Understanding Uniform Probability Distribution in Programming
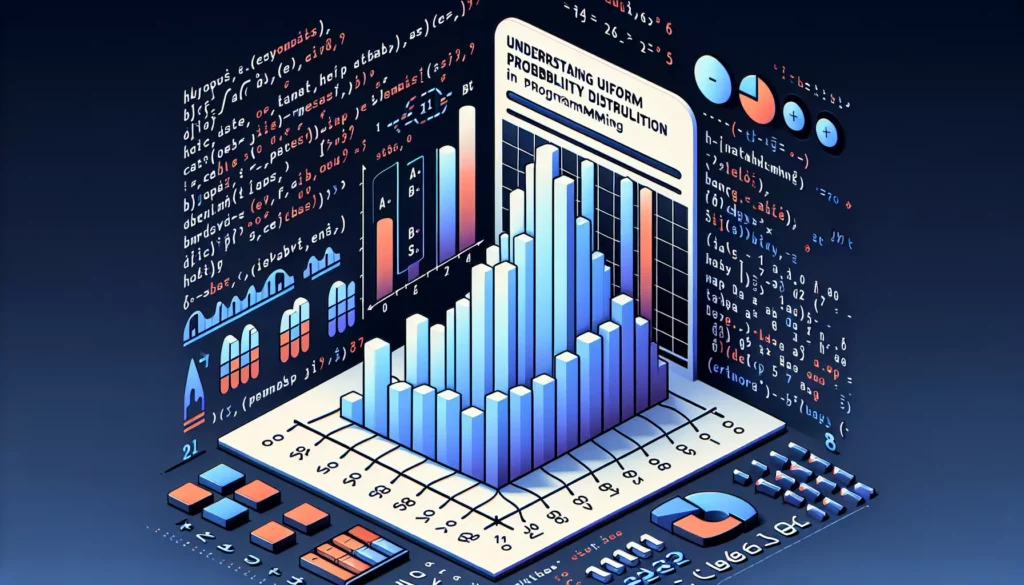
Probability distributions are fundamental concepts in statistics, data science, and computer science. Among these, the uniform probability distribution stands out for its simplicity and importance in various programming applications. Whether you’re developing simulations, implementing randomized algorithms, or working on machine learning models, understanding uniform probability distribution is essential.
In this comprehensive guide, we’ll explore what uniform probability distribution is, how it’s implemented in code, and its applications in programming and algorithmic problem-solving.
What is Uniform Probability Distribution?
A uniform probability distribution describes a situation where all outcomes in a sample space are equally likely to occur. It’s one of the simplest probability distributions to understand and implement.
There are two types of uniform distributions:
1. Discrete Uniform Distribution
In a discrete uniform distribution, a finite number of outcomes have the same probability of occurring. The classic example is rolling a fair die, where each number (1 through 6) has an equal 1/6 probability of appearing.
The probability mass function (PMF) for a discrete uniform distribution is:
P(X = x) = 1/n for each x in the sample space, where n is the number of possible outcomes.
2. Continuous Uniform Distribution
In a continuous uniform distribution, all intervals of the same length within the distribution’s support have equal probability. This is often referred to as a rectangular distribution because its probability density function (PDF) graph forms a rectangle.
The PDF for a continuous uniform distribution over interval [a, b] is:
f(x) = 1/(b-a) for a ≤ x ≤ b, and 0 elsewhere.
