Using Visual Diagrams to Explain Your Approach in Interviews
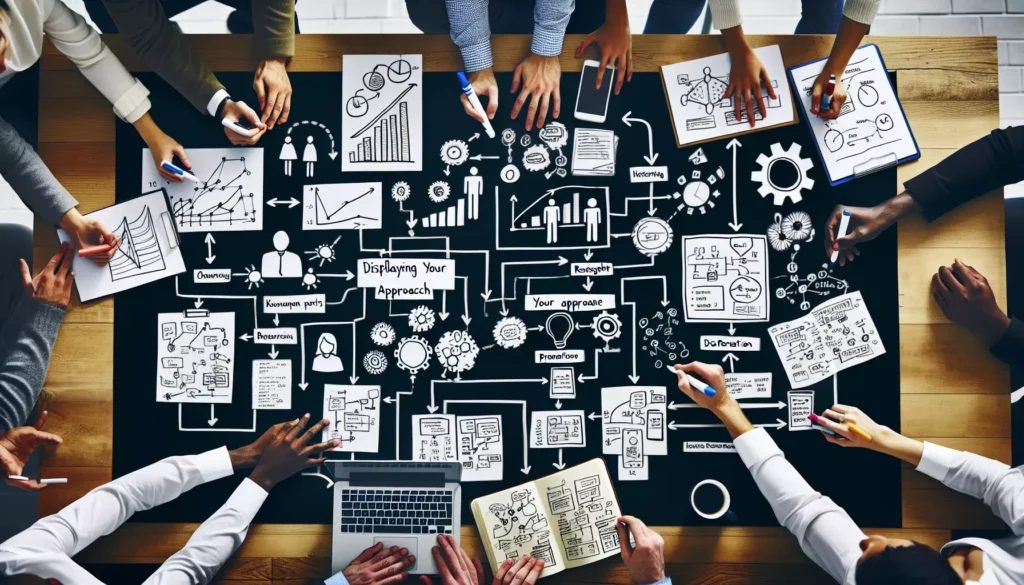
In the competitive world of technical interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), your ability to communicate complex ideas clearly and effectively can set you apart from other candidates. One powerful tool that is often overlooked in interview preparation is the use of visual diagrams to explain your approach to solving coding problems. This blog post will explore how incorporating visual aids into your interview responses can significantly enhance your performance and leave a lasting impression on your interviewers.
Why Visual Diagrams Matter in Technical Interviews
Before we dive into the specifics of using visual diagrams, let’s understand why they are so valuable in the context of a technical interview:
- Clarity of Thought: Diagrams help you organize your thoughts and present a clear, structured approach to problem-solving.
- Enhanced Communication: Visual aids can bridge the gap between your internal thought process and the interviewer’s understanding.
- Demonstration of Problem-Solving Skills: By visually breaking down a problem, you showcase your analytical abilities and methodical approach.
- Memory Aid: Diagrams can serve as a reference point throughout the interview, helping both you and the interviewer keep track of the discussion.
- Differentiation: Using visual aids can set you apart from other candidates who may rely solely on verbal explanations.
Types of Visual Diagrams for Coding Interviews
There are several types of diagrams that can be particularly useful in coding interviews. Let’s explore some of the most effective ones:
1. Flowcharts
Flowcharts are excellent for illustrating the logical flow of an algorithm or process. They use standardized symbols to represent different types of actions and decisions.
Example use case: Explaining the control flow of a sorting algorithm like quicksort.
<!-- Pseudo-HTML representation of a flowchart -->
<flowchart>
<start>Start</start>
<arrow></arrow>
<decision>Is array length > 1?</decision>
<arrow label="Yes"></arrow>
<process>Choose pivot</process>
<arrow></arrow>
<process>Partition array</process>
<arrow></arrow>
<process>Recursively sort left partition</process>
<arrow></arrow>
<process>Recursively sort right partition</process>
<arrow></arrow>
<end>End</end>
<arrow label="No" from="decision" to="end"></arrow>
</flowchart>
2. Data Structure Diagrams
These diagrams visually represent data structures like arrays, linked lists, trees, and graphs. They are invaluable when discussing problems that involve manipulating these structures.
Example use case: Illustrating a binary search tree and its operations.
<!-- Pseudo-HTML representation of a binary search tree -->
<tree>
<node value="8">
<left>
<node value="3">
<left>
<node value="1"></node>
</left>
<right>
<node value="6">
<left>
<node value="4"></node>
</left>
<right>
<node value="7"></node>
</right>
</node>
</right>
</node>
</left>
<right>
<node value="10">
<right>
<node value="14">
<left>
<node value="13"></node>
</left>
</node>
</right>
</node>
</right>
</node>
</tree>
3. State Diagrams
State diagrams are useful for illustrating how a system or algorithm transitions between different states. They’re particularly helpful when discussing finite state machines or explaining the lifecycle of an object.
Example use case: Describing the states of a traffic light system.
