Understanding Version Control Systems: Git Essentials
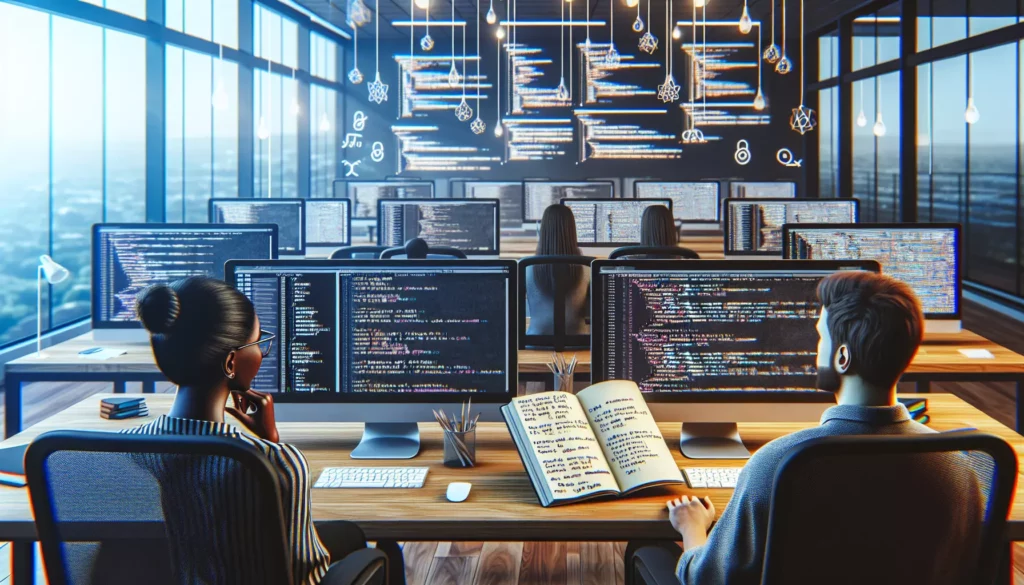
In the world of software development, version control systems (VCS) play a crucial role in managing and tracking changes to code over time. Among these systems, Git has emerged as the industry standard, beloved by developers for its flexibility, speed, and distributed nature. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, understanding Git is essential. In this comprehensive guide, we’ll dive deep into Git essentials, exploring its core concepts, commands, and best practices that will elevate your coding skills and collaboration abilities.
What is Git?
Git is a distributed version control system created by Linus Torvalds in 2005. It was designed to handle everything from small to very large projects with speed and efficiency. Git tracks changes in source code during software development and allows multiple developers to work together on non-linear development.
Key features of Git include:
- Distributed development
- Strong support for non-linear development
- Efficient handling of large projects
- Cryptographic authentication of history
- Toolkit-based design
Setting Up Git
Before we dive into Git commands and workflows, let’s ensure Git is properly set up on your system.
Installation
To install Git, visit the official Git website (https://git-scm.com/) and download the appropriate version for your operating system. Follow the installation instructions provided.
Configuration
After installation, you need to set up your Git configuration. Open your terminal or command prompt and enter the following commands, replacing the email and name with your own:
git config --global user.email "you@example.com"
git config --global user.name "Your Name"
These commands set your email address and name, which will be associated with your Git commits.
Core Concepts of Git
Before we start using Git commands, it’s crucial to understand some core concepts:
Repository
A repository (or “repo”) is a directory where Git has been initialized to start version controlling your files. This is typically one project.
Commit
A commit is a snapshot of your repository at a specific point in time. It’s like a save point in a game, allowing you to revert to this state later if needed.
Branch
A branch is a parallel version of your repository. It allows you to work on different parts of your project without impacting the main branch.
Remote
A remote is a common repository that all team members use to exchange their changes. In most cases, it’s stored on a code hosting service like GitHub or GitLab.
Fork
A fork is a copy of a repository. Forking a repository allows you to freely experiment with changes without affecting the original project.
Essential Git Commands
Now that we understand the core concepts, let’s explore some essential Git commands that you’ll use frequently in your development workflow.
Initializing a Repository
To start version controlling a project, navigate to your project directory and run:
git init
This command creates a new Git repository in the current directory.
Cloning a Repository
To create a local copy of a remote repository, use:
git clone <repository-url>
Replace
Checking Status
To see the current state of your working directory and staging area, use:
git status
This command shows which files have been modified, which are staged for commit, and which are untracked.
Adding Files to Staging Area
To stage files for commit, use:
git add <file>
To stage all modified files, use:
git add .
Committing Changes
To create a new commit with the staged changes, use:
git commit -m "Your commit message"
Always provide a clear and concise commit message that describes the changes you’ve made.
Pushing Changes to Remote
To send your local commits to a remote repository, use:
git push origin <branch-name>
Replace
Pulling Changes from Remote
To fetch and merge changes from a remote repository to your local branch, use:
git pull origin <branch-name>
Creating and Switching Branches
To create a new branch, use:
git branch <branch-name>
To switch to a different branch, use:
git checkout <branch-name>
You can combine these two commands with:
git checkout -b <new-branch-name>
This creates a new branch and switches to it in one command.
