What Are Chatbots: A Comprehensive Guide to Conversational AI
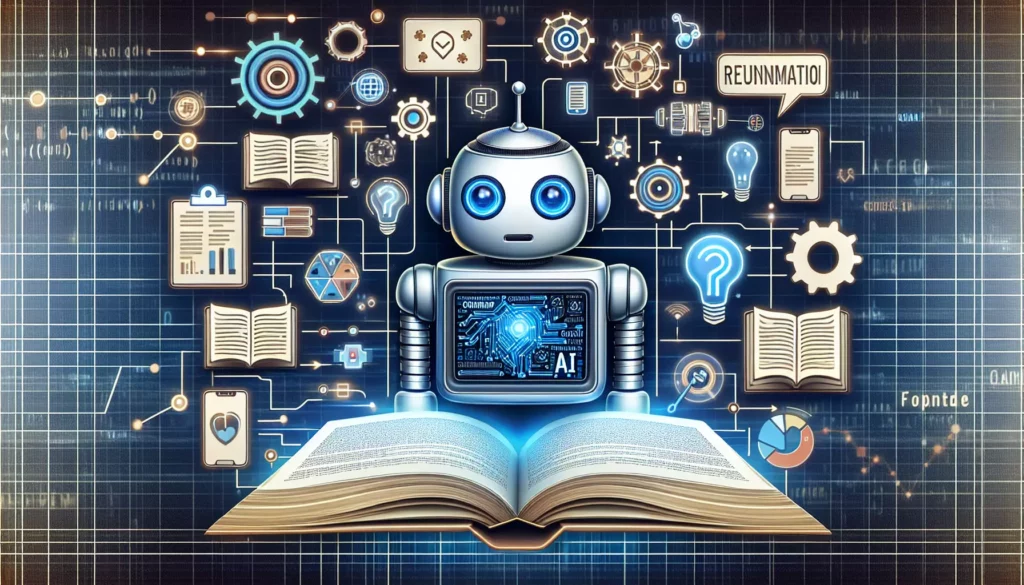
In the rapidly evolving landscape of technology, chatbots have emerged as a revolutionary tool, transforming the way businesses interact with customers and how we interface with digital systems. But what exactly are chatbots, and why have they become such a crucial element in the tech ecosystem? This comprehensive guide will delve into the world of chatbots, exploring their functionality, types, applications, and the impact they’re having across various industries.
Understanding Chatbots: The Basics
At their core, chatbots are computer programs designed to simulate human conversation. They use natural language processing (NLP) and artificial intelligence (AI) to understand user inputs and provide appropriate responses. The goal is to create an interaction that feels as natural and helpful as possible, mimicking a conversation with a human customer service representative or assistant.
Key Components of Chatbots
- Natural Language Processing (NLP): This is the technology that allows chatbots to understand and interpret human language.
- Machine Learning: Many advanced chatbots use machine learning algorithms to improve their responses over time.
- Dialog Management: This component handles the flow of conversation, ensuring coherent and contextually appropriate responses.
- Integration Capabilities: Chatbots often need to integrate with various systems to access data and perform actions.
Types of Chatbots
Chatbots come in various forms, each with its own level of sophistication and capability. Understanding these types can help in choosing the right chatbot for specific needs.
1. Rule-Based Chatbots
These are the simplest form of chatbots. They operate on a set of predefined rules and can only respond to specific commands or questions. While limited in their scope, they can be effective for straightforward tasks like FAQ responses or simple customer service queries.
2. AI-Powered Chatbots
These more advanced chatbots use machine learning and natural language processing to understand context and intent. They can handle more complex queries and improve their responses over time through learning from interactions.
3. Hybrid Chatbots
Combining elements of both rule-based and AI-powered chatbots, hybrid models aim to balance the reliability of predefined responses with the flexibility of AI-driven conversation.
Applications of Chatbots
The versatility of chatbots has led to their adoption across numerous industries and use cases. Here are some prominent applications:
Customer Service
Perhaps the most common use of chatbots is in customer service. They can handle a wide range of customer inquiries, from product information to troubleshooting, often resolving issues without human intervention.
