Union-Find (Disjoint Set): A Comprehensive Guide for Efficient Data Structure Operations
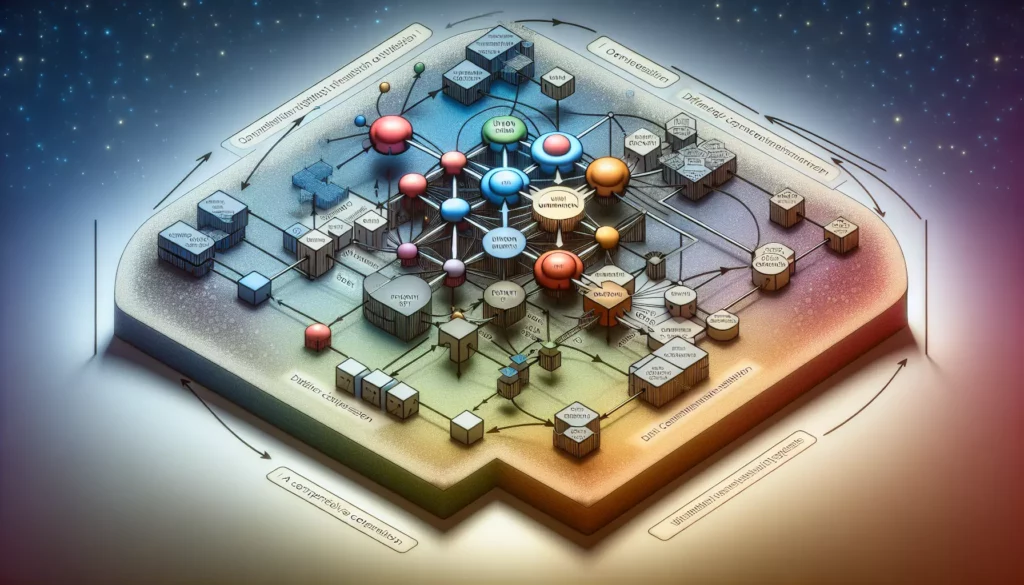
In the world of computer science and algorithmic problem-solving, efficient data structures play a crucial role in optimizing operations and improving overall performance. One such powerful data structure is the Union-Find, also known as the Disjoint Set. This data structure is particularly useful for solving problems related to connectivity and grouping elements efficiently. In this comprehensive guide, we’ll dive deep into the Union-Find data structure, exploring its concepts, implementation, and applications in various programming scenarios.
What is Union-Find?
Union-Find, also referred to as the Disjoint Set data structure, is a data structure that maintains a collection of disjoint sets. Each set is represented by a representative element, often called the parent or root. The primary operations supported by Union-Find are:
- Find: Determine which set an element belongs to by identifying its representative element.
- Union: Merge two sets into a single set.
The Union-Find data structure is particularly efficient for these operations, with near-constant time complexity when implemented with optimizations like path compression and union by rank.
Basic Concepts and Terminology
Before diving into the implementation details, let’s familiarize ourselves with some key concepts and terminology associated with Union-Find:
- Disjoint Sets: Sets that have no elements in common.
- Representative Element: An element chosen to represent an entire set.
- Parent: The immediate ancestor of an element in the tree structure.
- Root: The topmost element in a set’s tree structure, serving as the representative element.
- Rank: A measure of the depth or size of a tree, used for optimization.
- Path Compression: An optimization technique that flattens the tree structure during find operations.
Implementing Union-Find
Let’s implement a basic version of the Union-Find data structure in Python. We’ll start with a simple implementation and then add optimizations to improve its performance.
Basic Implementation
class UnionFind:
def __init__(self, n):
self.parent = list(range(n))
def find(self, x):
if self.parent[x] != x:
self.parent[x] = self.find(self.parent[x])
return self.parent[x]
def union(self, x, y):
root_x = self.find(x)
root_y = self.find(y)
if root_x != root_y:
self.parent[root_y] = root_x
# Usage example
uf = UnionFind(5)
uf.union(0, 1)
uf.union(2, 3)
uf.union(1, 2)
print(uf.find(0) == uf.find(3)) # True
print(uf.find(0) == uf.find(4)) # False
In this basic implementation, we initialize each element as its own parent. The find
operation recursively traverses the parent links until it reaches the root. The union
operation merges two sets by setting the root of one set as the parent of the root of the other set.
Optimized Implementation
To improve the performance of Union-Find, we can implement two key optimizations: path compression and union by rank.
class UnionFind:
def __init__(self, n):
self.parent = list(range(n))
self.rank = [0] * n
def find(self, x):
if self.parent[x] != x:
self.parent[x] = self.find(self.parent[x]) # Path compression
return self.parent[x]
def union(self, x, y):
root_x = self.find(x)
root_y = self.find(y)
if root_x == root_y:
return
# Union by rank
if self.rank[root_x] < self.rank[root_y]:
self.parent[root_x] = root_y
elif self.rank[root_x] > self.rank[root_y]:
self.parent[root_y] = root_x
else:
self.parent[root_y] = root_x
self.rank[root_x] += 1
# Usage example
uf = UnionFind(5)
uf.union(0, 1)
uf.union(2, 3)
uf.union(1, 2)
print(uf.find(0) == uf.find(3)) # True
print(uf.find(0) == uf.find(4)) # False
In this optimized implementation, we’ve added path compression in the find
method and union by rank in the union
method. These optimizations significantly improve the time complexity of operations, making them nearly constant time in practice.
Time Complexity Analysis
Let’s analyze the time complexity of Union-Find operations:
- Initialization: O(n), where n is the number of elements.