Merging Branches
To merge changes from one branch into another, first switch to the target branch, then use:
git merge <source-branch>
Git Workflows
Understanding Git workflows is crucial for effective collaboration in software development. Here are some popular Git workflows:
Centralized Workflow
This is the simplest workflow, where all developers work on a single branch (usually “master” or “main”). It’s suitable for small teams or projects with infrequent commits.
Feature Branch Workflow
In this workflow, each new feature is developed in a dedicated branch. Once the feature is complete, it’s merged back into the main branch. This allows for parallel development and easier code reviews.
Gitflow Workflow
Gitflow is a robust framework for managing larger projects. It uses separate branches for features, releases, and hotfixes, providing a structured approach to development and deployment.
Forking Workflow
This workflow is common in open-source projects. Developers “fork” the main repository, make changes in their fork, and then submit a pull request to have their changes merged into the main project.
Best Practices for Using Git
To make the most of Git and avoid common pitfalls, consider these best practices:
Commit Often
Make small, frequent commits rather than large, infrequent ones. This makes it easier to track changes and revert if necessary.
Write Clear Commit Messages
Your commit messages should clearly describe what changes were made and why. A common format is:
Short (50 chars or less) summary of changes
More detailed explanatory text, if necessary. Wrap it to about 72
characters or so. In some contexts, the first line is treated as the
subject of an email and the rest of the text as the body.
- Bullet points are okay, too
- Typically a hyphen or asterisk is used for the bullet, preceded
by a single space, with blank lines in between
Use Branches
Always create a new branch for each feature or bug fix. This keeps your work organized and makes it easier to manage multiple tasks simultaneously.
Pull Before You Push
Always pull the latest changes from the remote repository before pushing your own changes. This helps avoid merge conflicts.
Review Your Changes
Before committing, use git diff
to review your changes. This helps catch unintended modifications and ensures you’re only committing what you mean to.
Use .gitignore
Create a .gitignore
file in your repository to specify which files or directories Git should ignore. This is useful for excluding build artifacts, temporary files, and sensitive information.
Advanced Git Techniques
As you become more comfortable with Git, you might want to explore some advanced techniques:
Interactive Rebasing
Interactive rebasing allows you to modify commits in various ways as you move them to a new base. This is useful for cleaning up your commit history before merging or pushing. To start an interactive rebase:
git rebase -i <base>
Cherry-picking
Cherry-picking allows you to apply the changes introduced by some existing commits. This is useful when you want to pick specific commits from one branch and apply them to another. To cherry-pick a commit:
git cherry-pick <commit-hash>
Reflog
The reflog is a mechanism to record when the tips of branches and other references were updated in the local repository. It’s useful for recovering lost commits. To view the reflog:
git reflog
Submodules
Submodules allow you to keep a Git repository as a subdirectory of another Git repository. This is useful for including external dependencies in your project. To add a submodule:
git submodule add <repository-url> <path>
Git in Technical Interviews
Understanding Git is not just important for day-to-day development; it’s also a topic that may come up in technical interviews, especially for positions at major tech companies. Here are some Git-related topics that interviewers might ask about:
Branching Strategies
You might be asked to explain different branching strategies and when you would use each one. Be prepared to discuss feature branching, Gitflow, and trunk-based development.
Merge Conflicts
Interviewers might ask how you handle merge conflicts. Be ready to explain the process of resolving conflicts and strategies for minimizing them.
Git Internals
Some interviewers might delve into how Git works under the hood. Understanding concepts like the object model (blobs, trees, commits) and the .git directory structure can be beneficial.
Git Workflow Scenarios
You might be presented with a scenario and asked how you would handle it using Git. For example, “How would you revert a commit that has already been pushed to a remote repository?”
Git Tools and Integrations
While the command line is powerful, there are many tools and integrations that can enhance your Git experience:
GUI Clients
GUI clients like GitKraken, SourceTree, or GitHub Desktop provide a visual interface for Git operations, which can be helpful for visualizing complex branching structures.
IDE Integrations
Most modern IDEs like Visual Studio Code, IntelliJ IDEA, and Eclipse have built-in Git integration, allowing you to perform Git operations directly from your development environment.
CI/CD Tools
Continuous Integration and Continuous Deployment (CI/CD) tools like Jenkins, GitLab CI, and GitHub Actions integrate closely with Git to automate testing and deployment processes.
Code Review Platforms
Platforms like GitHub, GitLab, and Bitbucket provide powerful code review features that integrate with Git, allowing for pull requests, inline comments, and more.
Conclusion
Git is an indispensable tool in modern software development. Its distributed nature, powerful branching and merging capabilities, and robust ecosystem of tools and integrations make it an essential skill for any developer. Whether you’re just starting your coding journey or preparing for technical interviews at top tech companies, a solid understanding of Git will serve you well.
Remember, like any skill, proficiency with Git comes with practice. Don’t be afraid to experiment with different commands and workflows in a test repository. As you become more comfortable with Git, you’ll find it an invaluable ally in managing your code, collaborating with others, and tackling complex software projects.
Keep learning, keep practicing, and happy coding!