Understanding Time Complexity in Coding Challenges
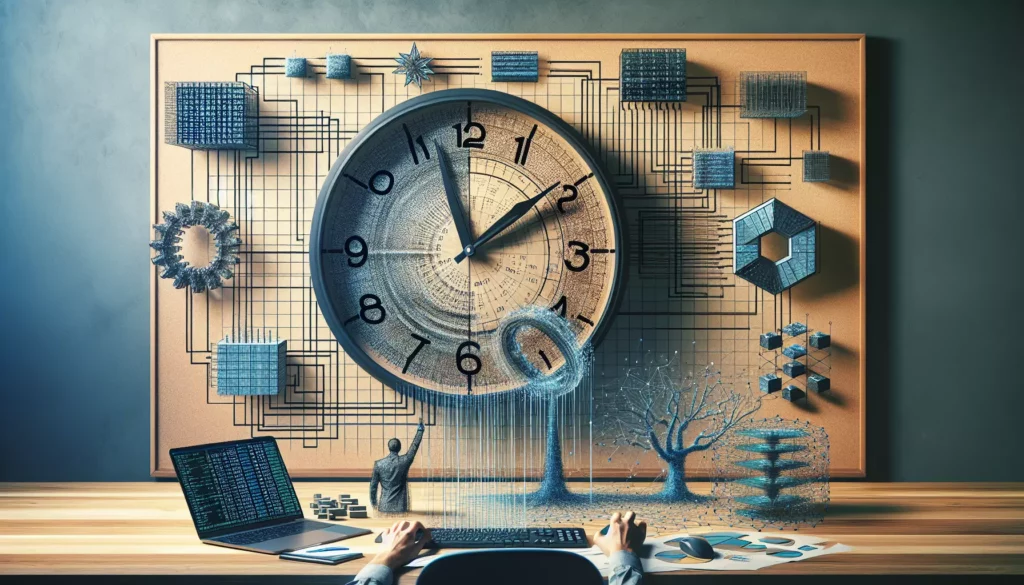
In the world of competitive programming and technical interviews, particularly for major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), understanding time complexity is crucial. It’s not just about writing code that works; it’s about writing efficient code that can handle large-scale problems. This is where time complexity comes into play. In this comprehensive guide, we’ll dive deep into the concept of time complexity, why it matters, and how to analyze and optimize your code for better performance.
What is Time Complexity?
Time complexity is a measure of how the runtime of an algorithm grows as the input size increases. It’s typically expressed using Big O notation, which describes the upper bound of the growth rate of an algorithm’s execution time. Understanding time complexity helps programmers predict how their code will perform with different input sizes and optimize their algorithms for better efficiency.
Why is Time Complexity Important?
In coding challenges and real-world applications, efficiency matters. An algorithm that works well for small inputs might become impractical or even unusable for larger datasets. By understanding time complexity, you can:
- Predict how your code will perform with different input sizes
- Compare different algorithms and choose the most efficient one for your specific problem
- Optimize your code to handle larger datasets
- Meet performance requirements in technical interviews and real-world applications
Common Time Complexities
Let’s explore some of the most common time complexities you’ll encounter in coding challenges:
O(1) – Constant Time
An algorithm with O(1) time complexity performs a fixed number of operations, regardless of the input size. These are typically the most efficient algorithms.
Example: Accessing an element in an array by its index.
def get_element(arr, index):
return arr[index] # O(1) time complexity
O(log n) – Logarithmic Time
Algorithms with O(log n) time complexity divide the problem in half with each step. These are very efficient, especially for large datasets.
Example: Binary search in a sorted array.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # O(log n) time complexity
O(n) – Linear Time
An O(n) algorithm’s runtime grows linearly with the input size. These are generally considered efficient for most problems.
Example: Finding the maximum element in an unsorted array.
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val # O(n) time complexity
O(n log n) – Linearithmic Time
Algorithms with O(n log n) time complexity are often seen in efficient sorting algorithms. They’re more efficient than quadratic time but less efficient than linear time.
Example: Merge sort algorithm.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right) # O(n log n) time complexity
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
O(n^2) – Quadratic Time
Algorithms with O(n^2) time complexity have nested iterations over the input. They can become slow for larger inputs.
Example: Bubble sort algorithm.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr # O(n^2) time complexity
O(2^n) – Exponential Time
Exponential time algorithms have a runtime that doubles with each additional input element. These are typically seen in recursive algorithms solving complex problems.
Example: Recursive Fibonacci sequence calculation.