- Find: O(α(n)) amortized, where α(n) is the inverse Ackermann function, which grows very slowly and is effectively constant for all practical values of n.
- Union: O(α(n)) amortized, as it involves two find operations and a constant-time merging step.
The inverse Ackermann function α(n) grows so slowly that for all practical values of n, it can be considered constant. This makes Union-Find operations effectively constant time in practice.
Applications of Union-Find
Union-Find has numerous applications in various areas of computer science and algorithm design. Let’s explore some common use cases:
1. Connected Components in Graphs
Union-Find is excellent for finding connected components in an undirected graph. As you process edges, you can use union operations to merge connected components.
def connected_components(n, edges):
uf = UnionFind(n)
for u, v in edges:
uf.union(u, v)
components = {}
for i in range(n):
root = uf.find(i)
if root not in components:
components[root] = []
components[root].append(i)
return list(components.values())
# Usage example
n = 5
edges = [(0, 1), (1, 2), (3, 4)]
print(connected_components(n, edges)) # [[0, 1, 2], [3, 4]]
2. Kruskal’s Algorithm for Minimum Spanning Tree
Union-Find is a key component in Kruskal’s algorithm for finding the minimum spanning tree of a graph.
def kruskal_mst(n, edges):
uf = UnionFind(n)
mst = []
edges.sort(key=lambda x: x[2]) # Sort edges by weight
for u, v, weight in edges:
if uf.find(u) != uf.find(v):
uf.union(u, v)
mst.append((u, v, weight))
return mst
# Usage example
n = 4
edges = [(0, 1, 1), (1, 2, 2), (2, 3, 3), (3, 0, 4), (0, 2, 5)]
print(kruskal_mst(n, edges)) # [(0, 1, 1), (1, 2, 2), (2, 3, 3)]
3. Detecting Cycles in Graphs
Union-Find can be used to detect cycles in an undirected graph efficiently.
def has_cycle(n, edges):
uf = UnionFind(n)
for u, v in edges:
if uf.find(u) == uf.find(v):
return True
uf.union(u, v)
return False
# Usage example
n = 4
edges = [(0, 1), (1, 2), (2, 3), (3, 0)]
print(has_cycle(n, edges)) # True
4. Percolation
Union-Find is useful in solving percolation problems, where you need to determine if there’s a path through a grid of open and closed sites.
class Percolation:
def __init__(self, n):
self.n = n
self.uf = UnionFind(n * n + 2) # +2 for virtual top and bottom
self.open_sites = set()
self.top = n * n
self.bottom = n * n + 1
def open(self, row, col):
if not self.is_open(row, col):
site = row * self.n + col
self.open_sites.add(site)
if row == 0:
self.uf.union(site, self.top)
if row == self.n - 1:
self.uf.union(site, self.bottom)
for dr, dc in [(-1, 0), (1, 0), (0, -1), (0, 1)]:
new_row, new_col = row + dr, col + dc
if 0 <= new_row < self.n and 0 <= new_col < self.n:
if self.is_open(new_row, new_col):
self.uf.union(site, new_row * self.n + new_col)
def is_open(self, row, col):
return row * self.n + col in self.open_sites
def percolates(self):
return self.uf.find(self.top) == self.uf.find(self.bottom)
# Usage example
perc = Percolation(3)
perc.open(0, 1)
perc.open(1, 1)
perc.open(2, 1)
print(perc.percolates()) # True
Advanced Techniques and Variations
As you become more comfortable with the basic Union-Find data structure, you can explore advanced techniques and variations to solve more complex problems:
1. Weighted Quick Union
This variation keeps track of the size of each tree and always attaches the smaller tree to the root of the larger tree. This ensures that the tree depth remains logarithmic in the worst case.
2. Path Splitting and Halving
These are alternative path compression techniques that can be used instead of full path compression. They offer a good balance between implementation simplicity and performance.
3. Persistent Union-Find
This variation allows you to maintain the history of unions and perform operations on past versions of the data structure. It’s useful in scenarios where you need to answer queries about the state of the structure at different points in time.
4. Dynamic Connectivity
Union-Find can be extended to handle dynamic connectivity problems, where you need to support both union and delete operations efficiently.
Practice Problems
To solidify your understanding of Union-Find, try solving these practice problems:
- Number of Islands II (LeetCode 305): Given a 2D matrix initially filled with water, add land to cells and count the number of islands after each addition.
- Accounts Merge (LeetCode 721): Given a list of accounts where each account is a list of emails, merge accounts with common emails.
- Redundant Connection (LeetCode 684): In a graph that started as a tree with N nodes, find the edge that can be removed to turn the graph back into a tree.
- Satisfiability of Equality Equations (LeetCode 990): Given an array of strings representing equations (e.g., “a==b”, “b!=c”), determine if the equations are satisfiable.
- Minimize Malware Spread (LeetCode 924): Given a network of computers and initial infected computers, determine which computer to remove to minimize malware spread.
Conclusion
Union-Find is a powerful and efficient data structure that plays a crucial role in solving various graph-related problems and connectivity issues. Its near-constant time complexity for operations makes it an invaluable tool in a programmer’s toolkit. By mastering Union-Find, you’ll be well-equipped to tackle a wide range of algorithmic challenges, from basic graph problems to advanced network analysis.
As you continue your journey in algorithmic problem-solving, remember that Union-Find is just one of many important data structures and algorithms. Keep practicing, exploring new concepts, and applying your knowledge to real-world problems. With persistence and dedication, you’ll develop the skills necessary to excel in technical interviews and become a proficient problem solver.
Happy coding, and may your Union-Find implementations always be optimized and bug-free!