Utilizing Memoization in Dynamic Programming: A Comprehensive Guide
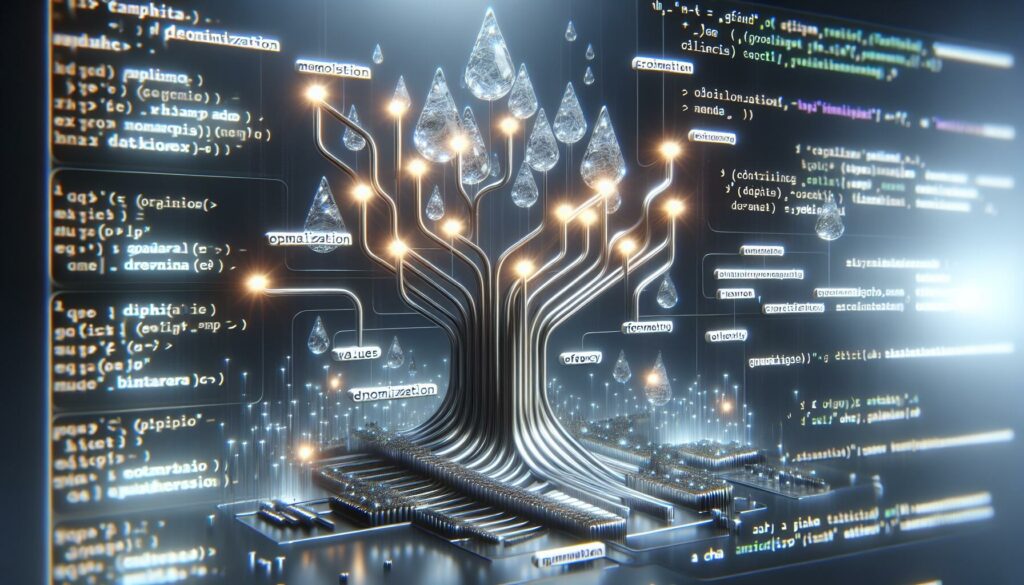
Dynamic programming is a powerful technique used in computer science and mathematics to solve complex problems by breaking them down into simpler subproblems. One of the key strategies employed in dynamic programming is memoization, which can significantly improve the efficiency of algorithms. In this comprehensive guide, we’ll explore the concept of memoization, its implementation in dynamic programming, and how it can be utilized to optimize your code.
What is Memoization?
Memoization is an optimization technique used primarily to speed up computer programs by storing the results of expensive function calls and returning the cached result when the same inputs occur again. It’s a way to avoid redundant computations and can dramatically improve the performance of algorithms that involve repetitive calculations.
The term “memoization” comes from the word “memorandum,” meaning “to be remembered.” In programming, it’s a way of remembering previous results to avoid unnecessary recalculation.
How Memoization Works in Dynamic Programming
In dynamic programming, memoization is typically implemented using a data structure (often an array or a hash table) to store the results of subproblems. When a problem is encountered, the algorithm first checks if the solution to this subproblem has already been computed and stored. If it has, the stored result is returned immediately, saving computation time. If not, the subproblem is solved, and its result is stored for future use.
This approach is particularly useful in problems with overlapping subproblems, where the same calculations would otherwise be performed multiple times.
Implementing Memoization: A Step-by-Step Guide
Let’s walk through the process of implementing memoization in a dynamic programming solution:
- Identify the problem: Determine if the problem has overlapping subproblems that could benefit from memoization.
- Choose a data structure: Select an appropriate data structure to store the results of subproblems. This is often an array or a hash table.
- Initialize the memoization structure: Set up the chosen data structure with initial values (often null or -1) to indicate uncalculated results.
- Modify the recursive function: Adapt your recursive function to check the memoization structure before performing calculations.
- Store results: After calculating a result, store it in the memoization structure before returning.
- Return memoized results: When a subproblem is encountered again, return the stored result instead of recalculating.
Example: Fibonacci Sequence with Memoization
Let’s illustrate the power of memoization by implementing the Fibonacci sequence calculator. First, we’ll look at a naive recursive implementation, and then we’ll optimize it using memoization.
Naive Recursive Implementation
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
console.log(fibonacci(40)); // This will take a long time to compute
This implementation is simple but highly inefficient for large values of n, as it recalculates the same Fibonacci numbers many times.
