Using Real-World Analogies to Master Data Structures
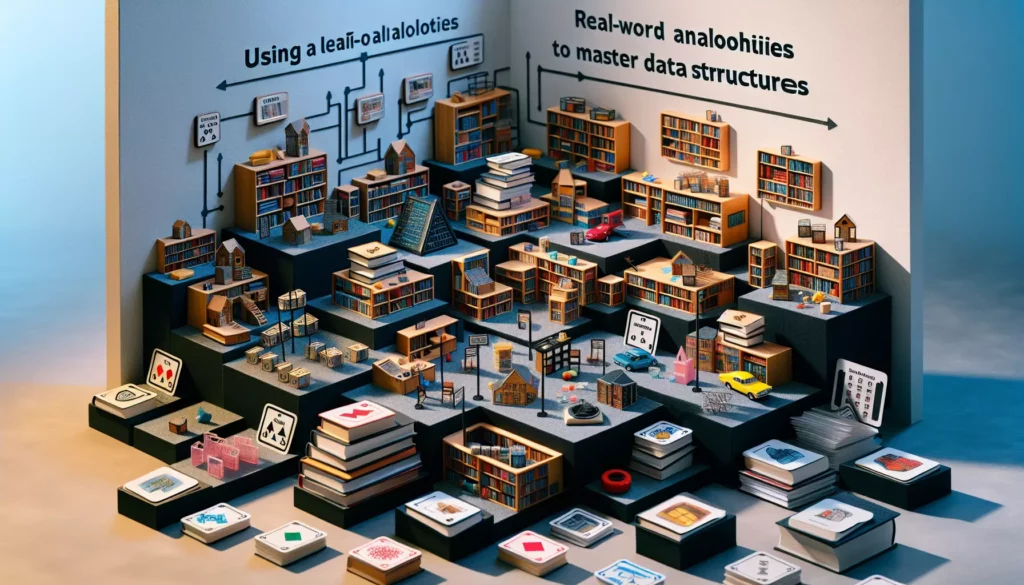
Data structures are the building blocks of efficient programming, but for many learners, they can seem abstract and challenging to grasp. At AlgoCademy, we believe that one of the most effective ways to understand and remember complex coding concepts is by relating them to familiar real-world objects and scenarios. In this comprehensive guide, we’ll explore how using analogies can help you master various data structures, making your journey from beginner to FAANG-ready developer smoother and more intuitive.
Why Analogies Work in Learning Data Structures
Before we dive into specific analogies, let’s understand why this approach is so powerful:
- Relatability: Analogies connect abstract concepts to concrete, everyday experiences.
- Memorability: It’s easier to recall information when it’s linked to familiar ideas.
- Visualization: Real-world comparisons help create mental images of how data structures function.
- Simplification: Complex ideas become more digestible when broken down into relatable parts.
Now, let’s explore some common data structures and their real-world counterparts.
1. Arrays: The Bookshelf Analogy
Imagine a bookshelf in your room. This bookshelf is a perfect analogy for an array in programming.
Key Points:
- Fixed Size: Like a bookshelf with a set number of slots, arrays have a predetermined size.
- Ordered Storage: Books on a shelf are arranged in a specific order, just as elements in an array have indexed positions.
- Direct Access: You can quickly grab the 5th book from the left, similar to accessing an array element by its index.
- Homogeneous Content: A bookshelf typically contains only books, mirroring how arrays store elements of the same data type.
Code Example:
// Creating an array (bookshelf) of 5 books
String[] bookshelf = new String[5];
// Adding books to the shelf
bookshelf[0] = "Harry Potter";
bookshelf[1] = "Lord of the Rings";
bookshelf[2] = "The Hobbit";
bookshelf[3] = "1984";
bookshelf[4] = "To Kill a Mockingbird";
// Accessing the third book (index 2)
System.out.println("The third book is: " + bookshelf[2]);
2. Linked Lists: The Train Analogy
Think of a linked list as a train, where each train car represents a node in the list.
Key Points:
- Dynamic Size: Trains can add or remove cars easily, just as linked lists can grow or shrink dynamically.
- Sequential Access: To reach a specific car, you must go through all the preceding cars, similar to traversing a linked list.
