Vue vs. React: A Detailed Comparison for Frontend Developers
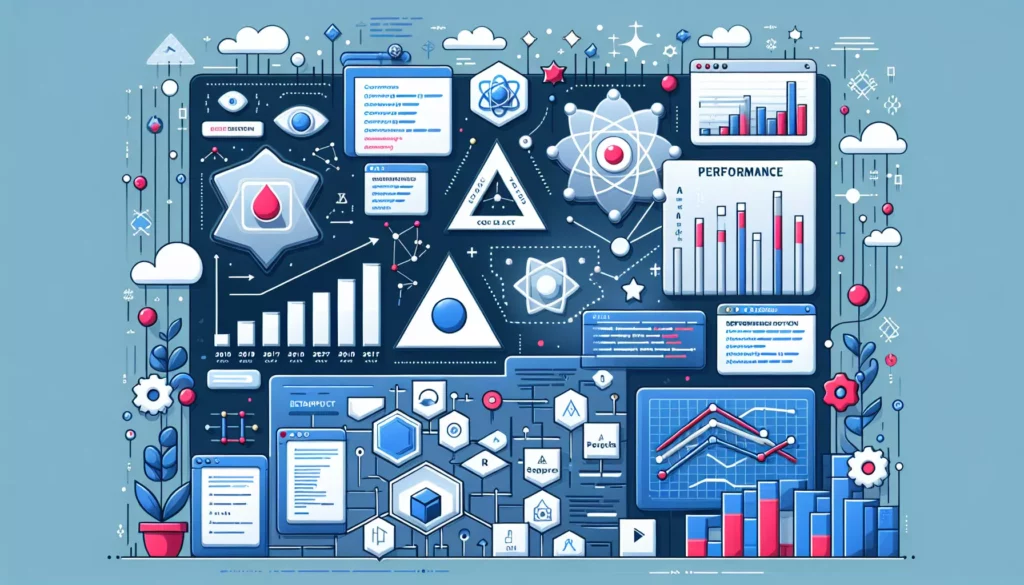
In the ever-evolving landscape of frontend development, two powerful JavaScript frameworks have emerged as frontrunners: Vue.js and React. Both offer robust solutions for building dynamic user interfaces, but they come with their own unique philosophies, strengths, and ecosystems. As a developer, choosing between Vue and React can significantly impact your project’s architecture, development speed, and long-term maintainability. In this comprehensive comparison, we’ll dive deep into the nuances of Vue and React, exploring their core concepts, performance, learning curves, and real-world applications to help you make an informed decision for your next project.
1. Introduction to Vue and React
Vue.js: The Progressive Framework
Vue.js, often referred to simply as Vue, was created by Evan You in 2014. It’s described as a progressive framework for building user interfaces, meaning you can adopt it incrementally in your projects. Vue is known for its simplicity, flexibility, and excellent documentation, making it a popular choice for both beginners and experienced developers.
React: The Library for Web and Native User Interfaces
React, developed and maintained by Facebook, was first released in 2013. It’s not a full-fledged framework but a library for building user interfaces. React’s component-based architecture and virtual DOM implementation have revolutionized the way developers think about building web applications. Its ecosystem has grown to include tools for state management, routing, and even mobile app development with React Native.
2. Core Concepts and Architecture
Vue’s Core Concepts
- Components: Vue applications are built using a component-based architecture, where each component encapsulates its own HTML, CSS, and JavaScript.
- Reactivity: Vue uses a reactive data model, automatically updating the DOM when data changes.
- Directives: Special attributes that extend HTML elements with additional behavior.
- Computed Properties and Watchers: For handling complex logic and side effects based on data changes.
- Single-File Components: .vue files that combine template, script, and style in one file.
React’s Core Concepts
- Components: React also uses a component-based architecture, with components as the building blocks of the UI.
- JSX: A syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files.
- Virtual DOM: An in-memory representation of the real DOM for optimized rendering.
- State and Props: Mechanisms for managing and passing data within the application.
- Hooks: Functions that let you use state and other React features without writing a class.
Architectural Differences
While both frameworks employ a component-based architecture, their approaches differ:
- Vue separates template, script, and style within a single file, promoting a clear separation of concerns.
- React encourages a JavaScript-centric approach, with JSX mixing markup and logic in the same file.
- Vue’s reactivity system is built-in and automatic, while React requires explicit state updates.
- React’s one-way data flow is more strict, while Vue allows for easier two-way data binding.
3. Performance Comparison
Performance is a crucial factor in choosing a framework, especially for large-scale applications. Both Vue and React are known for their excellent performance, but they achieve it through different means.