Memoized Implementation
function fibonacciMemo(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacciMemo(n - 1, memo) + fibonacciMemo(n - 2, memo);
return memo[n];
}
console.log(fibonacciMemo(40)); // This will compute much faster
In this memoized version, we use an object (memo) to store previously calculated Fibonacci numbers. This dramatically reduces the number of recursive calls and improves the time complexity from O(2^n) to O(n).
When to Use Memoization
Memoization is particularly useful in scenarios where:
- The problem has overlapping subproblems
- The function is pure (always produces the same output for the same input)
- The function is called with the same inputs multiple times
- The space complexity trade-off is acceptable
Common problems where memoization is effective include:
- Fibonacci sequence
- Factorial calculation
- Longest Common Subsequence
- Knapsack problem
- Matrix Chain Multiplication
Advanced Memoization Techniques
1. Top-Down vs. Bottom-Up Approach
Memoization is typically associated with the top-down approach in dynamic programming, where we start with the main problem and break it down into subproblems. However, it’s worth noting that there’s also a bottom-up approach, often referred to as tabulation.
Top-Down (Memoization):
- Starts with the main problem and recursively breaks it down
- Uses a recursive approach with memoization
- Calculates only the needed subproblems
Bottom-Up (Tabulation):
- Starts from the smallest subproblems and builds up to the main problem
- Uses an iterative approach
- Calculates all subproblems in a predetermined order
While both approaches can be effective, memoization (top-down) is often more intuitive and easier to implement, especially when transitioning from a recursive solution.
2. Memoization in Object-Oriented Programming
In object-oriented languages, you can implement memoization as a class decorator or within a class method. Here’s an example in Python:
class Memoize:
def __init__(self, fn):
self.fn = fn
self.memo = {}
def __call__(self, *args):
if args not in self.memo:
self.memo[args] = self.fn(*args)
return self.memo[args]
@Memoize
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(100)) # Computes quickly
This implementation uses a class to create a memoized version of any function, demonstrating how memoization can be generalized and reused across different problems.
3. Memoization with Limited Cache Size
In some cases, you might want to limit the size of your memoization cache to prevent excessive memory usage. This can be achieved using a Least Recently Used (LRU) cache. Here’s an example using Python’s functools module:
from functools import lru_cache
@lru_cache(maxsize=100)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(200)) # Computes quickly and efficiently
This implementation limits the cache to the 100 most recently used results, balancing memory usage with computational efficiency.
Common Pitfalls and How to Avoid Them
While memoization is a powerful technique, there are some common pitfalls to be aware of:
1. Excessive Memory Usage
Pitfall: Memoizing every possible input can lead to high memory consumption, especially for functions with a large input space.
Solution: Use bounded memoization techniques like LRU caching, or clear the memoization cache periodically if appropriate for your use case.
2. Memoizing Impure Functions
Pitfall: Memoizing functions that depend on external state or have side effects can lead to incorrect results.
Solution: Only apply memoization to pure functions that always return the same output for the same input.
3. Overhead for Simple Computations
Pitfall: For very simple or quick computations, the overhead of memoization might outweigh its benefits.
Solution: Profile your code and only apply memoization where it provides a significant performance improvement.
4. Incorrect Memoization Key
Pitfall: Using an incomplete or incorrect key for memoization can lead to cache misses or incorrect results.
Solution: Ensure that your memoization key captures all relevant input parameters that affect the function’s output.
Real-World Applications of Memoization
Memoization isn’t just a theoretical concept; it has numerous practical applications in software development:
1. Web Development
In web applications, memoization can be used to cache the results of expensive API calls or database queries. This can significantly improve response times and reduce server load.
const memoizedFetch = memoize(async (url) => {
const response = await fetch(url);
return response.json();
});
// Subsequent calls with the same URL will return cached results
memoizedFetch('https://api.example.com/data');
2. Graphics and Game Development
In computer graphics and game development, memoization can be used to cache the results of complex calculations, such as physics simulations or path-finding algorithms.
const memoizedPathfind = memoize((start, end, obstacles) => {
// Complex path-finding algorithm
// ...
});
// Cached for repeated calls with the same parameters
memoizedPathfind(playerPosition, goalPosition, levelObstacles);
3. Machine Learning
In machine learning, memoization can be used to cache the results of feature extraction or data preprocessing steps, speeding up training and inference processes.
const memoizedFeatureExtraction = memoize((rawData) => {
// Complex feature extraction process
// ...
