Using Git Submodules for Large-Scale Projects: A Comprehensive Guide
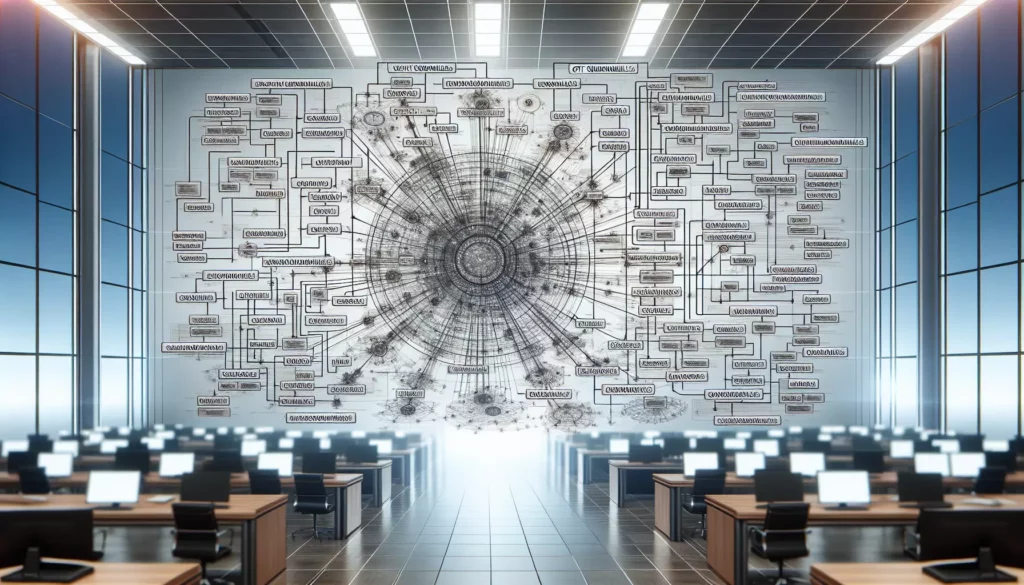
As software projects grow in complexity and scale, managing dependencies and organizing code becomes increasingly challenging. Git submodules offer a powerful solution for handling large-scale projects by allowing you to include and manage external repositories within your main project. In this comprehensive guide, we’ll explore the ins and outs of Git submodules, their benefits, and how to effectively use them in your development workflow.
Table of Contents
- What are Git Submodules?
- Benefits of Using Submodules
- Creating and Adding Submodules
- Cloning a Project with Submodules
- Updating Submodules
- Working with Submodules
- Best Practices for Using Submodules
- Alternatives to Submodules
- Conclusion
1. What are Git Submodules?
Git submodules are a feature that allows you to include one Git repository as a subdirectory within another Git repository. This means you can keep a Git repository as a subdirectory of another Git repository, enabling you to manage multiple repositories as a single project while keeping them separate.
Submodules are particularly useful when you want to:
- Include third-party libraries or frameworks in your project
- Share common code across multiple projects
- Separate large codebases into smaller, manageable pieces
- Maintain different versions of dependencies for different branches
Each submodule is essentially a pointer to a specific commit in the external repository. This allows you to lock the submodule to a particular version, ensuring consistency across your project and team.
2. Benefits of Using Submodules
Using Git submodules in large-scale projects offers several advantages:
- Code Reusability: Submodules allow you to reuse code across multiple projects without duplicating it. This is particularly useful for shared libraries or components.
- Version Control: Each submodule has its own version history, allowing you to manage and track changes independently from the main project.
- Flexibility: You can easily update submodules to newer versions or roll back to previous versions as needed.
- Modularity: Submodules promote a modular approach to software development, making it easier to maintain and scale large projects.
- Collaboration: Team members can work on different submodules independently, reducing conflicts and improving workflow efficiency.
- Dependency Management: Submodules provide a way to manage external dependencies directly within your Git repository.
3. Creating and Adding Submodules
To add a submodule to your Git repository, you use the git submodule add
command. Here’s how to do it:
git submodule add <repository-url> <path>
For example, to add a library called “awesome-lib” as a submodule in a “lib” directory:
git submodule add https://github.com/example/awesome-lib.git lib/awesome-lib
This command does several things:
- Clones the specified repository into the given path
- Adds the submodule to the
.gitmodules
file - Stages the
.gitmodules
file and the submodule directory
After adding the submodule, you need to commit these changes:
git commit -m "Add awesome-lib submodule"
4. Cloning a Project with Submodules
When you clone a repository that contains submodules, the submodule directories are initially empty. You need to initialize and update the submodules to populate them with the correct content. There are two ways to do this:
Method 1: Clone and then initialize submodules
git clone <repository-url>
cd <repository-name>
git submodule init
git submodule update
Method 2: Clone with submodules in one command
git clone --recurse-submodules <repository-url>
The second method is more convenient as it automatically initializes and updates the submodules during the cloning process.
