What Are Neural Nets? A Comprehensive Guide for Aspiring Programmers
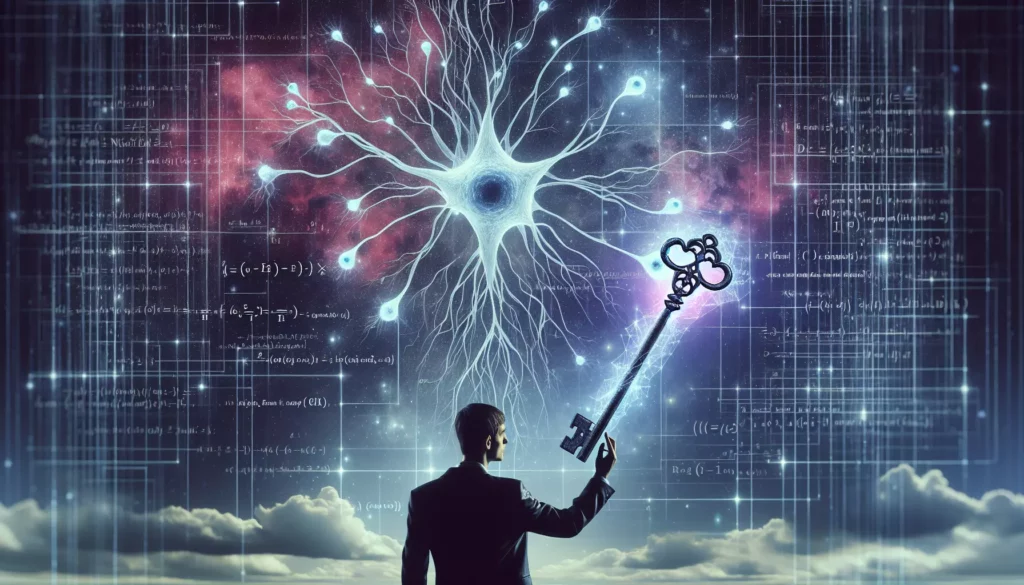
As you embark on your journey to become a proficient programmer, particularly if you’re aiming for a career in tech giants like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding neural networks is crucial. Neural nets are at the heart of many cutting-edge technologies and are a fundamental concept in machine learning and artificial intelligence. In this comprehensive guide, we’ll dive deep into the world of neural networks, exploring their structure, functionality, and applications in modern programming.
Table of Contents
- Introduction to Neural Networks
- Structure of Neural Networks
- How Neural Networks Work
- Types of Neural Networks
- Applications of Neural Networks in Programming
- Implementing Neural Networks: A Practical Approach
- Challenges and Considerations
- The Future of Neural Networks in Tech
- Conclusion
1. Introduction to Neural Networks
Neural networks, also known as artificial neural networks (ANNs) or simulated neural networks (SNNs), are a subset of machine learning and are at the core of deep learning algorithms. Their name and structure are inspired by the human brain, mimicking the way that biological neurons signal to one another.
At its core, a neural network is an interconnected group of nodes, akin to the vast network of neurons in a brain. These artificial nodes, known as “neurons” or “units,” are connected in layers, and each connection, like the synapses in a biological brain, can transmit a signal from one artificial neuron to another.
The idea behind neural networks is to have machines that can problem-solve and make decisions in a human-like manner. This is achieved through a process of learning from examples, rather than being explicitly programmed with a specific set of rules.
2. Structure of Neural Networks
To understand neural networks, it’s essential to grasp their basic structure. A typical neural network consists of three main layers:
- Input Layer: This is where the network receives data. Each input neuron represents a feature in your dataset.
- Hidden Layer(s): These intermediate layers process the inputs. A network can have one or multiple hidden layers.
- Output Layer: This layer produces the final result or prediction.
Each neuron in these layers is connected to neurons in the adjacent layers. These connections have associated weights, which determine the strength of the signal passed from one neuron to another.
