Understanding the Take-Home Coding Assignment Interview
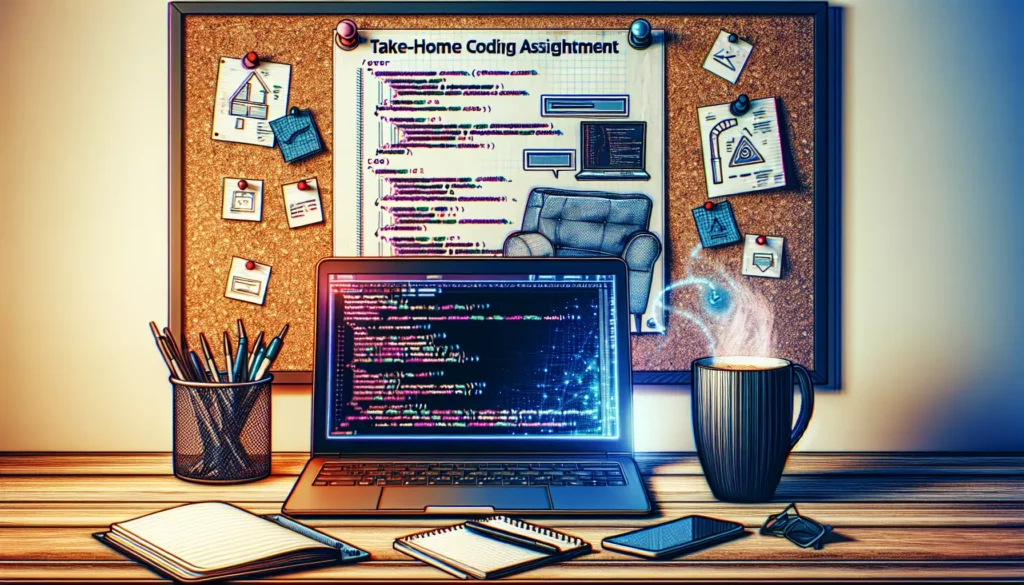
In the ever-evolving landscape of technical interviews, the take-home coding assignment has become an increasingly popular method for assessing a candidate’s skills. This approach offers both advantages and challenges for job seekers in the software development field. In this comprehensive guide, we’ll delve into the intricacies of take-home coding assignments, exploring their purpose, best practices, and how to excel in this crucial step of the interview process.
What is a Take-Home Coding Assignment?
A take-home coding assignment is a practical test given to job candidates during the interview process for software development positions. Unlike traditional whiteboard interviews or live coding sessions, take-home assignments allow candidates to work on a project in their own environment and on their own time. These assignments typically involve building a small application, solving a specific problem, or implementing a particular feature.
Key Characteristics of Take-Home Assignments:
- Time-boxed: Usually given a specific timeframe (e.g., 24-72 hours)
- Realistic: Often reflect real-world scenarios or problems
- Open-ended: Allow for creativity and showcase problem-solving skills
- Technology-specific: May require use of particular programming languages or frameworks
The Purpose of Take-Home Coding Assignments
Take-home coding assignments serve several purposes in the hiring process:
- Skill Assessment: They provide a more comprehensive view of a candidate’s coding abilities, problem-solving skills, and attention to detail.
- Work Style Evaluation: Employers can gauge how candidates approach tasks, manage time, and handle project requirements.
- Code Quality Review: Assignments allow for a thorough examination of code structure, cleanliness, and best practices.
- Reducing Interview Bias: By focusing on actual work output, take-home assignments can help mitigate some forms of interview bias.
Advantages of Take-Home Coding Assignments
For both candidates and employers, take-home assignments offer several benefits:
For Candidates:
- Reduced pressure compared to live coding interviews
- Opportunity to showcase skills in a familiar environment
- More time to think through problems and optimize solutions
- Chance to demonstrate coding style and documentation practices
For Employers:
- More realistic assessment of a candidate’s capabilities
- Ability to evaluate code quality and project structure
- Insight into a candidate’s time management and project planning skills
- Standardized comparison between candidates
Common Challenges and How to Overcome Them
While take-home assignments have many advantages, they also present some challenges:
1. Time Management
Challenge: Balancing the assignment with other commitments can be difficult.