5. Updating Submodules
Submodules don’t automatically track the latest changes in their respective repositories. To update a submodule to its latest commit, you can use the following commands:
cd <submodule-directory>
git fetch
git merge origin/master
# Or, to update all submodules at once from the root of your project:
git submodule update --remote
After updating the submodules, you need to commit these changes in your main repository:
git add <submodule-directory>
git commit -m "Update submodule to latest version"
6. Working with Submodules
When working with submodules, it’s important to understand a few key concepts and operations:
Checking out a specific version of a submodule
You can checkout a specific commit or tag in a submodule:
cd <submodule-directory>
git checkout <commit-hash-or-tag>
# Then, in the main repository:
git add <submodule-directory>
git commit -m "Update submodule to specific version"
Making changes in a submodule
If you need to make changes to a submodule, you should:
- Create a new branch in the submodule
- Make and commit your changes
- Push the changes to the submodule’s remote repository
- Update the main repository to point to the new commit
cd <submodule-directory>
git checkout -b new-feature
# Make changes
git add .
git commit -m "Implement new feature"
git push origin new-feature
# In the main repository
git add <submodule-directory>
git commit -m "Update submodule with new feature"
git push
Removing a submodule
To remove a submodule, you need to:
git submodule deinit <submodule-path>
git rm <submodule-path>
rm -rf .git/modules/<submodule-path>
Then commit the changes:
git commit -m "Remove submodule"
7. Best Practices for Using Submodules
To effectively use Git submodules in large-scale projects, consider the following best practices:
- Use specific commits or tags: Always point your submodules to specific commits or tags rather than branches. This ensures consistency and reproducibility across your project.
- Document submodule usage: Clearly document how submodules are used in your project, including initialization and update procedures.
- Regularly update submodules: Keep your submodules up-to-date to benefit from bug fixes and new features. However, be cautious and test thoroughly after updates.
- Avoid nested submodules: While possible, nested submodules can become complex to manage. Try to keep your submodule structure as flat as possible.
- Use shallow clones for large submodules: If a submodule has a large history, consider using shallow clones to reduce clone times and save disk space.
- Communicate changes: When updating submodules, communicate changes to your team to ensure everyone is aware and can update their local copies.
- Use CI/CD to validate submodule integrity: Implement checks in your CI/CD pipeline to ensure submodules are correctly initialized and up-to-date.
8. Alternatives to Submodules
While Git submodules are powerful, they may not always be the best solution for every project. Here are some alternatives to consider:
Git Subtrees
Git subtrees allow you to include the contents of one repository within another. Unlike submodules, subtrees don’t require special commands to clone or update, making them easier for team members unfamiliar with submodules.
Pros of Git Subtrees:
- Simpler for contributors who don’t need to know about the subtree
- All code is available in the main repository
- No need for additional steps when cloning the repository
Cons of Git Subtrees:
- More complex to set up and manage compared to submodules
- Can lead to large repository sizes
- Harder to track the origin of code from subtrees
Package Managers
For many projects, especially those involving third-party libraries, using a package manager (like npm for JavaScript, pip for Python, or Maven for Java) might be a more appropriate solution.
Pros of Package Managers:
- Easier dependency management
- Widely used and understood by developers
- Can handle version conflicts more gracefully
Cons of Package Managers:
- Less control over the exact version of the code
- May require additional setup for private packages
- Can introduce security risks if not properly managed
Monorepos
A monorepo is a single repository containing multiple projects or components. This approach can be an alternative to using submodules for large-scale projects.
Pros of Monorepos:
- Simplified dependency management
- Easier to make atomic changes across multiple projects
- Encourages code sharing and reuse
Cons of Monorepos:
- Can become very large and slow to clone
- May require specialized tools for efficient management
- Can be overkill for smaller projects or teams
9. Conclusion
Git submodules are a powerful tool for managing large-scale projects, offering a way to include external repositories and maintain complex codebases efficiently. By allowing you to treat sub-projects as separate entities while still integrating them into a larger project, submodules provide flexibility and modularity that can greatly benefit development teams.
However, like any tool, submodules come with their own set of challenges and complexities. They require a good understanding of Git and careful management to use effectively. When implemented correctly and following best practices, submodules can significantly improve code organization, reusability, and version control in large-scale projects.
As you consider using submodules in your projects, remember to weigh the benefits against the alternatives and choose the approach that best fits your team’s needs and workflow. Whether you opt for submodules, subtrees, package managers, or monorepos, the key is to establish clear processes and ensure that all team members understand how to work with the chosen system.
By mastering Git submodules and understanding their place in the broader context of dependency management and code organization, you’ll be well-equipped to tackle the challenges of large-scale software development. As you apply these concepts in real-world projects, you’ll develop a deeper appreciation for the power and flexibility that Git and its advanced features provide in managing complex codebases.