Uniform Cost Search: A Comprehensive Guide to Efficient Path Finding
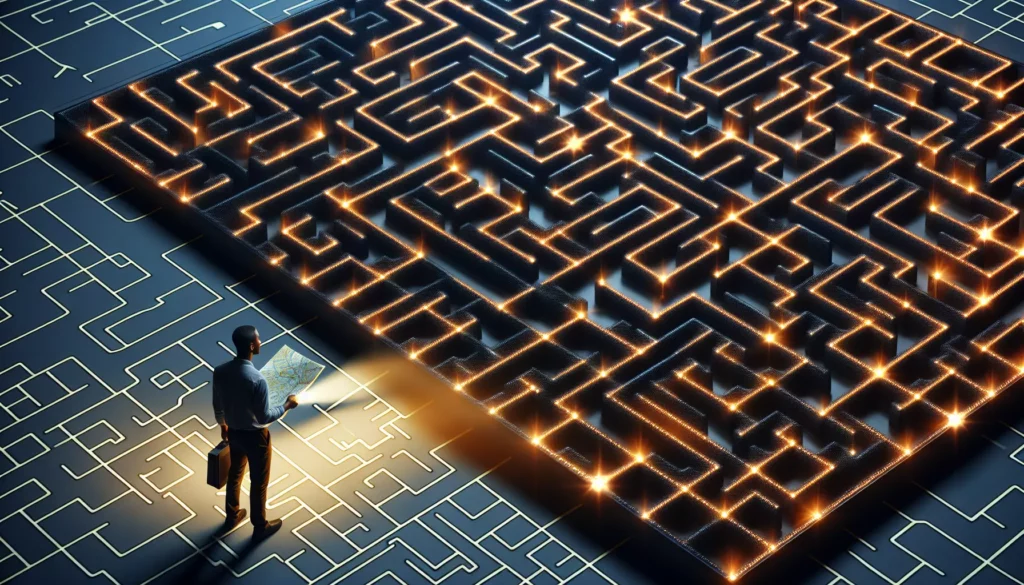
In the world of artificial intelligence and computer science, path finding algorithms play a crucial role in solving various problems, from navigating maps to optimizing network routes. Among these algorithms, Uniform Cost Search (UCS) stands out as a powerful and versatile method for finding the optimal path between two points. In this comprehensive guide, we’ll dive deep into the intricacies of Uniform Cost Search, exploring its principles, implementation, advantages, and real-world applications.
What is Uniform Cost Search?
Uniform Cost Search is an uninformed search algorithm used to find the lowest-cost path between a starting node and a goal node in a weighted graph. It’s an extension of Breadth-First Search (BFS) that takes into account the cost of each step, making it particularly useful in scenarios where different actions have varying costs.
The key principle behind UCS is that it always expands the node with the lowest cumulative cost from the start node. This ensures that the algorithm finds the optimal path to the goal, assuming all edge costs are non-negative.
How Uniform Cost Search Works
The Uniform Cost Search algorithm follows these steps:
- Initialize the frontier (priority queue) with the start node, setting its cost to 0.
- Create an empty set for explored nodes.
- While the frontier is not empty:
- Remove the node with the lowest cost from the frontier.
- If this node is the goal, return the solution.
- Add the node to the explored set.
- For each neighbor of the current node:
- If the neighbor is not in the explored set and not in the frontier, add it to the frontier.
- If the neighbor is in the frontier with a higher cost, update its cost and parent.
- If the frontier is empty and the goal hasn’t been reached, return failure.
