Understanding the RSA Algorithm for Encryption
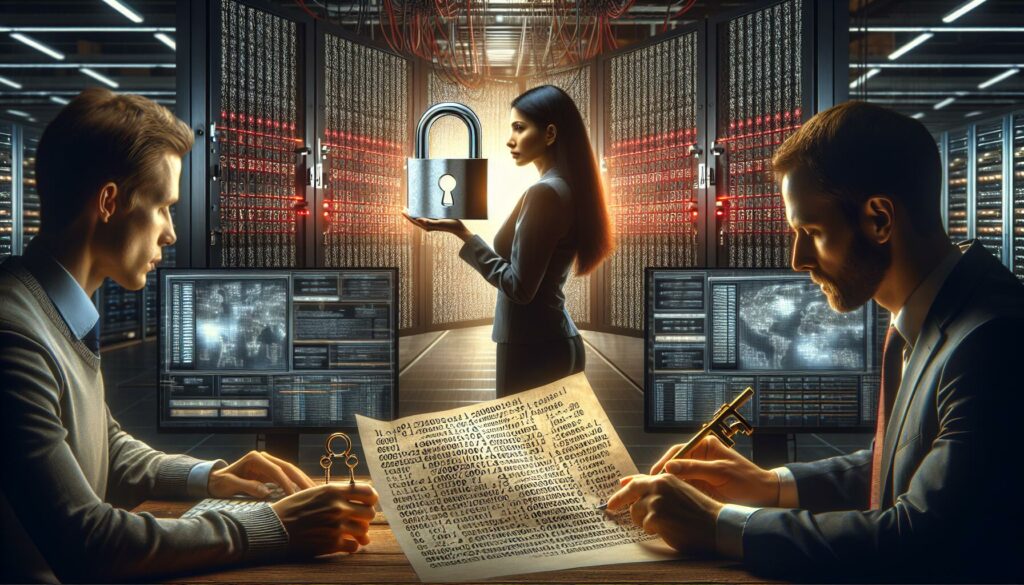
In the world of cybersecurity and cryptography, the RSA (Rivest-Shamir-Adleman) algorithm stands as one of the most widely used and respected encryption methods. Named after its creators Ron Rivest, Adi Shamir, and Leonard Adleman, RSA has been a cornerstone of secure communication since its introduction in 1977. In this comprehensive guide, we’ll delve deep into the RSA algorithm, exploring its principles, implementation, and significance in modern cryptography.
What is the RSA Algorithm?
The RSA algorithm is an asymmetric cryptographic algorithm, which means it uses two different keys: a public key for encryption and a private key for decryption. This dual-key system is what makes RSA particularly secure and useful for various applications, including secure data transmission, digital signatures, and key exchange protocols.
At its core, RSA relies on the practical difficulty of factoring the product of two large prime numbers. This mathematical problem forms the basis of RSA’s security, as breaking the encryption would require solving this factorization problem, which becomes exponentially more difficult as the size of the numbers increases.
The Mathematics Behind RSA
To truly understand RSA, we need to explore the mathematical principles that underpin its functionality. Let’s break down the key components:
1. Prime Number Selection
The process begins with the selection of two large prime numbers, typically referred to as p and q. These numbers should be kept secret and are used to generate both the public and private keys.
2. Modulus Calculation
The modulus, n, is calculated by multiplying p and q:
n = p * q
This modulus is part of both the public and private keys and is used in the encryption and decryption processes.
3. Euler’s Totient Function
The next step involves calculating Euler’s totient function, φ(n). For a number n that is the product of two primes p and q, the totient is given by:
φ(n) = (p - 1) * (q - 1)
This value is crucial for generating the public and private exponents.
4. Public Exponent Selection
A public exponent, e, is chosen such that:
- 1 < e < φ(n)
- e is coprime to φ(n) (their greatest common divisor is 1)
Commonly, e is set to 65537, as it’s a prime number that satisfies these conditions and offers a good balance between security and efficiency.
5. Private Exponent Calculation
The private exponent, d, is calculated using the modular multiplicative inverse of e modulo φ(n):
d ≡ e^(-1) mod φ(n)
In other words, d is the number that satisfies the equation:
e * d ≡ 1 mod φ(n)
6. Key Generation
With these calculations complete, we now have:
- Public Key: (n, e)
- Private Key: (n, d)
The public key can be freely distributed, while the private key must be kept secret.