- Connections: Each train car is connected to the next, mirroring how each node in a linked list points to the next node.
- Insertion and Deletion: Adding or removing a train car (node) only affects its immediate neighbors, not the entire train (list).
Code Example:
class TrainCar {
String cargo;
TrainCar next;
TrainCar(String cargo) {
this.cargo = cargo;
this.next = null;
}
}
// Creating a train (linked list)
TrainCar engine = new TrainCar("Engine");
engine.next = new TrainCar("Coal");
engine.next.next = new TrainCar("Passengers");
engine.next.next.next = new TrainCar("Freight");
// Traversing the train
TrainCar current = engine;
while (current != null) {
System.out.println(current.cargo);
current = current.next;
}
3. Stacks: The Plate Stack Analogy
A stack in programming is very similar to a stack of plates in your kitchen cupboard.
Key Points:
- Last In, First Out (LIFO): The last plate you put on top is the first one you’ll take off.
- Push and Pop: Adding a plate is like “pushing” onto the stack, removing is like “popping” off the stack.
- Limited Access: You can only interact with the top plate, just as you can only access the top element of a stack.
- Size Tracking: You can easily see how many plates are in the stack, similar to checking a stack’s size in programming.
Code Example:
import java.util.Stack;
Stack<String> plateStack = new Stack<>();
// Pushing plates onto the stack
plateStack.push("Blue Plate");
plateStack.push("Red Plate");
plateStack.push("Green Plate");
// Popping the top plate
String topPlate = plateStack.pop();
System.out.println("Removed: " + topPlate);
// Peeking at the new top plate
System.out.println("Top plate now: " + plateStack.peek());
// Checking the stack size
System.out.println("Plates left: " + plateStack.size());
4. Queues: The Ticket Line Analogy
A queue in programming works just like a line of people waiting to buy tickets at a movie theater.
Key Points:
- First In, First Out (FIFO): The first person in line gets served first, just as the first element added to a queue is the first to be removed.
- Enqueue and Dequeue: Joining the line is like “enqueuing”, while being served and leaving is like “dequeuing”.
- Front and Rear: There’s a clear front (first person) and rear (last person) of the line, similar to a queue’s structure.
- Order Preservation: People can’t cut in line, maintaining the order of elements in a queue.
Code Example:
import java.util.LinkedList;
import java.util.Queue;
Queue<String> ticketLine = new LinkedList<>();
// People joining the line (enqueue)
ticketLine.offer("Alice");
ticketLine.offer("Bob");
ticketLine.offer("Charlie");
// Serving people (dequeue)
String firstPerson = ticketLine.poll();
System.out.println("Served: " + firstPerson);
// Checking who's next in line
System.out.println("Next in line: " + ticketLine.peek());
// Checking line length
System.out.println("People still in line: " + ticketLine.size());
5. Trees: The Family Tree Analogy
A tree data structure closely resembles a family tree or an organizational chart.
Key Points:
- Hierarchy: Just as a family has ancestors and descendants, trees have parent and child nodes.
- Root: The topmost person (e.g., great-grandparent) is like the root node of the tree.
- Branches and Leaves: Family lines branch out, with the youngest generation as “leaf” nodes.
- Relationships: Siblings, cousins, and other relationships in a family tree mirror the connections between nodes in a tree structure.
Code Example:
class FamilyMember {
String name;
FamilyMember leftChild;
FamilyMember rightChild;
FamilyMember(String name) {
this.name = name;
this.leftChild = null;
this.rightChild = null;
}
}
// Creating a family tree
FamilyMember grandparent = new FamilyMember("Grandpa Joe");
grandparent.leftChild = new FamilyMember("Uncle Bob");
grandparent.rightChild = new FamilyMember("Mom");
grandparent.rightChild.leftChild = new FamilyMember("Me");
grandparent.rightChild.rightChild = new FamilyMember("Sister");
// Function to print family members
void printFamily(FamilyMember member) {
if (member != null) {
System.out.println(member.name);
printFamily(member.leftChild);
printFamily(member.rightChild);
}
}
// Print the family tree
printFamily(grandparent);
6. Graphs: The Social Network Analogy
A graph data structure is perfectly represented by a social network of friends.
