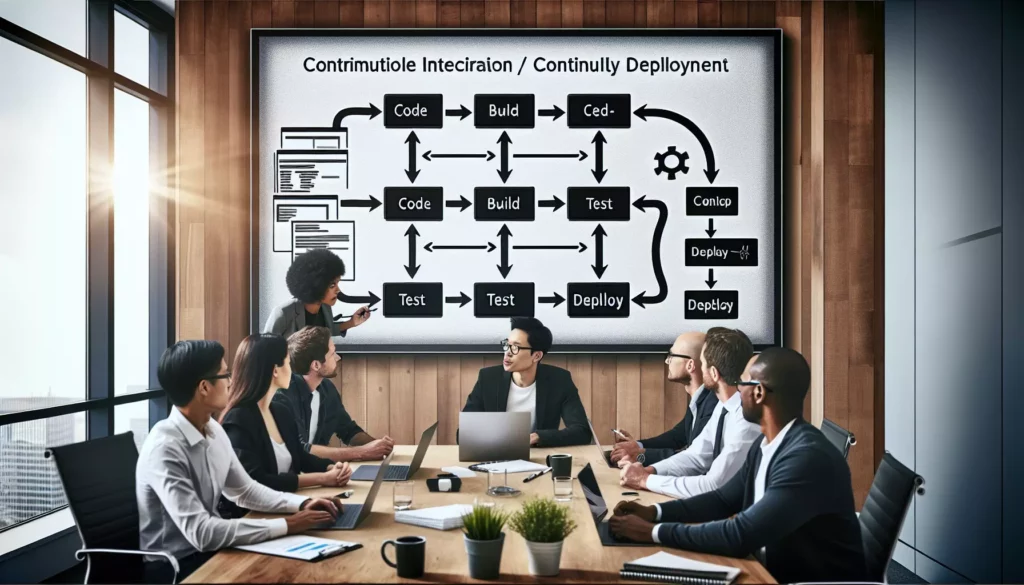
In the fast-paced world of software development, efficiency and reliability are paramount. As projects grow in complexity and teams become more distributed, the need for streamlined processes becomes increasingly critical. This is where Continuous Integration/Continuous Deployment (CI/CD) comes into play. In this comprehensive guide, we’ll explore the ins and outs of CI/CD, its benefits, implementation strategies, and how it can revolutionize your development workflow.
Table of Contents
- Understanding CI/CD
- Continuous Integration (CI)
- Continuous Deployment (CD)
- Benefits of CI/CD
- Implementing CI/CD
- Popular CI/CD Tools
- Best Practices for CI/CD
- Common Challenges and Solutions
- CI/CD in Action: Real-World Examples
- The Future of CI/CD
- Conclusion
Understanding CI/CD
Continuous Integration/Continuous Deployment (CI/CD) is a set of practices and principles in software development that aims to improve the speed, quality, and reliability of software delivery. It combines two interconnected concepts:
- Continuous Integration (CI): The practice of frequently merging code changes into a central repository, followed by automated builds and tests.
- Continuous Deployment (CD): The automatic deployment of code changes to production or staging environments after passing all tests in the CI pipeline.
Together, these practices form a pipeline that automates the software delivery process from code commit to production deployment. Let’s dive deeper into each component.
Continuous Integration (CI)
Continuous Integration is the foundation of the CI/CD pipeline. It involves developers regularly merging their code changes into a central repository, typically several times a day. Each integration triggers an automated build and test process, which helps detect and address integration issues early in the development cycle.
Key Components of CI:
- Version Control System: A central repository (e.g., Git) where developers push their code changes.
- Automated Build: A process that compiles the code, runs static code analysis, and creates deployable artifacts.
- Automated Testing: Running unit tests, integration tests, and other automated checks to ensure code quality and functionality.
- Feedback Mechanism: Notifying developers of build and test results, typically through email or chat notifications.
Benefits of CI:
- Early detection of integration issues
- Reduced time spent on debugging
- Improved code quality
- Faster development cycles
- Increased collaboration among team members
Example CI Workflow:
1. Developer writes code and commits changes to a feature branch
2. Developer opens a pull request to merge changes into the main branch
3. CI system detects the pull request and triggers an automated build
4. Automated tests run on the built code
5. CI system reports results back to the pull request
6. If all tests pass, the code can be reviewed and merged
7. Once merged, another CI build is triggered on the main branch
Continuous Deployment (CD)
Continuous Deployment takes the CI process a step further by automatically deploying code changes to production or staging environments after passing all tests in the CI pipeline. This practice aims to reduce the time between writing code and making it available to users.