Implementing Uniform Cost Search
Let’s implement Uniform Cost Search in Python to better understand how it works. We’ll use a priority queue to efficiently manage the frontier.
import heapq
def uniform_cost_search(graph, start, goal):
frontier = [(0, start)] # Priority queue of (cost, node) tuples
explored = set()
came_from = {}
cost_so_far = {start: 0}
while frontier:
current_cost, current_node = heapq.heappop(frontier)
if current_node == goal:
return reconstruct_path(came_from, start, goal), current_cost
explored.add(current_node)
for neighbor, step_cost in graph[current_node].items():
new_cost = cost_so_far[current_node] + step_cost
if neighbor not in explored and neighbor not in (node for _, node in frontier):
heapq.heappush(frontier, (new_cost, neighbor))
came_from[neighbor] = current_node
cost_so_far[neighbor] = new_cost
elif neighbor in (node for _, node in frontier):
if new_cost < cost_so_far[neighbor]:
# Remove the old entry from the frontier
frontier = [(c, n) for c, n in frontier if n != neighbor]
heapq.heapify(frontier)
# Add the new entry to the frontier
heapq.heappush(frontier, (new_cost, neighbor))
came_from[neighbor] = current_node
cost_so_far[neighbor] = new_cost
return None, float('inf') # No path found
def reconstruct_path(came_from, start, goal):
path = [goal]
while path[-1] != start:
path.append(came_from[path[-1]])
path.reverse()
return path
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'F': 5},
'D': {'G': 2},
'E': {'G': 3},
'F': {'G': 1},
'G': {}
}
start = 'A'
goal = 'G'
path, cost = uniform_cost_search(graph, start, goal)
print(f"Optimal path: {path}")
print(f"Total cost: {cost}")
This implementation uses a min-heap (priority queue) to efficiently select the node with the lowest cumulative cost at each step. The came_from
dictionary keeps track of the parent nodes, allowing us to reconstruct the path once the goal is reached.
Advantages of Uniform Cost Search
Uniform Cost Search offers several advantages over other search algorithms:
- Optimality: UCS guarantees finding the optimal (lowest-cost) path to the goal, assuming all edge costs are non-negative.
- Completeness: If a path to the goal exists, UCS will find it, given enough time and memory.
- Flexibility: UCS can handle graphs with varying edge costs, making it more versatile than algorithms like Breadth-First Search.
- Efficiency: In many cases, UCS explores fewer nodes than uninformed search algorithms like Depth-First Search, especially when the optimal path has a lower cost than other paths.
Limitations of Uniform Cost Search
Despite its advantages, Uniform Cost Search has some limitations:
- Memory consumption: UCS needs to store all generated nodes in memory, which can be problematic for large search spaces.
- Time complexity: In the worst case, UCS may explore all possible paths before reaching the goal, leading to exponential time complexity.
- Lack of heuristic information: Unlike informed search algorithms like A*, UCS doesn’t use any heuristic information to guide the search towards the goal more efficiently.
Uniform Cost Search vs. Other Algorithms
To better understand the strengths and weaknesses of Uniform Cost Search, let’s compare it to some other popular search algorithms:
UCS vs. Breadth-First Search (BFS)
BFS is a special case of UCS where all edge costs are equal. UCS becomes equivalent to BFS when dealing with unweighted graphs or graphs where all edges have the same cost. However, UCS is more flexible and can handle varying edge costs, making it superior in weighted graph scenarios.
UCS vs. Depth-First Search (DFS)
While DFS can be more memory-efficient than UCS, it doesn’t guarantee finding the optimal path. UCS explores paths in order of increasing cost, ensuring optimality. DFS, on the other hand, might get stuck exploring a deep, suboptimal path before considering better alternatives.
UCS vs. Dijkstra’s Algorithm
UCS and Dijkstra’s algorithm are essentially the same when finding the shortest path between two specific nodes. The main difference is that Dijkstra’s algorithm typically computes shortest paths from a single source to all other nodes, while UCS stops once the goal is reached.
UCS vs. A* Search
A* is an informed search algorithm that extends UCS by incorporating a heuristic function to estimate the cost from the current node to the goal. This allows A* to potentially find the optimal path more efficiently than UCS by exploring fewer nodes. However, UCS doesn’t require a heuristic function and is guaranteed to find the optimal path even when no good heuristic is available.