<!-- Pseudo-HTML representation of a state diagram -->
<state-diagram>
<state name="Red">
<transition to="Green" label="30 seconds"></transition>
</state>
<state name="Green">
<transition to="Yellow" label="25 seconds"></transition>
</state>
<state name="Yellow">
<transition to="Red" label="5 seconds"></transition>
</state>
</state-diagram>
4. Memory Diagrams
Memory diagrams help visualize how data is stored in memory. They’re particularly useful when discussing pointer operations, memory allocation, or explaining concepts like stack and heap.
Example use case: Illustrating how a linked list is stored in memory.
<!-- Pseudo-HTML representation of a memory diagram -->
<memory-diagram>
<address value="0x1000">
<data>5</data>
<pointer>0x1008</pointer>
</address>
<address value="0x1008">
<data>10</data>
<pointer>0x1016</pointer>
</address>
<address value="0x1016">
<data>15</data>
<pointer>NULL</pointer>
</address>
</memory-diagram>
5. Time Complexity Graphs
These graphs help visualize the performance characteristics of different algorithms. They’re particularly useful when discussing Big O notation and comparing algorithm efficiency.
Example use case: Comparing the time complexity of different sorting algorithms.
<!-- Pseudo-HTML representation of a time complexity graph -->
<graph>
<x-axis label="Input Size (n)"></x-axis>
<y-axis label="Time"></y-axis>
<curve label="O(n^2)" color="red"></curve>
<curve label="O(n log n)" color="blue"></curve>
<curve label="O(n)" color="green"></curve>
</graph>
How to Effectively Use Visual Diagrams in Interviews
Now that we’ve covered the types of diagrams you can use, let’s discuss how to incorporate them effectively into your interview responses:
1. Start with a Clear Problem Statement
Before diving into your diagram, clearly restate the problem to ensure you and the interviewer are on the same page. This sets the context for your visual explanation.
2. Choose the Right Type of Diagram
Select a diagram type that best fits the problem at hand. For example, use a flowchart for algorithmic processes, a data structure diagram for problems involving trees or graphs, or a memory diagram when discussing pointer operations.
3. Keep It Simple and Clear
Your diagram should be easy to understand at a glance. Use clear symbols, labels, and arrows. Avoid cluttering the diagram with unnecessary details.
4. Explain as You Draw
As you sketch your diagram, verbally explain each component. This helps the interviewer follow your thought process and demonstrates your ability to communicate complex ideas.
5. Use Color and Emphasis Sparingly
If you have access to colored markers, use them to highlight key parts of your diagram. However, don’t overdo it – use color strategically to draw attention to the most important elements.
6. Practice Beforehand
Like any skill, creating clear diagrams under pressure requires practice. Incorporate diagram creation into your problem-solving practice sessions.
7. Be Prepared to Iterate
Your initial diagram might not be perfect, and that’s okay. Be ready to modify or redraw parts of your diagram as you discuss the problem with the interviewer. This shows flexibility and willingness to refine your approach.
8. Connect the Diagram to Code
After explaining your approach visually, be prepared to translate your diagram into actual code. Show how each part of the diagram corresponds to specific code constructs or functions.
Common Pitfalls to Avoid
While visual diagrams can greatly enhance your interview performance, there are some common mistakes to avoid:
1. Overcomplicating the Diagram
A diagram that’s too complex can confuse rather than clarify. Keep your visuals simple and focused on the key aspects of the problem.
2. Neglecting Verbal Explanation
Don’t let the diagram speak for itself. Always provide a clear verbal explanation to accompany your visual aid.
3. Spending Too Much Time on the Diagram
While diagrams are helpful, remember that the interview is primarily about your problem-solving skills and code. Don’t spend an excessive amount of time perfecting your diagram at the expense of coding time.
4. Ignoring the Interviewer’s Cues
Pay attention to the interviewer’s reactions. If they seem confused or uninterested in your diagram, be prepared to switch to a different explanation method.
5. Using Diagrams for Every Question
Not every problem requires a visual explanation. Use diagrams judiciously, when they truly add value to your explanation.
Examples of Effective Diagram Usage in Common Interview Questions
Let’s look at some specific examples of how you might use diagrams to explain your approach to common coding interview questions:
1. Implementing a Binary Search Tree
For this problem, you could start with a data structure diagram showing a sample binary search tree. As you explain the insertion, deletion, and search operations, you can modify the diagram to show how the tree structure changes.
<!-- Pseudo-HTML representation of a binary search tree operation -->
<bst-operation>
<initial-tree>
<node value="8">
<left value="3"></left>
<right value="10"></right>
</node>
</initial-tree>
<insert value="6">
<step1>Compare with root (8)</step1>
<step2>Go left to 3</step2>
<step3>Insert as right child of 3</step3>
</insert>
<final-tree>
<node value="8">
<left value="3">
<right value="6"></right>
</left>
<right value="10"></right>
</node>
</final-tree>
</bst-operation>
2. Implementing a Graph Traversal Algorithm (e.g., BFS or DFS)
Here, you could draw a sample graph and use arrows to show the order in which nodes are visited. You can also use a separate queue or stack diagram to show how these data structures are used in the traversal process.
