Using Memoization to Improve Recursive Solutions
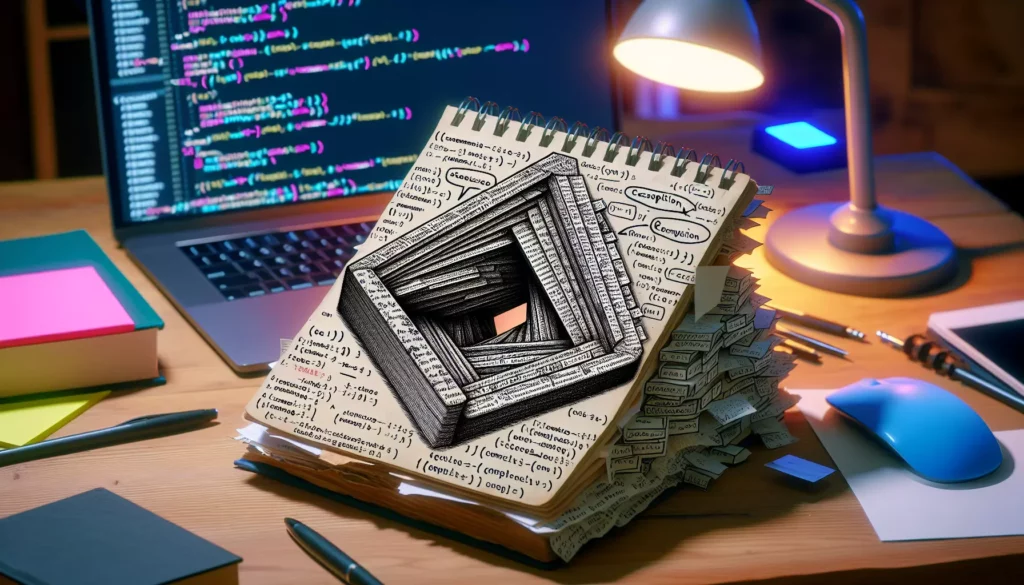
When it comes to solving complex programming problems, recursion is often a powerful and elegant approach. However, recursive solutions can sometimes be inefficient, especially when dealing with overlapping subproblems. This is where memoization comes to the rescue. In this comprehensive guide, we’ll explore how memoization can significantly improve the performance of recursive solutions, making them more efficient and practical for real-world applications.
What is Memoization?
Memoization is an optimization technique used primarily to speed up computer programs by storing the results of expensive function calls and returning the cached result when the same inputs occur again. It’s a form of caching that can dramatically improve the performance of algorithms that have overlapping subproblems or repeated calculations.
The term “memoization” comes from the word “memorandum,” meaning “to be remembered.” In the context of computer science, it refers to remembering the results of previous computations to avoid redundant calculations.
Why Use Memoization with Recursion?
Recursive solutions, while often elegant and intuitive, can suffer from performance issues due to repeated calculations. Let’s consider a classic example: the Fibonacci sequence.
The Fibonacci Sequence: A Classic Example
The Fibonacci sequence is defined as follows:
- F(0) = 0
- F(1) = 1
- F(n) = F(n-1) + F(n-2) for n > 1
A simple recursive implementation of the Fibonacci sequence might look like this:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
While this implementation is straightforward and mirrors the mathematical definition, it’s extremely inefficient for larger values of n. The reason? It recalculates the same values multiple times.
For example, to calculate fibonacci(5), the function will calculate fibonacci(4) and fibonacci(3). But to calculate fibonacci(4), it will again calculate fibonacci(3) and fibonacci(2), and so on. This leads to an exponential time complexity of O(2^n), which becomes impractical for even moderately large values of n.
Implementing Memoization
Now, let’s see how we can use memoization to improve our Fibonacci function:
def fibonacci_memoized(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memoized(n-1, memo) + fibonacci_memoized(n-2, memo)
return memo[n]
In this memoized version:
- We use a dictionary (memo) to store previously computed results.
- Before computing a value, we check if it’s already in our memo.
- If it is, we return the stored value instead of recomputing it.
- If it’s not, we compute it and store the result in the memo before returning.
