What Employers Look For in a Programming Portfolio
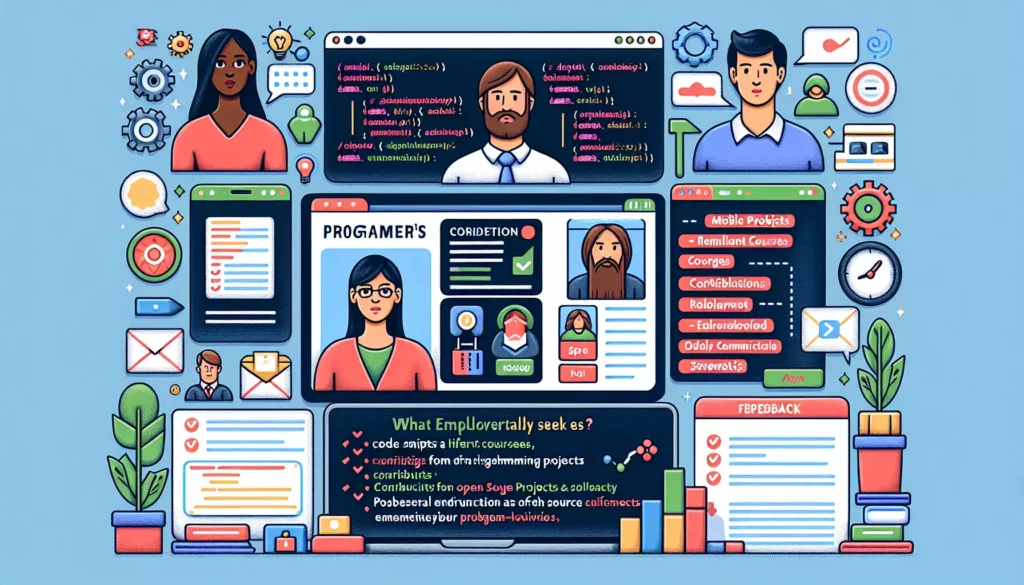
In the competitive world of software development, having a strong programming portfolio can be the key to landing your dream job. Whether you’re a fresh graduate or an experienced developer looking to switch careers, your portfolio is often the first impression you make on potential employers. But what exactly are these employers looking for when they review your programming portfolio? In this comprehensive guide, we’ll explore the essential elements that can make your portfolio stand out and increase your chances of impressing hiring managers at top tech companies.
1. Diverse Range of Projects
One of the first things employers look for in a programming portfolio is a diverse range of projects. This demonstrates your versatility as a developer and your ability to work with different technologies and solve various problems.
Why Diversity Matters
- Showcases adaptability
- Indicates a broad skill set
- Demonstrates willingness to learn new technologies
Include projects that span different domains, such as web development, mobile apps, data analysis, or machine learning. This variety will show that you’re not limited to a single area of expertise.
Types of Projects to Include
- Personal projects
- Open-source contributions
- Academic projects (if relevant)
- Hackathon submissions
- Client work (if permitted)
2. Clean and Well-Documented Code
Employers aren’t just looking at what you’ve built; they’re also interested in how you’ve built it. Clean, well-documented code is a hallmark of a professional developer.
Characteristics of Clean Code
- Readable and self-explanatory
- Follows consistent naming conventions
- Properly indented and formatted
- Modular and well-organized
Make sure to include code samples in your portfolio that showcase your ability to write clean, efficient code. Consider using platforms like GitHub Gists or CodePen to display your code snippets.
Documentation Best Practices
- Include clear README files for each project
- Add inline comments for complex logic
- Provide API documentation where applicable
- Use meaningful commit messages in version control
Here’s an example of a well-documented function in Python:
def calculate_fibonacci(n):
"""
Calculate the nth Fibonacci number.
Args:
n (int): The position of the Fibonacci number to calculate.
Returns:
int: The nth Fibonacci number.
Raises:
ValueError: If n is less than 0.
"""
if n < 0:
raise ValueError("n must be a non-negative integer")
if n <= 1:
return n
return calculate_fibonacci(n-1) + calculate_fibonacci(n-2)
3. Problem-Solving Skills
Employers are keen to see how you approach and solve complex problems. Your portfolio should showcase your problem-solving abilities and algorithmic thinking.