Real-World Applications of Uniform Cost Search
Uniform Cost Search finds applications in various domains where finding the optimal path or solution is crucial. Some real-world applications include:
1. Navigation and Route Planning
UCS can be used to find the shortest or fastest route between two locations on a map, taking into account factors like distance, travel time, or fuel consumption. This is particularly useful in GPS navigation systems and logistics planning.
2. Network Routing
In computer networks, UCS can be applied to find the most efficient path for data packets to travel from one node to another, considering factors like bandwidth, latency, and network congestion.
3. Game AI
UCS can be used in game development to create intelligent non-player characters (NPCs) that can navigate complex game environments efficiently, avoiding obstacles and finding optimal paths to objectives.
4. Resource Allocation
In project management and resource allocation problems, UCS can help find the most cost-effective way to allocate resources or schedule tasks, considering various constraints and costs associated with different options.
5. Robotics
UCS can be employed in robotic path planning to help robots navigate through complex environments, avoiding obstacles and finding the most efficient path to their goal.
Optimizing Uniform Cost Search
While Uniform Cost Search is already an efficient algorithm, there are several ways to optimize its performance:
1. Use an Efficient Priority Queue Implementation
The choice of priority queue implementation can significantly impact the performance of UCS. Using a binary heap or Fibonacci heap can improve the efficiency of insert and delete-min operations.
2. Implement Graph Pruning
By keeping track of visited nodes and their costs, you can avoid re-exploring nodes that have already been reached through a lower-cost path. This can significantly reduce the number of nodes explored.
3. Bidirectional Search
For problems where both the start and goal states are known, implementing a bidirectional search (searching from both the start and goal simultaneously) can potentially reduce the search space and improve performance.
4. State Space Reduction
If possible, reduce the state space by eliminating irrelevant or redundant states. This can be done through problem-specific optimizations or by using domain knowledge to identify and remove unnecessary paths.
5. Parallel Processing
For large-scale problems, implementing a parallel version of UCS can significantly improve performance by distributing the workload across multiple processors or machines.
Implementing Uniform Cost Search in Different Programming Languages
While we’ve already seen a Python implementation of Uniform Cost Search, it’s worth exploring how the algorithm can be implemented in other popular programming languages. This can help you choose the most suitable language for your specific use case.
Java Implementation
import java.util.*;
class Node implements Comparable<Node> {
String name;
int cost;
public Node(String name, int cost) {
this.name = name;
this.cost = cost;
}
@Override
public int compareTo(Node other) {
return Integer.compare(this.cost, other.cost);
}
}
public class UniformCostSearch {
public static Map<String, Integer> uniformCostSearch(Map<String, Map<String, Integer>> graph, String start, String goal) {
PriorityQueue<Node> frontier = new PriorityQueue<>();
frontier.add(new Node(start, 0));
Map<String, Integer> costSoFar = new HashMap<>();
costSoFar.put(start, 0);
Map<String, String> cameFrom = new HashMap<>();
while (!frontier.isEmpty()) {
Node current = frontier.poll();
if (current.name.equals(goal)) {
return reconstructPath(cameFrom, start, goal);
}
for (Map.Entry<String, Integer> neighbor : graph.get(current.name).entrySet()) {
int newCost = costSoFar.get(current.name) + neighbor.getValue();
if (!costSoFar.containsKey(neighbor.getKey()) || newCost < costSoFar.get(neighbor.getKey())) {
costSoFar.put(neighbor.getKey(), newCost);
frontier.add(new Node(neighbor.getKey(), newCost));