This simple change reduces the time complexity from O(2^n) to O(n), a dramatic improvement that makes the function practical for much larger values of n.
The Benefits of Memoization
Memoization offers several key benefits when applied to recursive solutions:
- Improved Performance: By avoiding redundant calculations, memoized solutions can be orders of magnitude faster than their non-memoized counterparts.
- Reduced Time Complexity: Memoization can often reduce the time complexity of algorithms from exponential to polynomial time.
- Preserved Readability: Memoization allows us to maintain the clarity and intuition of recursive solutions while dramatically improving their efficiency.
- Space-Time Tradeoff: Memoization trades space for time, using additional memory to store results and save computation time.
When to Use Memoization
Memoization is particularly useful in scenarios where:
- Overlapping Subproblems: The problem can be broken down into subproblems, and these subproblems are solved multiple times.
- Expensive Computations: The function calls are computationally expensive, making redundant calculations costly.
- Pure Functions: The function’s output depends solely on its inputs, without side effects.
- Frequent Repeated Inputs: The function is likely to be called multiple times with the same inputs.
Real-World Applications of Memoization
Memoization isn’t just a theoretical concept; it has practical applications in various domains:
1. Dynamic Programming
Many dynamic programming problems benefit from memoization. Examples include:
- Longest Common Subsequence
- Knapsack Problem
- Matrix Chain Multiplication
2. Graph Algorithms
Certain graph algorithms can use memoization to avoid revisiting nodes:
- Depth-First Search (DFS) in certain scenarios
- Shortest Path Algorithms
3. Computer Graphics
In computer graphics, memoization can be used to cache expensive rendering calculations:
- Texture mapping
- Ray tracing
4. Web Development
In web applications, memoization can improve performance by caching expensive computations:
- API result caching
- Complex UI calculations
Implementing Memoization in Different Languages
While the concept of memoization is language-agnostic, the implementation can vary slightly across different programming languages. Let’s look at how to implement memoization in a few popular languages:
Python
In Python, we can use the @functools.lru_cache
decorator for a simple memoization implementation:
import functools
@functools.lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
JavaScript
In JavaScript, we can create a memoize function:
function memoize(fn) {
const cache = {};
return function(...args) {
const key = JSON.stringify(args);
if (key in cache) {
return cache[key];
}
const result = fn.apply(this, args);
cache[key] = result;
return result;
}
}
const fibonacci = memoize(function(n) {
if (n < 2) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
});
Java
In Java, we can use a HashMap for memoization:
import java.util.HashMap;
import java.util.Map;
public class Fibonacci {
private static Map<Integer, Integer> memo = new HashMap<>();
public static int fibonacci(int n) {
if (memo.containsKey(n)) {
return memo.get(n);
}
int result;
if (n < 2) {
result = n;
} else {
result = fibonacci(n - 1) + fibonacci(n - 2);
}
memo.put(n, result);
return result;
}
}
Advanced Memoization Techniques
While basic memoization is powerful, there are advanced techniques that can further optimize your code:
1. Partial Memoization
Sometimes, memoizing every function call isn’t necessary. Partial memoization involves caching only specific inputs or ranges of inputs that are known to be computationally expensive or frequently used.
2. Memoization with Expiration
In scenarios where data might become stale, implementing memoization with an expiration time can be useful. This ensures that cached results are refreshed periodically.
3. Memoization in Multithreaded Environments
When working with multiple threads, care must be taken to ensure thread-safety in memoized functions. This might involve using thread-safe data structures or synchronization mechanisms.
4. Memoization with Limited Cache Size
To prevent memory issues in long-running applications, implementing a cache with a maximum size and an eviction policy (like Least Recently Used) can be beneficial.
Common Pitfalls and How to Avoid Them
While memoization is a powerful technique, it’s not without its pitfalls. Here are some common issues and how to avoid them:
1. Excessive Memory Usage
Problem: Memoizing every function call can lead to high memory usage, especially for functions with many possible inputs.
Solution: Implement a cache with a maximum size or use partial memoization for only the most expensive calculations.
2. Memoizing Impure Functions
Problem: Memoizing functions with side effects or that depend on external state can lead to incorrect results.