Implementing Uniform Distributions in Code
Most programming languages provide built-in functions to generate uniform random numbers. Let’s explore implementations in popular languages:
Python Implementation
Python’s random
module provides several functions for generating uniform random values:
import random
import numpy as np
import matplotlib.pyplot as plt
# Discrete uniform distribution (like rolling a die)
die_roll = random.randint(1, 6) # Returns a random integer between 1 and 6 (inclusive)
print(f"Die roll: {die_roll}")
# Continuous uniform distribution
random_value = random.uniform(0, 1) # Returns a random float between 0 and 1
print(f"Random value between 0 and 1: {random_value}")
# Generating multiple samples and visualizing
samples = [random.uniform(0, 10) for _ in range(1000)]
plt.hist(samples, bins=20)
plt.title("Uniform Distribution Samples")
plt.xlabel("Value")
plt.ylabel("Frequency")
plt.show()
Java Implementation
import java.util.Random;
public class UniformDistributionExample {
public static void main(String[] args) {
Random random = new Random();
// Discrete uniform (like rolling a die)
int dieRoll = random.nextInt(6) + 1; // Returns a random integer between 1 and 6
System.out.println("Die roll: " + dieRoll);
// Continuous uniform
double randomValue = random.nextDouble(); // Returns a random double between 0.0 and 1.0
System.out.println("Random value between 0 and 1: " + randomValue);
// Custom range for continuous uniform: a + (b-a) * random
double min = 5.0;
double max = 10.0;
double customRangeValue = min + (max - min) * random.nextDouble();
System.out.println("Random value between " + min + " and " + max + ": " + customRangeValue);
}
}
JavaScript Implementation
// Discrete uniform (like rolling a die)
function rollDie() {
return Math.floor(Math.random() * 6) + 1; // Returns a random integer between 1 and 6
}
console.log(`Die roll: ${rollDie()}`);
// Continuous uniform between 0 and 1
const randomValue = Math.random(); // Returns a random number between 0 (inclusive) and 1 (exclusive)
console.log(`Random value between 0 and 1: ${randomValue}`);
// Custom range for continuous uniform
function getRandomInRange(min, max) {
return min + (max - min) * Math.random();
}
console.log(`Random value between 5 and 10: ${getRandomInRange(5, 10)}`);
Applications of Uniform Probability Distribution in Programming
Understanding and implementing uniform distributions is crucial for many programming tasks:
1. Randomized Algorithms
Many efficient algorithms rely on randomization, which often uses uniform distributions:
- Quicksort with random pivot selection
- Randomized primality testing algorithms like Miller-Rabin
- Skip lists and other probabilistic data structures
Here’s an example of randomized quicksort in Python:
import random
def randomized_quicksort(arr):
if len(arr) <= 1:
return arr
# Choose a random pivot
pivot_index = random.randint(0, len(arr) - 1)
pivot = arr[pivot_index]
# Partition the array
less = [x for i, x in enumerate(arr) if x <= pivot and i != pivot_index]
greater = [x for x in arr if x > pivot]
# Recursively sort and combine
return randomized_quicksort(less) + [pivot] + randomized_quicksort(greater)
# Example usage
array = [3, 8, 2, 5, 1, 4, 7, 6]
sorted_array = randomized_quicksort(array)
print(f"Sorted array: {sorted_array}")
2. Monte Carlo Simulations
Monte Carlo methods use random sampling to obtain numerical results. They’re widely used in physics, finance, and computational biology.
Here’s a simple example estimating π using Monte Carlo simulation:
import random
import matplotlib.pyplot as plt
import numpy as np
def estimate_pi(num_samples):
points_inside_circle = 0
x_inside = []
y_inside = []
x_outside = []
y_outside = []
for _ in range(num_samples):
x = random.uniform(-1, 1)
y = random.uniform(-1, 1)
# Check if point is inside unit circle
if x**2 + y**2 <= 1:
points_inside_circle += 1
x_inside.append(x)
y_inside.append(y)
else:
x_outside.append(x)
y_outside.append(y)
# Area of circle / Area of square = π/4
# So π ≈ 4 * (points inside circle / total points)
pi_estimate = 4 * points_inside_circle / num_samples
# Visualization
plt.figure(figsize=(8, 8))
plt.scatter(x_inside, y_inside, color='blue', s=1)
plt.scatter(x_outside, y_outside, color='red', s=1)
plt.axis('equal')
plt.title(f"Monte Carlo Pi Estimation: {pi_estimate:.6f}")
return pi_estimate
# Estimate π with 10,000 samples
pi_approximation = estimate_pi(10000)
print(f"Estimated value of π: {pi_approximation}")
print(f"Actual value of π: {np.pi}")
3. Cryptography and Security
Uniform random number generation is crucial for cryptographic applications, although cryptographically secure random number generators are needed rather than standard PRNGs.