RSA Encryption and Decryption Process
Now that we understand the key components, let’s look at how RSA actually encrypts and decrypts messages.
Encryption
To encrypt a message m, the sender uses the recipient’s public key (n, e). The encryption formula is:
c ≡ m^e mod n
Where:
- c is the ciphertext (encrypted message)
- m is the plaintext (original message)
- e is the public exponent
- n is the modulus
Decryption
To decrypt the ciphertext c, the recipient uses their private key (n, d). The decryption formula is:
m ≡ c^d mod n
Where:
- m is the recovered plaintext
- c is the ciphertext
- d is the private exponent
- n is the modulus
Implementing RSA in Code
To better understand how RSA works in practice, let’s implement a simple version of the algorithm in Python. Note that this implementation is for educational purposes only and should not be used for actual encryption in production environments.
import random
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
def generate_prime(min_value, max_value):
prime = random.randint(min_value, max_value)
while not is_prime(prime):
prime = random.randint(min_value, max_value)
return prime
def mod_inverse(e, phi):
for d in range(3, phi):
if (d * e) % phi == 1:
return d
return None
def generate_keypair(p, q):
n = p * q
phi = (p - 1) * (q - 1)
e = random.randrange(1, phi)
g = math.gcd(e, phi)
while g != 1:
e = random.randrange(1, phi)
g = math.gcd(e, phi)
d = mod_inverse(e, phi)
return ((e, n), (d, n))
def encrypt(pk, plaintext):
key, n = pk
cipher = [pow(ord(char), key, n) for char in plaintext]
return cipher
def decrypt(pk, ciphertext):
key, n = pk
plain = [chr(pow(char, key, n)) for char in ciphertext]
return ''.join(plain)
# Example usage
p = generate_prime(1000, 5000)
q = generate_prime(1000, 5000)
public, private = generate_keypair(p, q)
message = "Hello, RSA!"
encrypted_msg = encrypt(public, message)
decrypted_msg = decrypt(private, encrypted_msg)
print(f"Original message: {message}")
print(f"Encrypted message: {encrypted_msg}")
print(f"Decrypted message: {decrypted_msg}")
This implementation includes functions for generating prime numbers, creating key pairs, and performing encryption and decryption. While it demonstrates the core concepts of RSA, it’s important to note that real-world implementations would use much larger prime numbers and more sophisticated methods for ensuring security.
RSA in Practice: Strengths and Limitations
RSA has stood the test of time as a robust encryption algorithm, but like all cryptographic systems, it has both strengths and limitations that are important to understand.
Strengths of RSA
- Security: When implemented correctly with sufficiently large key sizes, RSA provides a high level of security. The difficulty of factoring large numbers makes it resistant to many types of attacks.
- Asymmetric Nature: The use of separate public and private keys allows for secure communication without the need to exchange secret keys beforehand.
- Digital Signatures: RSA can be used to create digital signatures, providing authentication and non-repudiation in digital communications.
- Widely Adopted: Its widespread use means that RSA is well-understood and has been thoroughly tested in real-world scenarios.
Limitations and Considerations
- Computational Intensity: RSA operations, especially key generation and decryption, can be computationally expensive, particularly with very large key sizes.
- Key Size Requirements: As computing power increases, larger key sizes are needed to maintain security, which can impact performance.
- Vulnerability to Certain Attacks: While generally secure, RSA can be vulnerable to specific types of attacks if not implemented correctly, such as padding oracle attacks or timing attacks.
- Quantum Computing Threat: The development of powerful quantum computers could potentially break RSA encryption, as quantum algorithms like Shor’s algorithm could efficiently factor large numbers.
RSA in the Context of Modern Cryptography
While RSA remains a cornerstone of many cryptographic systems, the field of cryptography continues to evolve. Here’s how RSA fits into the broader landscape of modern cryptography:
Hybrid Cryptosystems
In practice, RSA is often used in conjunction with symmetric encryption algorithms in what’s known as a hybrid cryptosystem. This approach leverages the strengths of both asymmetric and symmetric encryption:
- A symmetric key is generated for fast encryption of the actual message data.
- RSA is used to securely encrypt and transmit this symmetric key.
- The recipient uses their RSA private key to decrypt the symmetric key, which is then used to decrypt the message.
This hybrid approach offers the security benefits of RSA while mitigating its performance limitations for large data sets.
