Unlocking the Power of React Hooks: A Comprehensive Guide for Developers
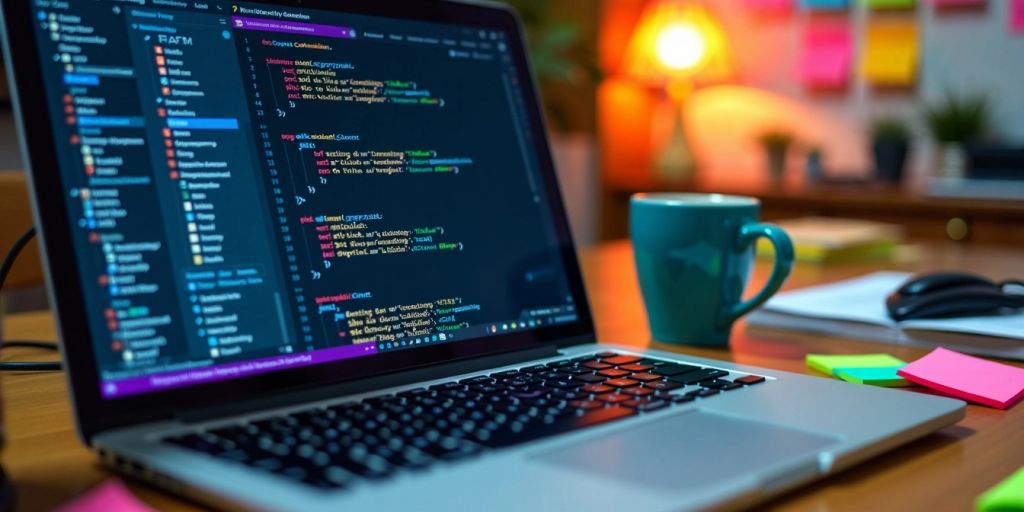
React Hooks have changed how developers create applications with React, making it easier to manage state and side effects. In this guide, we’ll break down what React Hooks are, how they work, and why they’re important. Whether you’re new to React or looking to enhance your skills, this guide will help you understand and use React Hooks effectively.
Key Takeaways
- React Hooks allow you to manage state and side effects in functional components.
- The useState hook is essential for adding state to your components easily.
- The useEffect hook helps you perform tasks like data fetching and updating the DOM.
- Custom Hooks let you create reusable logic that can be shared across components.
- Understanding best practices for Hooks can improve your code’s performance and readability.
Introduction to React Hooks
React has changed the way we build web applications, and React Hooks are a big part of that change. They were introduced in React 16.8 to help developers manage state and side effects in functional components. This guide will help you understand the basics of React Hooks and why they are so useful.
What Are React Hooks?
React Hooks are special functions that let you use state and other React features without writing a class. They make it easier to manage state and side effects in your components. Here are some key points about React Hooks:
- Simplified State Management: Hooks like
useState
allow you to add state to functional components easily. - Cleaner Code: Hooks help you write less code and make it more readable.
- Reusability: You can create custom hooks to share logic between components.
History and Evolution of React Hooks
React Hooks were introduced to solve some common problems developers faced with class components. Before Hooks, managing state and lifecycle methods was often complicated. With Hooks, you can write more straightforward and maintainable code. Here’s a brief timeline:
Year | Event |
---|---|
2013 | React is released |
2018 | React 16.8 introduces Hooks |
2020 | Hooks become widely adopted |
Why Use React Hooks?
Using React Hooks can greatly improve your development experience. Here are some reasons to consider:
- Easier State Management: You can manage state without needing to convert components to classes.
- Better Performance: Hooks can help optimize your app by controlling when effects run.
- Enhanced Developer Experience: Hooks provide a consistent way to manage state and side effects, making it easier to understand your code.
React Hooks are a powerful tool that can help you write better, more efficient code. By mastering React Hooks, you can unlock new capabilities in your React applications and improve your overall development skills. This article will explore hooks’ capabilities, best practices, and advanced techniques, which will hopefully level up your React development skills.
State Management with useState
Understanding useState
The useState hook is a key feature in React that allows developers to manage state in functional components. It provides a simple way to add state to your components without needing to use class components. When you call useState
, it returns an array with two elements: the current state and a function to update that state.
Common Use Cases for useState
Here are some common scenarios where you might use the useState hook:
- Form Inputs: Managing the values of input fields in forms.
- Toggle States: Keeping track of whether a component is open or closed.
