What Is A Web App? A Comprehensive Guide for Aspiring Developers
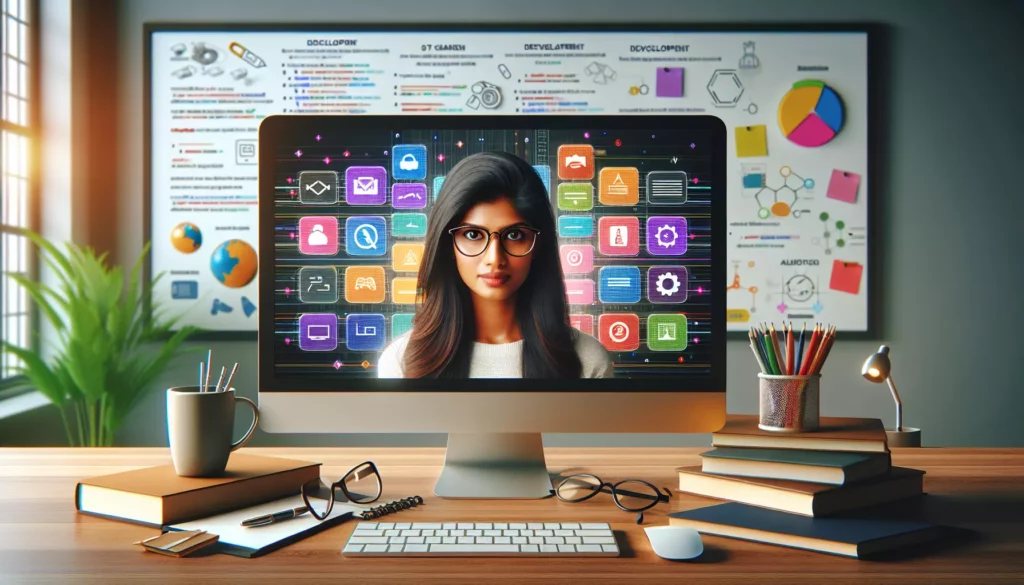
In today’s digital age, web applications (web apps) have become an integral part of our online experience. Whether you’re checking your email, scrolling through social media, or managing your finances online, chances are you’re interacting with a web app. But what exactly is a web app, and how does it differ from a traditional website? In this comprehensive guide, we’ll explore the world of web apps, their characteristics, and why they’re essential for modern developers to understand.
Understanding Web Applications
A web application, often abbreviated as “web app,” is a software program that runs on a web server and is accessed through a web browser. Unlike traditional desktop applications that need to be installed on a user’s computer, web apps can be used from any device with an internet connection and a compatible web browser.
Web apps are dynamic, interactive, and often provide a more app-like experience compared to static websites. They can perform a wide range of functions, from simple tasks like calculators and to-do lists to complex operations like word processing, video editing, and even coding environments.
Key Characteristics of Web Apps
- Browser-based: Web apps run in web browsers, eliminating the need for installation on local devices.
- Cross-platform compatibility: They work across different operating systems and devices.
- Real-time updates: Web apps can be updated instantly without requiring user action.
- Internet-dependent: They require an internet connection to function fully.
- User interaction: Web apps often involve high levels of user engagement and input.
- Data storage: They can store and retrieve data from servers or databases.
Web Apps vs. Websites: Understanding the Difference
While web apps and websites may seem similar at first glance, there are key differences that set them apart:
Web Apps | Websites |
---|---|
Interactive and dynamic | Primarily informational and static |
Complex functionality | Simple navigation and content display |
User authentication often required | Usually accessible without login |
Task-oriented | Content-oriented |
Higher level of user engagement | Lower level of user interaction |
While these distinctions exist, the line between web apps and websites can sometimes blur, especially as websites become more interactive and feature-rich.
Types of Web Applications
Web applications come in various forms, each serving different purposes and utilizing different technologies. Here are some common types of web apps:
1. Single-Page Applications (SPAs)
Single-page applications load a single HTML page and dynamically update content as the user interacts with the app. This creates a fluid, desktop-like experience without page reloads.
Examples of SPAs:
- Gmail
- Google Maps
2. Progressive Web Apps (PWAs)
Progressive Web Apps combine the best of web and mobile apps. They can work offline, send push notifications, and even be installed on a user’s home screen.
Features of PWAs:
- Responsive design
- Offline functionality
- App-like interface
- Push notifications
3. Server-Side Rendered Apps
These applications generate the HTML on the server for each request. This approach can be beneficial for SEO and initial page load times.
Advantages:
- Better SEO performance
- Faster initial page loads
- Reduced client-side processing
4. Static Web Apps
Static web apps are pre-built files that are served to the browser as-is. They’re simple, fast, and secure, making them ideal for content-focused websites.
Use cases:
- Blogs
- Documentation sites
- Landing pages
Technologies Used in Web App Development
Developing web applications involves a wide array of technologies. Here’s an overview of the key components:
Front-end Technologies
The front-end, or client-side, is what users interact with directly. It’s built with:
- HTML (HyperText Markup Language): Structures the content of web pages.