Key Points:
- Nodes and Edges: Each person is a node, and friendships are the edges connecting them.
- Directed vs Undirected: Some relationships might be one-way (directed) like following on Twitter, while others are mutual (undirected) like Facebook friendships.
- Weighted Edges: The strength of friendships can represent weighted edges in a graph.
- Connectivity: The concept of “friends of friends” illustrates how nodes in a graph are interconnected.
Code Example:
import java.util.*;
class Person {
String name;
List<Person> friends;
Person(String name) {
this.name = name;
this.friends = new ArrayList<>();
}
void addFriend(Person friend) {
friends.add(friend);
friend.friends.add(this); // For undirected graph
}
}
// Creating a social network
Person alice = new Person("Alice");
Person bob = new Person("Bob");
Person charlie = new Person("Charlie");
Person david = new Person("David");
alice.addFriend(bob);
alice.addFriend(charlie);
bob.addFriend(david);
charlie.addFriend(david);
// Function to print friends
void printFriends(Person person) {
System.out.print(person.name + "'s friends: ");
for (Person friend : person.friends) {
System.out.print(friend.name + " ");
}
System.out.println();
}
// Print everyone's friends
printFriends(alice);
printFriends(bob);
printFriends(charlie);
printFriends(david);
7. Hash Tables: The Library Catalog Analogy
A hash table can be likened to a library’s card catalog system.
Key Points:
- Key-Value Pairs: Each book (value) is associated with a unique call number (key).
- Fast Lookup: You can quickly find a book’s location using its call number, similar to accessing data in a hash table.
- Collisions: Sometimes two books might have similar call numbers, requiring additional organization, just like collision resolution in hash tables.
- Efficient Storage: Books are distributed across many shelves for easy access, mirroring how hash tables distribute data for efficient retrieval.
Code Example:
import java.util.HashMap;
// Creating a library catalog (hash table)
HashMap<String, String> libraryCatalog = new HashMap<>();
// Adding books to the catalog
libraryCatalog.put("823.8 AUS", "Pride and Prejudice");
libraryCatalog.put("813.5 ORW", "1984");
libraryCatalog.put("813.5 FIT", "The Great Gatsby");
// Looking up a book
String bookTitle = libraryCatalog.get("813.5 ORW");
System.out.println("Book found: " + bookTitle);
// Checking if a book exists
boolean hasBook = libraryCatalog.containsKey("823.8 AUS");
System.out.println("'Pride and Prejudice' in catalog: " + hasBook);
// Removing a book
libraryCatalog.remove("813.5 FIT");
// Printing all books in the catalog
for (String callNumber : libraryCatalog.keySet()) {
System.out.println(callNumber + ": " + libraryCatalog.get(callNumber));
}
Applying Analogies in Your Learning Journey
As you progress through your coding education with AlgoCademy, keep these analogies in mind and try to create your own. Here are some tips to make the most of this approach:
- Visualize the Analogy: Create mental images or even simple drawings to reinforce the connections between the data structure and its real-world counterpart.
- Explain to Others: Try explaining these concepts to friends or fellow learners using the analogies. Teaching others solidifies your own understanding.
- Extend the Analogies: As you learn more complex operations on data structures, try to extend these analogies to cover those scenarios.
- Create Your Own: Come up with personal analogies that resonate with your experiences and interests. The more personal the analogy, the more memorable it becomes.
- Practice Implementation: Use these analogies as a starting point to implement the data structures in code. This bridges the gap between conceptual understanding and practical application.
Conclusion
Understanding data structures is crucial for becoming a proficient programmer, especially when preparing for technical interviews at top tech companies. By leveraging real-world analogies, you can create strong mental models of these abstract concepts, making them easier to understand, remember, and apply in your coding projects.
At AlgoCademy, we encourage this approach as part of our comprehensive learning strategy. Combined with our interactive coding tutorials, AI-powered assistance, and step-by-step guidance, these analogies will help you build a solid foundation in data structures and algorithms.
Remember, the journey from beginner to FAANG-ready developer is a marathon, not a sprint. Take your time to truly understand each concept, and don’t hesitate to revisit these analogies whenever you need a refresher. Happy coding, and may your data structures always be as organized as a well-maintained library catalog!