- Counters: Implementing simple counters that increase or decrease values.
Best Practices for useState
To make the most of the useState hook, consider these best practices:
- Initialize State Properly: Always set an initial state that makes sense for your component.
- Use Functional Updates: When updating state based on the previous state, use the functional form of the state setter to avoid stale closures.
- Group Related States: If you have multiple related state variables, consider using a single state object to keep them together.
Managing state is a fundamental concept in React, and the useState hook is one of the most commonly used hooks for handling state in functional components.
By following these guidelines, you can effectively manage state in your React applications, leading to cleaner and more maintainable code.
Handling Side Effects with useEffect
Understanding useEffect
The useEffect hook is essential for managing side effects in your React components. Side effects can include tasks like fetching data, subscribing to events, or directly manipulating the DOM. This hook runs after every render, allowing you to perform these actions at the right time.
Common Use Cases for useEffect
Here are some common scenarios where you might use the useEffect hook:
- Data fetching: Retrieve data from an API when the component mounts.
- Event listeners: Set up event listeners for user interactions.
- Timers: Manage timers or intervals for animations or updates.
Best Practices for useEffect
To make the most of the useEffect hook, consider these best practices:
- Specify dependencies: Always provide a dependency array to control when the effect runs. This helps avoid unnecessary re-renders.
- Cleanup: Return a cleanup function to remove any subscriptions or event listeners when the component unmounts.
- Avoid overusing: Use useEffect only when necessary to keep your components efficient.
Remember, the useEffect hook is your go-to for handling side effects in React. It helps keep your components clean and efficient while managing tasks that need to happen outside the normal rendering flow.
Context Management with useContext
Understanding useContext
The useContext hook is a powerful feature that allows you to share state logic across components without the need to pass props manually at every level. This makes it easier to manage data that needs to be accessed by many components.
Using useContext for Global State
Using useContext can help you manage global state effectively. Here are some key points to consider:
- Avoid Prop Drilling: You can access context directly without passing props through every component.
- Simplify State Management: It makes your code cleaner and easier to understand.
- Share Data Easily: You can share data like themes, user information, or settings across your app.
Best Practices for useContext
To get the most out of useContext, follow these best practices:
- Create Context Wisely: Only create context for data that needs to be shared widely.
- Use Providers: Wrap your components with a context provider to make the context available.
- Combine with useReducer: For complex state management, consider using useReducer with useContext.
Using the useContext hook can greatly simplify your component structure and improve code readability.
Context Name | Purpose | Example Usage |
---|---|---|
ThemeContext | Manage theme settings | Themed components |
AuthContext | Handle user authentication | Access user info |
LanguageContext | Manage language preferences | Multi-language support |
Advanced State Management with useReducer
Understanding useReducer
The useReducer
hook is a powerful tool for managing complex state in React applications. It allows you to handle state transitions in a more organized way compared to useState
. This is especially useful when you have multiple sub-values or complex logic.
Common Use Cases for useReducer
Here are some situations where useReducer
shines:
- Managing form state: Instead of using
useState
for each field,useReducer
allows you to combine form state management into a single reducer function. - Handling complex state logic: When your state changes depend on multiple actions,
useReducer
can simplify your code. - Optimizing performance: It can help prevent unnecessary re-renders by managing state updates more efficiently.
Best Practices for useReducer
To get the most out of useReducer
, consider these best practices:
- Keep your reducer pure: Ensure that your reducer function does not have side effects.
- Use meaningful action types: This makes your code easier to read and maintain.
- Combine with useContext: For global state management, combine
useReducer
withuseContext
to avoid prop drilling.
Using useReducer can greatly enhance your application’s state management, making it cleaner and more efficient.
Action Type | Description |
---|---|
increment | Increases the count by 1 |
decrement | Decreases the count by 1 |
reset | Resets the count to the initial value |
By following these guidelines, you can effectively manage complex state in your React applications using useReducer
.
Optimizing Performance with useMemo and useCallback
Understanding useMemo
The useMemo hook is a tool that helps you optimize your React component by memoizing computed values. This means it saves the result of a calculation so that it doesn’t have to be redone every time the component renders. This is especially useful for expensive calculations that take a lot of time to compute.
For example, if you have a function that processes a large array, you can use useMemo to only recalculate the result when the array changes. Here’s a simple example:
const result = useMemo(() => {
return expensiveCalculation(data);
}, [data]);
Understanding useCallback
The useCallback hook is similar but focuses on functions. It memoizes callback functions, which means it prevents them from being recreated on every render. This is important when you pass functions to child components, as it can help avoid unnecessary re-renders.