Vue’s Performance Features
- Lightweight: Vue has a smaller bundle size compared to React, which can lead to faster initial load times.
- Efficient Updates: Vue’s reactivity system allows for granular updates, potentially reducing unnecessary re-renders.
- Compiler Optimizations: Vue’s template compiler can apply optimizations at build time, improving runtime performance.
React’s Performance Features
- Virtual DOM: React’s virtual DOM implementation is highly optimized for efficient updates.
- Fiber Architecture: Introduced in React 16, it allows for incremental rendering and better prioritization of updates.
- Concurrent Mode: An experimental feature that enables React to work on multiple tasks simultaneously, improving responsiveness.
Benchmarks and Real-World Performance
While synthetic benchmarks often show Vue performing slightly better in terms of raw speed, real-world performance can vary greatly depending on the specific use case and implementation. Factors such as application size, complexity, and development practices play a significant role in determining actual performance.
It’s worth noting that both frameworks are constantly evolving, with each new version bringing performance improvements. In most cases, the performance difference between Vue and React is negligible, and other factors like developer experience and ecosystem support may be more important considerations.
4. Learning Curve and Developer Experience
Vue’s Learning Curve
Vue is often praised for its gentle learning curve, making it an excellent choice for beginners and those transitioning from traditional web development:
- Clear and comprehensive documentation
- Intuitive template syntax that feels familiar to HTML
- Progressive adoption allows for gradual integration into existing projects
- Less boilerplate code required to get started
For developers coming from jQuery or vanilla JavaScript backgrounds, Vue’s approach feels more natural and easier to grasp initially.
React’s Learning Curve
React has a steeper initial learning curve but offers a powerful and flexible development experience:
- JSX syntax requires some adjustment for developers new to the concept
- Functional programming concepts are more prevalent in React
- State management can be more complex, especially in larger applications
- Extensive ecosystem with many third-party libraries to learn
While React might take longer to master, many developers find its concepts and patterns beneficial for understanding modern frontend development practices.
Developer Tools and IDE Support
Both Vue and React have excellent developer tools and IDE support:
- Vue DevTools and React DevTools for browser-based debugging
- Strong TypeScript support for both frameworks
- Rich ecosystem of IDE plugins and extensions
React’s larger community has led to a more extensive selection of third-party tools and extensions, but Vue is quickly catching up in this area.
5. State Management
As applications grow in complexity, managing state becomes increasingly important. Both Vue and React offer solutions for state management, but their approaches differ.
Vue’s State Management
Vue provides several options for state management:
- Vuex: The official state management library for Vue, inspired by Flux architecture.
- Pinia: A newer, lighter-weight state management solution that’s gaining popularity in the Vue community.
- Composition API: Introduced in Vue 3, it allows for more flexible state management within components.
Example of using Vuex in a Vue component:
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { mapState, mapMutations } from 'vuex'
export default {
computed: {
...mapState(['count'])
},
methods: {
...mapMutations(['increment'])
}
}
</script>
React’s State Management
React’s ecosystem offers various state management solutions:
- Redux: A popular state management library, often used with React but not exclusive to it.
- MobX: An alternative to Redux that uses observable state.
- Context API: Built into React, it provides a way to pass data through the component tree without prop drilling.
- Recoil: A newer state management library developed by Facebook.
Example of using Redux in a React component:
import React from 'react'
import { useSelector, useDispatch } from 'react-redux'
import { increment } from './counterSlice'
function Counter() {
const count = useSelector((state) => state.counter.value)
const dispatch = useDispatch()
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch(increment())}>Increment</button>
</div>
)
}
Comparing State Management Approaches
Vue’s Vuex is more tightly integrated with the framework and follows Vue’s philosophy of simplicity. React’s ecosystem offers more choices, which can be both a blessing and a curse, depending on your perspective. The choice often comes down to personal preference and project requirements.
6. Routing
Routing is essential for building single-page applications (SPAs). Both Vue and React have official and community-supported routing solutions.