Solution: Plan your time carefully. Break the project into smaller tasks and set mini-deadlines for each. Use time management techniques like the Pomodoro method to stay focused and productive.
2. Scope Creep
Challenge: It’s easy to get carried away and add unnecessary features.
Solution: Stick to the requirements provided. If you have extra time, focus on polishing existing features, improving code quality, or adding thorough documentation rather than implementing additional functionality.
3. Technical Issues
Challenge: Unfamiliarity with required technologies or unexpected technical problems can arise.
Solution: Before starting, ensure you have all necessary tools and technologies set up. If issues occur, communicate promptly with the hiring team and document any workarounds you implement.
4. Perfectionism
Challenge: Striving for perfection can lead to missed deadlines or incomplete work.
Solution: Aim for a minimum viable product (MVP) first. Once you have a working solution, iterate and improve if time allows. Remember, done is better than perfect in most cases.
Best Practices for Take-Home Coding Assignments
To maximize your chances of success, follow these best practices:
1. Read and Understand the Requirements
Carefully review the assignment instructions. If anything is unclear, don’t hesitate to ask for clarification from the hiring team. Understanding the requirements fully before you begin can save time and prevent misunderstandings.
2. Plan Before You Code
Spend time planning your approach. Create a basic design or architecture for your solution. This can include:
- Sketching out the main components or classes
- Deciding on the overall structure of your code
- Identifying potential challenges and how you’ll address them
3. Write Clean, Readable Code
Your code should be easy to read and understand. Follow these guidelines:
- Use meaningful variable and function names
- Keep functions small and focused on a single task
- Comment your code where necessary, but aim for self-documenting code
- Follow consistent formatting and style guidelines
4. Implement Error Handling
Robust error handling demonstrates foresight and attention to detail. Consider potential edge cases and how your code will handle unexpected inputs or situations.
5. Write Tests
Including unit tests or integration tests shows that you value code quality and reliability. Even if not explicitly required, adding tests can set your submission apart.
6. Document Your Work
Provide clear documentation for your project. This should include:
- Setup instructions
- How to run the application
- Any assumptions you made
- Explanations for significant design decisions
- Known limitations or areas for improvement
7. Use Version Control
Utilize a version control system like Git. This demonstrates your familiarity with professional development practices and allows you to track your progress.
8. Manage Your Time Effectively
Set milestones for yourself and try to stick to them. If you’re running out of time, focus on delivering core functionality rather than trying to implement every feature perfectly.
9. Review and Refactor
If time permits, review your code and refactor where necessary. Look for opportunities to improve efficiency, readability, or structure.
10. Double-Check Submission Requirements
Before submitting, ensure you’ve met all the requirements, including file formats, naming conventions, and submission methods specified by the employer.
Example Take-Home Coding Assignment
Let’s look at a simplified example of a take-home coding assignment to better understand what you might encounter:
Assignment: Build a Simple Todo List Application
Requirements:
1. Create a web-based todo list application using [specified technology stack].
2. Users should be able to add, edit, and delete tasks.
3. Tasks should have a title, description, due date, and status (completed/incomplete).
4. Implement basic filtering (e.g., show only incomplete tasks).
5. Store tasks in a backend database of your choice.
6. Provide API documentation for your backend endpoints.
7. Include unit tests for key components.
8. Submit your code via a GitHub repository with setup instructions in the README.
Time Limit: 48 hours
Evaluation Criteria:
- Functionality: Does the application meet all requirements?
- Code Quality: Is the code well-structured, readable, and maintainable?
- Testing: Are there sufficient and meaningful tests?
- Documentation: Is the project well-documented, including setup instructions and API documentation?
- Design: Is the user interface intuitive and responsive?
This example demonstrates the typical scope and expectations of a take-home coding assignment. It’s specific enough to guide the candidate but open-ended enough to allow for creativity and showcase problem-solving skills.