<!-- Pseudo-HTML representation of a graph traversal -->
<graph-traversal>
<graph>
<node id="A" connected-to="B,C"></node>
<node id="B" connected-to="D"></node>
<node id="C" connected-to="D,E"></node>
<node id="D" connected-to=""></node>
<node id="E" connected-to=""></node>
</graph>
<bfs-steps>
<step1>Visit A (add B, C to queue)</step1>
<step2>Visit B (add D to queue)</step2>
<step3>Visit C (add D, E to queue)</step3>
<step4>Visit D</step4>
<step5>Visit E</step5>
</bfs-steps>
</graph-traversal>
3. Explaining a Dynamic Programming Solution
For dynamic programming problems, you can use a table or grid to show how the solution is built up from smaller subproblems. This is particularly effective for problems like the knapsack problem or longest common subsequence.
<!-- Pseudo-HTML representation of a dynamic programming table -->
<dp-table problem="Fibonacci Sequence">
<row>
<cell>n</cell>
<cell>0</cell>
<cell>1</cell>
<cell>2</cell>
<cell>3</cell>
<cell>4</cell>
<cell>5</cell>
</row>
<row>
<cell>F(n)</cell>
<cell>0</cell>
<cell>1</cell>
<cell>1</cell>
<cell>2</cell>
<cell>3</cell>
<cell>5</cell>
</row>
</dp-table>
4. Illustrating a Divide and Conquer Algorithm
For algorithms like merge sort or quick sort, you can use a tree-like diagram to show how the problem is divided into smaller subproblems and then combined.
<!-- Pseudo-HTML representation of a merge sort process -->
<merge-sort>
<initial-array>[38, 27, 43, 3, 9, 82, 10]</initial-array>
<divide>
<left>[38, 27, 43, 3]</left>
<right>[9, 82, 10]</right>
</divide>
<subdivide>
<left>
<left>[38, 27]</left>
<right>[43, 3]</right>
</left>
<right>
<left>[9, 82]</left>
<right>[10]</right>
</right>
</subdivide>
<merge>
<step1>[27, 38] [3, 43] [9, 82] [10]</step1>
<step2>[3, 27, 38, 43] [9, 10, 82]</step2>
<final>[3, 9, 10, 27, 38, 43, 82]</final>
</merge>
</merge-sort>
Adapting to Different Interview Formats
While the principles of using visual diagrams remain the same, you may need to adapt your approach based on the interview format:
In-Person Interviews
In traditional in-person interviews, you’ll likely have access to a whiteboard or pen and paper. This is the ideal scenario for creating detailed diagrams. Remember to position yourself so that both you and the interviewer can easily see the diagram as you explain it.
Virtual Interviews
For virtual interviews, you may need to get creative:
- Digital Whiteboard Tools: Many video conferencing platforms offer built-in whiteboard features. Familiarize yourself with these tools before the interview.
- Screen Sharing: You can use simple drawing tools like Paint or more advanced diagramming software and share your screen as you create the diagram.
- Pen and Paper: In some cases, you might need to draw on paper and hold it up to the camera. Ensure you have good lighting and a stable setup for this.
- Verbal Descriptions: If visual aids are not possible, practice describing your diagrams verbally in a clear, structured manner.
Take-Home Assignments
For take-home coding assignments, you can include well-crafted diagrams in your documentation. This shows attention to detail and strong communication skills. Use digital tools to create clean, professional-looking diagrams.
Developing Your Diagramming Skills
Improving your ability to create effective diagrams is an ongoing process. Here are some tips to help you develop this skill:
1. Study Existing Diagrams
Analyze diagrams in computer science textbooks, research papers, and online resources. Pay attention to how complex concepts are simplified and represented visually.
2. Practice Regularly
Incorporate diagram creation into your regular problem-solving practice. Try to visualize and sketch out your approach before coding.
3. Seek Feedback
Show your diagrams to peers or mentors and ask for their input. What’s clear to you might not be immediately obvious to others.
4. Learn Basic Design Principles
Familiarize yourself with basic design concepts like hierarchy, alignment, and use of white space. These can make your diagrams more readable and professional.
5. Explore Diagramming Tools
While you may be limited to hand-drawn diagrams in most interviews, practicing with digital tools can help you understand different diagram types and layouts. Tools like Draw.io, Lucidchart, or even PowerPoint can be useful for this purpose.
Conclusion
Incorporating visual diagrams into your coding interview responses can significantly enhance your ability to communicate complex ideas and demonstrate your problem-solving skills. By clearly illustrating your thought process, you not only make it easier for interviewers to understand your approach but also showcase your ability to break down and analyze problems – a crucial skill in the tech industry.
Remember, the goal is not to create perfect, artistic diagrams, but to use visual aids as a tool to support and clarify your verbal explanations. With practice, you’ll become more comfortable and proficient at quickly sketching out relevant diagrams during interviews.
As you prepare for your next coding interview, particularly for positions at top tech companies, consider how you can incorporate visual explanations into your responses. This skill, combined with strong coding abilities and problem-solving skills, can give you a significant edge in the competitive world of technical interviews.
Keep practicing, stay curious, and don’t be afraid to pick up that marker and start drawing in your next interview. Your ability to visually communicate your ideas might just be the key that unlocks your next big career opportunity in the world of software development.