Key Components of CD:
- Deployment Pipeline: A series of stages that code changes must pass through before reaching production.
- Environment Management: Provisioning and configuring various environments (development, staging, production) for deployment.
- Release Automation: Tools and scripts that automate the deployment process to different environments.
- Monitoring and Rollback: Systems to monitor application performance and quickly roll back changes if issues arise.
Benefits of CD:
- Faster time-to-market for new features
- Reduced risk in deployments
- Increased reliability and stability of releases
- Improved customer satisfaction through rapid feature delivery
- Reduced manual intervention in the deployment process
Example CD Workflow:
1. Code passes all CI tests on the main branch
2. CD system automatically deploys to a staging environment
3. Additional tests (e.g., integration, performance) run in staging
4. If staging tests pass, the code is automatically deployed to production
5. Post-deployment tests and monitoring ensure stability
6. If issues arise, automatic rollback procedures are triggered
Benefits of CI/CD
Implementing CI/CD practices in your development workflow can bring numerous benefits to your team and organization:
- Faster Time-to-Market: By automating the build, test, and deployment processes, new features and bug fixes can be delivered to users more quickly.
- Improved Code Quality: Regular integration and automated testing help catch and fix bugs early in the development cycle.
- Reduced Risk: Smaller, more frequent releases minimize the risk associated with large, infrequent deployments.
- Increased Collaboration: CI/CD encourages developers to work together more closely and share code more frequently.
- Better Visibility: Automated pipelines provide clear insight into the state of the codebase and deployment status.
- Continuous Feedback: Rapid deployment allows for quick user feedback, enabling faster iteration and improvement.
- Cost Reduction: Automation reduces the need for manual intervention, saving time and resources.
- Improved Developer Productivity: Developers can focus on writing code rather than dealing with integration and deployment issues.
Implementing CI/CD
Implementing a CI/CD pipeline requires careful planning and execution. Here’s a step-by-step guide to get you started:
- Assess Your Current Workflow: Evaluate your existing development and deployment processes to identify areas for improvement.
- Choose Your Tools: Select appropriate CI/CD tools that integrate well with your existing tech stack (more on this in the next section).
- Set Up Version Control: Ensure all your code is in a version control system like Git.
- Implement Automated Testing: Develop a comprehensive suite of automated tests, including unit tests, integration tests, and end-to-end tests.
- Create Build Automation: Set up scripts to automate your build process, including compiling code and creating deployable artifacts.
- Configure CI Pipeline: Set up your chosen CI tool to trigger builds and run tests automatically on code commits.
- Implement Continuous Deployment: Automate the deployment process to your various environments (staging, production).
- Set Up Monitoring and Alerts: Implement monitoring tools to track application performance and set up alerts for any issues.
- Train Your Team: Ensure all team members understand the new CI/CD processes and tools.
- Iterate and Improve: Continuously evaluate and refine your CI/CD pipeline based on feedback and changing needs.
Popular CI/CD Tools
There are numerous tools available to help implement CI/CD pipelines. Here are some popular options:
- Jenkins: An open-source automation server that supports building, deploying, and automating any project.
- GitLab CI/CD: Integrated CI/CD functionality within the GitLab version control platform.
- Travis CI: A hosted CI service that integrates easily with GitHub repositories.
- CircleCI: A cloud-based CI/CD platform that supports rapid software development and deployment.
- GitHub Actions: GitHub’s built-in CI/CD solution that allows automation directly from GitHub repositories.
- TeamCity: A CI server from JetBrains that provides out-of-the-box continuous integration.
- Bamboo: Atlassian’s CI/CD server that ties automated builds, tests, and releases together in a single workflow.
- Azure DevOps: Microsoft’s suite of DevOps tools, including Azure Pipelines for CI/CD.
When choosing a CI/CD tool, consider factors such as ease of use, integration capabilities, scalability, and cost.
Best Practices for CI/CD
To get the most out of your CI/CD implementation, follow these best practices:
- Commit Code Frequently: Encourage developers to push small, incremental changes often to catch integration issues early.