import secrets # More secure than the random module for cryptographic purposes
# Generate a random token
token = secrets.token_hex(16) # Generates a random hex string of 32 characters (16 bytes)
print(f"Random token: {token}")
# Generate a random number for cryptographic use
secure_random_number = secrets.randbelow(100) # Random integer in range [0, 100)
print(f"Secure random number: {secure_random_number}")
# Make a secure choice from a sequence
options = ['apple', 'banana', 'cherry', 'date', 'elderberry']
secure_choice = secrets.choice(options)
print(f"Secure random choice: {secure_choice}")
4. Game Development
Games frequently use uniform distributions for various mechanics:
- Dice rolls and card shuffling
- Random enemy spawning
- Procedural content generation
import random
class DiceGame:
def __init__(self, num_dice=2, sides_per_die=6):
self.num_dice = num_dice
self.sides_per_die = sides_per_die
def roll_dice(self):
return [random.randint(1, self.sides_per_die) for _ in range(self.num_dice)]
def play_round(self):
dice_values = self.roll_dice()
total = sum(dice_values)
print(f"You rolled: {dice_values}, Total: {total}")
# Simple win condition
if total == 7 or total == 11:
return "Win"
elif total == 2 or total == 3 or total == 12:
return "Lose"
else:
return f"Point is {total}"
# Play a few rounds
game = DiceGame()
for _ in range(5):
result = game.play_round()
print(f"Result: {result}\n")
Common Pitfalls and Best Practices
When working with uniform distributions in programming, be aware of these common issues:
1. Modulo Bias
Using the modulo operator to generate random numbers within a range can introduce bias if not done carefully:
# Incorrect way (can introduce bias)
def biased_random(min_val, max_val):
range_size = max_val - min_val + 1
return min_val + (random.randint(0, 1000000) % range_size)
# Correct way
def unbiased_random(min_val, max_val):
return random.randint(min_val, max_val)
2. Seed Management
For reproducible results (important in testing and scientific computing), manage your random seeds carefully:
import random
import numpy as np
# Set seed for reproducibility
random_seed = 42
random.seed(random_seed)
np.random.seed(random_seed)
# Now random operations will produce the same results each time the program runs
print(random.random())
print(random.random())
# Reset with a different seed for a different sequence
random.seed(100)
print(random.random())
3. Using the Right Distribution
Not all random processes should use uniform distributions. Consider whether other distributions (normal, exponential, etc.) better model your problem:
import numpy as np
import matplotlib.pyplot as plt
# Generate samples from different distributions
uniform_samples = np.random.uniform(0, 1, 1000)
normal_samples = np.random.normal(0, 1, 1000)
exponential_samples = np.random.exponential(1, 1000)
# Plot to compare
plt.figure(figsize=(15, 5))
plt.subplot(1, 3, 1)
plt.hist(uniform_samples, bins=30)
plt.title("Uniform Distribution")
plt.subplot(1, 3, 2)
plt.hist(normal_samples, bins=30)
plt.title("Normal Distribution")
plt.subplot(1, 3, 3)
plt.hist(exponential_samples, bins=30)
plt.title("Exponential Distribution")
plt.tight_layout()
plt.show()
Interview Problems Involving Uniform Probability Distribution
Technical interviews at major tech companies often include probability problems that involve uniform distributions. Here are some examples:
Problem 1: Random Point in a Circle
Generate a random point uniformly distributed inside a unit circle.
import random
import math
import matplotlib.pyplot as plt
def random_point_in_circle():
# Incorrect approach (creates bias toward the center)
# x = random.uniform(-1, 1)
# y = random.uniform(-1, 1)
# if x*x + y*y > 1:
# return random_point_in_circle()
# return (x, y)
# Correct approach: use polar coordinates
r = math.sqrt(random.uniform(0, 1)) # Square root for uniform distribution in the area
theta = random.uniform(0, 2 * math.pi)
x = r * math.cos(theta)
y = r * math.sin(theta)
return (x, y)
# Generate and plot points
points = [random_point_in_circle() for _ in range(1000)]
x_coords, y_coords = zip(*points)
plt.figure(figsize=(8, 8))
plt.scatter(x_coords, y_coords, s=5)
plt.axis('equal')
plt.title("Uniform Random Points in a Circle")
plt.show()
Problem 2: Implement a Function That Returns 1 with Probability p
Given a function that returns 1 with probability 0.5 and 0 otherwise, implement a function that returns 1 with probability p.
import random
# Given function that returns 1 with probability 0.5
def flip_coin():
return random.randint(0, 1)
# Implement a function that returns 1 with probability p
def biased_coin(p):
# Convert p to binary representation
# For example, 0.625 = 0.101 in binary = 1/2 + 0/4 + 1/8
# Special cases
if p == 0:
return 0
if p == 1:
return 1
# Generate a random number with enough precision
r = 0
for i in range(32): # Use 32 bits of precision
r = r / 2 + flip_coin() / 2
return 1 if r < p else 0
# Test the function
def test_biased_coin(p, num_trials=10000):
successes = sum(biased_coin(p) for _ in range(num_trials))
observed_p = successes / num_trials
print(f"Target probability: {p}, Observed probability: {observed_p}")
# Test with different probabilities
test_biased_coin(0.3)
test_biased_coin(0.5)
test_biased_coin(0.8)
Conclusion
Uniform probability distribution is a foundational concept that appears throughout computer science and programming. From basic randomization to complex simulations and algorithms, the ability to generate and work with uniform random values is an essential skill for programmers.
By understanding the principles behind uniform distributions and their implementations in code, you’ll be better equipped to solve a wide range of programming problems, optimize algorithms, and ace technical interviews at top tech companies.
As you continue your programming journey, consider how probability and randomness can be leveraged in your projects. Often, the right application of randomization can lead to elegant solutions for otherwise complex problems.
Practice implementing the examples in this guide and try to solve the interview problems to strengthen your understanding of uniform probability distributions in programming contexts.