Ways to Demonstrate Problem-Solving Skills
- Include projects that solve real-world problems
- Showcase algorithms you’ve implemented
- Highlight optimization work you’ve done
- Include coding challenge solutions
Consider adding a section to your portfolio that specifically focuses on problem-solving. You could include solutions to LeetCode problems, hackerrank challenges, or even custom algorithms you’ve developed.
Example: Solving the Two Sum Problem
Here’s an efficient solution to the classic Two Sum problem, demonstrating both problem-solving skills and clean code:
def two_sum(nums, target):
"""
Find two numbers in the array that add up to the target.
Args:
nums (List[int]): An array of integers.
target (int): The target sum.
Returns:
List[int]: Indices of the two numbers that add up to the target.
"""
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
4. Familiarity with Industry-Standard Tools and Practices
Employers want to see that you’re familiar with the tools and practices commonly used in professional development environments.
Key Areas to Showcase
- Version control (e.g., Git)
- Continuous Integration/Continuous Deployment (CI/CD)
- Testing frameworks and methodologies
- Agile development practices
- Containerization (e.g., Docker)
Demonstrate your familiarity with these tools by including them in your project workflows and mentioning them in your project descriptions.
Example: Implementing CI/CD
Show that you understand CI/CD by including a .gitlab-ci.yml or .github/workflows/main.yml file in your projects. Here’s a simple example for a Python project:
name: Python CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m unittest discover tests
- name: Run linter
run: pylint **/*.py
5. Attention to User Experience and Design
While functionality is crucial, employers also value developers who understand the importance of user experience (UX) and design principles.
Aspects of UX to Consider
- Intuitive navigation
- Responsive design
- Accessibility features
- Performance optimization
For web-based projects, ensure that your applications are responsive and work well on different devices. Consider including before-and-after examples of UI improvements you’ve made.
Demonstrating Design Skills
- Include mockups or wireframes in your project documentation
- Showcase your ability to implement designs accurately
- Highlight any UI/UX improvements you’ve made to existing projects
6. Continuous Learning and Growth
The tech industry evolves rapidly, and employers value candidates who demonstrate a commitment to continuous learning and staying up-to-date with new technologies.
Ways to Show Continuous Learning
- Include recent projects using cutting-edge technologies
- Showcase certificates or courses you’ve completed
- Maintain a blog discussing new tech trends or learnings
- Participate in coding challenges or hackathons
Consider creating a “Learning Journey” section in your portfolio where you document your progress and new skills acquired over time.
7. Collaboration and Communication Skills
While technical skills are crucial, employers also look for evidence of strong collaboration and communication abilities.
Demonstrating Collaboration
- Include group projects in your portfolio
- Highlight your contributions to open-source projects
- Showcase any mentoring or teaching experiences
Effective Communication
- Write clear and concise project descriptions
- Include documentation for your projects
- Share presentations or talks you’ve given
Consider including testimonials from collaborators or clients to further emphasize your teamwork and communication skills.
8. Understanding of Software Architecture
As you progress in your career, employers will be looking for evidence that you understand larger-scale software architecture concepts.
Key Architectural Concepts to Demonstrate
- Design patterns
- Microservices architecture
- RESTful API design
- Database schema design
- Scalability considerations
Include diagrams or explanations of the architecture used in your more complex projects. This shows that you can think beyond individual components and understand how systems fit together.
Example: Microservices Architecture Diagram
Consider including a diagram like this to illustrate your understanding of microservices:
+----------------+ +----------------+ +----------------+
| User Service | | Product Service | | Order Service |
| | | | | |
| +------------+ | | +------------+ | | +------------+ |
| | API | | | | API | | | | API | |
| +------------+ | | +------------+ | | +------------+ |
| | | | | |
| +------------+ | | +------------+ | | +------------+ |
| | Database | | | | Database | | | | Database | |
| +------------+ | | +------------+ | | +------------+ |
+----------------+ +----------------+ +----------------+
^ ^ ^
| | |
v v v
+-------------------------------------------------------+
| API Gateway |
+-------------------------------------------------------+
^
|
v
+-------------------------------------------------------+
| Client Application |
+-------------------------------------------------------+
9. Testing and Quality Assurance
Employers value developers who prioritize code quality and understand the importance of testing.