E-commerce
In online retail, chatbots assist customers in finding products, tracking orders, and even making purchase recommendations based on browsing history and preferences.
Healthcare
Chatbots in healthcare can schedule appointments, provide basic medical information, and even assist in preliminary diagnoses, although they always defer to medical professionals for actual medical advice.
Education
In the realm of education, chatbots can serve as virtual tutors, answering students’ questions, providing explanations, and even quizzing learners on various subjects.
Personal Assistants
Virtual assistants like Siri, Alexa, and Google Assistant are sophisticated chatbots that can perform a wide range of tasks, from setting reminders to controlling smart home devices.
The Technology Behind Chatbots
To truly understand chatbots, it’s essential to delve into the technology that powers them. This is where we bridge the gap between chatbots and the broader field of coding and software development.
Natural Language Processing (NLP)
NLP is a branch of AI that focuses on the interaction between computers and human language. It’s what allows chatbots to understand and generate human-like text. Key components of NLP include:
- Tokenization: Breaking down text into individual words or phrases.
- Part-of-speech tagging: Identifying the grammatical parts of speech in a sentence.
- Named entity recognition: Identifying and classifying named entities (like people, places, organizations) in text.
- Sentiment analysis: Determining the emotional tone behind words.
Implementing NLP often involves using libraries and frameworks such as NLTK (Natural Language Toolkit) in Python. Here’s a simple example of tokenization using NLTK:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
text = "Hello, how can I help you today?"
tokens = word_tokenize(text)
print(tokens)
# Output: ['Hello', ',', 'how', 'can', 'I', 'help', 'you', 'today', '?']
Machine Learning Algorithms
Machine learning is crucial for creating chatbots that can learn and improve over time. Common algorithms used in chatbot development include:
- Neural Networks: Particularly recurrent neural networks (RNNs) and transformer models for sequence-to-sequence tasks.
- Decision Trees: For creating rule-based logic in simpler chatbots.
- Support Vector Machines (SVM): For classification tasks in NLP.
Here’s a basic example of how you might use a machine learning model (in this case, a simple classifier) to determine the intent of a user’s message:
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.naive_bayes import MultinomialNB
# Training data
X = ["How do I reset my password?", "What are your business hours?", "I want to return an item"]
y = ["account", "general", "order"]
# Vectorize the text
vectorizer = CountVectorizer()
X_vectorized = vectorizer.fit_transform(X)
# Train the model
clf = MultinomialNB()
clf.fit(X_vectorized, y)
# Predict intent for a new message
new_message = "Can you help me change my password?"
new_message_vectorized = vectorizer.transform([new_message])
predicted_intent = clf.predict(new_message_vectorized)
print(f"Predicted intent: {predicted_intent[0]}")
# Output: Predicted intent: account
Dialog Management Systems
Dialog management is crucial for maintaining the flow and context of a conversation. It involves tracking the state of the conversation, managing context, and deciding on appropriate responses. Frameworks like Rasa and Dialogflow provide tools for building sophisticated dialog management systems.
Here’s a simplified example of how you might structure a basic dialog manager in Python:
class DialogManager:
def __init__(self):
self.state = "greeting"
def get_response(self, user_input):
if self.state == "greeting":
self.state = "awaiting_query"
return "Hello! How can I assist you today?"
elif self.state == "awaiting_query":
if "password" in user_input.lower():
self.state = "password_reset"
return "I can help you reset your password. Would you like to proceed?"
else:
return "I'm sorry, I didn't understand that. Could you please rephrase?"
elif self.state == "password_reset":
if "yes" in user_input.lower():
self.state = "reset_instructions"
return "Great! To reset your password, please follow these steps..."
else:
self.state = "awaiting_query"
return "Okay, is there anything else I can help you with?"