Vue Router
Vue Router is the official routing library for Vue.js. It integrates deeply with Vue core to make building SPAs with Vue a breeze. Key features include:
- Nested route/view mapping
- Modular, component-based router configuration
- Route params, query, wildcards
- View transition effects powered by Vue’s transition system
- Fine-grained navigation control
- Links with automatic active CSS classes
- HTML5 history mode or hash mode
Example of Vue Router usage:
// router.js
import { createRouter, createWebHistory } from 'vue-router'
import Home from './views/Home.vue'
import About from './views/About.vue'
const routes = [
{ path: '/', component: Home },
{ path: '/about', component: About }
]
const router = createRouter({
history: createWebHistory(),
routes
})
export default router
// main.js
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
createApp(App).use(router).mount('#app')
React Router
React Router is the most popular routing library for React applications. While not officially maintained by the React team, it’s widely adopted in the React community. Features include:
- Declarative routing
- Nested routes and layouts
- Route parameters
- Programmatic navigation
- Support for hash and browser history
- Server-side rendering support
Example of React Router usage:
import React from 'react'
import { BrowserRouter as Router, Route, Link } from 'react-router-dom'
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
</ul>
</nav>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</div>
</Router>
)
}
function Home() {
return <h2>Home</h2>
}
function About() {
return <h2>About</h2>
}
Routing Comparison
Both Vue Router and React Router provide similar functionality, but their integration and syntax differ:
- Vue Router is more tightly integrated with Vue and follows Vue’s design philosophy.
- React Router is more flexible but requires more setup and configuration.
- Vue Router’s configuration is typically more centralized, while React Router’s is often more distributed throughout the application.
7. Ecosystem and Community Support
The ecosystem and community surrounding a framework can greatly influence its adoption and long-term viability. Both Vue and React have strong ecosystems, but there are some differences to consider.
Vue Ecosystem
- Official Libraries: Vue provides official solutions for common needs like routing (Vue Router) and state management (Vuex).
- Vue CLI: A powerful CLI tool for quickly scaffolding Vue projects.
- Nuxt.js: A popular framework for building server-side rendered Vue applications.
- Community Plugins: A growing collection of community-created plugins and components.
React Ecosystem
- Extensive Third-party Libraries: React’s ecosystem is larger, with a vast array of third-party libraries for various purposes.
- Create React App: An officially supported way to create single-page React applications.
- Next.js: A popular framework for server-side rendering and static site generation with React.
- React Native: For building native mobile applications using React.
Community Comparison
React has a larger community due to its earlier release and backing by Facebook. This translates to:
- More job opportunities for React developers
- A larger pool of third-party libraries and tools
- More resources, tutorials, and community support
Vue, while having a smaller community, is known for:
- A more centralized ecosystem with official solutions
- High-quality documentation and learning resources
- A rapidly growing and passionate community
8. Testing
Both Vue and React have robust testing ecosystems, supporting various types of tests including unit, integration, and end-to-end testing.
Testing in Vue
Vue provides official testing utilities and recommends certain testing libraries:
- Vue Test Utils: The official testing library for Vue components
- Jest: A popular JavaScript testing framework, often used with Vue
- Cypress: For end-to-end testing
Example of a Vue component test:
import { mount } from '@vue/test-utils'
import Counter from '@/components/Counter.vue'
describe('Counter', () => {
test('increments count when button is clicked', async () => {
const wrapper = mount(Counter)
const button = wrapper.find('button')
const text = wrapper.find('p')
expect(text.text()).toContain('Count: 0')
await button.trigger('click')
expect(text.text()).toContain('Count: 1')
})
})
Testing in React
React also has a rich testing ecosystem:
- React Testing Library: A lightweight solution for testing React components
- Jest: Often used as the test runner and assertion library
- Enzyme: A JavaScript Testing utility for React (though less popular in recent years)
Example of a React component test:
import React from 'react'
import { render, fireEvent } from '@testing-library/react'
import Counter from './Counter'
test('increments count when button is clicked', () => {
const { getByText } = render(<Counter />)
const button = getByText('Increment')
expect(getByText('Count: 0')).toBeInTheDocument()
fireEvent.click(button)
expect(getByText('Count: 1')).toBeInTheDocument()
})
Testing Comparison
Both frameworks provide excellent testing capabilities:
- Vue’s testing utilities are more tightly integrated with the framework.
- React’s testing ecosystem is more diverse, with multiple popular libraries to choose from.
- Both support component testing, mocking, and snapshot testing.
- The choice of testing tools often comes down to personal preference and project requirements.
9. Server-Side Rendering (SSR) and Static Site Generation (SSG)
Server-Side Rendering and Static Site Generation are crucial for improving initial load times, SEO, and providing a better user experience for content-heavy websites. Both Vue and React offer solutions in this area.