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2) # O(2^n) time complexity
Analyzing Time Complexity
To analyze the time complexity of an algorithm, follow these steps:
- Identify the basic operations: Determine which operations are performed most frequently in your algorithm.
- Count the number of basic operations: Estimate how many times these operations are executed in relation to the input size.
- Express the count as a function of input size: Use variables like n to represent the input size.
- Simplify the expression: Remove constant factors and lower-order terms, focusing on the dominant term.
- Express the result in Big O notation: Use the simplified expression to determine the time complexity.
Example: Analyzing a Simple Loop
def sum_array(arr):
total = 0
for num in arr:
total += num
return total
Analysis:
- The basic operation is the addition inside the loop.
- This operation is performed once for each element in the array.
- If n is the length of the array, the operation is performed n times.
- The time complexity is O(n), as it grows linearly with the input size.
Common Pitfalls in Time Complexity Analysis
When analyzing time complexity, be aware of these common pitfalls:
1. Ignoring Constants
While we typically ignore constants in Big O notation, they can be significant for smaller inputs. For example, an O(n) algorithm might be slower than an O(n^2) algorithm for small n if the constant factor is much larger.
2. Focusing Only on the Worst Case
While Big O notation represents the upper bound (worst case), it’s also important to consider average-case performance, especially for real-world applications.
3. Overlooking Space Complexity
Time complexity is crucial, but don’t forget about space complexity. An algorithm might be time-efficient but impractical due to high memory usage.
4. Misidentifying Nested Loops
Be careful with nested loops. Two nested loops don’t always mean O(n^2) complexity. For example, if the inner loop runs a fixed number of times, the complexity might still be O(n).
Optimizing for Better Time Complexity
Improving the time complexity of your algorithms is a key skill for coding challenges and technical interviews. Here are some strategies to optimize your code:
1. Use Appropriate Data Structures
Choosing the right data structure can significantly impact your algorithm’s efficiency. For example, using a hash table for lookups instead of a list can reduce time complexity from O(n) to O(1).
2. Avoid Unnecessary Computations
Look for opportunities to reduce redundant calculations. Techniques like memoization can help avoid recomputing values in recursive algorithms.
3. Divide and Conquer
Break down complex problems into smaller, manageable subproblems. This approach often leads to more efficient algorithms, like in merge sort (O(n log n)) compared to simpler sorting algorithms like bubble sort (O(n^2)).
4. Use Dynamic Programming
For problems with overlapping subproblems, dynamic programming can significantly reduce time complexity by storing and reusing intermediate results.
5. Optimize Loops
Look for ways to combine or eliminate loops. Sometimes, multiple passes through data can be combined into a single pass.
Real-World Application: Optimizing a Frequency Counter
Let’s look at a practical example of optimizing an algorithm for better time complexity. We’ll create a function to count the frequency of elements in an array.
Initial Implementation (O(n^2)):
def count_frequency(arr):
result = {}
for num in arr:
count = 0
for item in arr:
if item == num:
count += 1
result[num] = count
return result # O(n^2) time complexity
This implementation uses nested loops, resulting in O(n^2) time complexity.
Optimized Implementation (O(n)):
def count_frequency_optimized(arr):
result = {}
for num in arr:
if num in result:
result[num] += 1
else:
result[num] = 1
return result # O(n) time complexity
This optimized version uses a single loop and a dictionary for constant-time lookups, reducing the time complexity to O(n).
Practice Problems
To reinforce your understanding of time complexity, try solving these practice problems:
- Implement a function to find the kth largest element in an unsorted array. Optimize for time complexity.
- Write an algorithm to determine if a string has all unique characters. What is the time complexity of your solution?
- Implement a function to find the longest palindromic substring in a given string. Analyze its time complexity.
- Create an algorithm to find the missing number in an array containing n distinct numbers taken from 0, 1, 2, …, n. What’s the most efficient time complexity you can achieve?
- Implement a function to reverse a linked list. Analyze its time and space complexity.
Conclusion
Understanding time complexity is a fundamental skill for any programmer, especially those preparing for technical interviews at top tech companies. It allows you to write more efficient code, optimize algorithms, and tackle complex problems with confidence. As you practice coding challenges, always consider the time complexity of your solutions and look for ways to improve efficiency.
Remember, the goal isn’t always to achieve the lowest possible time complexity. Sometimes, a slightly less efficient algorithm might be more readable or easier to implement. The key is to understand the trade-offs and make informed decisions based on the specific requirements of your problem.
Continue practicing, analyzing different algorithms, and challenging yourself with complex problems. With time and experience, evaluating and optimizing time complexity will become second nature, making you a more skilled and valuable programmer.