Understanding Typed vs. Dynamic Programming Languages: A Comprehensive Guide
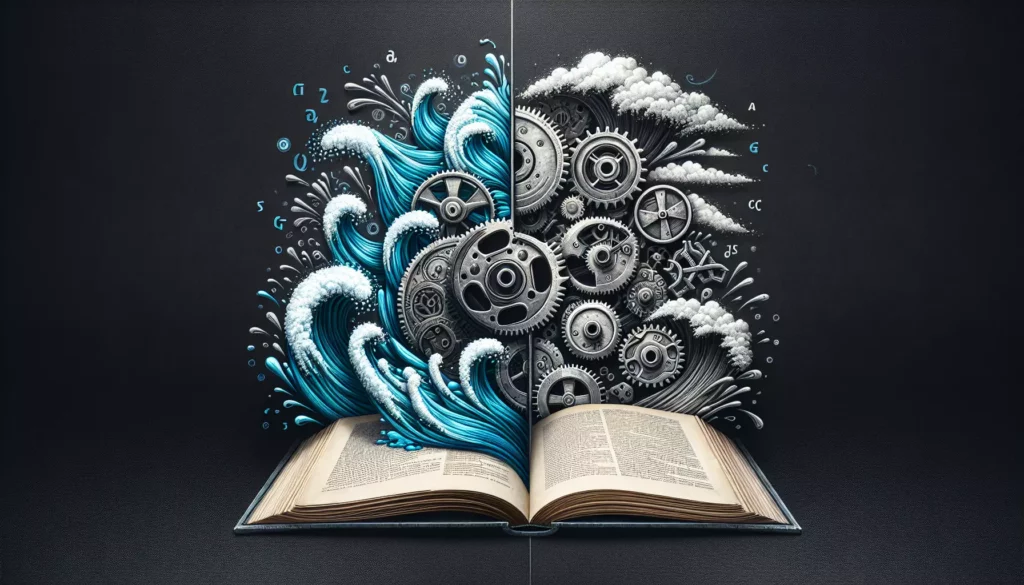
In the world of programming, one of the fundamental distinctions between languages is whether they are typed or dynamic. This distinction plays a crucial role in how code is written, executed, and maintained. As aspiring developers or those preparing for technical interviews at major tech companies, understanding these differences is essential. In this comprehensive guide, we’ll dive deep into the world of typed and dynamic programming languages, exploring their characteristics, advantages, disadvantages, and real-world applications.
What are Typed Programming Languages?
Typed programming languages, also known as statically-typed languages, require variables to be declared with specific data types before they can be used. The type checking is done at compile-time, which means that the compiler verifies that the types are used correctly before the program is executed.
Characteristics of Typed Languages:
- Variables must be declared with a specific type
- Type checking occurs at compile-time
- Type errors are caught early in the development process
- Generally offer better performance due to optimizations based on type information
- Provide clearer code documentation through type annotations
Examples of Typed Languages:
- Java
- C++
- Rust
- Go
- TypeScript (a typed superset of JavaScript)
Advantages of Typed Languages:
- Early Error Detection: Type errors are caught at compile-time, reducing runtime errors.
- Improved Performance: The compiler can optimize code based on type information.
- Better Code Documentation: Type annotations serve as built-in documentation.
- Enhanced IDE Support: IDEs can provide better code completion and refactoring tools.
- Easier Maintenance: Types make it easier to understand and maintain large codebases.
Disadvantages of Typed Languages:
- Verbosity: Code can be more verbose due to type declarations.
- Longer Development Time: Initial development may take longer due to the need for type declarations.
- Less Flexibility: Strict typing can sometimes limit flexibility in code design.
What are Dynamic Programming Languages?
Dynamic programming languages, also known as dynamically-typed languages, do not require explicit type declarations. Variables can hold values of any type, and type checking is done at runtime.
Characteristics of Dynamic Languages:
- Variables do not need to be declared with a specific type
- Type checking occurs at runtime
- More flexible and allows for rapid prototyping
- Generally easier to learn and write code quickly
- Can be more expressive and concise
Examples of Dynamic Languages:
- Python
- JavaScript
- Ruby
- PHP
- Lua
Advantages of Dynamic Languages:
- Rapid Development: Faster initial development due to less boilerplate code.
- Flexibility: Easier to work with different data types and structures.
- Concise Code: Often results in shorter, more readable code.
- Easy Prototyping: Ideal for quick prototyping and experimentation.
- Duck Typing: Allows for more generic and reusable code.
Disadvantages of Dynamic Languages:
- Runtime Errors: Type errors may only be discovered during program execution.
- Performance Overhead: Dynamic type checking can lead to slower execution.
- Less Explicit Code: Lack of type information can make code harder to understand in large projects.
- Limited Compiler Optimizations: Fewer opportunities for compile-time optimizations.
Typed vs. Dynamic Languages: A Comparative Analysis
To better understand the differences between typed and dynamic languages, let’s compare them across various aspects:
1. Type Safety
Typed Languages: Offer stronger type safety, catching type-related errors at compile-time.
Dynamic Languages: Provide more flexibility but may allow type-related errors to slip through to runtime.
2. Performance
Typed Languages: Generally offer better performance due to compile-time optimizations and lack of runtime type checking.
Dynamic Languages: May have performance overhead due to runtime type checking and dynamic dispatch.
3. Development Speed
Typed Languages: Initial development may be slower due to the need for type declarations, but can lead to faster development in large, long-term projects.
Dynamic Languages: Often allow for faster initial development and prototyping.
4. Code Readability
Typed Languages: Type annotations can serve as documentation, making code more self-explanatory.
Dynamic Languages: Can be more concise, but may require additional documentation for clarity in larger projects.