cameFrom.put(neighbor.getKey(), current.name);
}
}
}
return null; // No path found
}
private static Map<String, Integer> reconstructPath(Map<String, String> cameFrom, String start, String goal) {
List<String> path = new ArrayList<>();
String current = goal;
while (!current.equals(start)) {
path.add(current);
current = cameFrom.get(current);
}
path.add(start);
Collections.reverse(path);
Map<String, Integer> result = new LinkedHashMap<>();
for (int i = 0; i < path.size() - 1; i++) {
result.put(path.get(i) + " -> " + path.get(i + 1), 0);
}
return result;
}
public static void main(String[] args) {
Map<String, Map<String, Integer>> graph = new HashMap<>();
graph.put("A", new HashMap<>() {{ put("B", 4); put("C", 2); }});
graph.put("B", new HashMap<>() {{ put("D", 3); put("E", 1); }});
graph.put("C", new HashMap<>() {{ put("F", 5); }});
graph.put("D", new HashMap<>() {{ put("G", 2); }});
graph.put("E", new HashMap<>() {{ put("G", 3); }});
graph.put("F", new HashMap<>() {{ put("G", 1); }});
graph.put("G", new HashMap<>());
Map<String, Integer> path = uniformCostSearch(graph, "A", "G");
System.out.println("Optimal path: " + path);
}
}
C++ Implementation
#include <iostream>
#include <queue>
#include <unordered_map>
#include <vector>
#include <limits>
using namespace std;
typedef pair<int, string> pis;
vector<string> uniform_cost_search(const unordered_map<string, unordered_map<string, int>>& graph, const string& start, const string& goal) {
priority_queue<pis, vector<pis>, greater<pis>> frontier;
frontier.push({0, start});
unordered_map<string, int> cost_so_far;
cost_so_far[start] = 0;
unordered_map<string, string> came_from;
while (!frontier.empty()) {
auto [current_cost, current_node] = frontier.top();
frontier.pop();
if (current_node == goal) {
vector<string> path;
while (current_node != start) {
path.push_back(current_node);
current_node = came_from[current_node];
}
path.push_back(start);
reverse(path.begin(), path.end());
return path;
}
for (const auto& [neighbor, step_cost] : graph.at(current_node)) {
int new_cost = cost_so_far[current_node] + step_cost;
if (!cost_so_far.count(neighbor) || new_cost < cost_so_far[neighbor]) {
cost_so_far[neighbor] = new_cost;
frontier.push({new_cost, neighbor});
came_from[neighbor] = current_node;
}
}
}
return {}; // No path found
}
int main() {
unordered_map<string, unordered_map<string, int>> graph = {
{"A", {{"B", 4}, {"C", 2}}},
{"B", {{"D", 3}, {"E", 1}}},
{"C", {{"F", 5}}},
{"D", {{"G", 2}}},
{"E", {{"G", 3}}},
{"F", {{"G", 1}}},
{"G", {}}
};
string start = "A";
string goal = "G";
vector<string> path = uniform_cost_search(graph, start, goal);
if (!path.empty()) {
cout << "Optimal path: ";
for (size_t i = 0; i < path.size(); ++i) {
cout << path[i];
if (i < path.size() - 1) cout << " -> ";
}
cout << endl;
} else {
cout << "No path found." << endl;
}
return 0;
}
Conclusion
Uniform Cost Search is a powerful and versatile algorithm for finding optimal paths in weighted graphs. Its ability to consider varying edge costs makes it suitable for a wide range of applications, from navigation systems to resource allocation problems. While it may not be as efficient as informed search algorithms like A* in some scenarios, UCS remains a fundamental tool in the field of artificial intelligence and computer science.
By understanding the principles behind Uniform Cost Search and its implementation in various programming languages, you can leverage this algorithm to solve complex path-finding problems efficiently. As you continue to explore the world of search algorithms, remember that UCS serves as a solid foundation for more advanced techniques and can be optimized or extended to suit specific problem domains.
Whether you’re developing a route planning system, optimizing network traffic, or creating intelligent game AI, Uniform Cost Search provides a reliable method for finding the best solution. As you apply this algorithm to your own projects, you’ll gain a deeper appreciation for its elegance and versatility in solving real-world problems.