Solution: Only memoize pure functions whose output depends solely on their inputs.
3. Overhead for Simple Calculations
Problem: For very simple calculations, the overhead of memoization might outweigh its benefits.
Solution: Use memoization judiciously, focusing on computationally expensive functions.
4. Stale Data in Long-Running Applications
Problem: In long-running applications, memoized results might become outdated if the underlying data changes.
Solution: Implement memoization with expiration or provide a way to invalidate the cache when necessary.
Memoization vs. Other Optimization Techniques
While memoization is a powerful optimization technique, it’s not the only tool in a developer’s arsenal. Let’s compare it with some other common optimization techniques:
Memoization vs. Tabulation
Memoization (Top-Down):
- Recursively solves problems and caches results
- Lazy evaluation – only computes needed values
- Can be easier to implement for some problems
- May have stack overflow issues for deeply recursive problems
Tabulation (Bottom-Up):
- Iteratively builds up the solution
- Computes all values in the defined range
- Often more space-efficient
- Avoids recursion-related issues like stack overflow
Memoization vs. Dynamic Programming
Memoization is actually a specific technique used in dynamic programming. Dynamic programming is a broader method for solving complex problems by breaking them down into simpler subproblems. It can be implemented using either memoization (top-down) or tabulation (bottom-up) approaches.
Memoization vs. Caching
While similar, memoization and caching have some distinctions:
- Memoization typically caches all results automatically
- Caching often involves more manual control over what gets cached and for how long
- Caching is a broader concept that can apply to various types of data, not just function results
Testing and Benchmarking Memoized Functions
When implementing memoization, it’s crucial to verify that it’s actually improving performance. Here are some strategies for testing and benchmarking memoized functions:
1. Unit Testing
Ensure that your memoized function produces the same results as the original function for a variety of inputs. Here’s a simple example using Python’s unittest framework:
import unittest
class TestFibonacci(unittest.TestCase):
def test_fibonacci(self):
test_cases = [(0, 0), (1, 1), (2, 1), (5, 5), (10, 55)]
for n, expected in test_cases:
with self.subTest(n=n):
self.assertEqual(fibonacci(n), expected)
self.assertEqual(fibonacci_memoized(n), expected)
if __name__ == '__main__':
unittest.main()
2. Performance Testing
Measure the execution time of your original and memoized functions for various inputs. Here’s a simple timing function in Python:
import time
def time_function(func, *args):
start = time.time()
result = func(*args)
end = time.time()
return result, end - start
# Usage
result, time_taken = time_function(fibonacci, 30)
print(f"Result: {result}, Time: {time_taken:.6f} seconds")
3. Profiling
Use profiling tools to get a detailed breakdown of where time is spent in your functions. In Python, you can use the cProfile module:
import cProfile
cProfile.run('fibonacci(30)')
cProfile.run('fibonacci_memoized(30)')
4. Memory Usage
Monitor memory usage, especially for large inputs or long-running processes. In Python, you can use the memory_profiler package:
from memory_profiler import profile
@profile
def fibonacci_memoized(n, memo={}):
# ... (function implementation)
fibonacci_memoized(100)
Conclusion
Memoization is a powerful technique that can significantly improve the performance of recursive solutions, especially those with overlapping subproblems. By caching the results of expensive function calls, memoization can transform exponential-time algorithms into linear-time ones, making previously impractical solutions viable for real-world use.
However, like any optimization technique, memoization should be applied judiciously. It’s most effective when dealing with pure functions that have expensive computations and are likely to be called multiple times with the same inputs. When implemented correctly, memoization can provide substantial performance benefits while maintaining the clarity and elegance of recursive solutions.
As you continue to develop your programming skills, remember that memoization is just one tool in your optimization toolkit. The key is to understand when and how to apply it effectively, always considering the specific requirements and constraints of your particular problem.
By mastering techniques like memoization, you’ll be better equipped to tackle complex programming challenges, optimize your code, and create more efficient and scalable solutions. Whether you’re preparing for technical interviews at top tech companies or working on real-world projects, the ability to recognize opportunities for memoization and implement it effectively will be a valuable skill in your programming arsenal.