- CSS (Cascading Style Sheets): Styles the appearance of HTML elements.
- JavaScript: Adds interactivity and dynamic behavior to web pages.
Popular front-end frameworks and libraries include:
- React.js
- Vue.js
- Angular
- Svelte
Back-end Technologies
The back-end, or server-side, handles the logic, database operations, and server management. Common back-end technologies include:
- Programming Languages: Python, Ruby, PHP, Java, C#, Node.js
- Frameworks: Django (Python), Ruby on Rails, Laravel (PHP), Express.js (Node.js)
- Databases: MySQL, PostgreSQL, MongoDB, Redis
- Server Software: Apache, Nginx, Microsoft IIS
Full-stack Development
Full-stack development involves working with both front-end and back-end technologies. Full-stack developers have a broad skill set that allows them to work on all aspects of web application development.
The Web App Development Process
Creating a web application involves several stages. Here’s a typical development process:
- Planning and Requirements Gathering: Define the app’s purpose, features, and target audience.
- Design: Create wireframes and mockups of the user interface.
- Front-end Development: Build the user interface using HTML, CSS, and JavaScript.
- Back-end Development: Develop server-side logic, APIs, and database interactions.
- Integration: Connect the front-end with the back-end.
- Testing: Perform various tests to ensure functionality, performance, and security.
- Deployment: Launch the web app on a production server.
- Maintenance and Updates: Continuously improve and update the app based on user feedback and changing requirements.
Advantages of Web Applications
Web applications offer numerous benefits over traditional desktop software:
- Accessibility: Can be accessed from any device with an internet connection.
- Cross-platform compatibility: Works across different operating systems.
- Easy updates: Changes can be pushed to all users simultaneously.
- Cost-effective: No need for distribution or installation of software.
- Scalability: Can handle growing numbers of users more easily than desktop apps.
- Reduced device storage: Most data is stored on servers, not on the user’s device.
Challenges in Web App Development
While web apps offer many advantages, they also come with their own set of challenges:
- Internet Dependency: Most web apps require a constant internet connection to function fully.
- Security Concerns: Web apps can be vulnerable to various cyber attacks.
- Performance Issues: Complex web apps may face performance challenges, especially on slower connections.
- Browser Compatibility: Ensuring consistent functionality across different browsers can be challenging.
- SEO Limitations: Some web app architectures (like SPAs) can pose challenges for search engine optimization.
The Future of Web Applications
The world of web applications is constantly evolving. Here are some trends shaping the future of web app development:
1. Progressive Web Apps (PWAs)
PWAs are becoming increasingly popular due to their ability to provide app-like experiences through web browsers. They offer offline functionality, push notifications, and can be added to a device’s home screen.
2. Artificial Intelligence and Machine Learning
AI and ML are being integrated into web apps to provide personalized experiences, automate tasks, and offer intelligent insights.
3. WebAssembly
WebAssembly allows high-performance applications to run in web browsers, opening up new possibilities for web-based games, video and audio editors, and more.
4. Serverless Architecture
Serverless computing is gaining traction, allowing developers to build and run applications without managing server infrastructure.
5. Voice User Interfaces
As voice recognition technology improves, we can expect to see more web apps incorporating voice commands and interactions.
Building Your First Web App: A Simple Tutorial
To give you a taste of web app development, let’s create a simple “Hello, World!” web app using HTML, CSS, and JavaScript. This basic example will help you understand the fundamental components of a web application.
Step 1: Create the HTML Structure
First, create a file named index.html
and add the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My First Web App</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="app">
<h1>Hello, World!</h1>
<button id="changeTextBtn">Change Text</button>
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Add Some Style with CSS
Create a file named styles.css
and add the following styles:
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
}
#app {
text-align: center;
}
button {
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
}
Step 3: Add Interactivity with JavaScript
Create a file named script.js
and add the following code:
document.addEventListener('DOMContentLoaded', () => {
const heading = document.querySelector('h1');
const changeTextBtn = document.getElementById('changeTextBtn');
changeTextBtn.addEventListener('click', () => {
heading.textContent = 'Hello, Web App Developer!';
});
});
Step 4: Run Your Web App
Open the index.html
file in a web browser. You should see a “Hello, World!” heading and a button. When you click the button, the heading will change to “Hello, Web App Developer!”
Congratulations! You’ve just created a simple web application. While this example is basic, it demonstrates the core concepts of web app development: structure (HTML), style (CSS), and interactivity (JavaScript).
Conclusion
Web applications have revolutionized the way we interact with software and services online. They offer unparalleled accessibility, flexibility, and potential for innovation. As an aspiring developer, understanding web apps is crucial for building modern, user-friendly solutions that can reach a global audience.
Whether you’re interested in front-end development, back-end programming, or full-stack expertise, the world of web applications offers endless opportunities to create, innovate, and solve real-world problems. As you continue your journey in coding education and skill development, remember that platforms like AlgoCademy can provide valuable resources and guidance to help you master the intricacies of web app development and prepare for technical interviews in the ever-evolving tech industry.
Keep exploring, keep coding, and who knows? The next groundbreaking web application might be the one you create!