What Are Coding Challenges, and Why Are They Important?
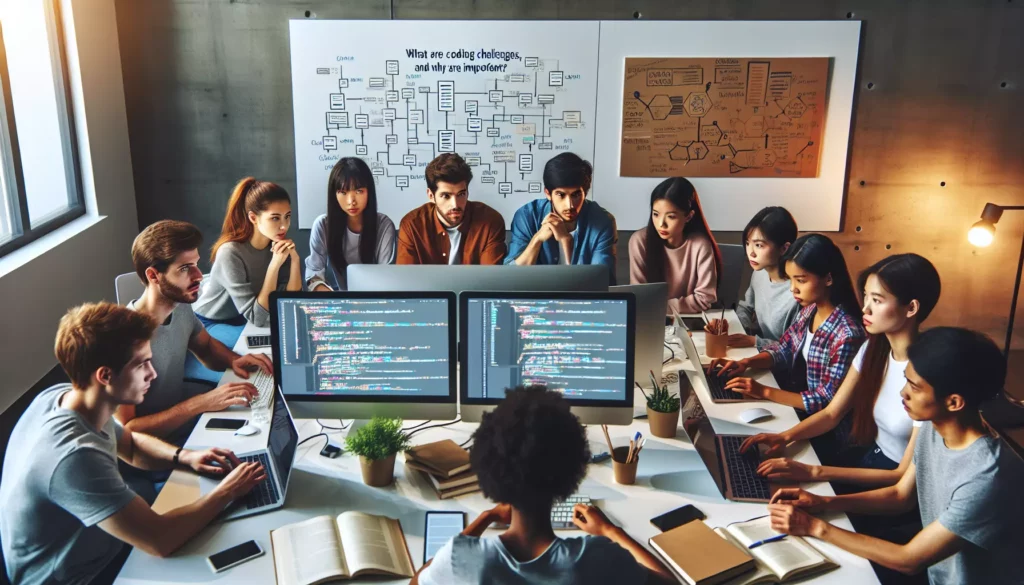
In the ever-evolving world of technology and software development, coding challenges have become an integral part of a programmer’s journey. Whether you’re a beginner just starting to learn the ropes or an experienced developer looking to sharpen your skills, coding challenges offer a unique and valuable way to enhance your programming abilities. In this comprehensive guide, we’ll explore what coding challenges are, why they’re important, and how they can benefit your career in the tech industry.
What Are Coding Challenges?
Coding challenges, also known as programming challenges or algorithmic puzzles, are problem-solving exercises designed to test and improve a programmer’s skills. These challenges typically involve writing code to solve a specific problem or implement a particular algorithm within given constraints. They can range from simple tasks that take a few minutes to complex problems that require hours of thoughtful consideration and coding.
Coding challenges come in various forms and can be found on numerous platforms, including:
- Online coding platforms (e.g., LeetCode, HackerRank, CodeSignal)
- Competitive programming websites (e.g., Codeforces, TopCoder)
- Coding bootcamps and educational resources
- Technical interview preparation tools
- Coding contests and hackathons
These challenges often cover a wide range of topics, including:
- Data structures (arrays, linked lists, trees, graphs)
- Algorithms (sorting, searching, dynamic programming)
- Problem-solving techniques
- Time and space complexity optimization
- Language-specific features and best practices
Why Are Coding Challenges Important?