Here’s a simple visual representation of a basic neural network structure:
<!-- ASCII art representation of a neural network -->
Input Layer Hidden Layer Output Layer
(x) (h) (y)
o o
| \ / | \
| \ / | \
o o o o o
| / \ | /
| / \ | /
o o
3. How Neural Networks Work
The functioning of neural networks can be broken down into several key steps:
3.1 Initialization
When a neural network is created, its weights are initialized, often with small random values. This randomness is crucial as it gives the network a starting point from which to learn.
3.2 Forward Propagation
During forward propagation, input data moves through the network:
- Each neuron receives inputs from the previous layer.
- These inputs are multiplied by their respective weights.
- The results are summed, and a bias term is added.
- This sum is passed through an activation function, which introduces non-linearity into the model.
- The output of the activation function becomes the input for the next layer.
Here’s a simplified Python representation of forward propagation in a single neuron:
def neuron_output(inputs, weights, bias):
# Calculate the weighted sum of inputs
weighted_sum = sum([i * w for i, w in zip(inputs, weights)]) + bias
# Apply activation function (e.g., ReLU)
return max(0, weighted_sum) # ReLU activation
3.3 Loss Calculation
After forward propagation, the network’s output is compared to the expected output. The difference is quantified using a loss function, which measures how far off the prediction is from the actual value.
3.4 Backpropagation
Backpropagation is the process of propagating the error backwards through the network:
- The error is calculated at the output layer.
- This error is then propagated backwards, layer by layer.
- At each layer, the error is used to calculate gradients for the weights and biases.
3.5 Weight Update
Using the gradients calculated during backpropagation, the weights and biases are updated to minimize the loss. This is typically done using an optimization algorithm like gradient descent.
def update_weights(weights, gradients, learning_rate):
return [w - learning_rate * g for w, g in zip(weights, gradients)]
3.6 Iteration
Steps 3.2 to 3.5 are repeated many times with different training examples. This iterative process allows the network to learn and improve its predictions over time.
4. Types of Neural Networks
There are several types of neural networks, each designed for specific tasks:
4.1 Feedforward Neural Networks (FNN)
The simplest type of artificial neural network. Information moves in only one direction, forward, from the input nodes, through the hidden nodes, to the output nodes. There are no cycles or loops in the network.
4.2 Convolutional Neural Networks (CNN)
Primarily used for image processing and computer vision tasks. CNNs use convolution in place of general matrix multiplication in at least one of their layers.
4.3 Recurrent Neural Networks (RNN)
Designed to recognize patterns in sequences of data, such as text, genomes, handwriting, or numerical time series data. They use internal memory to process sequences of inputs.
4.4 Long Short-Term Memory Networks (LSTM)
A special kind of RNN capable of learning long-term dependencies. LSTMs are explicitly designed to avoid the long-term dependency problem.
4.5 Generative Adversarial Networks (GAN)
Consist of two networks, a generator and a discriminator, pitted against each other. GANs can generate new, synthetic instances of data that can pass for real data.
5. Applications of Neural Networks in Programming
Neural networks have a wide range of applications in modern programming and technology:
5.1 Image and Speech Recognition
CNNs are widely used for image classification, object detection, and facial recognition. Similarly, neural networks power speech recognition systems in virtual assistants like Siri or Alexa.
5.2 Natural Language Processing (NLP)
RNNs and transformers are used in language translation, sentiment analysis, and text generation tasks.
5.3 Autonomous Vehicles
Neural networks play a crucial role in processing sensor data and making decisions in self-driving cars.
5.4 Game Playing
Deep neural networks have been used to create AI that can beat human champions at complex games like Go and chess.
5.5 Financial Forecasting
Neural networks are used for stock market prediction, credit scoring, and fraud detection in the financial sector.
5.6 Healthcare
In medical imaging, neural networks assist in diagnosing diseases from X-rays, MRIs, and CT scans.