5. Flexibility
Typed Languages: Less flexible, but provide more structure and safety.
Dynamic Languages: More flexible, allowing for easier manipulation of different data types and structures.
6. Tooling Support
Typed Languages: Often have better IDE support, including more accurate code completion and refactoring tools.
Dynamic Languages: IDE support has improved, but may still lag behind typed languages in some areas.
Real-World Applications and Use Cases
Both typed and dynamic languages have their place in the software development ecosystem. Let’s explore some common use cases for each:
Typed Languages:
- Large-Scale Enterprise Applications: Java and C# are often used for building complex, large-scale enterprise systems where type safety and maintainability are crucial.
- System Programming: Languages like C++ and Rust are preferred for system-level programming, operating systems, and embedded systems where performance and memory safety are critical.
- Game Development: C++ is widely used in game engines and high-performance game development due to its speed and low-level control.
- Financial Systems: Typed languages are often chosen for financial applications where precision and type safety are paramount.
Dynamic Languages:
- Web Development: JavaScript is the de facto language for client-side web development, while Python and Ruby are popular for server-side development and web frameworks.
- Data Analysis and Machine Learning: Python has become the go-to language for data science and machine learning due to its flexibility and rich ecosystem of libraries.
- Scripting and Automation: Python, Ruby, and Perl are commonly used for scripting, automation, and rapid prototyping.
- Startups and MVPs: Dynamic languages often allow startups to quickly develop and iterate on minimum viable products (MVPs).
Code Examples: Typed vs. Dynamic Languages
To illustrate the differences between typed and dynamic languages, let’s look at some code examples:
Typed Language Example (Java):
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public static void main(String[] args) {
Person person = new Person("Alice", 30);
System.out.println(person.getName() + " is " + person.getAge() + " years old.");
// This would cause a compile-time error:
// person.age = "Thirty";
}
}
Dynamic Language Example (Python):
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def get_name(self):
return self.name
def get_age(self):
return self.age
person = Person("Alice", 30)
print(f"{person.get_name()} is {person.get_age()} years old.")
# This is allowed in Python, but might cause runtime errors later:
person.age = "Thirty"
In the Java example, the types are explicitly declared, and attempting to assign a string to the age
field would result in a compile-time error. In the Python example, no type declarations are needed, and you can freely change the type of the age
attribute, which might lead to runtime errors if not handled carefully.
The Rise of Gradual Typing
In recent years, there has been a trend towards gradual typing, which aims to combine the benefits of both typed and dynamic languages. Gradual typing allows developers to add type annotations to dynamic languages incrementally, providing a middle ground between the two approaches.
Examples of Gradual Typing:
- TypeScript: A typed superset of JavaScript that compiles to plain JavaScript.
- Python with Type Hints: Python 3.5+ supports optional type annotations.
- Hack: A gradually typed version of PHP developed by Facebook.
Gradual typing offers several advantages:
- Allows for incremental adoption of typing in existing projects
- Combines the flexibility of dynamic languages with the safety of typed languages
- Improves code documentation and IDE support
- Enables better tooling and static analysis
Example of Gradual Typing (Python with Type Hints):
from typing import List, Dict
def process_data(data: List[Dict[str, int]]) -> int:
total = 0
for item in data:
total += item.get("value", 0)
return total
# Usage
data = [{"value": 10}, {"value": 20}, {"value": 30}]
result = process_data(data)
print(f"Total: {result}")
In this example, we’ve added type hints to the process_data
function, specifying that it takes a list of dictionaries with string keys and integer values, and returns an integer. These type hints don’t affect the runtime behavior but can be used by IDEs and static analysis tools to catch potential errors and provide better code completion.
Choosing Between Typed and Dynamic Languages
When deciding between typed and dynamic languages for a project, consider the following factors:
- Project Size and Complexity: Larger, more complex projects often benefit from the structure and safety of typed languages, while smaller projects or prototypes might favor the flexibility of dynamic languages.
- Team Size and Experience: Larger teams or those with varying levels of experience might benefit from the explicit nature of typed languages, while smaller, experienced teams might prefer the conciseness of dynamic languages.
- Performance Requirements: If performance is critical, typed languages generally have an advantage.
- Development Speed: For rapid prototyping or MVP development, dynamic languages often allow for faster initial development.
- Maintainability: Long-term maintainability is often easier with typed languages due to their self-documenting nature and compile-time checks.
- Ecosystem and Libraries: Consider the available libraries and tools for your specific domain in each language.
- Learning Curve: Dynamic languages are often easier for beginners to learn, while typed languages may have a steeper learning curve but provide more structure for learning proper programming practices.
Conclusion
Understanding the differences between typed and dynamic programming languages is crucial for developers, especially those preparing for technical interviews at major tech companies. Both approaches have their strengths and weaknesses, and the choice between them often depends on the specific requirements of the project and the preferences of the development team.
Typed languages offer stronger guarantees about code correctness and often lead to better performance, making them suitable for large-scale, complex applications where reliability and efficiency are paramount. Dynamic languages, on the other hand, provide greater flexibility and faster development cycles, making them ideal for rapid prototyping, scripting, and domains where adaptability is key.
The trend towards gradual typing demonstrates the industry’s recognition of the benefits of both approaches and the desire to find a middle ground that combines their strengths. As you continue your journey in programming and prepare for technical interviews, make sure to gain experience with both typed and dynamic languages, understanding their trade-offs and appropriate use cases.
Remember, the best language for a given task depends on various factors, and a skilled developer should be comfortable working with both paradigms. By mastering both typed and dynamic languages, you’ll be well-equipped to tackle a wide range of programming challenges and excel in technical interviews at top tech companies.