Here’s how you might use it:
const handleClick = useCallback(() => {
setCount(count + 1);
}, [count]);
Best Practices for useMemo and useCallback
To get the most out of useMemo and useCallback, consider these best practices:
- Use them for expensive calculations: Only use useMemo for calculations that are costly in terms of performance.
- Avoid overusing them: Don’t use these hooks everywhere; they can add complexity. Use them only when necessary.
- Keep dependencies in mind: Always provide the correct dependencies in the array to ensure the memoized values are updated correctly.
Using useMemo and useCallback can significantly improve your app’s performance, but they should be used wisely to avoid unnecessary complexity.
By understanding and applying these hooks, you can make your React applications faster and more efficient!
Creating Custom Hooks
What Are Custom Hooks?
Custom Hooks are special functions in React that allow you to encapsulate reusable logic. They help you share stateful logic between components without repeating code. By following a simple naming convention, starting with the word "use," you can create your own hooks that can be used just like built-in hooks.
How to Create Custom Hooks
Creating a custom hook is straightforward. Here’s how you can do it:
- Define a function that starts with "use."
- Inside this function, use other hooks like
useState
oruseEffect
as needed. - Return the values or functions you want to expose.
Here’s a simple example:
import { useState } from 'react';
function useCounter(initialValue = 0) {
const [count, setCount] = useState(initialValue);
const increment = () => setCount(count + 1);
return { count, increment };
}
Examples of Custom Hooks
Custom Hooks can be used in various scenarios. Here are a few examples:
- useFetch: A hook to fetch data from an API.
- useForm: A hook to manage form state and validation.
- useLocalStorage: A hook to sync state with local storage.
Custom Hooks are a powerful way to enhance code reusability and keep your components clean and organized. By using them, you can avoid code duplication and make your codebase easier to maintain.
Testing Components with React Hooks
Setting Up Tests for Hooks
Testing React components that use hooks is essential for ensuring code quality. To get started, you need to set up your testing environment. Here are the steps:
- Install Jest and React Testing Library.
- Create a test file for your component.
- Import necessary functions from the testing library.
Testing useState and useEffect
When testing components that utilize useState
and useEffect
, you can simulate user interactions and check the component’s behavior. Here’s how:
- Use
render
to display your component. - Simulate events like clicks or form submissions.
- Use assertions to check if the state updates correctly.
For example, if you have a counter component:
import { render, screen, fireEvent } from '@testing-library/react';
import Counter from './Counter';
test('increments counter', () => {
render(<Counter />);
fireEvent.click(screen.getByText(/increment/i));
expect(screen.getByText(/count: 1/i)).toBeInTheDocument();
});
Testing Custom Hooks
Testing custom hooks can be done using a helper function. Here’s a simple approach:
- Create a test file for your custom hook.
- Use
renderHook
from React Testing Library. - Write tests to check the hook’s behavior.
For instance, if you have a custom hook that fetches data:
import { renderHook } from '@testing-library/react-hooks';
import useFetch from './useFetch';
test('fetches data', async () => {
const { result, waitForNextUpdate } = renderHook(() => useFetch('api/data'));
await waitForNextUpdate();
expect(result.current.data).toBeDefined();
});
Testing your components ensures they work as expected and helps catch bugs early. Effective testing leads to more reliable applications and happier users.
Real-World Examples and Use Cases
Building a Counter with useState
Creating a simple counter is a great way to understand the useState hook. Here’s how you can do it:
- Set up state: Use
useState
to create a state variable for the count. - Display the count: Show the current count in your component.
- Update the count: Add a button to increase the count when clicked.
Here’s a quick example:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
Fetching Data with useEffect
Fetching data from an API is another common use case. The useEffect hook helps manage side effects like data fetching. Here’s a simple way to do it:
- Set up state: Use
useState
to hold the fetched data. - Fetch data: Use
useEffect
to call the API when the component mounts. - Display data: Render the fetched data in your component.
Example:
import React, { useState, useEffect } from 'react';
const DataFetcher = () => {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
}, []);
return <div>{data ? <p>{data}</p> : <p>Loading...</p>}</div>;
};
Managing Global State with useContext
The useContext hook allows you to share data across components without passing props. Here’s how to use it:
- Create context: Use
React.createContext()
to create a context. - Provide context: Wrap your components with the context provider.