Types of Testing to Showcase
- Unit testing
- Integration testing
- End-to-end testing
- Test-Driven Development (TDD)
Include test suites in your projects and mention your testing approach in project descriptions. This demonstrates your commitment to producing reliable, high-quality code.
Example: Unit Test in Python
Here’s an example of a simple unit test for the two_sum function we defined earlier:
import unittest
class TestTwoSum(unittest.TestCase):
def test_two_sum(self):
self.assertEqual(two_sum([2, 7, 11, 15], 9), [0, 1])
self.assertEqual(two_sum([3, 2, 4], 6), [1, 2])
self.assertEqual(two_sum([3, 3], 6), [0, 1])
self.assertEqual(two_sum([1, 2, 3, 4, 5], 10), [])
if __name__ == '__main__':
unittest.main()
10. Project Management and Planning
For more senior roles, employers often look for evidence of project management skills.
Ways to Demonstrate Project Management
- Include project timelines or Gantt charts
- Show how you’ve used project management tools (e.g., Jira, Trello)
- Describe how you’ve managed resources or led teams
- Showcase your ability to break down large projects into manageable tasks
Consider creating a case study for one of your larger projects, detailing the planning process, challenges faced, and how you overcame them.
11. Performance Optimization
Employers value developers who can write not just functional code, but efficient code that performs well at scale.
Areas of Performance Optimization
- Algorithm efficiency
- Database query optimization
- Front-end performance (e.g., load times, rendering)
- Scalability considerations
Include examples of how you’ve optimized code or improved system performance in your projects. Use metrics to quantify the improvements you’ve made.
Example: Optimizing Database Queries
Show how you’ve optimized a slow database query:
-- Original slow query
SELECT *
FROM orders
JOIN customers ON orders.customer_id = customers.id
WHERE orders.status = 'shipped'
AND customers.country = 'USA'
-- Optimized query with indexing and limiting columns
CREATE INDEX idx_orders_status ON orders(status);
CREATE INDEX idx_customers_country ON customers(country);
SELECT o.id, o.order_date, c.name, c.email
FROM orders o
JOIN customers c ON o.customer_id = c.id
WHERE o.status = 'shipped'
AND c.country = 'USA'
12. Security Awareness
With the increasing importance of cybersecurity, employers look for developers who understand and implement security best practices.
Security Aspects to Consider
- Input validation and sanitization
- Protection against common vulnerabilities (e.g., SQL injection, XSS)
- Secure authentication and authorization
- Data encryption
Highlight any security measures you’ve implemented in your projects, and consider including a security audit or penetration testing results if applicable.
Example: Secure Password Hashing
Demonstrate your understanding of security by showing how you properly hash and salt passwords:
import bcrypt
def hash_password(password):
"""
Securely hash a password using bcrypt.
Args:
password (str): The password to hash.
Returns:
bytes: The hashed password.
"""
salt = bcrypt.gensalt()
hashed = bcrypt.hashpw(password.encode('utf-8'), salt)
return hashed
def check_password(password, hashed):
"""
Check if a password matches the hashed version.
Args:
password (str): The password to check.
hashed (bytes): The hashed password to compare against.
Returns:
bool: True if the password matches, False otherwise.
"""
return bcrypt.checkpw(password.encode('utf-8'), hashed)
Conclusion
Creating a programming portfolio that stands out to employers requires more than just showcasing your coding skills. It’s about demonstrating your problem-solving abilities, your understanding of software development best practices, and your capacity for growth and learning.
Remember to:
- Showcase a diverse range of projects
- Emphasize clean, well-documented code
- Highlight your problem-solving skills
- Demonstrate familiarity with industry tools and practices
- Show attention to user experience and design
- Illustrate your commitment to continuous learning
- Emphasize collaboration and communication skills
- Display understanding of software architecture
- Showcase your testing and quality assurance practices
- Highlight project management abilities
- Demonstrate performance optimization skills
- Show awareness of security best practices
By focusing on these areas, you’ll create a comprehensive portfolio that not only showcases your technical skills but also demonstrates your value as a well-rounded software developer. This approach will significantly increase your chances of impressing potential employers and landing your dream job in the competitive world of software development.
Remember, your portfolio is a living document. Continue to update it with new projects, skills, and experiences as you grow in your career. Good luck with your job search!