Coding challenges play a crucial role in a programmer’s development and career progression. Here are some key reasons why they are important:
1. Skill Enhancement and Practice
Regular practice is essential for improving any skill, and programming is no exception. Coding challenges provide a structured way to practice writing code, implementing algorithms, and solving problems. By tackling diverse challenges, you can:
- Reinforce your understanding of fundamental concepts
- Learn new problem-solving techniques
- Improve your coding speed and efficiency
- Gain exposure to different types of problems and scenarios
2. Preparation for Technical Interviews
Many tech companies, especially larger ones like FAANG (Facebook, Amazon, Apple, Netflix, Google), use coding challenges as part of their interview process. By regularly solving coding challenges, you can:
- Familiarize yourself with common interview question patterns
- Improve your ability to solve problems under time pressure
- Gain confidence in explaining your thought process and solution
- Practice writing clean, efficient code that meets specific requirements
3. Algorithmic Thinking and Problem-Solving Skills
Coding challenges often require you to think critically and develop algorithmic solutions to complex problems. This process helps you:
- Improve your analytical and logical thinking skills
- Learn to break down complex problems into smaller, manageable parts
- Develop the ability to recognize patterns and apply appropriate algorithms
- Enhance your creativity in finding innovative solutions
4. Exposure to Different Programming Paradigms and Techniques
Through diverse coding challenges, you can explore various programming paradigms and techniques, such as:
- Object-oriented programming
- Functional programming
- Recursion
- Dynamic programming
- Greedy algorithms
This exposure broadens your skill set and makes you a more versatile programmer.
5. Time and Space Complexity Optimization
Many coding challenges require you to optimize your solutions for time and space efficiency. This focus helps you:
- Understand the importance of algorithmic efficiency
- Learn to analyze and improve the time and space complexity of your code
- Develop skills in writing scalable and performant solutions
6. Continuous Learning and Staying Updated
The tech industry is constantly evolving, and new programming languages, frameworks, and best practices emerge regularly. Coding challenges can help you:
- Stay updated with the latest industry trends and technologies
- Learn new programming languages or features
- Adapt to changing coding standards and best practices
7. Building a Portfolio and Showcasing Skills
Consistently solving coding challenges and participating in coding competitions can help you build a strong portfolio of work. This can be valuable when:
- Applying for jobs or internships
- Demonstrating your skills to potential employers
- Tracking your own progress and growth as a programmer
Types of Coding Challenges
Coding challenges come in various formats, each designed to test different aspects of your programming skills. Here are some common types of coding challenges you might encounter:
1. Algorithm Implementation
These challenges require you to implement a specific algorithm, such as sorting an array, searching for an element, or traversing a graph. For example:
// Implement a function to sort an array using the quicksort algorithm
function quickSort(arr) {
// Your implementation here
}
// Example usage
const unsortedArray = [64, 34, 25, 12, 22, 11, 90];
const sortedArray = quickSort(unsortedArray);
console.log(sortedArray); // Should output: [11, 12, 22, 25, 34, 64, 90]
2. Data Structure Manipulation
These challenges focus on working with various data structures, such as arrays, linked lists, trees, or graphs. For instance:
// Implement a function to reverse a linked list
class ListNode {
constructor(val = 0, next = null) {
this.val = val;
this.next = next;
}
}
function reverseLinkedList(head) {
// Your implementation here
}
// Example usage
const list = new ListNode(1, new ListNode(2, new ListNode(3, new ListNode(4))));
const reversedList = reverseLinkedList(list);
// Should output: 4 -> 3 -> 2 -> 1 -> null
3. Problem-Solving and Logic
These challenges test your ability to solve complex problems using logical thinking and programming skills. For example:
// Given a string, find the length of the longest palindromic substring
function longestPalindromicSubstring(s) {
// Your implementation here
}
// Example usage
console.log(longestPalindromicSubstring("babad")); // Should output: "bab" or "aba"
console.log(longestPalindromicSubstring("cbbd")); // Should output: "bb"
4. Optimization Challenges
These challenges require you to optimize an existing solution for better time or space complexity. For instance:
// Optimize the following function to find the nth Fibonacci number
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// Your optimized implementation here
function optimizedFibonacci(n) {
// Implement a more efficient solution
}
// Example usage
console.log(optimizedFibonacci(50)); // Should output the 50th Fibonacci number efficiently
5. System Design and Architecture
These challenges focus on designing and implementing larger systems or applications. They often require you to consider scalability, performance, and maintainability. For example:
// Design a simple in-memory key-value store with the following operations:
// - put(key, value): Insert a key-value pair
// - get(key): Retrieve the value associated with a key
// - delete(key): Remove a key-value pair
// - keys(): Return all keys in the store
class KeyValueStore {
constructor() {
// Initialize your data structure here
}
put(key, value) {
// Implement put operation
}
get(key) {
// Implement get operation
}
delete(key) {
// Implement delete operation
}
keys() {
// Implement keys operation
}
}
// Example usage
const store = new KeyValueStore();
store.put("name", "John");
store.put("age", 30);
console.log(store.get("name")); // Should output: "John"
console.log(store.keys()); // Should output: ["name", "age"]
store.delete("age");
console.log(store.keys()); // Should output: ["name"]
How to Approach Coding Challenges
To get the most out of coding challenges and improve your skills effectively, consider the following approach:
1. Understand the Problem
Before diving into coding, make sure you fully understand the problem statement, requirements, and constraints. Ask yourself:
- What are the inputs and expected outputs?