- Consume context: Use
useContext
to access the context value in child components.
Example:
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
const ThemedButton = () => {
const theme = useContext(ThemeContext);
return <button style={{ background: theme }}>Themed Button</button>;
};
const App = () => (
<ThemeContext.Provider value="dark">
<ThemedButton />
</ThemeContext.Provider>
);
Using React Hooks can greatly simplify your code and make it easier to manage state and side effects. Understanding these examples will help you unlock the full potential of React Hooks!
Common Pitfalls and How to Avoid Them
Avoiding Overuse of Hooks
Using hooks is great, but overusing them can lead to confusion. Here are some tips to avoid this pitfall:
- Use hooks only when necessary: Don’t add hooks for every small feature.
- Keep your components simple: If a component is doing too much, consider breaking it down.
- Limit the number of hooks in a single component: This helps in maintaining clarity.
Handling Dependencies in useEffect
The useEffect
hook can be tricky. If you don’t manage dependencies well, it can cause unexpected behavior. Here’s how to handle it:
- Always specify dependencies: This ensures your effect runs only when needed.
- Use the dependency array wisely: Include all variables that your effect uses.
- Check for stale closures: If you use state inside
useEffect
, make sure it’s the latest state.
Debugging Custom Hooks
Custom hooks can be powerful, but they can also introduce bugs. Here are some strategies to debug them:
- Log outputs: Use
console.log
to track values and states. - Test in isolation: Make sure your custom hook works independently before integrating it.
- Use React DevTools: This can help you visualize the state and props of your components.
Remember, understanding the rules of hooks is crucial for effective React development. By avoiding these common pitfalls, you can create cleaner and more efficient code.
Best Practices for Using React Hooks
Following the Rules of Hooks
To make the most of React Hooks, it’s crucial to follow the rules. Here are some key points:
- Only call hooks at the top level: Don’t call hooks inside loops, conditions, or nested functions.
- Only call hooks from React functions: This means you should only use hooks in functional components or custom hooks.
- Use hooks consistently: Always use the same order of hooks in your components to avoid unexpected behavior.
Writing Clean and Maintainable Code
Using hooks can lead to cleaner code. Here are some tips:
- Keep your components small: Break down large components into smaller ones to improve readability.
- Use custom hooks: If you find yourself repeating logic, create a custom hook to encapsulate that logic.
- Document your hooks: Write comments to explain what your hooks do, making it easier for others (and yourself) to understand.
Performance Optimization Tips
To enhance performance when using hooks, consider these strategies:
- Use useMemo and useCallback: These hooks help prevent unnecessary re-renders by memoizing values and functions.
- Optimize dependencies in useEffect: Make sure to include only necessary dependencies to avoid extra renders.
- Batch state updates: When updating state multiple times, try to batch them together to minimize re-renders.
Remember: React Hooks make your code easier to read and write. They simplify state management, enhance performance, and help reuse logic across multiple components.
By following these best practices, you can unlock the full potential of React Hooks and create efficient, maintainable applications.
When using React Hooks, it’s important to follow some key tips to make your coding smoother. Start by keeping your components simple and focused. This helps you avoid confusion and makes your code easier to read. Also, remember to use the right hooks for the right tasks. For more tips and to start your coding journey, visit our website today!
Conclusion
In summary, React Hooks have changed how we create web applications using React. They make it easier to manage state and side effects without needing to use class components. This not only leads to cleaner code but also helps developers reuse their logic across different parts of their applications. By learning and using React Hooks, you can boost your productivity and write better, more organized code. Embracing these tools will help you become a more effective developer and enhance your overall coding experience.
Frequently Asked Questions
What are React Hooks?
React Hooks are special functions that let you use state and other features in functional components without needing class components.
Why were Hooks introduced?
Hooks were introduced in React version 16.8 to make it easier to manage state and side effects in functional components.
Can I use Hooks in class components?
No, Hooks can only be used in functional components.
What is the useState Hook?
The useState Hook allows you to add state to functional components. It gives you a way to declare a state variable and a function to update it.
How does the useEffect Hook work?
The useEffect Hook lets you perform side effects in your components, like fetching data or changing the document title.
What are custom Hooks?
Custom Hooks are functions that allow you to reuse stateful logic across different components.
Are there any rules for using Hooks?
Yes, Hooks should only be called at the top level of a component and only in functional components.
How can Hooks improve performance?
Hooks can help improve performance by allowing you to control when effects run and by preventing unnecessary re-renders.