Understanding Virtual Environments and Package Management in Python
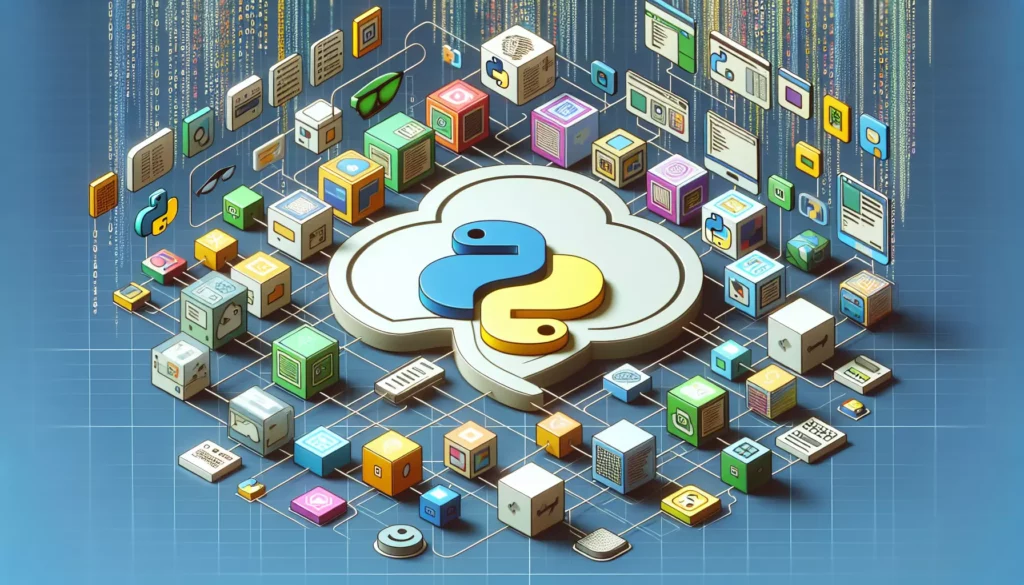
In the world of Python programming, two concepts that play a crucial role in maintaining clean, organized, and efficient development environments are virtual environments and package management. These tools are essential for both beginners and experienced developers, as they help manage dependencies, isolate projects, and ensure reproducibility. In this comprehensive guide, we’ll dive deep into virtual environments and package management, exploring their importance, how to use them effectively, and best practices for your Python projects.
Table of Contents
- Virtual Environments
- Package Management
- The venv Module
- Virtualenv
- Pip: The Python Package Installer
- Pipenv: Combining Virtual Environments and Package Management
- Conda: An Alternative Package and Environment Manager
- Best Practices for Virtual Environments and Package Management
- Common Issues and Troubleshooting
- Conclusion
1. Virtual Environments
Virtual environments are isolated Python environments that allow you to work on different projects with different dependencies without conflicts. They provide a way to create separate spaces for each project, ensuring that the packages and libraries used in one project don’t interfere with those in another.
Why Use Virtual Environments?
- Isolation: Each project can have its own set of dependencies, preventing conflicts between different versions of packages.
- Reproducibility: Virtual environments make it easier to recreate the exact environment needed for a project on different machines.
- Clean System: They keep your global Python installation clean by installing project-specific packages in isolated environments.
- Version Control: You can easily switch between different versions of Python or packages for different projects.
2. Package Management
Package management refers to the process of installing, updating, and removing external libraries and tools in your Python projects. Effective package management is crucial for maintaining a stable and efficient development environment.
Key Aspects of Package Management:
- Dependency Resolution: Automatically handling the installation of required dependencies for a package.
- Version Control: Managing different versions of packages and ensuring compatibility.
- Easy Installation: Simplifying the process of adding new libraries to your project.
- Updating and Removal: Keeping packages up-to-date and removing unused ones.
3. The venv Module
The venv
module is a built-in tool in Python 3.3 and later versions for creating lightweight virtual environments. It’s a simple and effective way to create isolated Python environments for your projects.
Creating a Virtual Environment with venv:
python3 -m venv myproject_env
This command creates a new virtual environment named myproject_env
in the current directory.
Activating the Virtual Environment:
On Unix or MacOS:
source myproject_env/bin/activate
On Windows:
myproject_env\Scripts\activate.bat
Deactivating the Virtual Environment:
To exit the virtual environment, simply run:
deactivate
4. Virtualenv
virtualenv
is a more feature-rich tool for creating virtual environments. It’s particularly useful for Python versions earlier than 3.3 or when you need more control over the environment creation process.