- Maintain a Comprehensive Test Suite: Ensure your automated tests cover a wide range of scenarios to catch potential issues.
- Keep the Build Fast: Aim for quick feedback by optimizing your build and test processes.
- Use Feature Flags: Implement feature flags to control the rollout of new features without blocking deployments.
- Implement Code Reviews: Use pull requests and code reviews to maintain code quality before merging.
- Automate Everything: Strive to automate as much of the software delivery process as possible.
- Use Consistent Environments: Ensure development, staging, and production environments are as similar as possible.
- Monitor and Log: Implement robust monitoring and logging to quickly identify and resolve issues in production.
- Practice Infrastructure as Code: Use tools like Terraform or CloudFormation to version and automate your infrastructure setup.
- Implement Security Scans: Include security vulnerability scans in your CI/CD pipeline to catch potential security issues early.
Common Challenges and Solutions
While CI/CD offers numerous benefits, implementing and maintaining it can present challenges. Here are some common issues and their solutions:
1. Slow Build Times
Challenge: As projects grow, build times can increase, slowing down the feedback loop.
Solution: Optimize build processes, use build caching, parallelize tests, and consider distributed builds.
2. Flaky Tests
Challenge: Inconsistent test results can erode trust in the CI/CD pipeline.
Solution: Identify and fix flaky tests, use retry mechanisms for transient failures, and quarantine unreliable tests.
3. Environment Inconsistencies
Challenge: Differences between development, staging, and production environments can lead to unexpected issues.
Solution: Use containerization (e.g., Docker) and infrastructure as code to ensure consistency across environments.
4. Resistance to Change
Challenge: Team members may resist adopting new CI/CD practices.
Solution: Provide training, demonstrate the benefits, and gradually introduce changes to build confidence.
5. Managing Secrets and Credentials
Challenge: Securely managing sensitive information in automated pipelines can be difficult.
Solution: Use secret management tools, encrypt sensitive data, and limit access to production credentials.
CI/CD in Action: Real-World Examples
To better understand the impact of CI/CD, let’s look at how some well-known companies have implemented these practices:
1. Amazon
Amazon is known for its ability to deploy code to production every 11.6 seconds on average. They achieve this through extensive automation and a microservices architecture that allows for independent deployments of different components.
2. Netflix
Netflix uses a custom-built CI/CD platform called Spinnaker, which allows them to manage multi-region deployments across their cloud infrastructure. This enables them to roll out updates to millions of users seamlessly.
3. Etsy
Etsy went from deploying twice a week to over 50 times a day by implementing CI/CD practices. They use feature flags extensively to control the rollout of new features and quickly roll back if issues arise.
4. Google
Google’s CI/CD practices involve extensive automated testing, with each code change going through over 4 million tests before being deployed. This allows them to maintain high quality across their vast codebase.
The Future of CI/CD
As technology continues to evolve, so too will CI/CD practices. Here are some trends shaping the future of CI/CD:
- AI and Machine Learning Integration: AI-powered tools will help optimize build processes, predict test failures, and automate code reviews.
- Serverless CI/CD: Serverless architectures will enable more scalable and cost-effective CI/CD pipelines.
- GitOps: The practice of using Git as the single source of truth for declarative infrastructure and applications.
- Shift-Left Security: Integrating security practices earlier in the development process, including automated security testing in CI/CD pipelines.
- Progressive Delivery: Advanced deployment techniques like canary releases and A/B testing will become more integrated into CD processes.
Conclusion
Continuous Integration/Continuous Deployment (CI/CD) has revolutionized the way software is developed and delivered. By automating the build, test, and deployment processes, CI/CD enables teams to deliver high-quality software faster and more reliably. While implementing CI/CD can be challenging, the benefits in terms of productivity, quality, and customer satisfaction make it a worthwhile investment for any software development team.
As you embark on your CI/CD journey, remember that it’s not just about tools and processes—it’s about fostering a culture of continuous improvement and collaboration. Start small, iterate often, and continuously refine your approach based on your team’s needs and feedback.
Whether you’re a seasoned developer or just starting your coding journey, understanding and implementing CI/CD practices will be invaluable in today’s fast-paced software development landscape. As you continue to learn and grow in your programming skills, consider how CI/CD can be integrated into your projects to streamline your workflow and improve the quality of your code.
Happy coding, and may your deployments be ever smooth and frequent!