6. Implementing Neural Networks: A Practical Approach
For aspiring programmers, especially those aiming for FAANG companies, it’s crucial to not just understand neural networks theoretically but also be able to implement them. Here’s a basic example of how you might implement a simple neural network in Python using NumPy:
import numpy as np
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# Initialize weights and biases
self.W1 = np.random.randn(self.input_size, self.hidden_size)
self.b1 = np.zeros((1, self.hidden_size))
self.W2 = np.random.randn(self.hidden_size, self.output_size)
self.b2 = np.zeros((1, self.output_size))
def forward(self, X):
# Forward propagation
self.z1 = np.dot(X, self.W1) + self.b1
self.a1 = self.sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.W2) + self.b2
self.a2 = self.sigmoid(self.z2)
return self.a2
def sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def sigmoid_derivative(self, z):
return z * (1 - z)
def backward(self, X, y, output):
# Backpropagation
self.error = y - output
self.delta2 = self.error * self.sigmoid_derivative(output)
self.dW2 = np.dot(self.a1.T, self.delta2)
self.db2 = np.sum(self.delta2, axis=0, keepdims=True)
self.error_hidden = np.dot(self.delta2, self.W2.T)
self.delta1 = self.error_hidden * self.sigmoid_derivative(self.a1)
self.dW1 = np.dot(X.T, self.delta1)
self.db1 = np.sum(self.delta1, axis=0)
def train(self, X, y, epochs, learning_rate):
for _ in range(epochs):
output = self.forward(X)
self.backward(X, y, output)
# Update weights and biases
self.W1 += learning_rate * self.dW1
self.b1 += learning_rate * self.db1
self.W2 += learning_rate * self.dW2
self.b2 += learning_rate * self.db2
# Example usage
nn = NeuralNetwork(2, 4, 1)
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
y = np.array([[0], [1], [1], [0]])
nn.train(X, y, epochs=10000, learning_rate=0.1)
# Test the trained network
for i in range(4):
print(f"Input: {X[i]}, Predicted Output: {nn.forward(X[i])}")
This example implements a simple neural network for the XOR problem. It’s a basic implementation and doesn’t include many optimizations used in practice, but it demonstrates the core concepts of neural networks.
7. Challenges and Considerations
While neural networks are powerful, they come with their own set of challenges:
7.1 Overfitting
Neural networks can sometimes perform well on training data but fail to generalize to new, unseen data. This is known as overfitting. Techniques like regularization and dropout are used to combat this.
7.2 Computational Complexity
Training deep neural networks can be computationally expensive, often requiring specialized hardware like GPUs.
7.3 Black Box Nature
The decision-making process in complex neural networks can be difficult to interpret, leading to challenges in explaining how a network arrived at a particular output.
7.4 Data Requirements
Neural networks typically require large amounts of labeled data for training, which can be expensive or time-consuming to obtain.
7.5 Hyperparameter Tuning
Choosing the right architecture and hyperparameters (like learning rate, number of layers, etc.) can be challenging and often requires extensive experimentation.
8. The Future of Neural Networks in Tech
As an aspiring programmer, especially if you’re aiming for top tech companies, it’s important to keep an eye on the future of neural networks:
8.1 Increased Efficiency
Research is ongoing to make neural networks more efficient, both in terms of computational requirements and energy consumption.
8.2 Improved Interpretability
There’s a growing focus on developing methods to better understand and interpret the decisions made by neural networks.
8.3 Neuromorphic Computing
This involves developing computer chips that mimic the structure and function of biological neural networks, potentially leading to more efficient AI systems.
8.4 Quantum Neural Networks
The intersection of quantum computing and neural networks is an exciting area of research that could lead to significant advancements in computational power.
8.5 Ethical AI
As neural networks become more prevalent in decision-making systems, there’s an increased focus on ensuring these systems are fair, unbiased, and ethically implemented.
9. Conclusion
Neural networks are a fascinating and powerful tool in the world of programming and artificial intelligence. As an aspiring programmer, especially one aiming for a career in major tech companies, understanding neural networks is crucial. They form the backbone of many cutting-edge technologies and are likely to play an even more significant role in the future of tech.
Remember, the journey to mastering neural networks is ongoing. As you progress in your coding education and skills development, continue to explore and experiment with neural networks. Practice implementing them, stay updated with the latest research, and don’t hesitate to apply them in your projects.
By building a strong foundation in neural networks, you’re not just learning a specific technology; you’re developing a way of thinking about problem-solving that will serve you well in your programming career, whether you end up at a FAANG company or anywhere else in the tech world. Keep coding, keep learning, and embrace the exciting possibilities that neural networks bring to the world of programming!