Vue SSR and SSG
Nuxt.js is the go-to framework for server-side rendering and static site generation with Vue. It offers:
- Automatic code splitting
- Server-Side Rendering out of the box
- Static site generation
- Powerful routing system based on file-system
- Module ecosystem for extending functionality
Example of a Nuxt.js page:
<template>
<div>
<h1>{{ title }}</h1>
<p>{{ content }}</p>
</div>
</template>
<script>
export default {
async asyncData({ params }) {
const post = await fetchPost(params.id)
return { title: post.title, content: post.content }
}
}
</script>
React SSR and SSG
Next.js is the most popular framework for server-side rendering and static site generation with React. It provides:
- Hybrid static & server rendering
- Typescript support
- Intelligent bundling, route pre-fetching, and more
- Built-in CSS support
- API routes to build API endpoints with serverless functions
Example of a Next.js page:
import { GetServerSideProps } from 'next'
function Post({ title, content }) {
return (
<div>
<h1>{title}</h1>
<p>{content}</p>
</div>
)
}
export const getServerSideProps: GetServerSideProps = async (context) => {
const res = await fetch(`https://api.example.com/posts/${context.params.id}`)
const post = await res.json()
return {
props: { title: post.title, content: post.content },
}
}
export default Post
SSR and SSG Comparison
Both Nuxt.js and Next.js offer powerful features for SSR and SSG:
- Nuxt.js is more opinionated and follows Vue’s simplicity philosophy.
- Next.js offers more flexibility and has a larger ecosystem due to React’s popularity.
- Both frameworks have excellent performance and developer experience.
- The choice often depends on whether you prefer Vue or React as your base framework.
10. Mobile and Desktop Development
While both Vue and React are primarily web frameworks, they can be used to develop mobile and desktop applications as well.
Vue for Mobile and Desktop
- Quasar Framework: A Vue-based framework for building responsive websites, PWAs, SSR applications, desktop applications (using Electron), and mobile apps (using Cordova).
- Weex: A framework for building mobile cross-platform UIs, although it’s less actively maintained now.
- Capacitor: Can be used with Vue to build native iOS, Android, and PWA apps from a single codebase.
React for Mobile and Desktop
- React Native: A popular framework for building native mobile apps using React. It’s widely used and has a large ecosystem.
- Electron: Often used with React to build desktop applications.
- React Native Web: Allows you to use React Native components and APIs on the web.
Comparison
React has a clear advantage in mobile development with React Native, which is more mature and widely adopted than Vue’s mobile solutions. For desktop development, both frameworks can use Electron effectively.
11. Performance Optimization Techniques
Both Vue and React offer various techniques for optimizing performance in large-scale applications.
Vue Performance Optimization
- Keep-alive Component: Caches component instances that are toggled often.
- Lazy Loading Components: Load components only when needed.
- Virtual Scrolling: Render only visible items in long lists.
- Computed Properties: Cache and recompute values only when dependencies change.
Example of lazy loading in Vue:
const AsyncComponent = () => ({
component: import('./AsyncComponent.vue'),
loading: LoadingComponent,
error: ErrorComponent,
delay: 200,
timeout: 3000
})
React Performance Optimization
- React.memo: For preventing unnecessary re-renders of functional components.
- useMemo and useCallback Hooks: For memoizing values and functions.
- Code Splitting: Using dynamic imports to split your code into smaller chunks.
- Virtualization: Libraries like react-window for efficiently rendering large lists.
Example of using React.memo:
const MyComponent = React.memo(function MyComponent(props) {
/* render using props */
});
// Or with arrow function
const MyComponent = React.memo((props) => {
/* render using props */
});
12. Future Trends and Evolution
Both Vue and React are actively evolving to meet the changing needs of web development.
Vue’s Future
- Vue 3 introduced the Composition API, offering more flexible and powerful ways to organize component logic.
- Improved TypeScript support.
- Continued focus on developer experience and performance improvements.
React’s Future
- Concurrent Mode and Suspense for improved rendering capabilities.
- Server Components for better server-side rendering integration.
- Continued evolution of Hooks and functional components.
Conclusion
Choosing between Vue and React ultimately depends on your project requirements, team expertise, and personal preferences. Both frameworks are excellent choices for modern web development, offering robust ecosystems, strong performance, and active communities.
Vue excels in its simplicity and gentle learning curve, making it an excellent choice for smaller teams or projects where rapid development is crucial. Its official libraries and clear documentation provide a more guided experience.
React, with its larger ecosystem and community, offers more flexibility and is often preferred for large-scale applications. Its popularity ensures a wide range of third-party libraries and tools, as well as a larger pool of experienced developers.
Remember that the best framework is the one that allows your team to be most productive and delivers the best results for your specific project. Both Vue and React are capable of building high-performance, scalable web applications, and either choice can lead to successful outcomes when used effectively.