# Usage
dm = DialogManager()
print(dm.get_response("")) # Initial greeting
print(dm.get_response("I need to reset my password"))
print(dm.get_response("Yes, please"))
Building a Simple Chatbot
To illustrate the process of creating a chatbot, let’s walk through building a simple rule-based chatbot using Python. This example will demonstrate the basic structure and logic behind a chatbot, albeit a very simple one.
import re
class SimpleBot:
def __init__(self):
self.patterns = {
r"hi|hello|hey": "Hello! How can I help you?",
r"how are you": "I'm doing well, thank you for asking!",
r"bye|goodbye": "Goodbye! Have a great day!",
r"name": "My name is SimpleBot. It's nice to meet you!",
r"help": "I can provide information or assist with basic queries. What do you need help with?"
}
def get_response(self, user_input):
user_input = user_input.lower()
for pattern, response in self.patterns.items():
if re.search(pattern, user_input):
return response
return "I'm sorry, I don't understand that. Could you please rephrase or ask something else?"
def chat():
bot = SimpleBot()
print("SimpleBot: Hello! Type 'bye' to exit.")
while True:
user_input = input("You: ")
if user_input.lower() == 'bye':
print("SimpleBot: Goodbye!")
break
response = bot.get_response(user_input)
print("SimpleBot:", response)
if __name__ == "__main__":
chat()
This simple chatbot uses regular expressions to match user inputs with predefined patterns and respond accordingly. While basic, it demonstrates the fundamental concept of input processing and response generation in chatbots.
Challenges in Chatbot Development
Despite their increasing sophistication, chatbot development comes with several challenges:
1. Understanding Context
One of the biggest challenges is enabling chatbots to understand and maintain context throughout a conversation. This requires advanced NLP techniques and often involves maintaining a conversation state.
2. Handling Ambiguity
Human language is inherently ambiguous. Chatbots need to be able to handle unclear or vague inputs, often requiring clarification or making educated guesses about user intent.
3. Maintaining Personality and Tone
For chatbots to feel natural, they need to maintain a consistent personality and tone. This can be challenging, especially when dealing with a wide range of topics or emotional states.
4. Integration with Backend Systems
Many chatbots need to integrate with various backend systems to access data or perform actions. This requires careful API design and robust error handling.
5. Scalability
As chatbots handle more conversations, ensuring they can scale efficiently while maintaining performance becomes crucial.
The Future of Chatbots
The field of chatbot technology is rapidly evolving, with several exciting trends on the horizon:
1. Advanced AI and Machine Learning
As AI technology advances, we can expect chatbots to become even more sophisticated, with better natural language understanding and generation capabilities.
2. Voice-based Chatbots
With the rise of voice assistants, we’re likely to see more integration between text-based chatbots and voice interfaces.
3. Emotional Intelligence
Future chatbots may be better equipped to recognize and respond to human emotions, leading to more empathetic and nuanced interactions.
4. Multilingual Capabilities
As global businesses expand, chatbots with robust multilingual capabilities will become increasingly important.
5. Integration with IoT
Chatbots may become central interfaces for controlling and interacting with Internet of Things (IoT) devices in homes and workplaces.
Conclusion
Chatbots represent a fascinating intersection of artificial intelligence, natural language processing, and user interface design. From simple rule-based systems to sophisticated AI-powered assistants, chatbots are transforming how we interact with technology and businesses.
For developers and aspiring programmers, chatbot development offers an exciting field that combines challenging technical problems with immediate, tangible impact on user experiences. It requires a blend of skills, from natural language processing and machine learning to user experience design and backend integration.
As we look to the future, chatbots are likely to become even more prevalent and capable, opening up new possibilities for automation, customer service, and human-computer interaction. Whether you’re a business looking to improve customer engagement or a developer interested in the cutting edge of AI and NLP, understanding chatbots is becoming increasingly crucial in our digital world.
The journey of chatbot development is an ongoing one, filled with challenges and opportunities. As technology continues to evolve, so too will the capabilities and applications of chatbots, making this an exciting field to watch and participate in for years to come.