});
// Faster repeated processing of the same raw data
memoizedFeatureExtraction(sensorData);
Memoization in Different Programming Languages
While the concept of memoization is language-agnostic, its implementation can vary across different programming languages. Let’s look at how memoization can be implemented in a few popular languages:
JavaScript
In JavaScript, we can use closures to create a memoized function:
function memoize(fn) {
const cache = {};
return function(...args) {
const key = JSON.stringify(args);
if (key in cache) {
return cache[key];
}
const result = fn.apply(this, args);
cache[key] = result;
return result;
}
}
const memoizedFactorial = memoize((n) => {
if (n <= 1) return 1;
return n * memoizedFactorial(n - 1);
});
console.log(memoizedFactorial(5)); // Computes and caches
console.log(memoizedFactorial(5)); // Returns cached result
Python
Python provides a built-in decorator for memoization in the functools module:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(100)) # Computes quickly
Java
In Java, we can use a HashMap to implement memoization:
import java.util.HashMap;
import java.util.Map;
public class Memoization {
private static Map<Integer, Integer> fibCache = new HashMap<>();
public static int fibonacci(int n) {
if (fibCache.containsKey(n)) {
return fibCache.get(n);
}
int result;
if (n < 2) {
result = n;
} else {
result = fibonacci(n - 1) + fibonacci(n - 2);
}
fibCache.put(n, result);
return result;
}
public static void main(String[] args) {
System.out.println(fibonacci(100)); // Computes quickly
}
}
Optimizing Memoization for Performance
While memoization itself is an optimization technique, there are ways to further enhance its performance:
1. Choose the Right Data Structure
The choice of data structure for your memoization cache can significantly impact performance. Hash tables (like objects in JavaScript or dictionaries in Python) are often a good choice due to their O(1) average-case lookup time. However, for certain problems, arrays might be more efficient.
2. Consider Space-Time Tradeoffs
Memoization trades space for time. In some cases, it might be beneficial to only cache results for inputs above a certain threshold, or to limit the cache size to prevent excessive memory usage.
3. Use Efficient Key Generation
For functions with multiple parameters, generating an efficient and unique key for the memoization cache is crucial. While JSON.stringify() is a common choice in JavaScript, it can be slow for large objects. Consider using a custom key generation function for better performance.
4. Leverage Language-Specific Optimizations
Many languages offer built-in or library functions for memoization that are highly optimized. For example, Python’s @lru_cache decorator is often more efficient than a custom implementation.
Memoization in Technical Interviews
Memoization is a popular topic in technical interviews, especially for positions at major tech companies. Here are some tips for discussing and implementing memoization in an interview setting:
1. Recognize Applicable Problems
Be on the lookout for problems with overlapping subproblems, such as recursive calculations or dynamic programming questions. Common examples include fibonacci sequences, climbing stairs problems, or coin change problems.
2. Start with the Naive Solution
Begin by implementing the straightforward, recursive solution. This demonstrates your problem-solving approach and provides a basis for optimization.
3. Identify Redundant Calculations
Explain how the naive solution leads to redundant calculations and how memoization can address this issue.
4. Implement Memoization
Show how you would modify the naive solution to include memoization. Be prepared to explain your choice of data structure for the cache.
5. Analyze Time and Space Complexity
Discuss how memoization affects both the time and space complexity of your solution. Be prepared to compare it with the naive approach.
6. Consider Edge Cases
Think about potential edge cases, such as very large inputs or inputs that might cause integer overflow.
Conclusion
Memoization is a powerful technique in the world of dynamic programming and algorithm optimization. By storing the results of expensive function calls and returning cached results when the same inputs occur again, memoization can dramatically improve the performance of algorithms that involve repetitive calculations.
As we’ve explored in this comprehensive guide, memoization has applications across various domains of computer science and software development, from web development to machine learning. It’s a crucial tool in a programmer’s toolkit, especially when dealing with recursive algorithms or problems with overlapping subproblems.
However, like any optimization technique, memoization comes with its own set of considerations. It’s important to understand when to apply memoization, how to implement it effectively, and how to avoid common pitfalls. By mastering these aspects, you can write more efficient code and solve complex problems more effectively.
As you continue your journey in coding education and programming skills development, remember that memoization is just one of many powerful techniques in the world of algorithms and data structures. Keep practicing, keep learning, and keep optimizing your code. Happy coding!