- Are there any special cases or edge cases to consider?
- What are the time and space complexity requirements, if any?
2. Plan Your Approach
Develop a high-level plan for solving the problem before writing any code. This may involve:
- Breaking down the problem into smaller, manageable steps
- Choosing appropriate data structures and algorithms
- Sketching out a flowchart or pseudocode
3. Implement Your Solution
Write clean, readable code that implements your planned approach. Remember to:
- Use meaningful variable and function names
- Comment your code where necessary
- Follow best practices and coding standards for your chosen language
4. Test Your Solution
Thoroughly test your implementation with various inputs, including:
- Normal cases
- Edge cases (e.g., empty input, maximum values)
- Special cases mentioned in the problem statement
5. Optimize and Refactor
Once you have a working solution, consider ways to improve it:
- Can you optimize the time or space complexity?
- Is there a more elegant or concise way to express the solution?
- Are there any redundant operations that can be eliminated?
6. Reflect and Learn
After completing a challenge, take time to reflect on what you’ve learned:
- What new concepts or techniques did you encounter?
- How can you apply this knowledge to future problems?
- Are there alternative solutions you could explore?
Resources for Coding Challenges
There are numerous resources available for practicing coding challenges and improving your programming skills. Here are some popular platforms and tools:
1. Online Coding Platforms
- LeetCode: Offers a wide range of coding challenges, from easy to hard, with a focus on interview preparation.
- HackerRank: Provides coding challenges across various domains, including algorithms, data structures, and specific programming languages.
- CodeSignal: Features coding challenges and assessments used by many companies for technical interviews.
2. Competitive Programming Websites
- Codeforces: Hosts regular coding contests and provides a platform for solving algorithmic problems.
- TopCoder: Offers competitive programming challenges and hosts algorithm competitions.
- AtCoder: A Japanese competitive programming platform with high-quality algorithmic problems.
3. Educational Resources
- AlgoCademy: Provides interactive coding tutorials and resources for learners, focusing on algorithmic thinking and problem-solving skills.
- Coursera: Offers courses on algorithms and data structures from top universities.
- edX: Provides free online courses on computer science topics, including algorithms and programming.
4. Books and Written Resources
- “Cracking the Coding Interview” by Gayle Laakmann McDowell: A comprehensive guide to preparing for technical interviews, including numerous coding challenges.
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein: A thorough textbook on algorithms and data structures.
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash: Provides a collection of challenging coding problems with detailed solutions.
Conclusion
Coding challenges are an essential tool for programmers of all levels to improve their skills, prepare for technical interviews, and stay competitive in the ever-evolving tech industry. By regularly engaging with coding challenges, you can enhance your problem-solving abilities, deepen your understanding of algorithms and data structures, and build a strong foundation for a successful career in software development.
Remember that consistency is key when it comes to improving your coding skills. Set aside regular time to practice coding challenges, and don’t be discouraged if you encounter difficult problems. Each challenge you tackle, whether you solve it immediately or struggle with it, is an opportunity to learn and grow as a programmer.
As you progress in your journey, consider participating in coding competitions, contributing to open-source projects, or even creating your own coding challenges to share with others. The skills you develop through coding challenges will not only help you in technical interviews but also make you a more effective and efficient programmer in your day-to-day work.
Embrace the challenge, enjoy the process of problem-solving, and watch as your coding skills reach new heights. Happy coding!