How to Approach This Example Assignment
Here’s a step-by-step approach to tackling this assignment:
- Plan Your Approach: Sketch out the basic architecture, deciding on frontend framework, backend structure, and database choice.
- Set Up Your Development Environment: Initialize your project, set up version control, and create a basic project structure.
- Implement Core Functionality: Start with the CRUD operations for tasks, ensuring you meet the basic requirements.
- Add Filtering Functionality: Implement the ability to filter tasks based on their status.
- Design and Implement the User Interface: Create a simple, intuitive UI for interacting with the todo list.
- Write Unit Tests: Add tests for your key components and functions.
- Create API Documentation: Document your backend endpoints, including request/response formats.
- Refine and Polish: Review your code, refactor where necessary, and ensure everything works smoothly.
- Write the README: Provide clear setup instructions and any other relevant information about your project.
- Final Review and Submission: Do a final check against the requirements and submit your repository link.
Common Mistakes to Avoid
When working on take-home coding assignments, be aware of these common pitfalls:
1. Overcomplicating the Solution
While it’s tempting to showcase all your skills, remember that the assignment is looking for a specific set of competencies. Stick to the requirements and avoid adding unnecessary complexity.
2. Neglecting Error Handling
Robust error handling is often overlooked but is crucial in demonstrating your ability to write production-ready code. Consider various edge cases and how your application will handle them.
3. Ignoring Code Organization and Structure
Even for small projects, maintaining a clear and logical code structure is important. Use appropriate design patterns and separate concerns in your codebase.
4. Skipping Documentation
Failing to provide adequate documentation can significantly impact the perception of your work. Ensure your code is well-commented and include a comprehensive README file.
5. Not Managing Time Effectively
Poor time management can result in incomplete submissions or rushed work. Plan your time carefully and prioritize core requirements over additional features.
6. Disregarding Performance Considerations
While small-scale assignments may not require heavy optimization, showing awareness of performance implications in your code can be impressive.
7. Submitting Without Testing
Always test your submission thoroughly before sending it. This includes not just unit tests, but also manual testing to ensure everything works as expected.
After Submission: The Follow-Up
Once you’ve submitted your take-home coding assignment, the process isn’t over. Here’s what typically happens next and how you can prepare:
1. Code Review Discussion
Many companies will schedule a follow-up interview to discuss your code. Be prepared to:
- Explain your design decisions and trade-offs
- Discuss alternative approaches you considered
- Talk about challenges you faced and how you overcame them
- Suggest improvements or extensions to your solution
2. Technical Deep Dive
Expect detailed questions about your implementation. Reviewers might ask about:
- Specific parts of your code and why you chose certain approaches
- How you would scale your solution for larger datasets or user bases
- Security considerations in your implementation
- Performance optimizations you could make
3. Reflection and Learning
Use this experience as a learning opportunity:
- Reflect on what you could have done differently or better
- Consider how you managed your time and process
- Think about how this assignment relates to real-world scenarios you might encounter in the role
4. Seeking Feedback
Whether you move forward in the process or not, don’t hesitate to ask for feedback on your submission. This can provide valuable insights for future assignments and interviews.
Conclusion
Take-home coding assignments are a valuable tool in the modern technical interview process, offering candidates a chance to showcase their skills in a more realistic and less pressured environment. By understanding the purpose of these assignments, following best practices, and avoiding common pitfalls, you can significantly increase your chances of success.
Remember, the key to excelling in take-home assignments is not just about writing code that works, but demonstrating your problem-solving approach, attention to detail, and ability to deliver a professional-quality product. Approach each assignment as an opportunity to learn and improve, and you’ll find that they become an enjoyable and rewarding part of your job search journey.
As you continue to develop your skills and prepare for technical interviews, consider leveraging resources like AlgoCademy. With its focus on algorithmic thinking, problem-solving, and practical coding skills, AlgoCademy can be an invaluable tool in honing the exact skills that take-home coding assignments are designed to evaluate. By combining hands-on practice with guided learning, you’ll be well-equipped to tackle any coding challenge that comes your way in your pursuit of a career in software development.