Post-Quantum Cryptography
With the looming threat of quantum computers, which could potentially break RSA encryption, there’s growing interest in post-quantum cryptography. These are cryptographic systems that are believed to be secure against both quantum and classical computers. While RSA may eventually be phased out for certain applications, the principles it established continue to influence the development of new cryptographic methods.
Ongoing Research and Improvements
Cryptographers continue to research and refine RSA implementations, focusing on areas such as:
- Optimizing performance for specific use cases
- Developing more efficient key generation algorithms
- Improving resistance to side-channel attacks
- Exploring variants of RSA that might offer additional security or performance benefits
Practical Applications of RSA
Understanding the theoretical aspects of RSA is crucial, but it’s equally important to recognize its practical applications in everyday technology. Here are some common uses of RSA in the real world:
1. Secure Communication Protocols
RSA is a key component in many secure communication protocols, including:
- HTTPS: Used for secure web browsing, where RSA often plays a role in the initial key exchange.
- SSL/TLS: These protocols, which underpin secure internet communications, frequently use RSA for key exchange and digital signatures.
- SSH (Secure Shell): Used for secure remote access to systems, often employing RSA for key-based authentication.
2. Email Encryption
Systems like PGP (Pretty Good Privacy) and S/MIME (Secure/Multipurpose Internet Mail Extensions) use RSA for encrypting email communications and creating digital signatures.
3. Digital Signatures
RSA’s ability to create unforgeable digital signatures makes it valuable for:
- Authenticating software updates
- Verifying the integrity of documents
- Implementing non-repudiation in financial transactions
4. VPNs (Virtual Private Networks)
Many VPN protocols use RSA for secure key exchange when establishing encrypted tunnels between clients and servers.
5. Smart Cards and Secure Hardware Tokens
RSA is often implemented in hardware security modules, smart cards, and secure tokens for authentication and secure data storage.
Implementing RSA Securely
While the basic principles of RSA are straightforward, implementing it securely requires careful consideration of several factors:
1. Key Size
The security of RSA largely depends on the size of the keys used. As of 2021, the minimum recommended key size for RSA is 2048 bits, with many organizations moving towards 4096-bit keys for long-term security.
2. Random Number Generation
The security of RSA relies heavily on the quality of the random numbers used in key generation. Always use cryptographically secure random number generators.
3. Padding Schemes
Simple implementations of RSA can be vulnerable to various attacks. Using proper padding schemes like OAEP (Optimal Asymmetric Encryption Padding) for encryption and PSS (Probabilistic Signature Scheme) for signatures is crucial for security.
4. Side-Channel Attack Prevention
Implementations should be resistant to side-channel attacks, which attempt to extract secret information through timing analysis, power consumption monitoring, or other indirect methods.
5. Key Management
Proper key management is critical. This includes secure key generation, storage, distribution, and retirement processes.
Future of RSA and Encryption
As we look to the future of cryptography, several trends and developments are worth noting:
1. Quantum Resistance
With the potential threat of quantum computers, there’s a growing focus on developing and standardizing quantum-resistant cryptographic algorithms. While RSA may eventually be replaced for certain applications, the principles it established continue to influence new cryptographic methods.
2. Homomorphic Encryption
Advances in homomorphic encryption, which allows computations to be performed on encrypted data without decrypting it, may lead to new applications and paradigms in secure computation.
3. Blockchain and Distributed Systems
The rise of blockchain technology and other distributed systems is driving innovation in cryptographic protocols, including new approaches to key management and consensus mechanisms.
4. AI and Cryptography
The intersection of artificial intelligence and cryptography is an emerging field, with potential applications in areas like automated vulnerability detection and cryptanalysis.
Conclusion
The RSA algorithm stands as a testament to the power of mathematical principles in solving real-world security challenges. Its elegant simplicity, coupled with its robust security properties, has made it a cornerstone of modern cryptography for over four decades.
As we’ve explored in this article, understanding RSA involves delving into number theory, modular arithmetic, and the practical considerations of implementing secure systems. While RSA may eventually be superseded by quantum-resistant algorithms, the fundamental concepts it embodies – the use of trapdoor functions, the power of asymmetric cryptography, and the importance of computational hardness assumptions – will continue to shape the future of secure communication and data protection.
For aspiring cryptographers, software engineers, and cybersecurity professionals, a deep understanding of RSA provides not just practical knowledge, but also a foundation for grasping the broader principles of cryptographic systems. As we continue to navigate an increasingly digital world, the lessons learned from RSA will undoubtedly play a crucial role in developing the next generation of secure communication technologies.