Installing Virtualenv:
pip install virtualenv
Creating a Virtual Environment with Virtualenv:
virtualenv myproject_env
Specifying Python Version:
You can create a virtual environment with a specific Python version:
virtualenv -p /usr/bin/python3.8 myproject_env
Activating and Deactivating:
The activation and deactivation process is the same as with venv
.
5. Pip: The Python Package Installer
Pip is the standard package manager for Python. It allows you to install and manage additional packages that are not part of the Python standard library.
Basic Pip Commands:
- Installing a package:
pip install package_name
- Installing a specific version:
pip install package_name==1.0.4
- Upgrading a package:
pip install --upgrade package_name
- Uninstalling a package:
pip uninstall package_name
- Listing installed packages:
pip list
- Generating a requirements file:
pip freeze > requirements.txt
- Installing from a requirements file:
pip install -r requirements.txt
Using Pip with Virtual Environments:
When you activate a virtual environment and use pip, it will install packages only in that environment, keeping your global Python installation clean.
6. Pipenv: Combining Virtual Environments and Package Management
Pipenv is a higher-level tool that aims to bring the best of all packaging worlds to the Python world. It automatically creates and manages a virtual environment for your projects, as well as adds/removes packages from your Pipfile as you install/uninstall packages.
Installing Pipenv:
pip install pipenv
Creating a New Project:
mkdir myproject
cd myproject
pipenv install
This creates a new virtual environment and a Pipfile for managing dependencies.
Installing Packages:
pipenv install requests
This installs the requests library and adds it to the Pipfile.
Activating the Virtual Environment:
pipenv shell
Running a Script:
pipenv run python script.py
7. Conda: An Alternative Package and Environment Manager
Conda is a package and environment management system that is particularly popular in the data science community. It can handle library dependencies outside of the Python packages as well, making it a powerful tool for complex scientific computing projects.
Installing Conda:
Download and install Miniconda or Anaconda from the official website.
Creating a New Environment:
conda create --name myenv python=3.8
Activating the Environment:
conda activate myenv
Installing Packages:
conda install numpy pandas matplotlib
Deactivating the Environment:
conda deactivate
8. Best Practices for Virtual Environments and Package Management
- Use Virtual Environments for Every Project: This ensures clean, isolated environments for each project.
- Keep Your Requirements Updated: Regularly update your requirements.txt or Pipfile to reflect the current state of your project.
- Version Control Your Dependencies: Include your requirements file or Pipfile in version control, but not the virtual environment itself.
- Use Specific Versions: Specify exact versions of packages to ensure reproducibility.
- Regularly Update Dependencies: Keep your packages updated, but test thoroughly after updates.
- Clean Up Unused Environments: Remove virtual environments that are no longer needed to save disk space.
- Document Your Setup: Include instructions on how to set up the development environment in your project’s README.
9. Common Issues and Troubleshooting
Issue: Package Conflicts
Solution: Use virtual environments to isolate project dependencies. If conflicts persist, try creating a new environment and installing packages one by one to identify the conflict.
Issue: “Command Not Found” Errors
Solution: Ensure that the virtual environment is activated. Check if the package is installed in the current environment using pip list
.
Issue: Different Behavior in Different Environments
Solution: Verify that all environments have the same versions of packages. Use a requirements.txt file to ensure consistency.
Issue: Unable to Activate Virtual Environment
Solution: Check the path to the activate script. On Windows, you might need to run the activate script with the full path.
Issue: Pip Installing Globally Instead of in Virtual Environment
Solution: Make sure the virtual environment is activated. You can verify the pip location using which pip
(Unix) or where pip
(Windows).
10. Conclusion
Understanding and effectively using virtual environments and package management is crucial for Python developers at all levels. These tools not only help in maintaining clean and organized development environments but also contribute significantly to the reproducibility and portability of your projects.
Virtual environments, whether created using venv
, virtualenv
, or managed through tools like Pipenv or Conda, provide isolated spaces for your projects. This isolation prevents conflicts between different projects and allows you to work with different versions of Python and packages as needed.
Package management, primarily through pip but also with advanced tools like Pipenv and Conda, ensures that you can easily install, update, and manage the external libraries your projects depend on. Proper package management is key to maintaining stable and efficient development environments.
By following best practices and understanding how to troubleshoot common issues, you can create more robust, maintainable, and shareable Python projects. Whether you’re working on small scripts or large-scale applications, mastering virtual environments and package management will significantly improve your development workflow and the quality of your code.
As you continue to grow as a Python developer, remember that these tools and practices are fundamental to professional Python development. They form the foundation upon which you can build more complex projects and collaborate effectively with other developers. Keep exploring, experimenting, and refining your use of these tools to become a more proficient and efficient Python programmer.